HARD React Interview Questions (3 patterns)
Summary
TLDRIn this video, the speaker discusses advanced React interview questions focused on component design, covering three key patterns: Higher-Order Components (HOCs), Render Props, and Custom Hooks. The speaker explains how each pattern helps developers write clean, reusable, and modular code. HOCs allow for code sharing by wrapping components, Render Props facilitate sharing behavior between components via functions, and Custom Hooks help reuse stateful logic in functional components. The video emphasizes the importance of understanding and applying these patterns in real-world scenarios, encouraging candidates to learn through practical experience rather than just interview preparation.
Takeaways
- 😀 HOCs (Higher Order Components) allow you to reuse component logic by taking a component as an input and returning a wrapped version with added props or behavior.
- 😀 The purpose of a Higher Order Component (HOC) is to share logic like API calls across multiple components, avoiding repetitive code.
- 😀 The keyword `with` is commonly used as a prefix in HOCs (e.g., `withRouter`) as a convention, though not a requirement.
- 😀 Render Props allow sharing state and behavior between components by passing a function as a prop, which the receiving component uses to render output.
- 😀 A render prop can help pass state, such as mouse position, from one component (e.g., `Mouse`) to another (e.g., `Cat`) to share behavior.
- 😀 It's important to not only understand these patterns but to use them in real-world projects to build a deeper understanding and improve coding practices.
- 😀 Custom Hooks allow you to extract and reuse logic across functional components, promoting modularity and code reusability.
- 😀 Custom Hooks are regular JavaScript functions that return logic, and they must follow the `use` prefix convention (e.g., `useCustomHook`).
- 😀 Custom Hooks don't share state between components; each component using the same hook gets its own independent state, promoting encapsulation.
- 😀 When writing a custom hook, it's helpful to follow React's pattern of returning the value and its setter function in an array to keep things consistent.
Q & A
What is a Higher-Order Component (HOC) in React?
-A Higher-Order Component (HOC) is a function that takes a component and returns a new component with additional props or functionality. It allows for code reuse and modularity by sharing logic or behaviors between components without modifying their original structure.
How does a Higher-Order Component (HOC) help in code reuse?
-HOCs help in code reuse by allowing common logic or functionality (such as making an API call) to be abstracted into a reusable component. This logic can then be passed down to other components, reducing redundancy in the codebase.
What is the naming convention for Higher-Order Components in React?
-While not strictly required, it is a common convention to prefix Higher-Order Components with the word 'with', such as 'withRouter' or 'withAuth'. This convention helps make it clear that the component is a higher-order component.
Can you explain what a render prop is and how it works in React?
-A render prop is a function that is passed as a prop to a component and is used to determine what that component should render. It enables sharing logic or state between components in a flexible way, allowing the receiving component to customize its rendering behavior based on the function passed in.
What are some common use cases for render props in React?
-Render props are commonly used when you want to share behavior or state across multiple components. A classic example is a `Mouse` component that shares the mouse position with other components, like a `Cat` component, allowing the cat to follow the mouse.
How do render props compare to Higher-Order Components in React?
-While both patterns enable code reuse and sharing of logic, render props are often considered more flexible than Higher-Order Components. Render props allow the component receiving the prop to control what is rendered, while HOCs wrap the component to inject additional functionality.
What is the purpose of custom hooks in React?
-Custom hooks allow you to extract and reuse stateful logic across multiple functional components. They are designed to keep the logic modular and make the codebase more maintainable by avoiding duplication of logic in individual components.
What are the naming conventions for custom hooks in React?
-Custom hooks should be named with the 'use' prefix, such as `useFetch`, `useInput`, or `useTimer`. This is a React convention to indicate that the function is a hook and can be used to manage state or side effects in functional components.
How does React ensure that components using the same custom hook don't share state?
-Each component that uses a custom hook gets its own independent state. React manages this by associating the state with the specific component instance. Even if multiple components use the same custom hook, they will each have their own isolated state.
Why is it important to not only know advanced React patterns but also to apply them in real projects?
