Complete Guide on Nest JS Caching | Redis
Summary
TLDRThis tutorial explores caching in NestJS, comparing in-memory caching to Redis for scalability and persistence. It demonstrates how to implement caching using the CacheManager package, set up Redis as a cache store, and utilize cache interceptors for automatic caching of data fetched from the database.
Takeaways
- 📒 Caching in NestJS can be implemented in multiple ways based on the project needs, primarily through in-memory caching and Redis.
- 📝 In-memory caching stores data directly in the application's memory, acting like a quick reference guide for fast access.
- 🚀 In-memory caching is fast and easy to set up, ideal for small-scale applications but has limitations with larger data volumes or multiple server instances.
- 🔄 Redis can be used for larger applications, ensuring consistency and efficiency by storing data in a dedicated data store.
- ⚙️ Implementing caching in a NestJS application involves installing the necessary packages and configuring the CacheModule.
- 🗂️ The CacheModule can be registered globally, making it available across the entire application, or locally within specific modules.
- 📥 Caching data with Redis involves setting up the Redis store in the CacheModule and configuring the host and port.
- 🔧 Caching interceptors can be used to automatically cache responses from specific routes or controllers in NestJS.
- 🔄 Redis provides benefits such as scalability and persistence, ensuring cache data is retained even if the application restarts or crashes.
- 📈 Using Redis for caching involves setting up a Redis container, interacting with Redis CLI, and verifying cached keys and data.
Q & A
What is caching in the context of a Node.js application?
-Caching in Node.js refers to the process of storing frequently accessed data in a temporary storage area to improve the performance of an application by reducing the need to fetch the data from its original source repeatedly.
What is the primary advantage of in-memory caching in Node.js?
-The primary advantage of in-memory caching is its speed. It allows for quick access to data stored directly in the application's memory, which is ideal for small-scale applications and does not require any external tools.
What limitations does in-memory caching have as an application grows?
-In-memory caching may struggle to keep up as the application grows due to its inability to handle large volumes of data or maintain consistency across multiple server instances.
What is Redis and how does it help overcome the limitations of in-memory caching?
-Redis is a data store that can be used for caching purposes. It helps overcome the limitations of in-memory caching by providing a shared, persistent, and scalable cache that can be accessed across different instances of an application.
What is the role of the 'CacheModule' in a NestJS application?
-The 'CacheModule' in NestJS is used to set up and configure caching within the application. It allows for the registration of various caching options and settings, such as the maximum number of keys and time-to-live (TTL) for cached data.
What does TTL stand for in the context of caching?
-TTL stands for 'Time To Live', which is the duration for which cached data is considered valid before it needs to be refreshed or expired.
How can you implement caching in a NestJS application?
-To implement caching in a NestJS application, you need to install the required packages, import the 'CacheModule' in the AppModule, register the caching options, and then inject the cache manager into your service classes to interact with the cache.
What is an 'Interceptor' in NestJS and how does it relate to caching?
-An 'Interceptor' in NestJS is a class annotated with the @Interceptor() decorator that can intercept incoming requests before they reach their respective handlers. In the context of caching, a cache interceptor can check for a cached response and return it if available, otherwise, it allows the request to proceed and caches the response for future requests.
How can you use Redis as a cache store in a NestJS application?
-To use Redis as a cache store in a NestJS application, you need to install the 'cache-manager-redis-store' package and its TypeScript type definitions. Then, configure the 'CacheModule' to use Redis by setting the 'store' property to 'RedisStore' and providing the host and port of the Redis instance.
What is the purpose of using a Docker container for running Redis?
-Using a Docker container for running Redis allows for easy deployment, isolation, and management of the Redis service. It ensures that the Redis cache store is separate from the application environment, promoting better resource management and consistency.
How can you verify if data is being cached in Redis?
-You can verify if data is being cached in Redis by using the Redis CLI (Command Line Interface) to connect to the Redis instance and executing the 'KEYS' command to list all cached keys or the 'GET' command to retrieve the cached data for a specific key.
