C++ 24 | Pola Persegi | Belajar Looping C++
Summary
TLDRThis C++ tutorial demonstrates how to create a rectangular pattern using loops. The tutorial guides viewers through setting up the program, defining variables for rows and columns, and using nested `for` loops to print stars both vertically and horizontally. It also shows how to handle user input to adjust the number of rows and columns dynamically. The video emphasizes debugging techniques to ensure the correct number of stars are displayed and wraps up by previewing more advanced pattern creation in future tutorials. A great introduction to using loops for graphical output in C++ programming.
Takeaways
- 😀 Introduction to creating patterns in C++ using loops.
- 😀 Basic setup in C++: using `#include` and defining the `main()` function.
- 😀 Explanation of the need for variables to control rows and columns of the pattern.
- 😀 How to print a simple rectangular pattern using nested `for` loops.
- 😀 Demonstration of how the outer loop controls rows and the inner loop controls columns.
- 😀 Introduction to dynamic input via the `cin` command to adjust the number of rows and columns.
- 😀 The `for` loop structure explained: how the loop variable is incremented and checked against the limit.
- 😀 How nested loops are used to print a grid-like pattern, first filling the rows, then the columns.
- 😀 The importance of using `endl` to move to the next line after printing each row.
- 😀 How user input affects the size of the pattern, with examples of dynamic row and column input.
- 😀 A step-by-step breakdown of the program's logic and how loops interact to generate the pattern.
- 😀 Encouragement to experiment with different shapes and pattern designs using similar loop structures.
Q & A
What is the main objective of the tutorial?
-The main objective of the tutorial is to teach how to create a rectangular pattern using loops in C++.
Which C++ feature is primarily used to create the pattern in the tutorial?
-The tutorial primarily uses the concept of 'for' loops to create the pattern by iterating through rows and columns.
How are rows and columns defined in the basic implementation of the program?
-In the basic implementation, the number of rows and columns is defined using integer variables, initially set to 3 each.
What is the significance of the 'for' loop in the context of this program?
-The 'for' loop is used to iterate through both rows and columns, controlling the number of times the pattern characters (asterisks) are printed.
How does the nested 'for' loop differ from a single loop in the pattern creation?
-The nested 'for' loop allows for two levels of iteration—one for rows and another for columns—enabling the creation of a rectangular pattern instead of just printing a single row of characters.
What happens when the program is modified to accept user input for the number of rows and columns?
-When user input is accepted, the program dynamically adjusts the number of rows and columns based on the values entered by the user, allowing for custom-sized patterns.
What would be the output if the user inputs '5' for rows and '7' for columns?
-The output would be a rectangular pattern with 5 rows and 7 columns, each filled with asterisks ('*').
Why is 'cout' used in the program, and what does it do?
-'cout' is used to display output on the screen. It is used in the program to print the asterisks for the pattern and to print text, such as prompts for user input.
What is the role of the 'endl' statement in the code?
-'endl' is used to insert a newline after each row of the pattern, ensuring that the asterisks are printed on separate lines, forming a grid structure.
How can the program be expanded to print more complex patterns, such as triangles or other shapes?
-The program can be expanded by modifying the loop conditions and print statements. For example, to create a triangle, you could adjust the number of columns printed based on the row number in the outer loop.
