Java Programming Tutorial - 49 - Inheritance
Summary
TLDRIn this Java tutorial on inheritance, the instructor explains how subclasses can inherit methods and variables from a superclass, reducing redundancy in code. Using examples like `Tuna` and `Potpie`, he demonstrates how to implement inheritance with the `extends` keyword and how to override methods for specific implementations. The video also covers class hierarchies and emphasizes that only public methods can be inherited, while private methods remain inaccessible. This foundational understanding of inheritance is essential for efficient object-oriented programming.
Takeaways
- 😀 Inheritance allows one class to inherit properties and methods from another, promoting code reuse.
- 😀 The tutorial uses `Tuna` and `Potpie` classes as examples to illustrate the concept of inheritance.
- 😀 By creating a superclass called `Food`, common methods like `eat` can be defined once and reused in subclasses.
- 😀 Changes to methods only need to be made in the superclass, reducing maintenance effort across subclasses.
- 😀 Subclasses are defined using the `extends` keyword, indicating that they inherit from a superclass.
- 😀 Even if a method isn't explicitly defined in a subclass, it can still be accessed if inherited from the superclass.
- 😀 Method overriding allows subclasses to provide specific implementations for inherited methods, offering flexibility.
- 😀 Hierarchical inheritance is possible; a subclass can extend another subclass, inheriting from both it and its superclass.
- 😀 Only public methods can be inherited; private methods from a superclass are not accessible in subclasses.
- 😀 Understanding inheritance is essential for efficient coding practices in Java, simplifying development and maintenance.
Q & A
What is the main purpose of inheritance in Java?
-Inheritance allows a class to inherit methods and properties from another class, enabling code reuse and reducing redundancy.
How do you define a subclass that inherits from a superclass?
-You define a subclass using the 'extends' keyword. For example, 'public class Tuna extends Food' means Tuna inherits from the Food class.
What is the difference between a superclass and a subclass?
-The superclass is the class being inherited from, while the subclass is the class that inherits from the superclass. In this context, 'Food' is the superclass, and 'Tuna' and 'PotPie' are subclasses.
What happens if a method is defined in both the superclass and the subclass?
-If a method is defined in both classes, the subclass's version will override the superclass's version when called on an instance of the subclass.
Can you inherit private methods from a superclass?
-No, private methods in a superclass cannot be inherited by a subclass. Only public and protected methods can be accessed by subclasses.
What is method overriding?
-Method overriding occurs when a subclass provides a specific implementation for a method that is already defined in its superclass, allowing for customized behavior.
What keyword is used to indicate inheritance in Java?
-The 'extends' keyword is used to indicate that a class is inheriting from another class.
What would happen if you try to call a private method from the subclass?
-You would receive an error indicating that the method is not visible, as private methods are not accessible to subclasses.
How can you implement a common method across multiple subclasses without repeating code?
-By placing the common method in the superclass, all subclasses can inherit this method, allowing them to use it without duplication.
Why is inheritance considered a fundamental concept in object-oriented programming?
-Inheritance is fundamental because it promotes code reuse, establishes a natural hierarchy among classes, and helps manage complexity by allowing new classes to build on existing ones.