-Understanding advanced React patterns is crucial, but applying them in real-world projects helps solidify your understanding. It allows you to grasp the nuances of how these patterns work in practice, and it also improves your ability to explain and use them effectively in an interview setting or in your development work.
Outlines
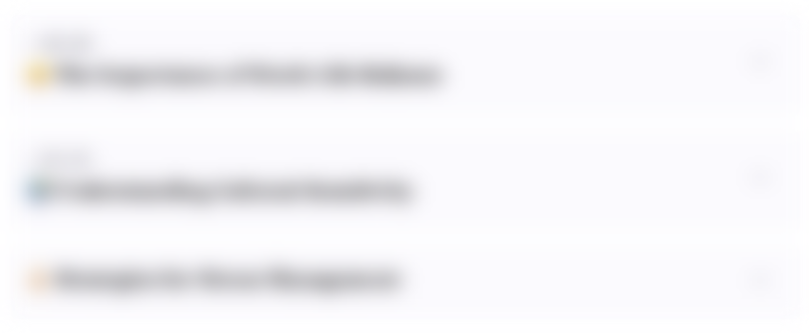
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
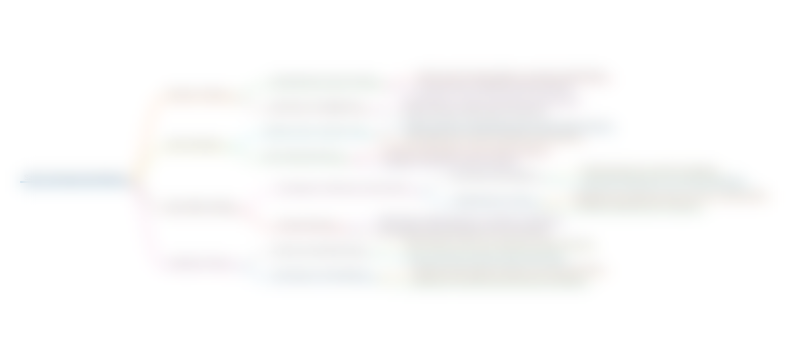
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
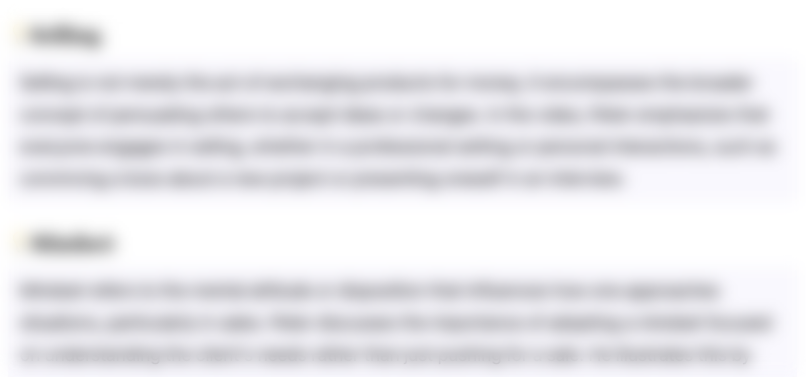