Outlines
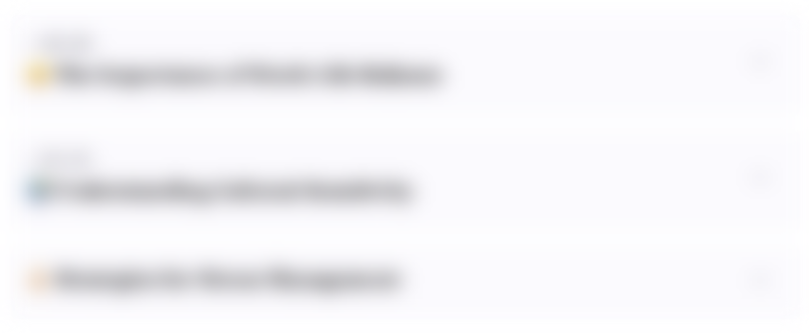
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
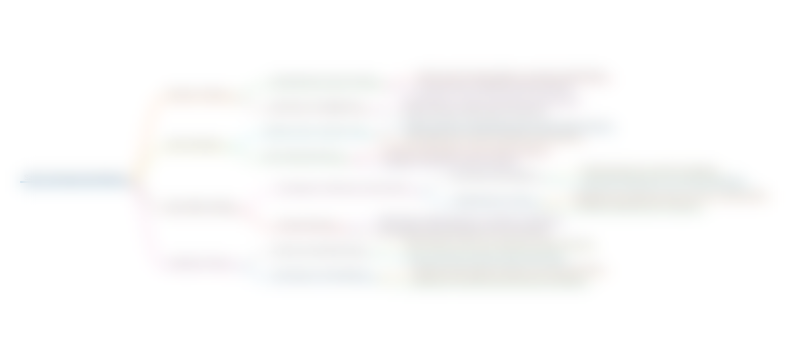
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
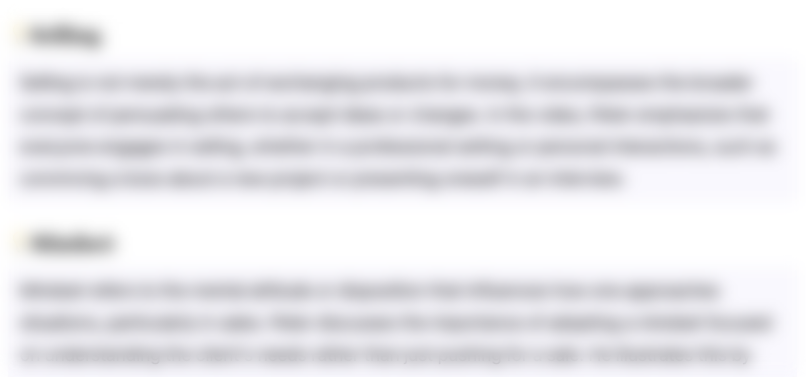
