Node.js Tutorial - 42 - Event Loop
Summary
TLDRThis video explains the event loop in Node.js, highlighting its role in asynchronous code execution. The speaker reviews key concepts such as JavaScript's synchronous, single-threaded nature and the importance of libuv in handling asynchronous tasks. The video provides an in-depth walkthrough of synchronous and asynchronous code execution, followed by a visual representation of the event loop. It covers how different queues (timer, I/O, check, close, and microtask) are prioritized and executed, helping viewers understand Node.js's internal processes for managing async code efficiently.
Takeaways
- 😀 JavaScript is a synchronous, blocking, single-threaded language, but asynchronous programming is enabled through external libraries like libuv.
- 💡 The V8 engine, which executes JavaScript, consists of a memory heap for variable storage and a call stack for function execution.
- 🛠️ When an async function is called, it's offloaded to libuv, which handles it using the system's async mechanisms or a thread pool, keeping the main thread free.
- 📜 In synchronous code execution, functions are pushed onto the call stack and popped off in a last-in-first-out order, producing a sequential output.
- ⏳ In asynchronous code, the async function is offloaded, and JavaScript continues executing other tasks while waiting for the async operation to finish.
- 🔄 The Node.js event loop is a key mechanism that manages the execution of async callbacks once the call stack is empty.
- 📝 There are multiple queues in the event loop, including the timer queue (setTimeout, setInterval), IO queue (async operations like file reads), check queue (setImmediate), close queue, and two microtask queues (nextTick, promise).
- ⏱️ The event loop processes these queues in a specific priority order, with microtasks being executed first, followed by timer callbacks, IO callbacks, and others.
- 🔀 Even if two async tasks complete at the same time (e.g., setTimeout and FS.readFile), timer callbacks are prioritized over IO callbacks.
- 📊 The event loop continues to run as long as there are callbacks to process, and exits when there are none left, ensuring efficient asynchronous execution in Node.js.
Q & A
What is the primary role of the event loop in Node.js?
-The event loop in Node.js orchestrates the execution of synchronous and asynchronous code. It ensures that tasks such as callbacks from async operations are handled appropriately and in a specific order after synchronous tasks have completed.
How does JavaScript handle asynchronous operations in Node.js?
-JavaScript offloads asynchronous operations to a library called libuv in Node.js. The async task is then executed either using native OS mechanisms or through a thread pool, ensuring the main thread is not blocked.
What happens to synchronous code in Node.js?
-Synchronous code in Node.js is executed in a straightforward, last-in-first-out manner on the call stack. Functions are pushed onto the stack and popped off once executed, with global scope execution starting first.
What is the role of the call stack and the heap in the V8 engine?
-The call stack is responsible for tracking the execution of functions in a last-in, first-out manner. The heap is where memory is allocated for variables and functions during the execution of code.
How does the event loop prioritize execution of different callback functions?
-The event loop executes callbacks in a specific order: first, callbacks in the microtask queues (next tick queue and promise queue), followed by callbacks in the timer queue (for setTimeout and setInterval), then the I/O queue, check queue, and close queue. Microtask queues are rechecked after every other callback queue.
What is the difference between the timer queue and the I/O queue in Node.js?
-The timer queue handles callbacks associated with setTimeout and setInterval, while the I/O queue handles callbacks related to asynchronous I/O operations, such as reading files with fs.readFile.
What are microtask queues, and why are they important?
-Microtask queues consist of the next tick queue (for process.nextTick callbacks) and the promise queue (for native promises). They are crucial because callbacks in these queues are given the highest priority and are executed before any other async tasks.
What happens if two async tasks, such as setTimeout and fs.readFile, complete simultaneously?
-If setTimeout and fs.readFile complete at the same time, the callback from the timer queue (setTimeout) is executed first, followed by the callback from the I/O queue (fs.readFile). This priority order is part of how the event loop manages task execution.
When does Node.js execute callbacks for asynchronous tasks?
-Node.js executes callbacks for asynchronous tasks only when the call stack is empty, ensuring that no synchronous code execution is interrupted.
What are some node-specific functions mentioned in the script?
-Node-specific functions mentioned in the script include setImmediate (which places callbacks in the check queue) and process.nextTick (which places callbacks in the next tick queue, a part of the microtask queues).
