Static Properties & Methods In Object Oriented PHP - Full PHP 8 Tutorial
Summary
TLDRThis video lesson explains static properties and methods in PHP, focusing on how they differ from regular class properties. Static properties are shared across all instances of a class and belong to the class itself rather than any object. The instructor demonstrates how to define static properties and methods, access them using the scope resolution operator, and discusses best practices for their use. Use cases like counters, caching, singleton patterns, and utility methods are covered, as well as potential issues with global state and recommendations to use dependency injection instead of static properties in many cases.
Takeaways
- 📌 Static properties and methods in a class can be defined using the `static` keyword.
- 🔑 Access static properties without needing an object instance, using the class name directly.
- 🔄 Static properties are shared across all instances of a class, unlike regular properties which are unique to each object.
- 🚫 Making a static property private restricts its access to within the class only.
- 🔄 Incrementing a static property like a counter every time a new instance is created demonstrates how static properties maintain state across instances.
- 👥 Static methods cannot access non-static properties or methods because they do not operate on a specific object instance.
- 💡 Use cases for static properties include counters, caching values, and implementing design patterns like Singleton.
- 🛠️ Static methods are useful for utility functions that do not require object state or for implementing design patterns like Factory.
- ❌ Overuse of static properties and methods is discouraged as they can lead to global state issues, making code harder to maintain and test.
- 🔒 Static closures or callbacks prevent access to the `$this` variable, which can prevent unexpected side effects and bugs.
Q & A
What is a static property in a class?
-A static property in a class is a variable that belongs to the class itself rather than any specific instance of the class. It is shared across all instances and can be accessed without creating an object of the class.
How can you define a static property in PHP?
-In PHP, you can define a static property by using the `static` keyword followed by the property name. For example, `public static $count = 0;`.
How do you access a static property in PHP?
-You can access a static property in PHP using the scope resolution operator (`::`). For example, `Transaction::$count` would access the static property `count` of the `Transaction` class.
What is the difference between static properties and regular class properties?
-Static properties are shared across all instances of a class, while regular class properties are specific to individual objects. Each object has its own copy of regular properties, but static properties are common to all objects of the class.
How do you increment a static property inside a class?
-You can increment a static property inside a class using the `self` keyword or the class name followed by the property name. For example, `self::$count++` or `Transaction::$count++`.
Can static properties be accessed from outside the class if their access modifier is set to private?
-No, if a static property is set to private, it cannot be accessed from outside the class directly. You need to use a public static method within the class to access it, like `getCount()`.
What is the use case for static properties in PHP?
-One use case for static properties is implementing a counter or caching values across instances. Static properties can also be used in design patterns like the Singleton pattern to maintain a single instance of a class.
Why is the use of static properties and methods generally discouraged?
-Static properties and methods represent a global state, which can be modified from anywhere in the code. This makes the code harder to maintain, test, and debug. Dependency injection is often a better alternative.
What is the Singleton pattern and how can static properties be used to implement it?
-The Singleton pattern ensures that a class has only one instance during the application's execution. Static properties can be used to store the single instance of the class, and static methods like `getInstance()` can control access to it.
What are static methods useful for in PHP?
-Static methods are useful for utility functions that don’t need an instance of the class to work. For example, methods that format data (e.g., currency, percentage) or handle object creation (as in the factory design pattern) can be static.