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
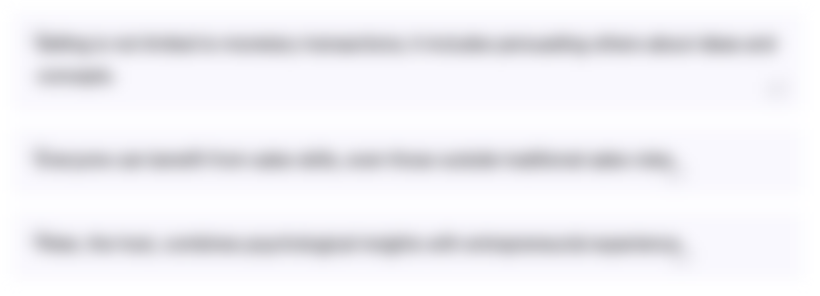
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
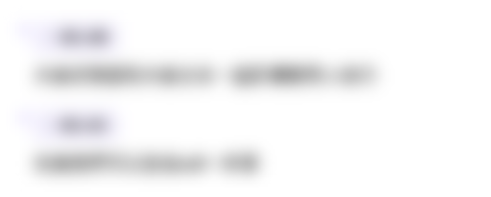
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
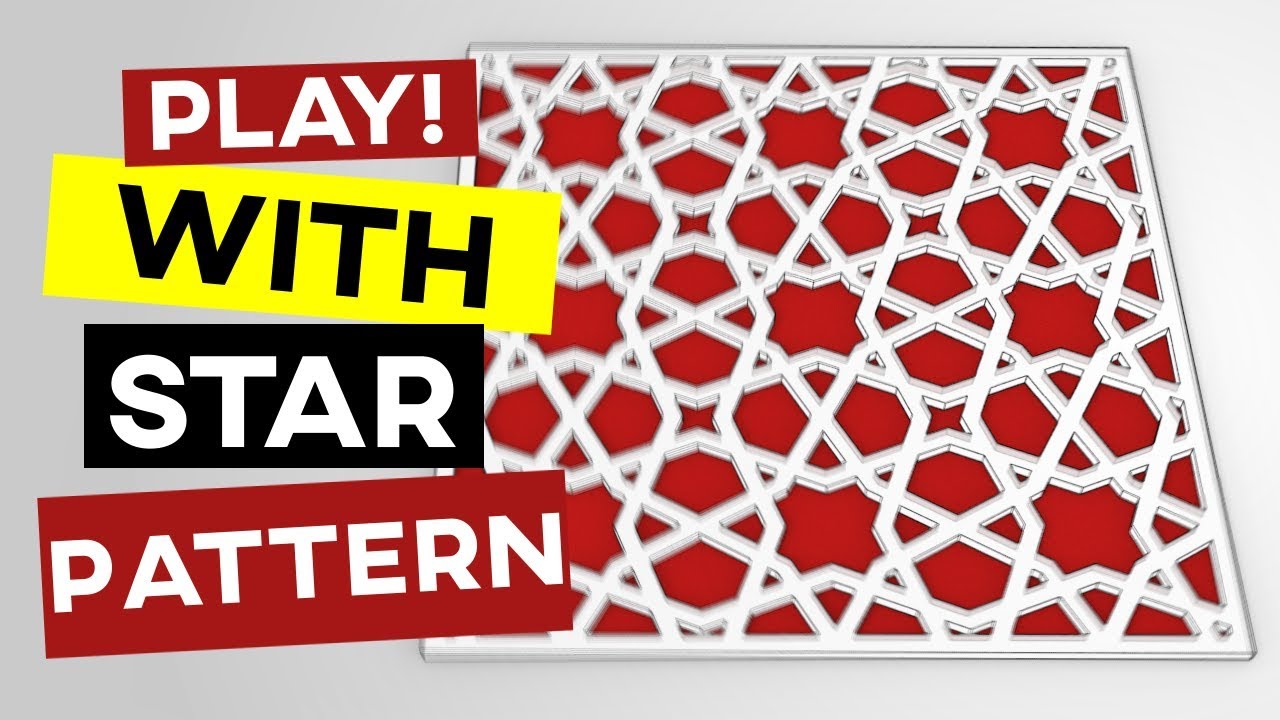
Islamic Geometric Pattern (Grasshopper Tutorial)
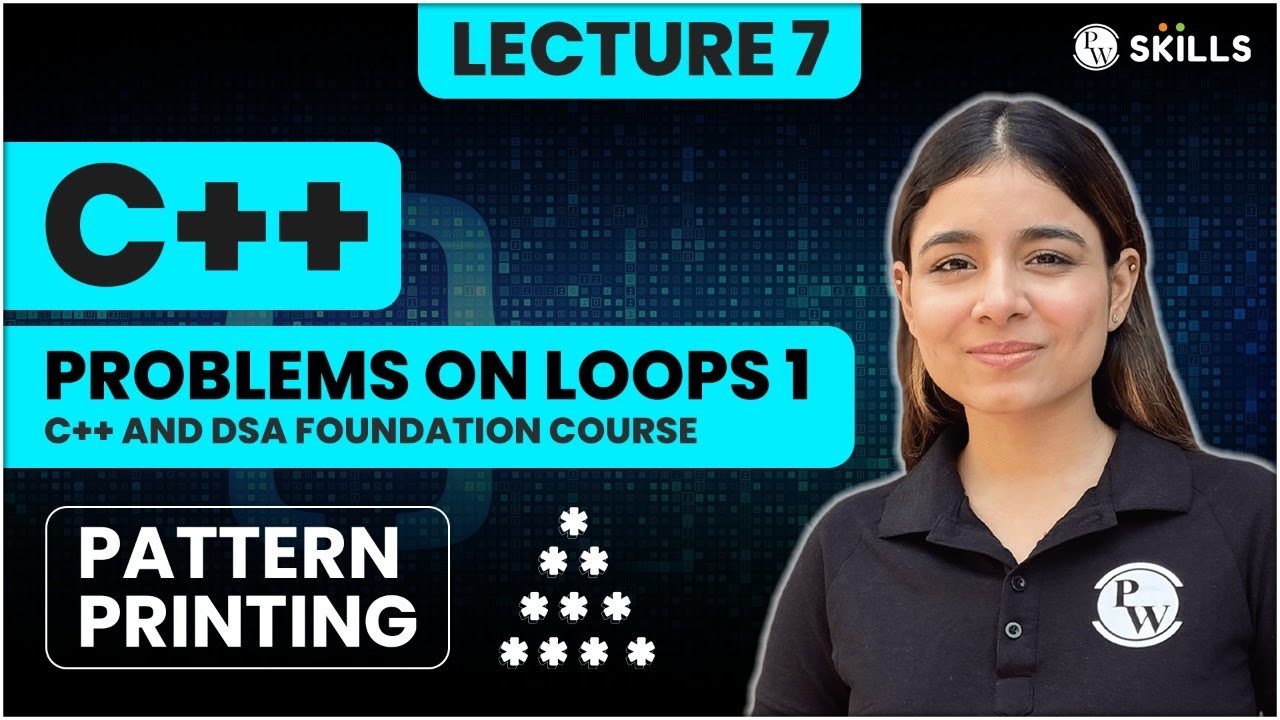
Pattern Printing | Problems on Loops - Part 1 | Lecture 