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
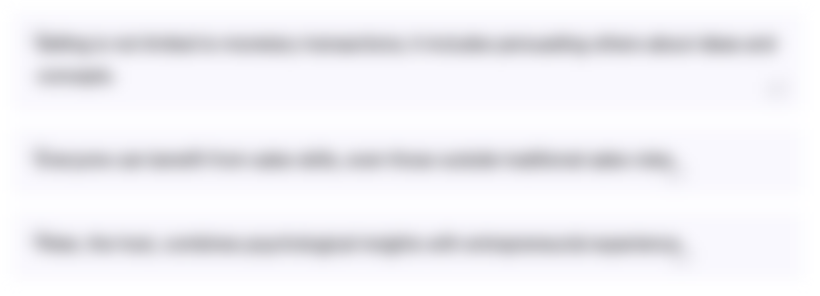
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
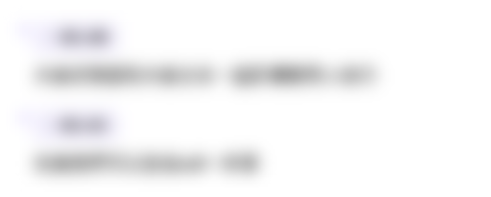
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
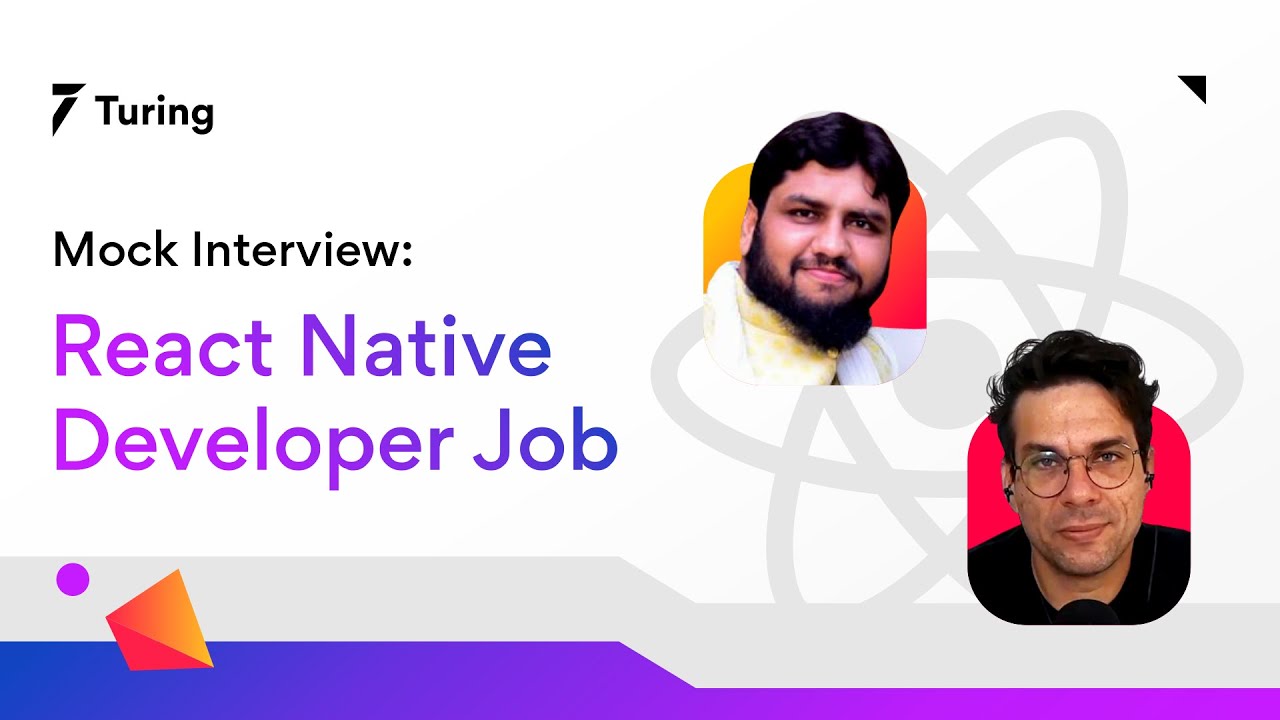