Outlines
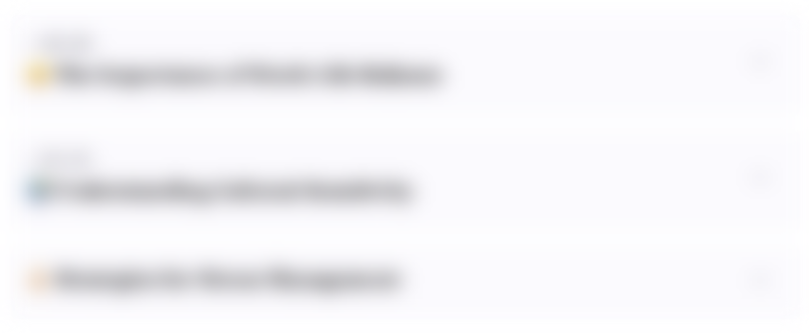
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
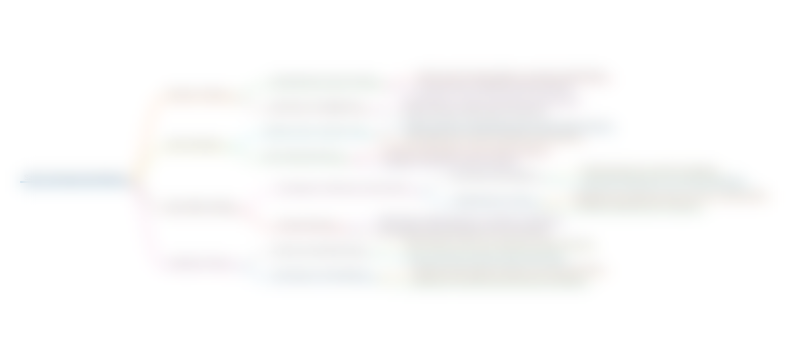
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
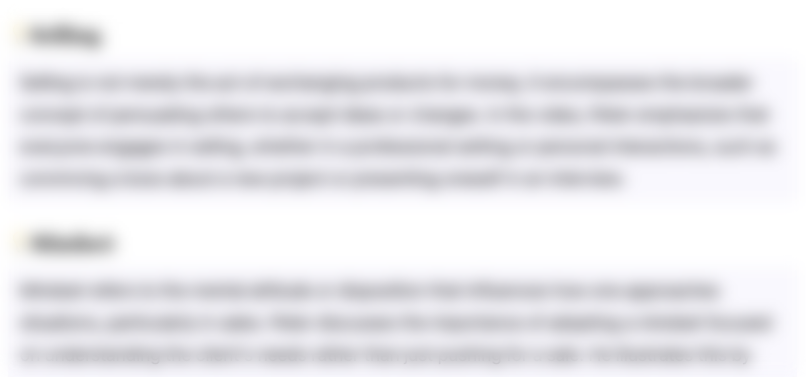