7 | C++ and DSA Foundation Course
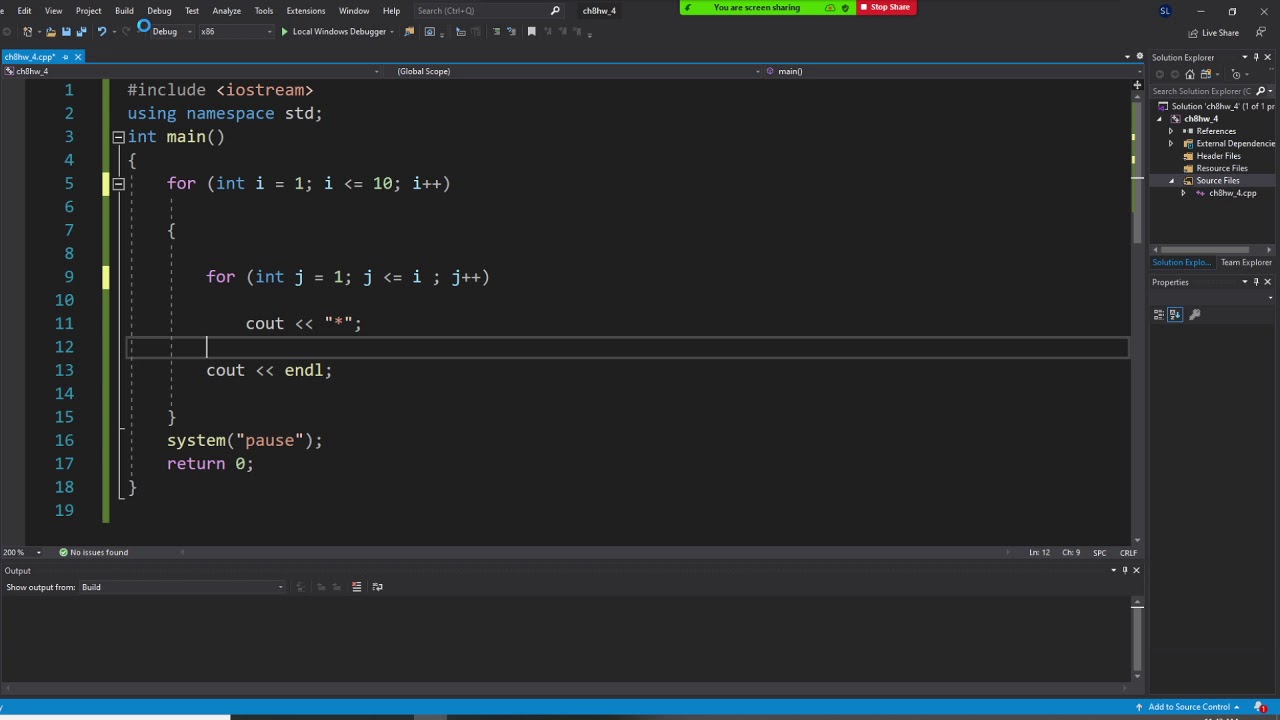
C++ programming, use double for loop to create patterns
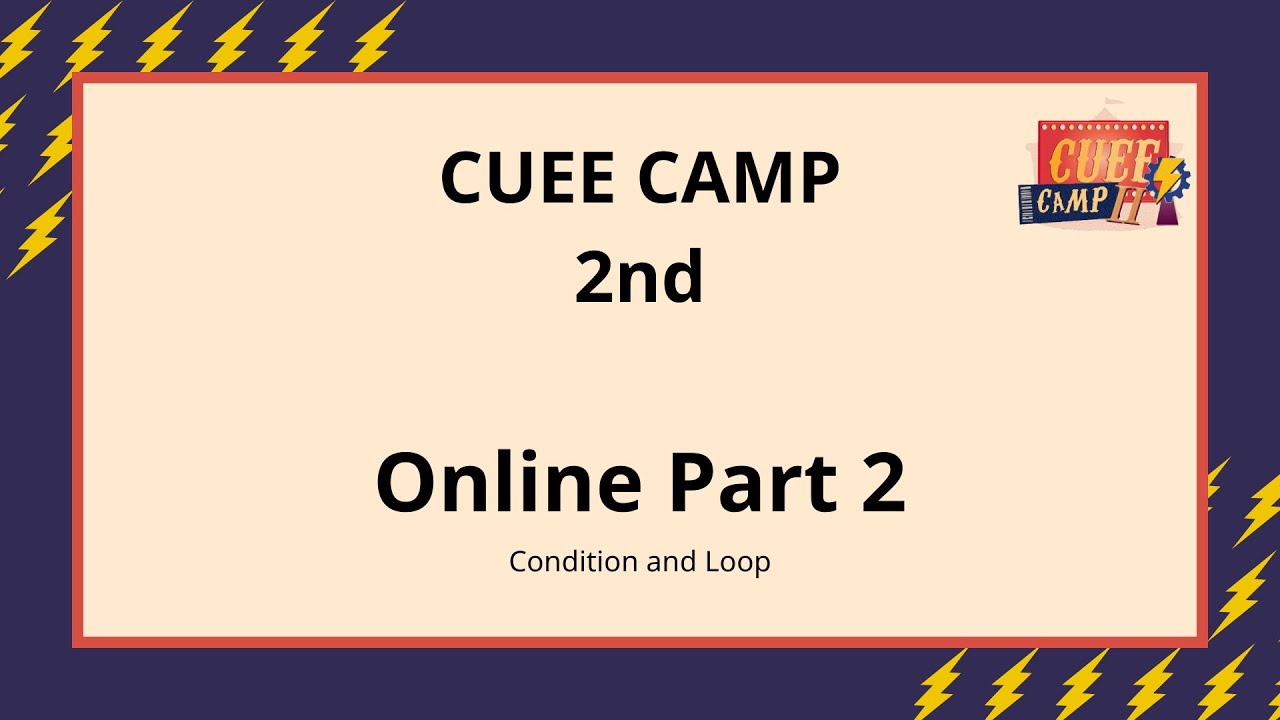
[CUEE Camp 2nd] Part 2 : Condition and Loop
