Sections Of The Assembly Program | first assembly program | | Assembly Language in MASM Part 4/16
Summary
TLDRThis lecture introduces fundamental assembly language concepts, including memory models and variable declaration. It explains different memory models like small, medium, compact, and huge, which determine how code and data are stored in RAM. The script also covers stack operations, variable naming conventions, and types like DB, DW, DD, and DQ for data storage. The tutorial demonstrates creating an assembly program to print a message using the 'mov' instruction and the 'int 21h' interrupt, providing a hands-on example for beginners.
Takeaways
- 💾 **Memory Model Basics**: Assembly programs use memory models to specify the amount of memory needed for code and data storage in RAM.
- 🔢 **Memory Models Explained**: There are five memory models - small, medium, compact, large, and huge, each determining how code and data are segmented.
- 📏 **Model Selection**: The 'small' model is chosen for this lecture, suitable for programs where both code and data fit in a single segment.
- 💼 **Stack Operations**: The 'stack' directive is used to allocate memory for stack operations, with '100h' specifying the size in hexadecimal.
- 🔑 **Variable Declaration**: Variables in assembly are declared with a name, type, and initial value, following specific naming conventions.
- 🚫 **Variable Name Rules**: Variable names are limited to eight characters, can include alphabets, digits, and underscores, but no spaces or special characters.
- 📚 **Variable Types**: Different variable types like 'db' for bytes, 'dw' for words, 'dd' for double words, and 'dq' for quad words are used based on the data size.
- 💬 **String Handling**: 'db' is used for single-character strings, while 'dw', 'dd', and 'dq' are for larger data types, allowing storage of more significant values.
- 🛠️ **Program Structure**: The script outlines the structure of an assembly program, including memory allocation, variable declaration, and instructions.
- 💻 **Practical Implementation**: The lecture demonstrates how to write, compile, and run an assembly program that displays a message on the screen.
Q & A
What is the default way to open any drive mode in assembly?
-The default way to open any drive mode in assembly is by using the command 'int 13h' with the appropriate drive number as the value of the DL register.
How is a message printed in an assembly program?
-A message is printed in an assembly program by using the 'int 21h' interrupt with the 'AH' register set to 09h, and the message to be printed is stored in a string variable.
What does 'model small' in an assembly program mean?
-'model small' specifies that the program uses a small memory model, where code and data are both stored in the same segment in RAM, with the code being less than or equal to 64KB.
What does 'stack 100h' do in an assembly program?
-'stack 100h' reserves 256 bytes (100h in hexadecimal) of memory for stack operations.
What is the significance of the '.data' section in an assembly program?
-The '.data' section is used to declare variables and store data in an assembly program.
What are the rules for naming variables in assembly?
-Variable names should be within eight characters, can use alphabets and digits, but no spaces. Only underscores are allowed as special characters, and no dollar signs or other special symbols.
What does 'db' stand for in assembly language and what does it represent?
-'db' stands for 'define byte' and it is used to declare a variable that can store one character or one byte of data.
What is the difference between 'db', 'dw', 'dd', and 'dq' in assembly language?
-In assembly language, 'db' defines a byte (1 byte), 'dw' defines a word (2 bytes), 'dd' defines a double word (4 bytes), and 'dq' defines a quad word (8 bytes).
How do you store a string in an assembly program?
-To store a string in an assembly program, you can use the 'db' directive for each character of the string, or use 'db' with a string enclosed in quotes.
What is the purpose of the 'mov' instruction in assembly?
-The 'mov' instruction is used to move data from one place to another, such as from a register to a memory location, or from a memory location to a register.
How do you compile and run an assembly program?
-To compile and run an assembly program, you typically use an assembler like MASM, then link the object code with a linker, and finally execute the resulting executable file.