Outlines
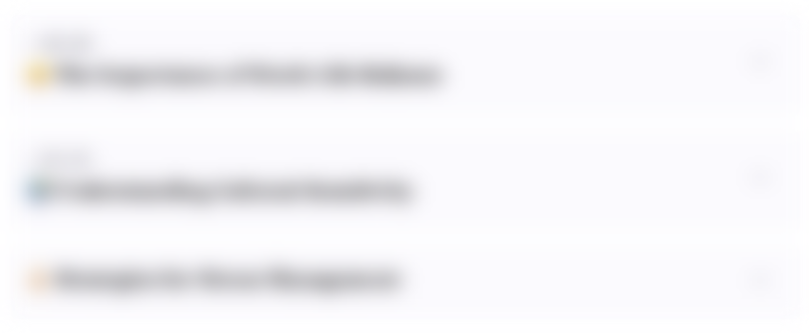
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
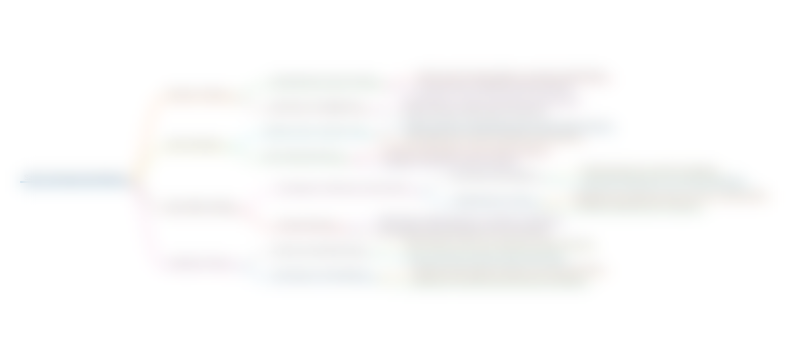
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
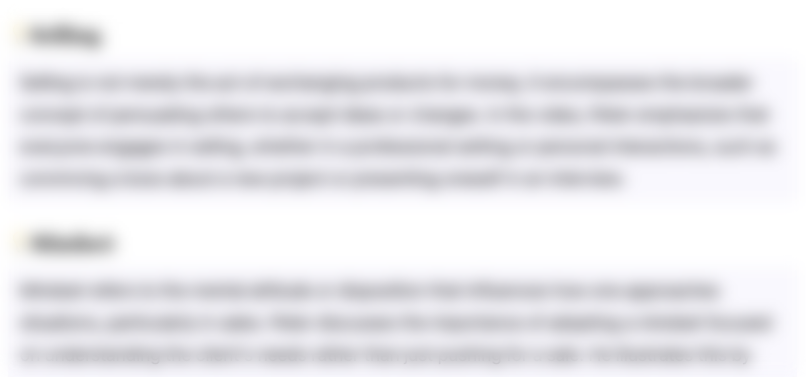