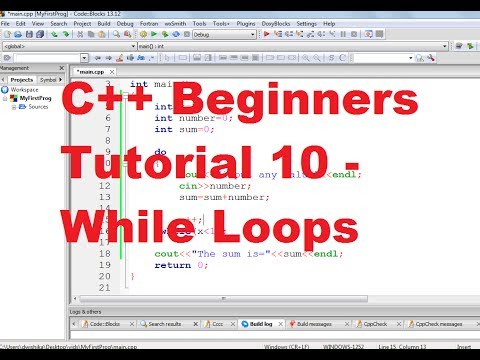
C++ Tutorial for Beginners 10 - While Loops
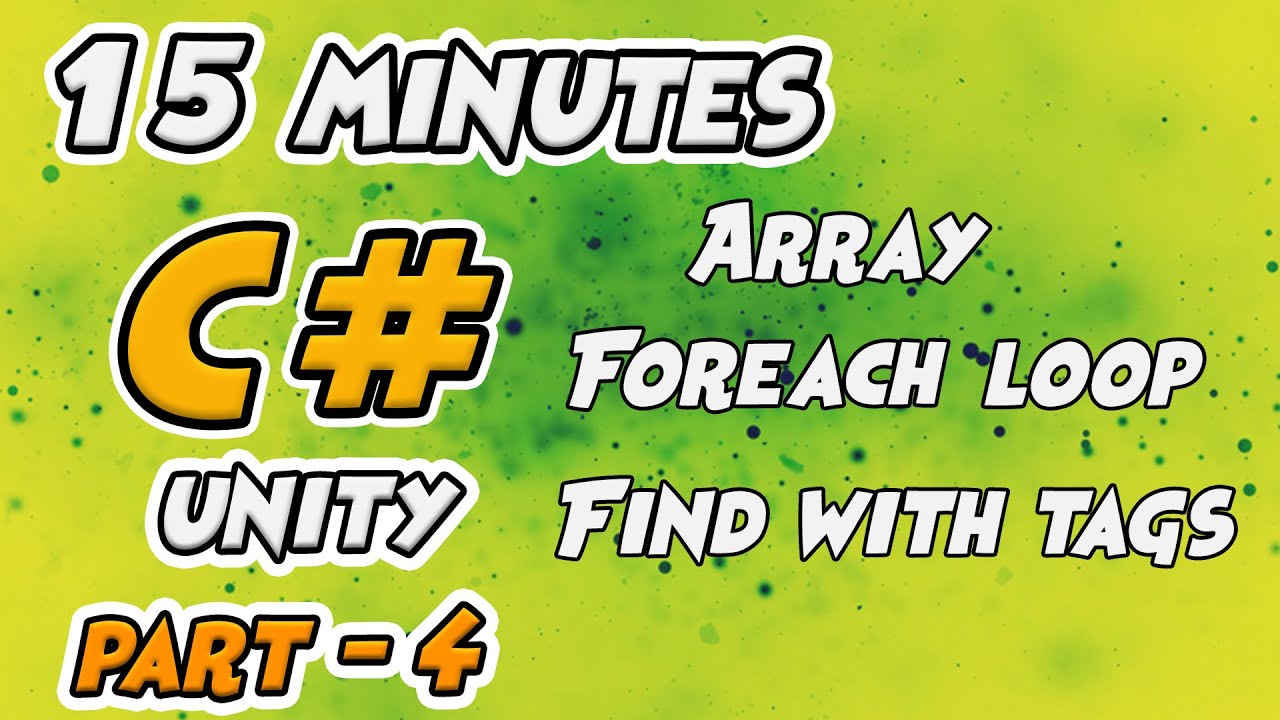
Learn C# Scripting for Unity 15 Minutes - Part 4 - Foreach Loop, Array, Find Tags
5.0 / 5 (0 votes)