Outlines
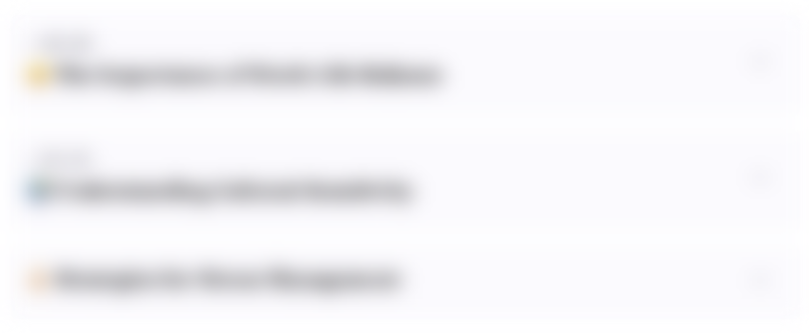
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
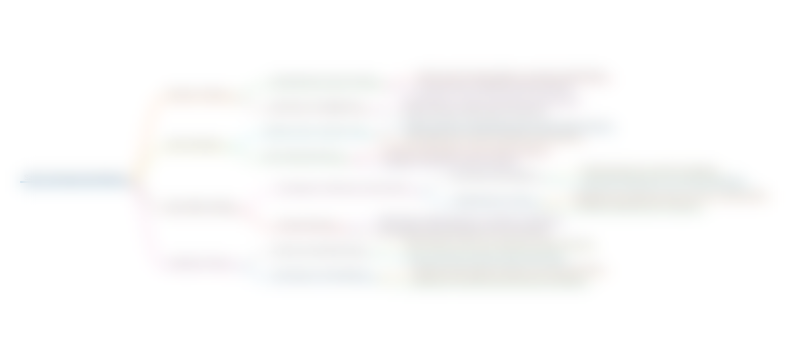
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
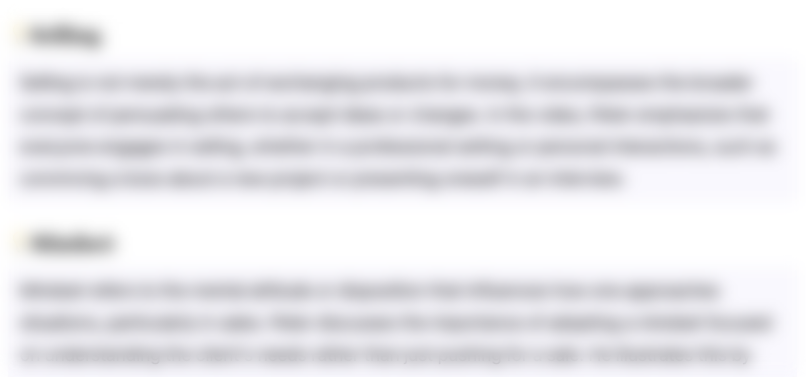
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
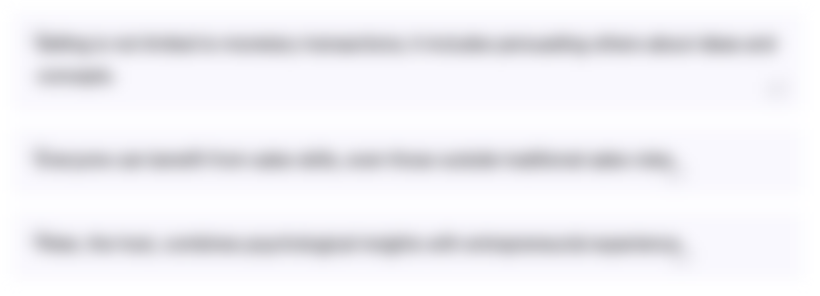
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
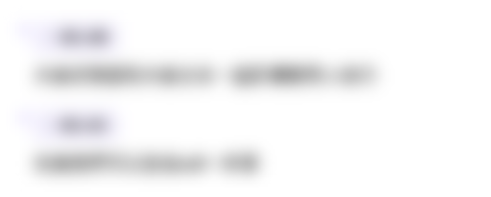
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
5.0 / 5 (0 votes)