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
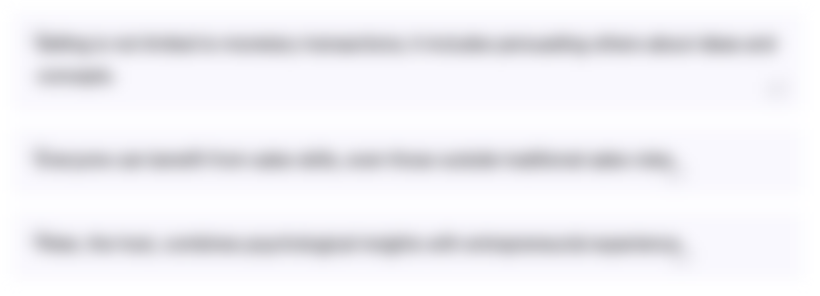
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
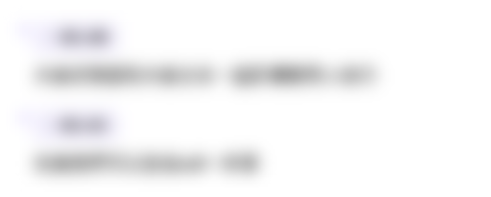
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
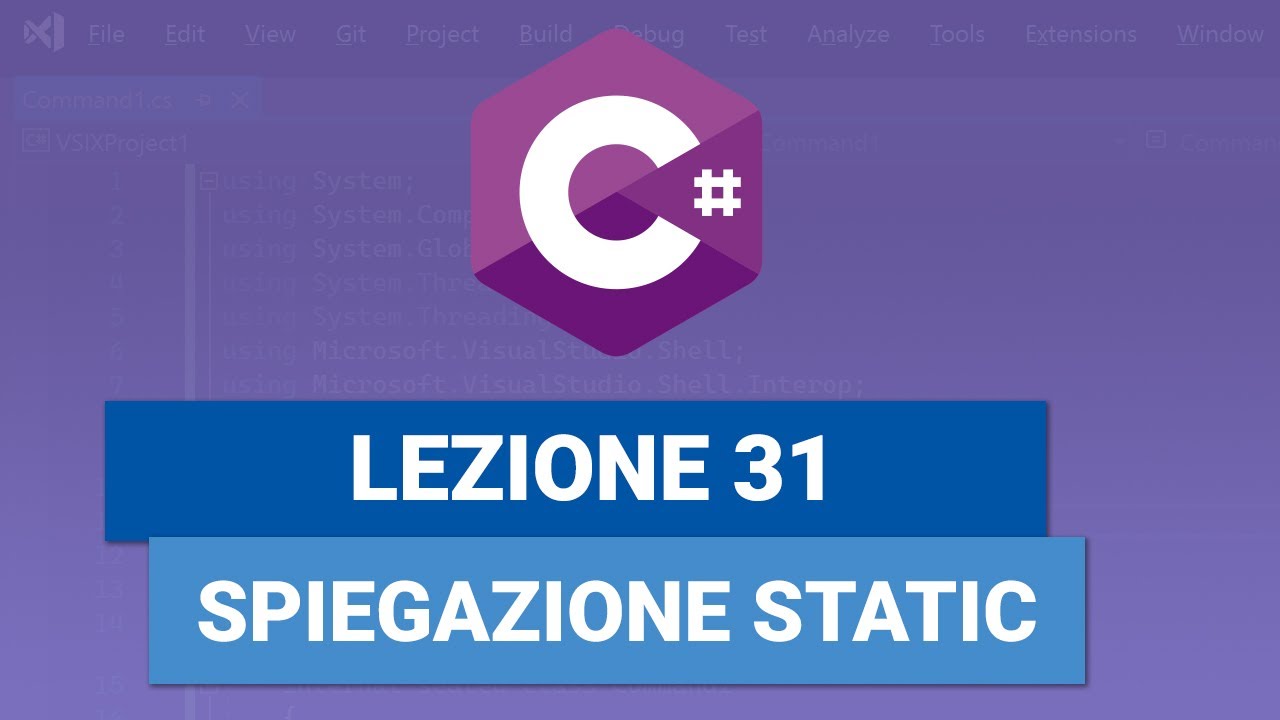
