C_15 Operators in C - Part 3 | C Programming Tutorials
Summary
TLDRIn this video, the presenter explains the increment (`++`) and decrement (`--`) operators in C programming, focusing on both prefix and postfix forms. The concepts are clarified through examples, showing how these operators modify variable values and how their precedence and associativity work in expressions. The video further discusses using these operators with both integers and floating-point numbers, as well as combining them in complex expressions. With clear examples and an easy-to-follow program walkthrough, viewers can grasp the fundamentals and nuances of these operators to enhance their C programming skills.
Takeaways
- 😀 Increment and decrement operators in C are unary operators that modify a variable's value by 1, either increasing (++) or decreasing (--) it.
- 😀 These operators can be used in two forms: prefix (e.g., ++x, --x) and postfix (e.g., x++, x--).
- 😀 The prefix form increments or decrements the variable first, and then the updated value is used in the expression.
- 😀 The postfix form uses the original value of the variable first, then increments or decrements the variable afterwards.
- 😀 The increment (`++`) and decrement (`--`) operators can be applied to both integer and floating-point variables.
- 😀 In the case of prefix increment, the value is modified before it is used in the expression, leading to the updated value being assigned.
- 😀 In postfix increment, the original value is used first, and only after that is the variable updated.
- 😀 When using both prefix and postfix operators in an expression, understanding their precedence and associativity is essential to predicting the output.
- 😀 Increment and decrement operators have higher precedence than the assignment operator (`=`), which means they execute before the assignment happens.
- 😀 The associativity of the increment and decrement operators is right-to-left, meaning when multiple operators are used, they are evaluated from right to left.
- 😀 Understanding and using these operators correctly in C can significantly impact the logic and outcome of programs, especially in loops or iterative structures.
Q & A
What are the two main types of increment and decrement operators in C?
-The two main types are prefix and postfix increment/decrement operators. In prefix, the operator is applied before the operand, while in postfix, it is applied after the operand.
How does the prefix increment operator (++x) work in an expression?
-In prefix notation, the operand's value is first incremented, and then the updated value is used in the expression.
What is the difference between prefix and postfix increment operators?
-In prefix, the operand's value is incremented first, and then used. In postfix, the original value of the operand is used first, and then it is incremented.
What happens when you use a postfix increment operator (x++) in an expression?
-The original value of the operand is used in the expression first, and then the operand is incremented after the expression is evaluated.
Can you use increment/decrement operators with float values in C?
-Yes, you can use increment and decrement operators with float values in C, though it's more common with integers.
How does the postfix decrement operator (x--) behave in an expression?
-In postfix notation, the original value of the operand is used in the expression first, and then the operand is decremented after the expression is evaluated.
What will happen if you use the expression y = x++ where x is 10?
-The original value of `x` (10) will be assigned to `y`, and then `x` will be incremented to 11.
What is the precedence of the increment and decrement operators compared to assignment operators?
-Increment and decrement operators have higher precedence than assignment operators. This means the increment or decrement operation is performed before the assignment.
What is the associativity of increment and decrement operators?
-The associativity of increment and decrement operators is right to left. This means that when multiple increment/decrement operators are used in an expression, they are evaluated from right to left.
How would you rewrite the expression y = x++ using an equivalent statement with a decrement operator?
-You can rewrite `y = x++` as `y = x; x = x + 1;` where `y` gets the original value of `x` and `x` is incremented afterward.
