Data Types | C# | Tutorial 5
Summary
TLDRIn this tutorial, Mike from Draft Academy introduces the core data types in C#, essential for representing different types of information in programming. He explains how to use variables to store data such as strings for text, characters for single letters, integers for whole numbers, and decimals for precise values. Mike also covers the float, double, and decimal types for representing numbers with different levels of accuracy. Additionally, he touches on the boolean data type for true or false values. The tutorial emphasizes the importance of these data types for beginners and how they can be used in C# to create various programs.
Takeaways
- 💻 C# is a programming language that deals with various types of data and information.
- 🗣️ A variable in C# is a container used to store data values or pieces of information.
- 📝 Strings in C# represent plain text and are denoted using open and closed quotation marks.
- 🔤 Characters in C# represent a single character and are enclosed in single quotation marks.
- 🔢 Integers in C# are used to represent whole numbers without decimals, such as counting numbers.
- ➖ Negative numbers can be represented in C# by placing a minus sign in front of the number.
- 🔄 C# provides three data types for decimal numbers: float, double, and decimal, with varying levels of precision.
- 💰 The decimal data type is the most precise and is suitable for representing money or other exact values.
- 📊 A double data type is a good balance between precision and performance for most use cases.
- 🤖 Booleans in C# represent true or false values and are useful for storing binary conditions.
- 🎯 Other, less common data types exist in C# but for beginners, focusing on strings, characters, numbers, and booleans is sufficient.
Q & A
What are the core data types discussed in the C# tutorial?
-The core data types discussed in the tutorial are strings, characters, integers, decimals (float, double, and decimal), and booleans.
What is a string in C# and how is it denoted?
-A string in C# represents plain text and is denoted using open and closed quotation marks.
How do you define a character in C# and what is the difference between a character and a string?
-A character in C# is defined using the 'char' keyword and single quotation marks, and it can only contain one single character. The difference from a string is that a string can contain multiple characters enclosed in double quotation marks.
What is an integer in C# and what type of numbers can it represent?
-An integer in C# is a whole number without decimals, representing counting numbers like 1, 2, 3, etc. It can also represent negative numbers.
What are the three data types used to represent decimal numbers in C# and which one is the most precise?
-The three data types used to represent decimal numbers in C# are float, double, and decimal. The most precise one is the decimal data type.
Why would you use a 'double' data type in C# and what is an example of its use?
-You would use a 'double' data type in C# for representing decimal numbers when a moderate level of precision is needed. An example of its use could be storing a person's GPA, like 3.2.
What is a boolean in C# and what values can it have?
-A boolean in C# is a data type that can only have two values: true or false, representing logical conditions or binary states.
Can data types in C# be used without being stored in variables, and if so, how?
-Yes, data types in C# can be used without being stored in variables. They can be directly used in expressions or printed out using methods like 'Console.WriteLine'.
What is the purpose of using different data types in C# programming?
-Different data types in C# are used to handle various kinds of data accurately, ensuring the program can store and manipulate data such as text, numbers, and logical states appropriately.
Are there more data types in C# besides the ones mentioned in the tutorial?
-Yes, there are more data types in C# besides strings, characters, integers, decimals, and booleans, but they are more obscure and not commonly used in basic programming.
What is the significance of using quotation marks when defining a string in C#?
-Using quotation marks when defining a string in C# signifies that the enclosed text is a sequence of characters to be treated as a single unit of data.
Outlines
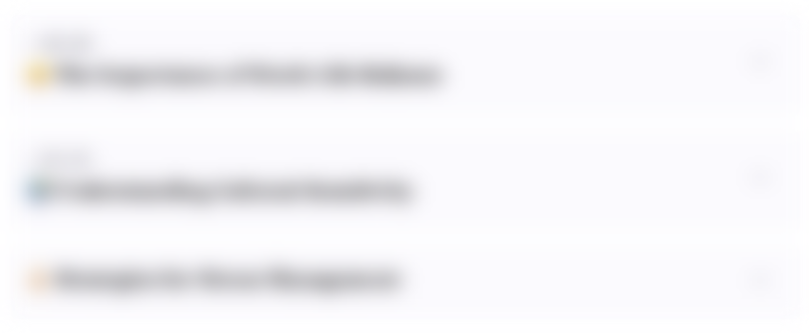
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
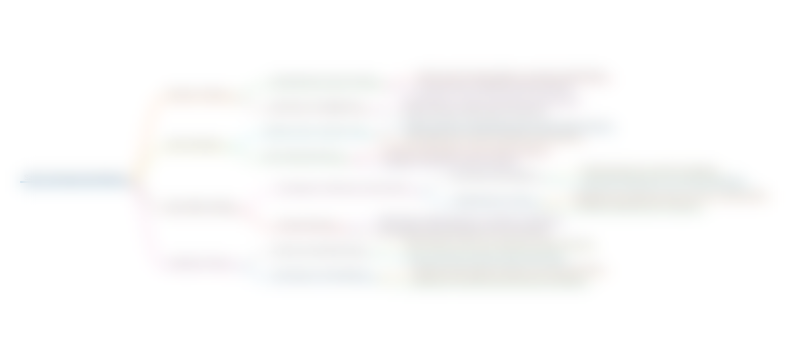
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
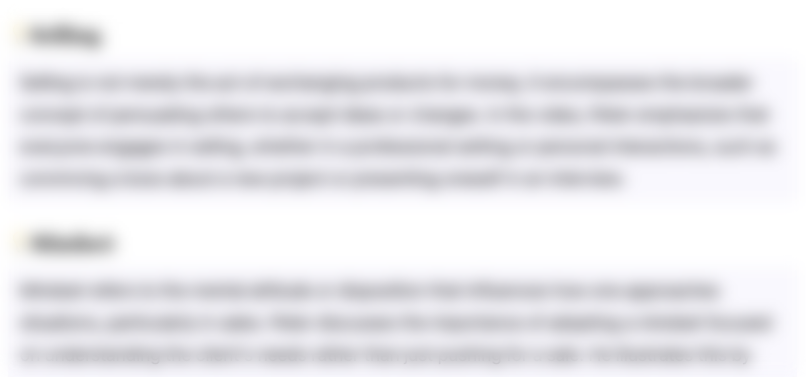
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
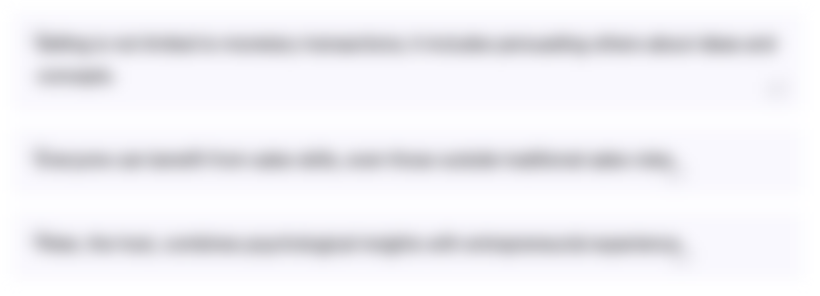
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
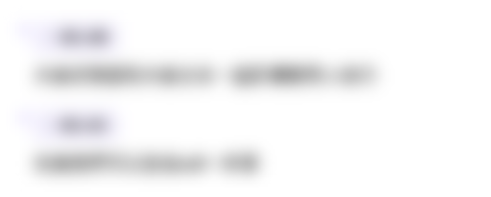
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahora5.0 / 5 (0 votes)