Parole chiave STATIC - C# TUTORIAL ITALIANO 31

#37 Static Variable in Java
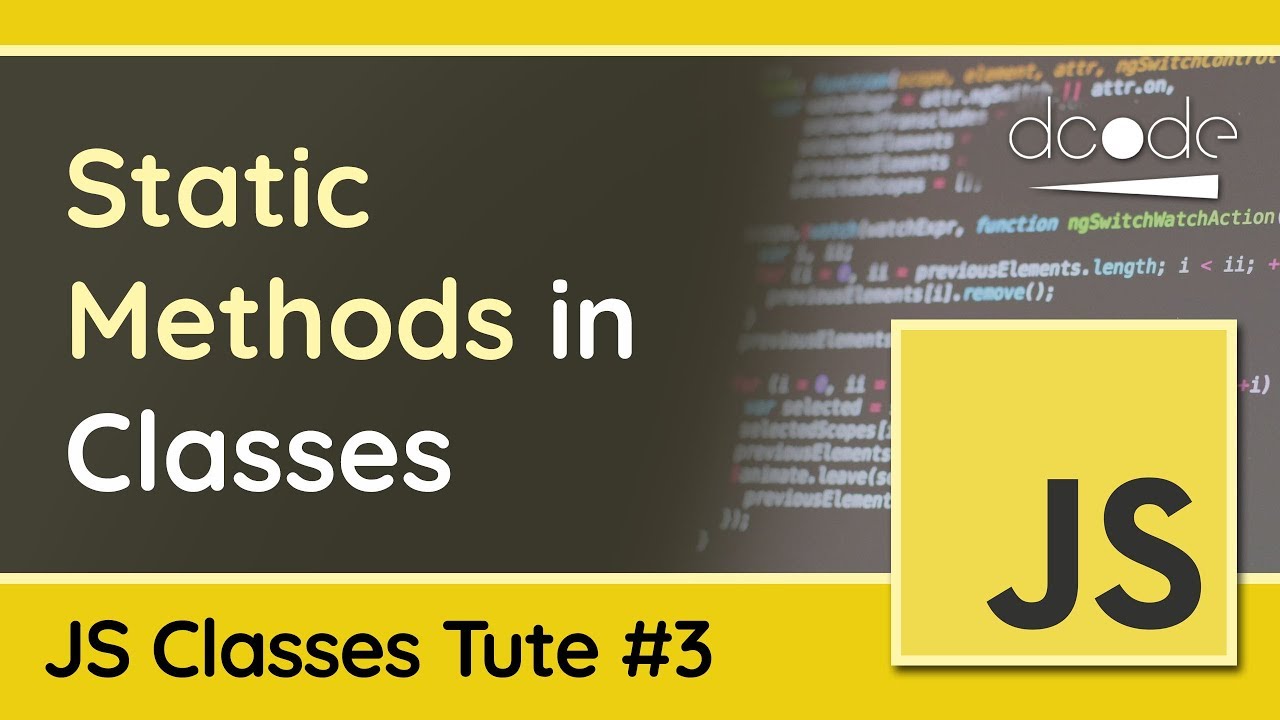
JavaScript Classes #3: Static Methods - JavaScript OOP Tutorial
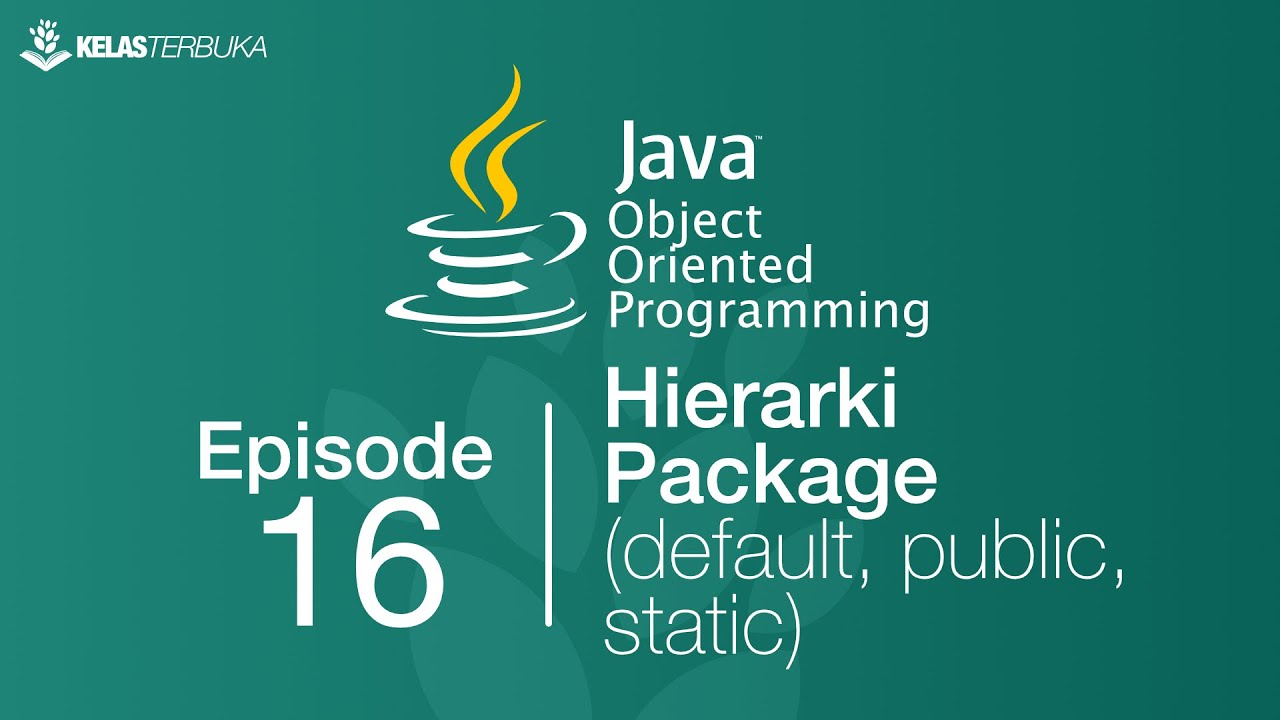
Belajar Java [OOP] - 16 - Hierarki Package (default, public, static)