React Native Mock Interview | Interview Questions for Senior React Native Developers
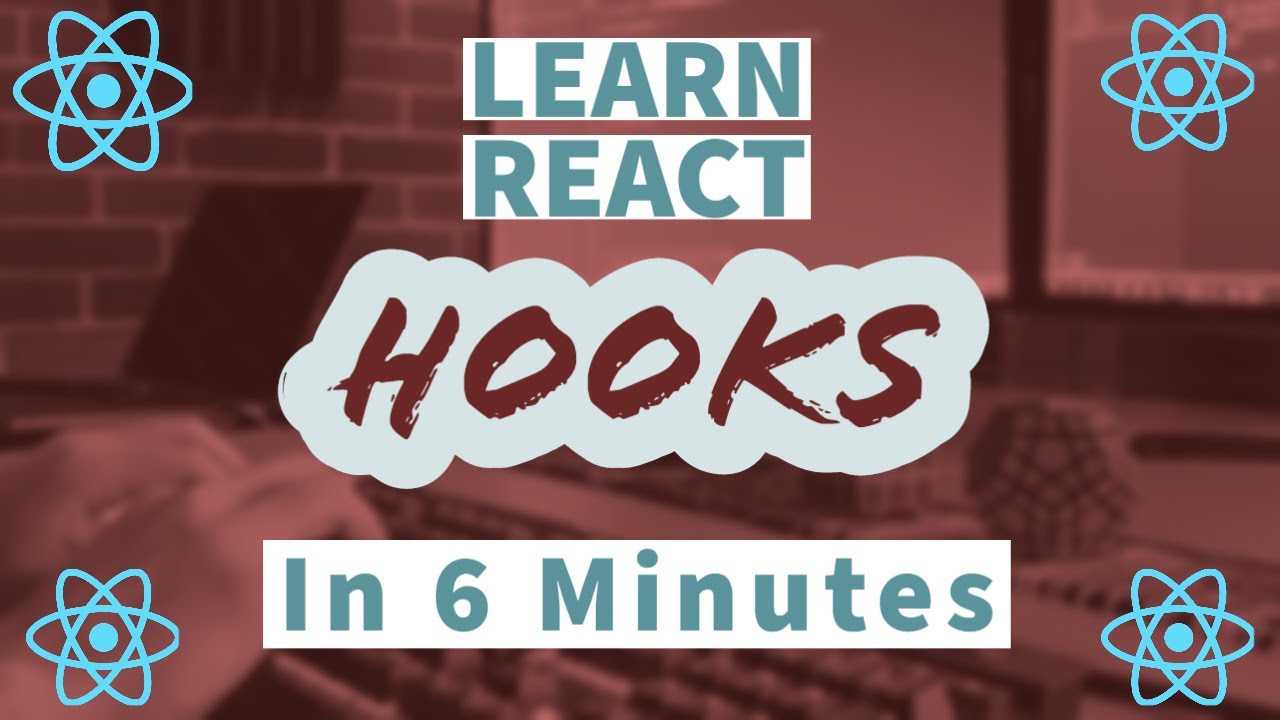
Learn React Hooks In 6 Minutes
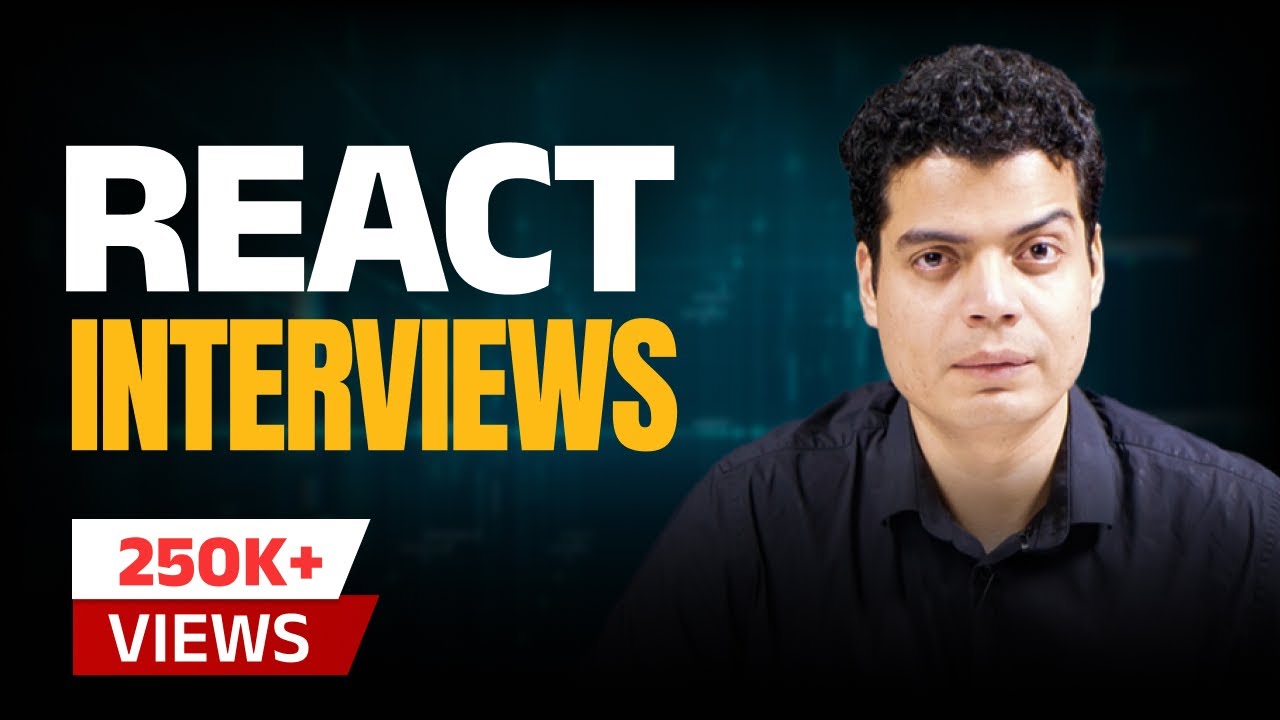
Mastering React: Decoding 09 Most Common Interview Questions | Tanay Pratap Hindi