Outlines
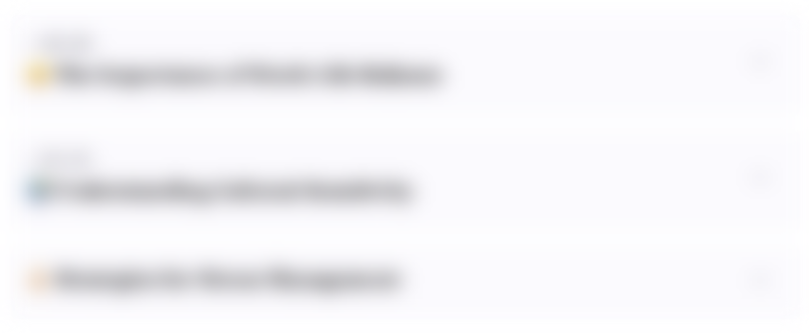
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
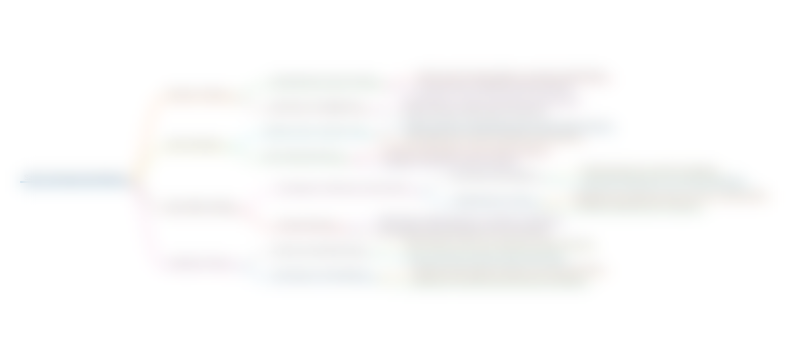
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
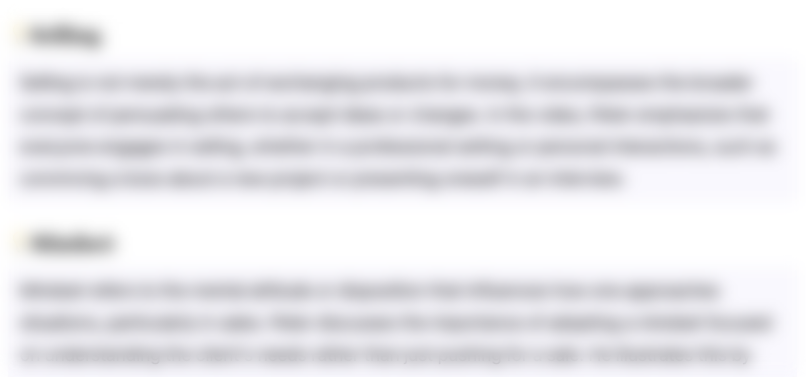
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
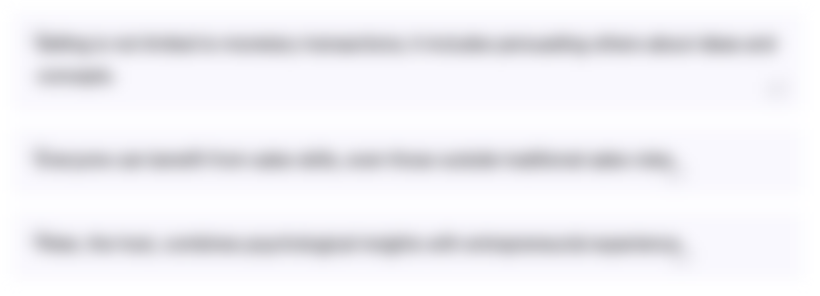
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
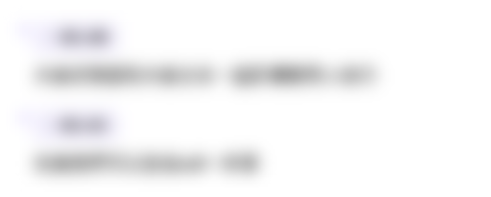