Outlines
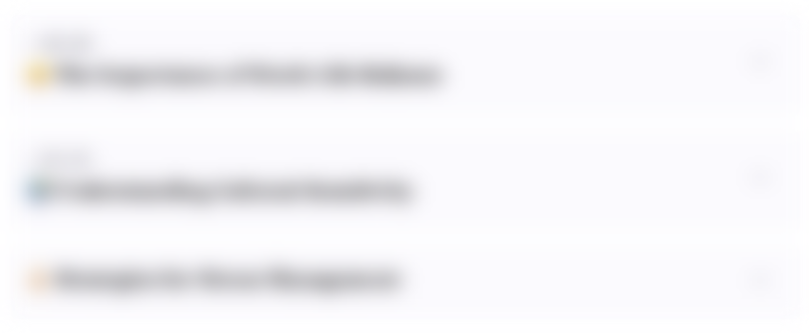
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
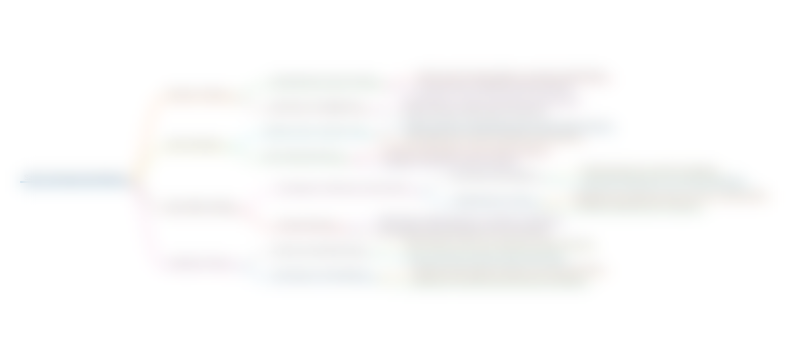
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
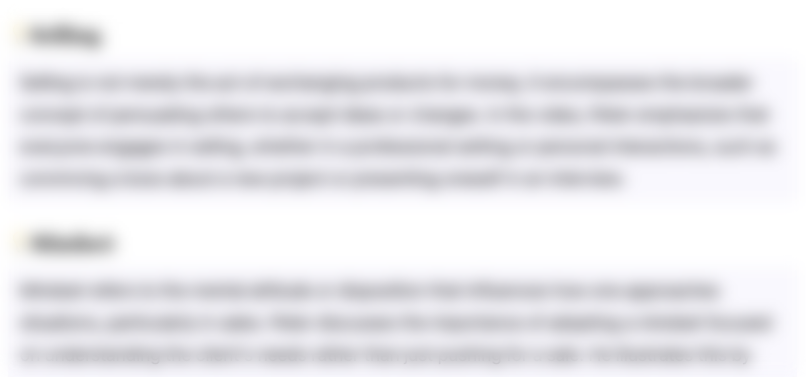
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
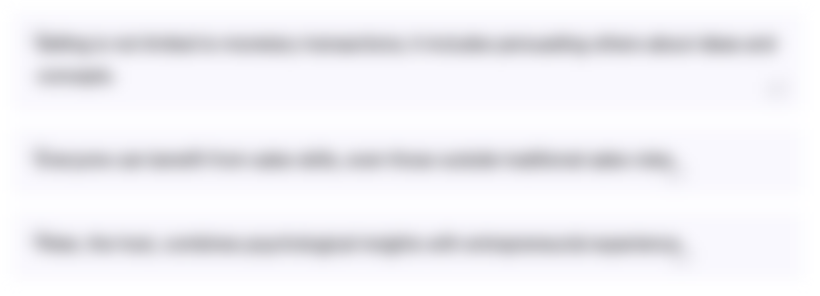