React Hooks and Their Rules | Lecture 157 | React.JS 🔥
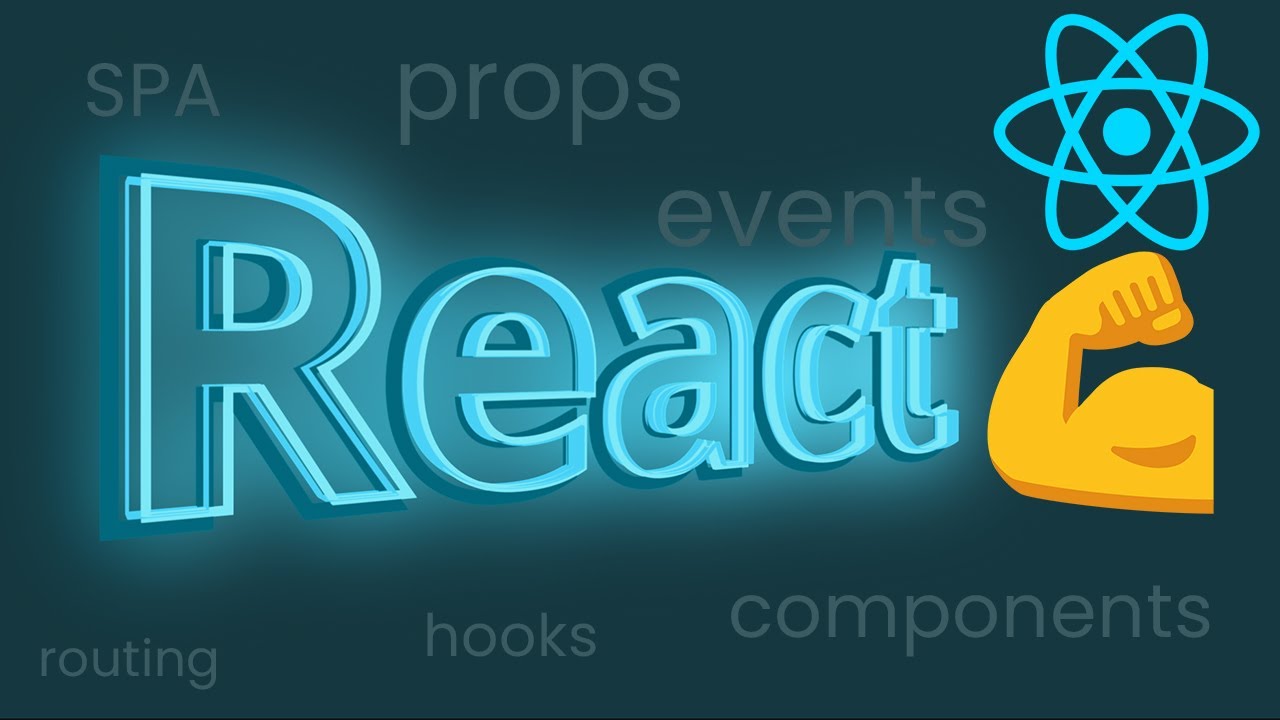
React JS Explained In 10 Minutes
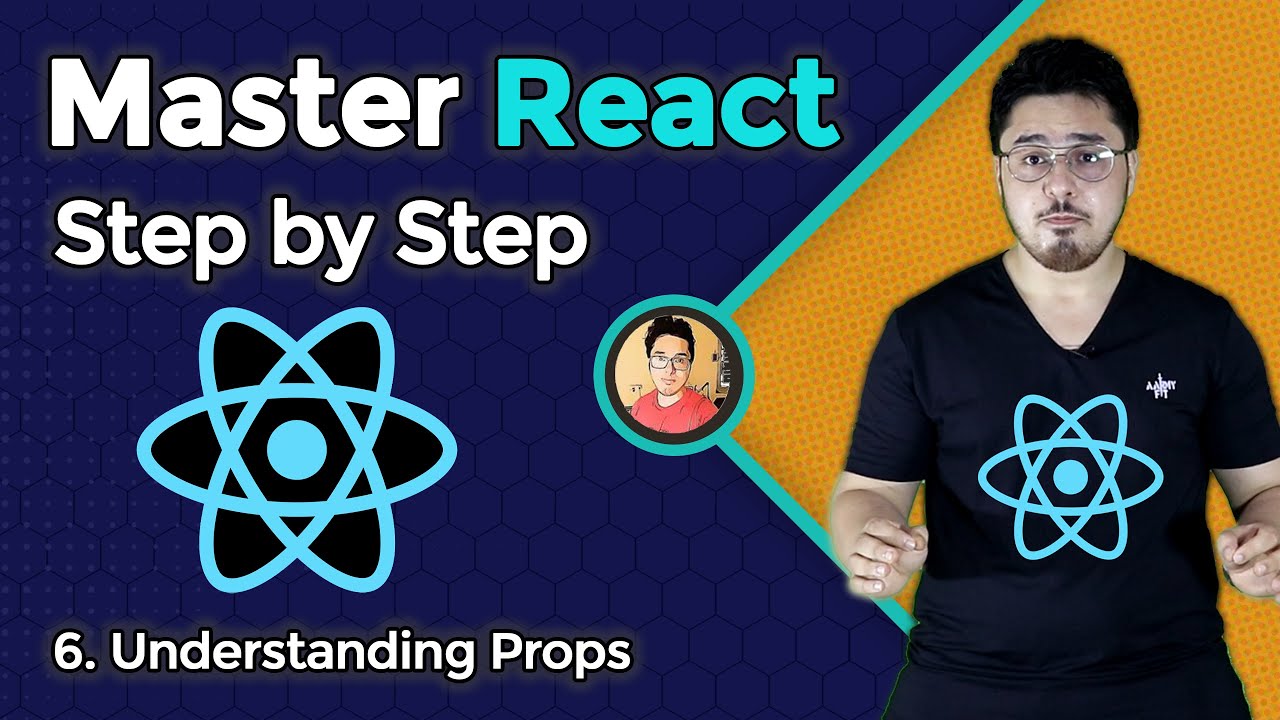
Understanding Props and PropTypes in React | Complete React Course in Hindi #6
5.0 / 5 (0 votes)