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
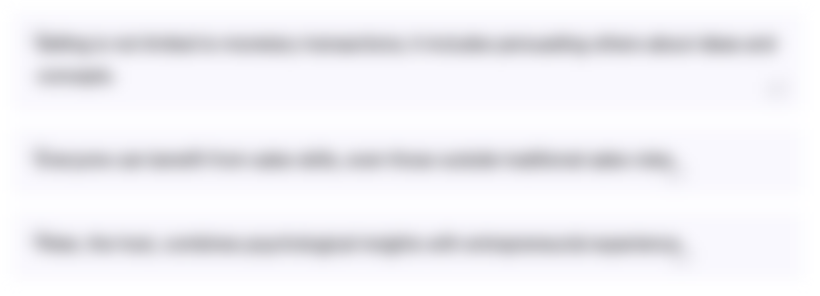
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
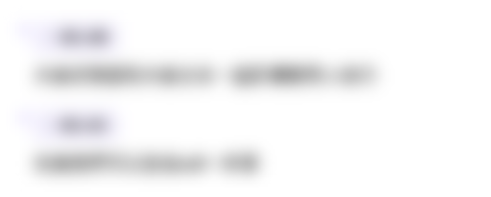
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados

Inheritance in Java - Java Inheritance Tutorial - Part 1
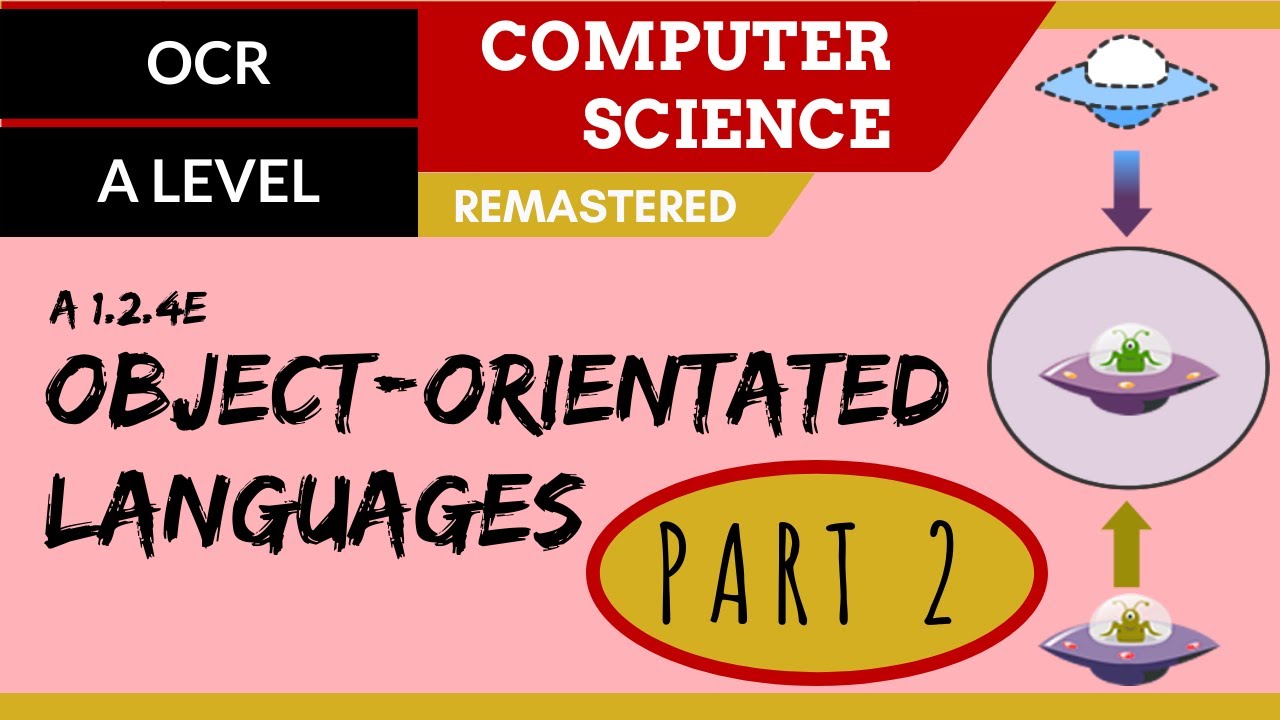
37. OCR A Level (H446) SLR7 - 1.2 Object-oriented languages part 2
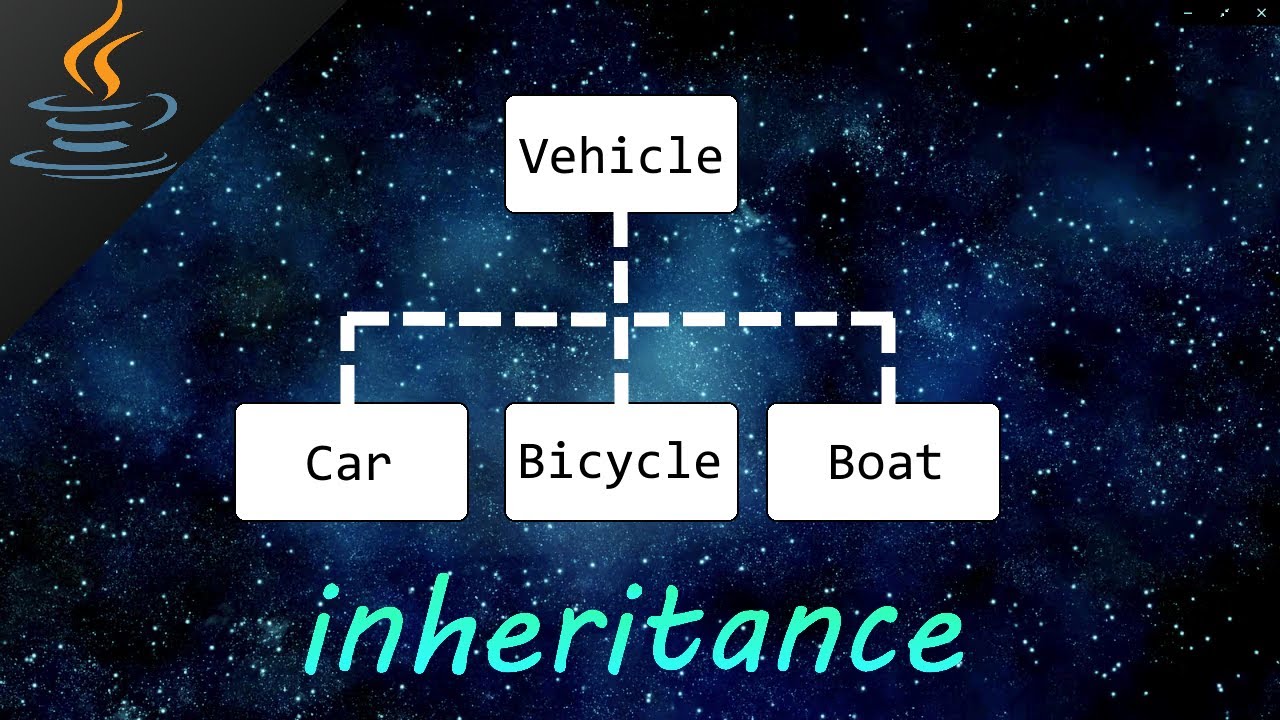
Java inheritance 👪

Java Inheritance | Java Inheritance Program