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados

Redis Tutorial for Beginners #1 - What is Redis?

Belajar Redis - 2 Kapan Butuh Redis?

Cache Systems Every Developer Should Know

Caching in ASP.NET C# - Memory Caching is AMAZING
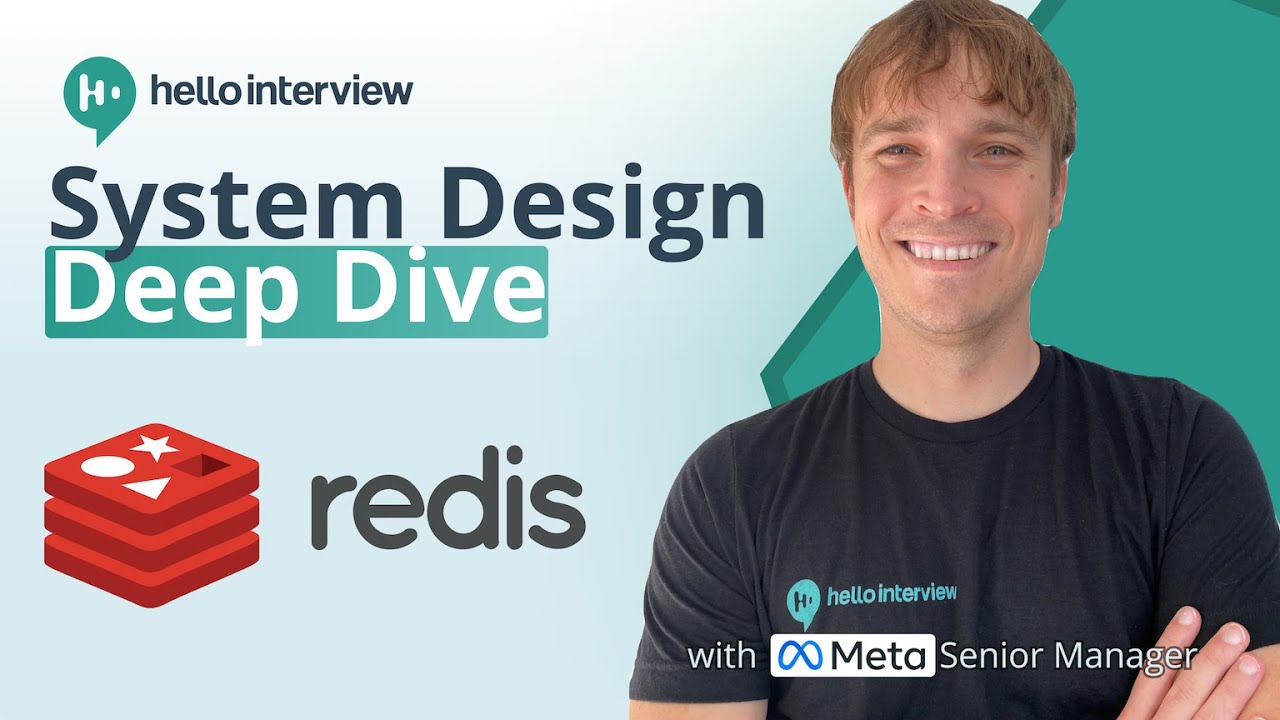
Redis Deep Dive w/ a Ex-Meta Senior Manager
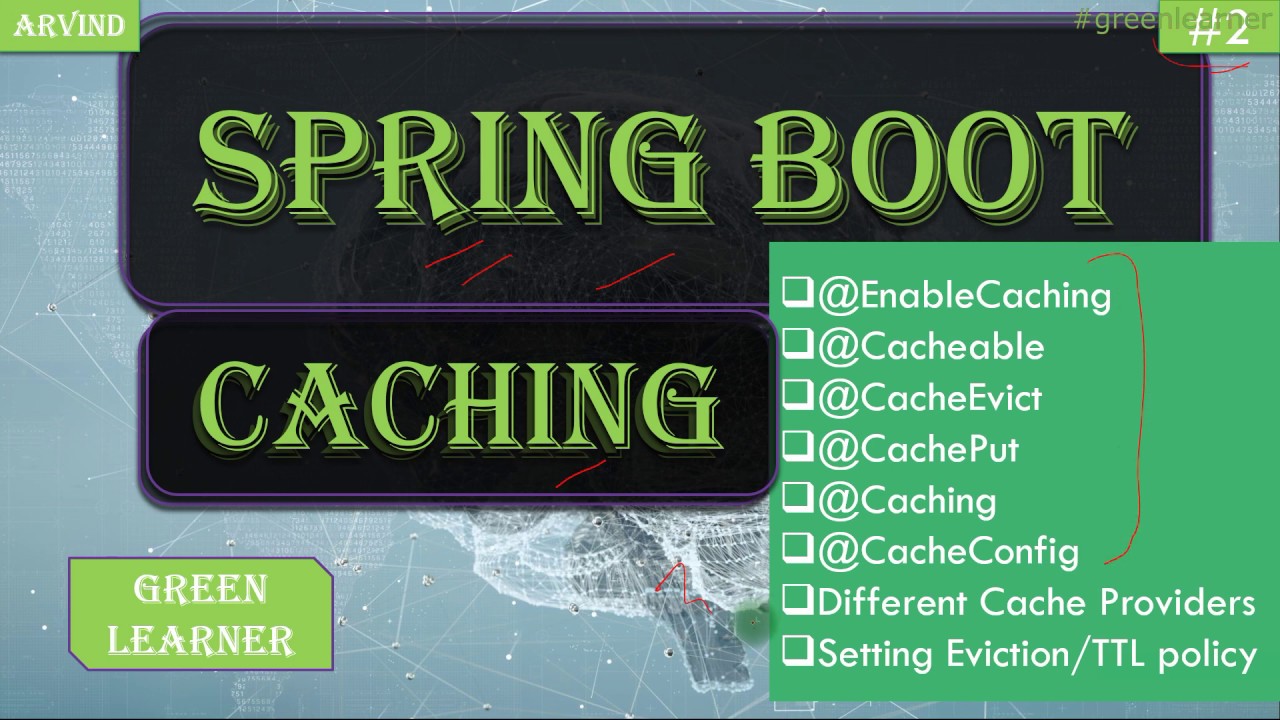
Spring Boot Cache Annotations || Cache Providers || Where to set Caching Policy || Green Learner
5.0 / 5 (0 votes)