Outlines
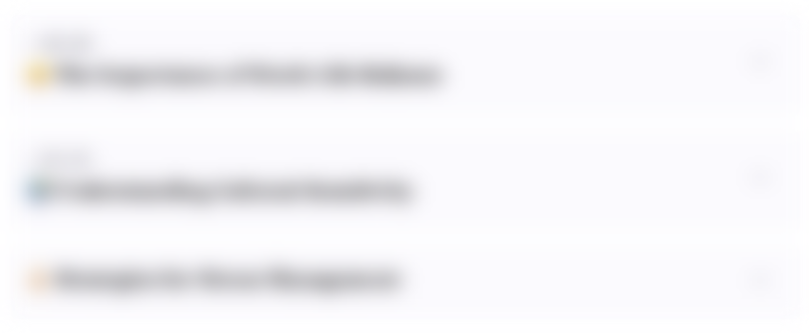
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
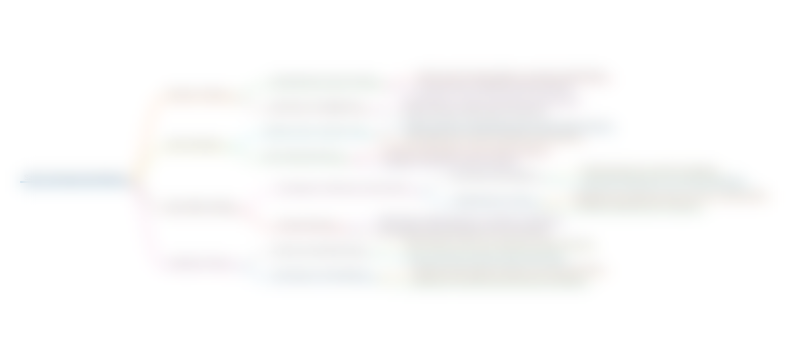
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
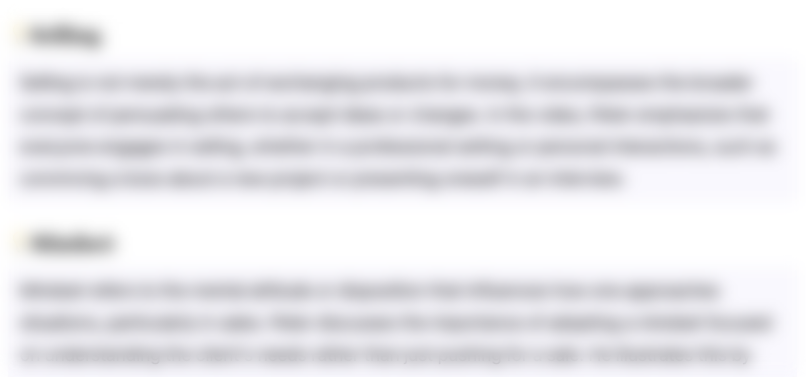