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
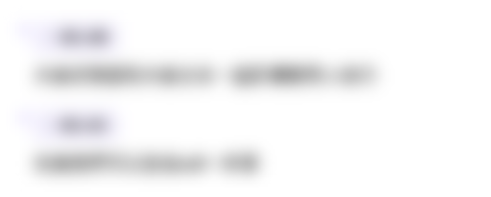
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
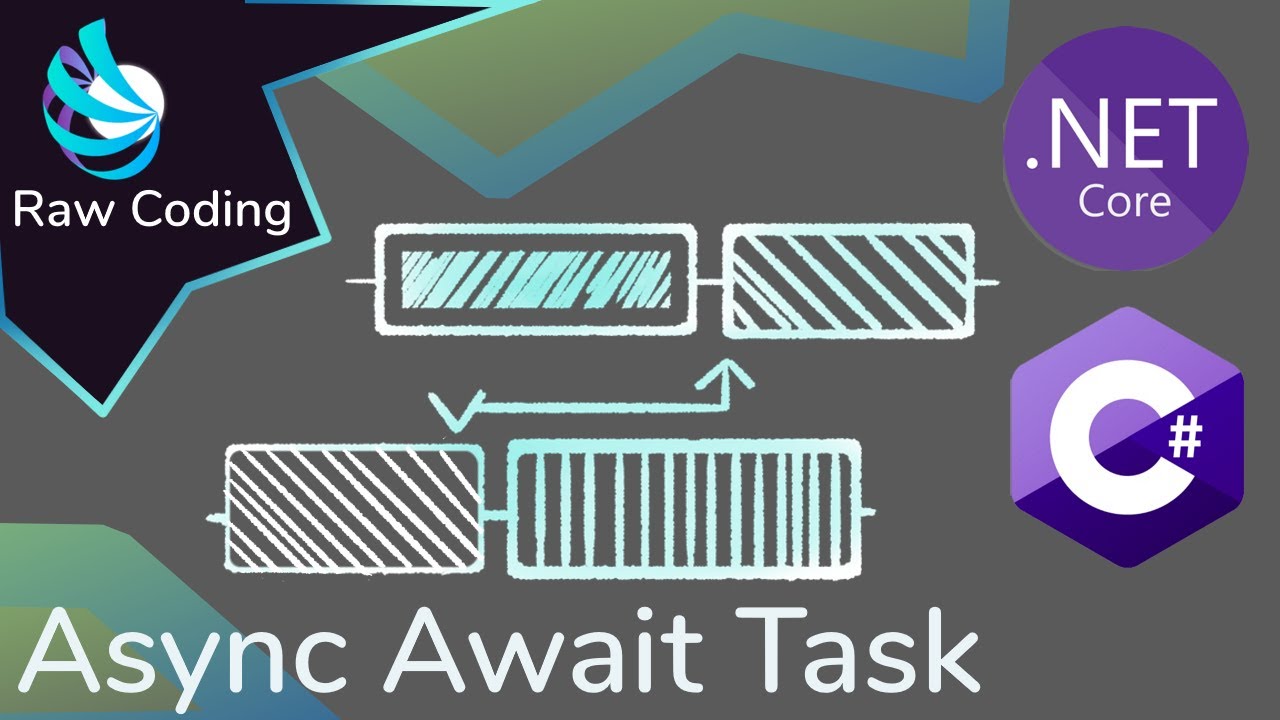
C# Async/Await/Task Explained (Deep Dive)

What the heck is the event loop anyway? | Philip Roberts | JSConf EU