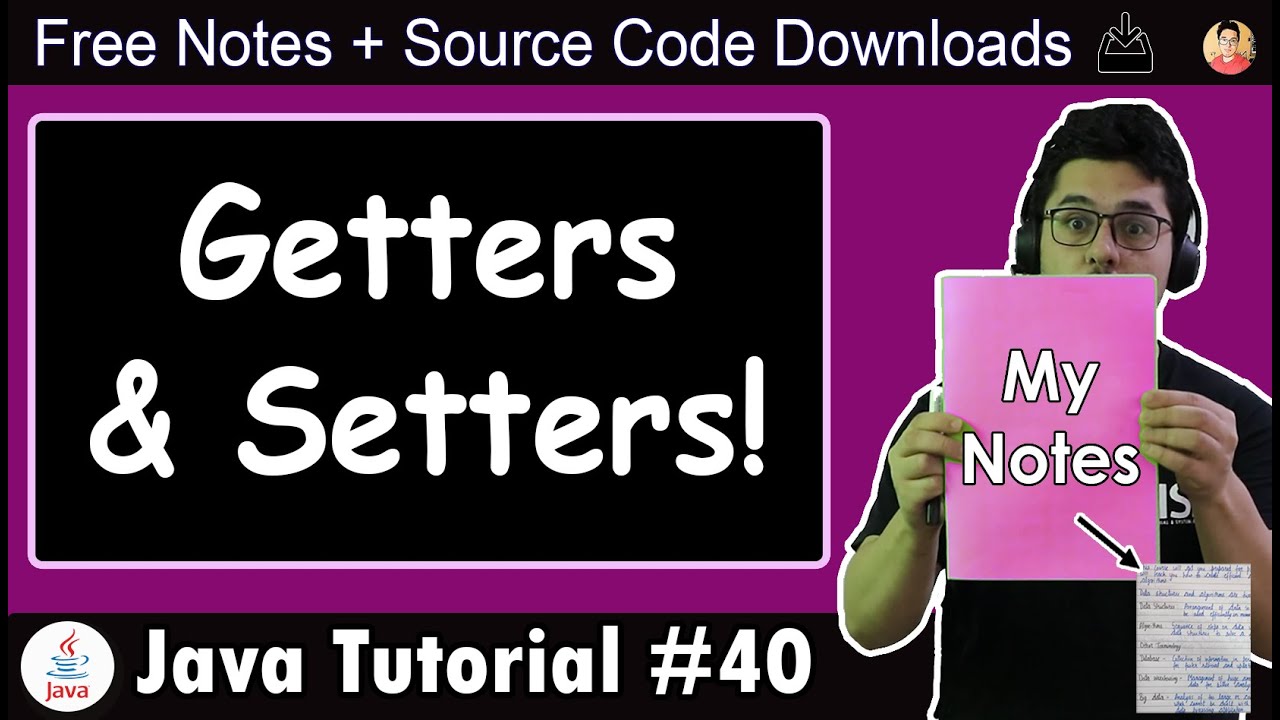
Java Tutorial: Access modifiers, getters & setters in Java
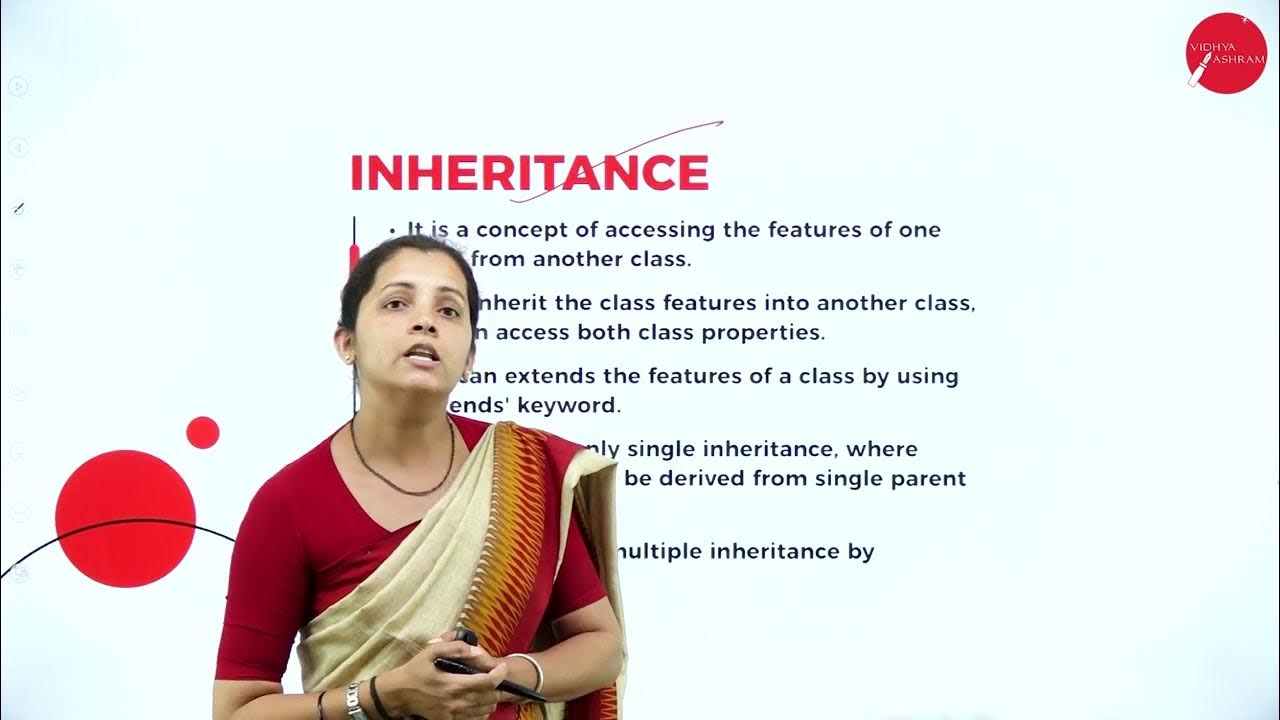
DAY 07 | PHP AND MYSQL | VI SEM | B.CA | CLASS AND OBJECTS IN PHP | L1
5.0 / 5 (0 votes)