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
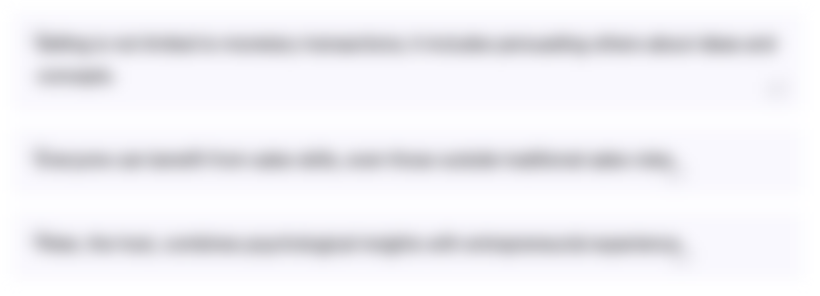
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
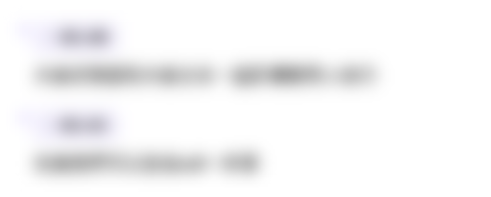
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
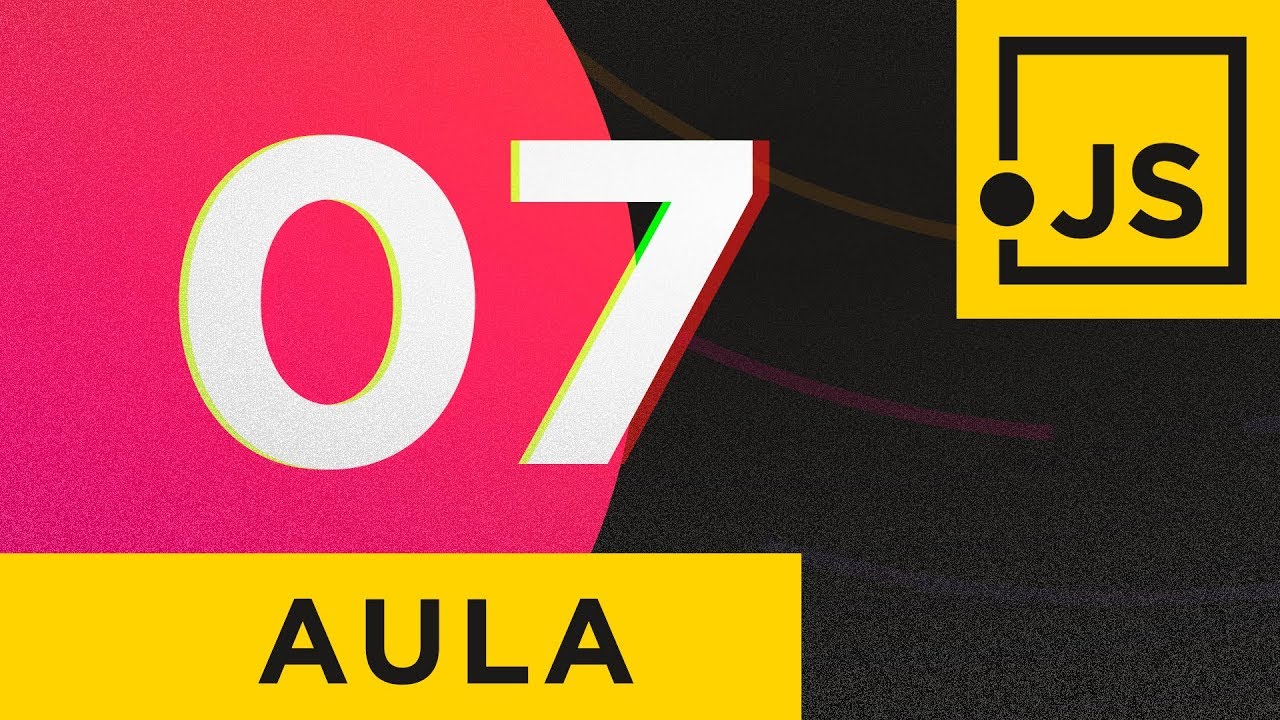