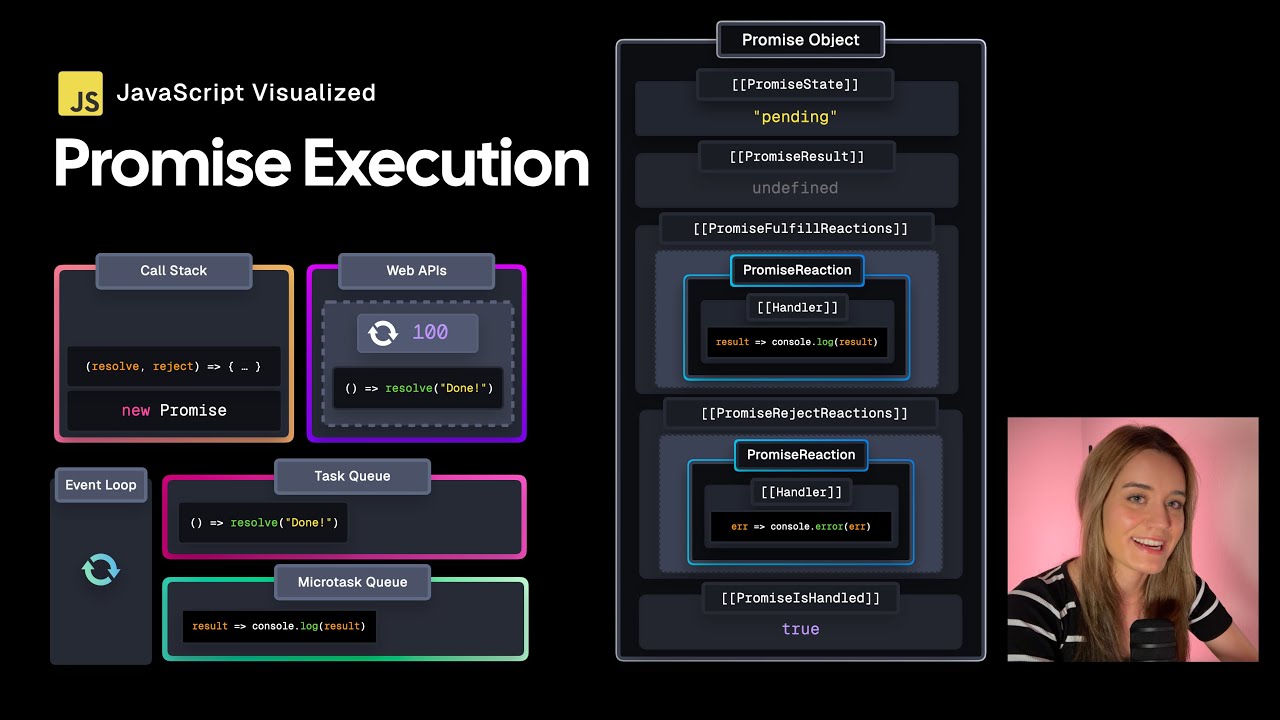
JavaScript Visualized - Promise Execution
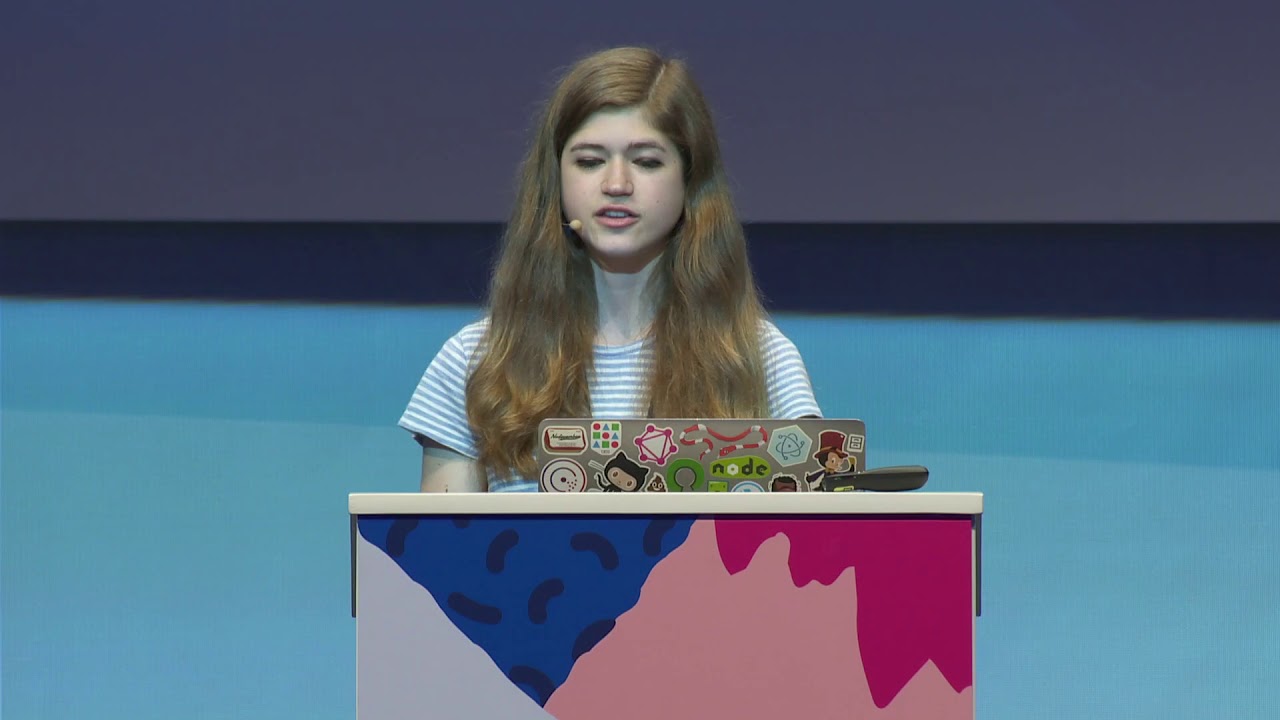
Asynchrony: Under the Hood - Shelley Vohr - JSConf EU

How JavaScript Works 🔥& Execution Context | Namaste JavaScript Ep.1

Plugin - Part 1 | What is Plugin? | Quick Introduction to Plugin | Dynamic CRM
5.0 / 5 (0 votes)