Example | Java Inheritance Tutorial | Simplilearn
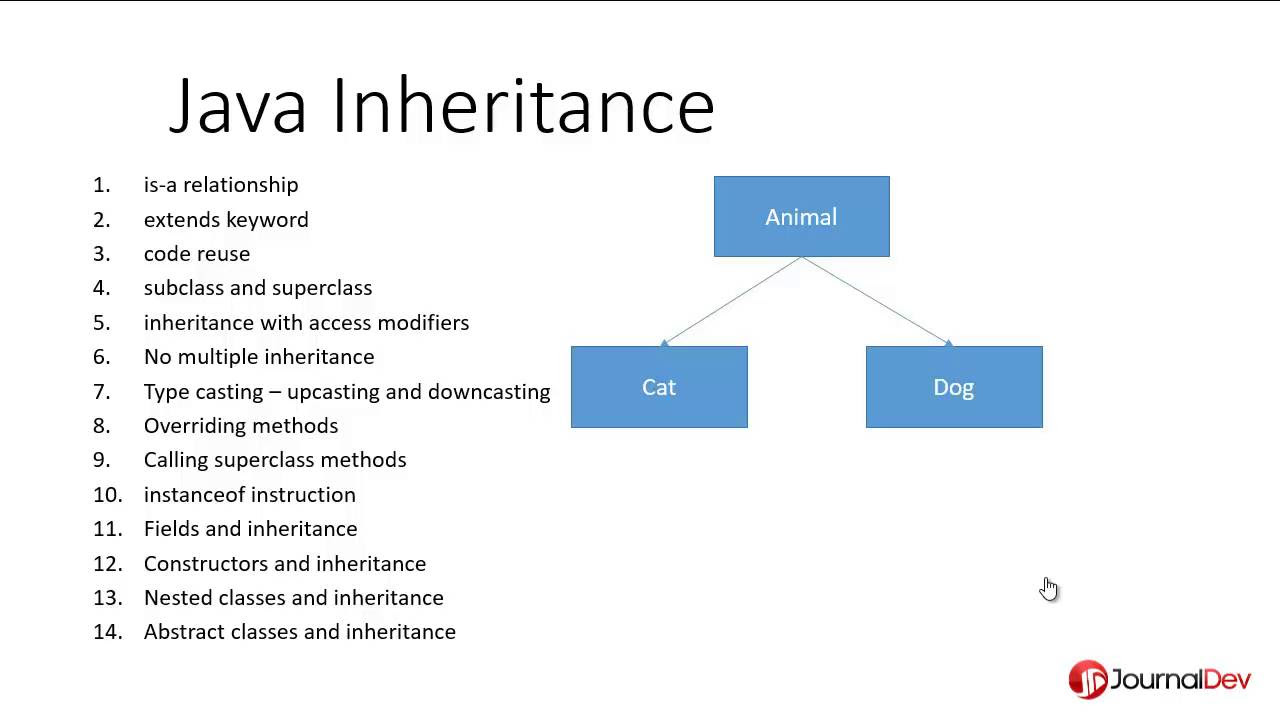
Inheritance in Java - Java Inheritance Tutorial - Part 2
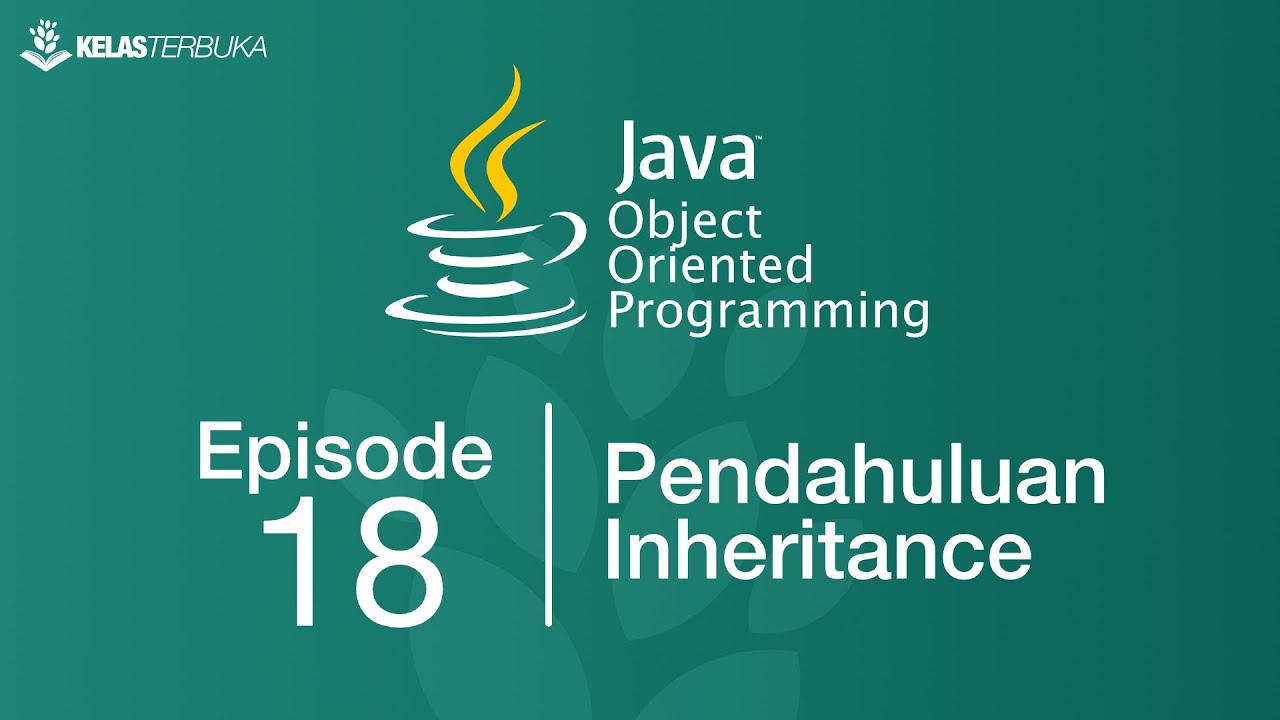
Belajar Java [OOP] - 18 - Pengenalan Inheritance
5.0 / 5 (0 votes)