Operators (Part1) - JavaScript Course #07
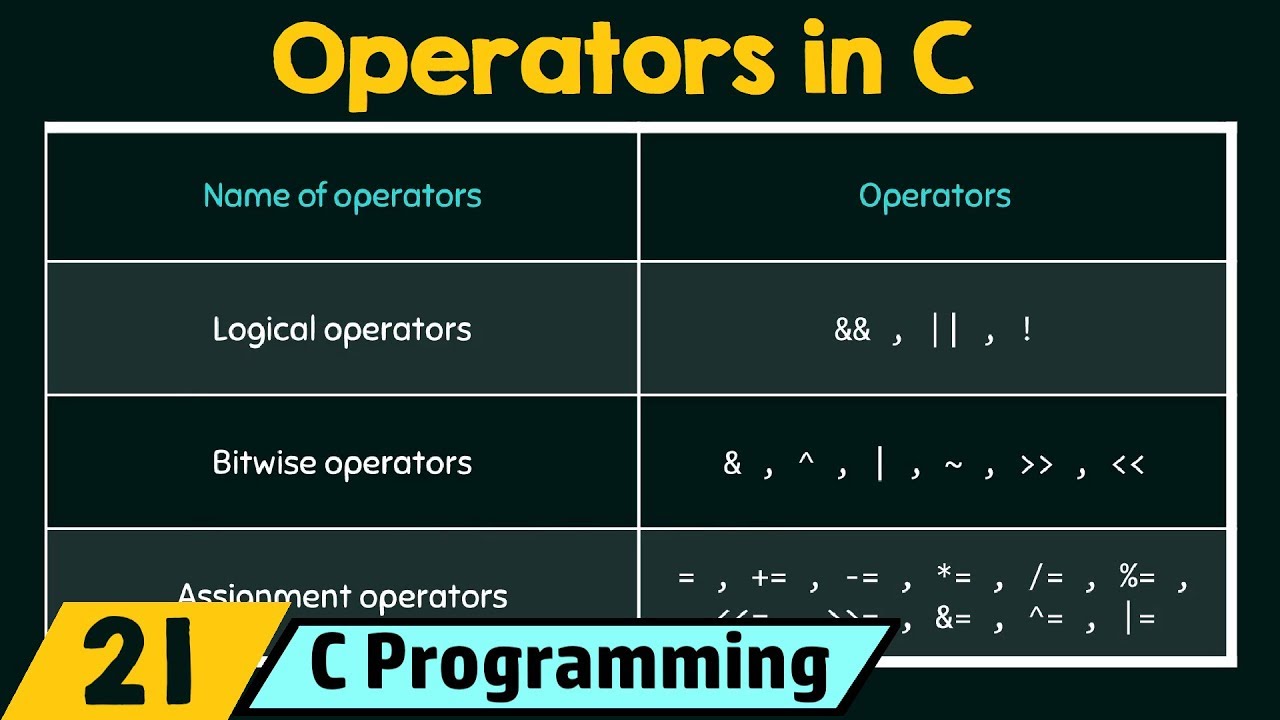
Introduction to Operators in C
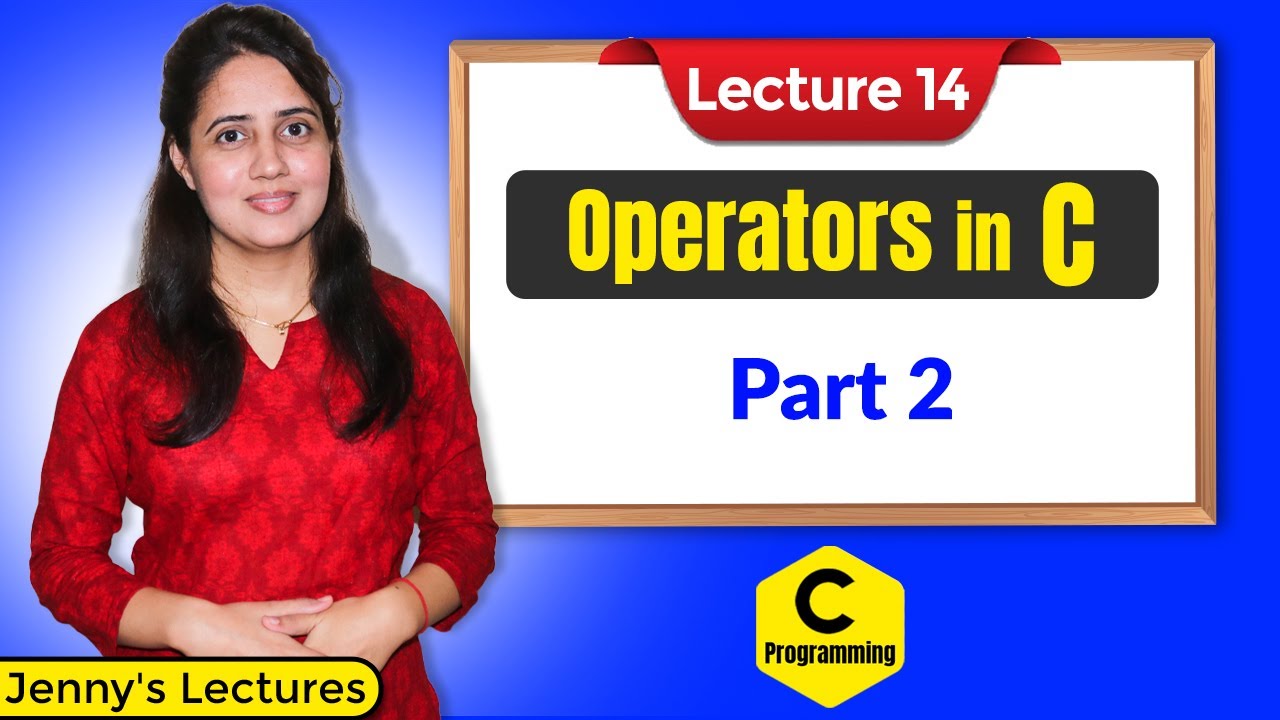
C_14 Operators in C - Part 2 | Arithmetic & Assignment Operators | C Programming Tutorials
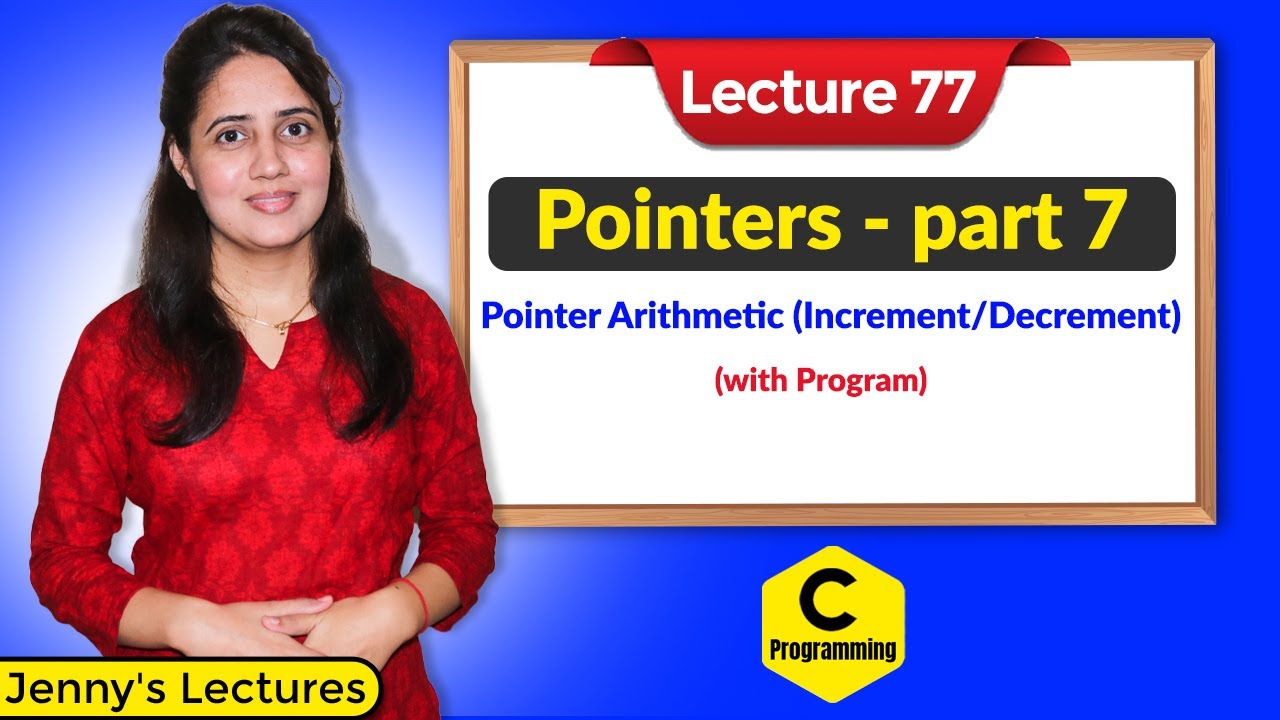
C_77 Pointers in C- part 7 | Pointer Arithmetic (Increment/Decrement) program

#42 Belajar Perulangan For C++
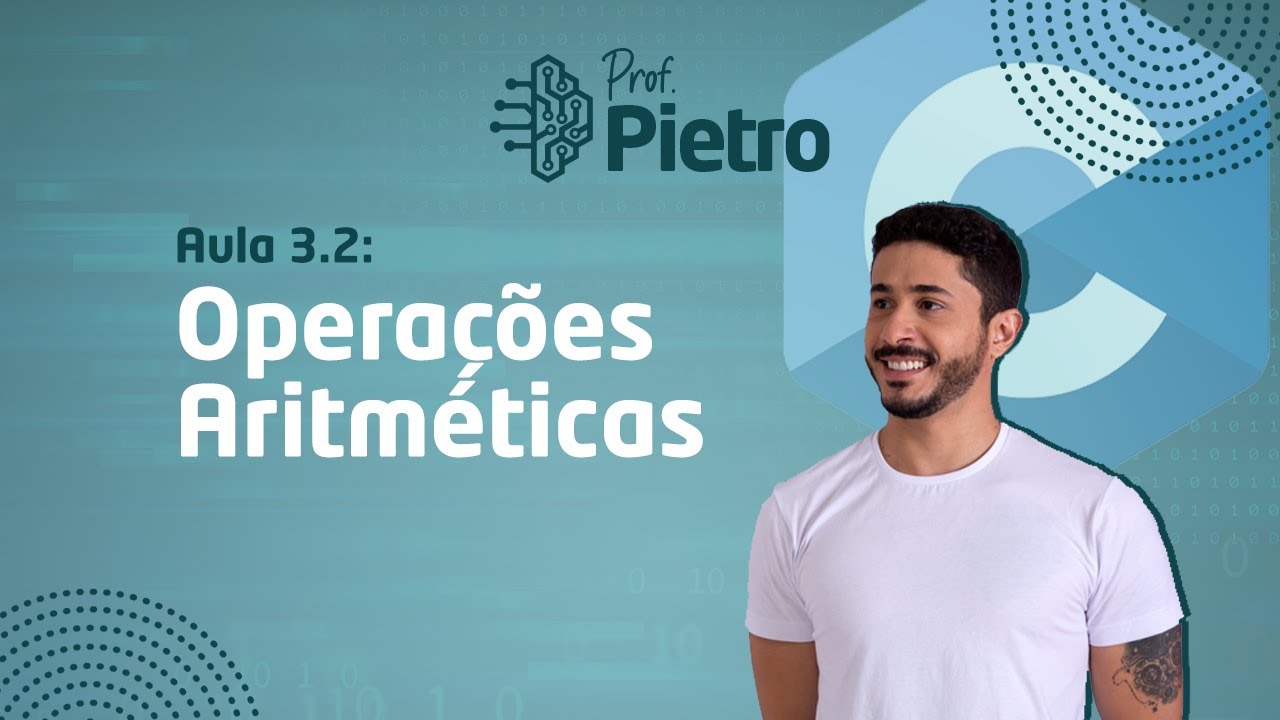
Linguagem C - Aula 3.2 - Aprenda a realizar cálculos em C (2022)
5.0 / 5 (0 votes)