Python 101: Learn the 5 Must-Know Concepts
Summary
TLDRThis video script is an educational guide for Python developers, focusing on five critical concepts: mutable vs. immutable types, list comprehensions, argument types in functions, the '__name__ == "__main__"' idiom, and the Global Interpreter Lock (GIL). It aims to enhance understanding of production code, enabling developers to write efficient and effective Python code. The script includes practical examples and a sponsored segment on Nord Pass, a password management solution.
Takeaways
- 💻 **Understanding Python Concepts**: The video emphasizes the importance of grasping five crucial Python concepts to avoid common mistakes and effectively read and write production code.
- 🔐 **Nord Pass Sponsorship**: Nord Pass is introduced as a secure password and credential management solution, useful for storing and sharing sensitive information like passwords and bank details.
- 🔄 **Mutable vs Immutable Types**: A key concept explained is the difference between mutable and immutable types in Python, where immutable types cannot be changed after creation, unlike mutable types.
- 📚 **List Comprehension**: The video covers list comprehensions, a Python feature used to create lists in a concise way, and how they can simplify or complicate code depending on usage.
- 📝 **Parameter Types in Functions**: It explains various types of parameters in Python functions, including positional, optional, and the use of *args and **kwargs for flexible argument passing.
- 🔑 **Global Interpreter Lock (GIL)**: The script discusses the GIL in Python, which allows only one thread to execute at a time, despite the presence of multiple CPU cores, limiting the performance benefits of multi-threading.
- 🛠️ **Function Side Effects**: The concept of side effects in functions is introduced, where functions modify the state of objects passed as arguments, which is crucial for understanding when working with mutable types.
- 🔍 **Conditional List Comprehensions**: The video demonstrates how to use conditional statements within list comprehensions to create lists based on specific criteria, like filtering even numbers.
- 📦 **Module Execution Control**: The use of `if __name__ == '__main__':` is explained to control code execution when a module is run directly versus when it's imported, preventing unintended code execution.
- 🔀 **Threading and Performance**: The script touches on the limitations of threading in Python due to the GIL, explaining that multi-threading does not speed up execution in Python as it might in other languages.
Q & A
What is the main goal of the video for Python developers?
-The main goal of the video is to ensure that Python developers understand key concepts when reading through production Python code, so they can reproduce and write their own code that other developers will understand and expect.
Why is it important to understand the difference between mutable and immutable types in Python?
-Understanding the difference between mutable and immutable types is crucial because it affects how variables and objects behave when they are assigned and modified. Immutable types cannot be changed after creation, whereas mutable types can be modified, which can lead to unexpected behavior if not properly managed.
What is a side effect in the context of functions in Python?
-A side effect in Python functions refers to a function modifying a parameter that affects the outside state, such as changing a mutable object passed as an argument. This can lead to changes in the original object, which might not be intended and can cause bugs or unexpected behavior.
How do list comprehensions in Python simplify code?
-List comprehensions in Python simplify code by allowing the creation of lists in a concise way, using a single line of code that includes an expression followed by a for loop. This can make the code more readable and efficient compared to traditional for loop methods.
What are the different types of parameters that can be used in Python functions?
-Python functions can use various parameter types, including positional parameters, keyword parameters, optional parameters with default values, *args for accepting a variable number of positional arguments, and **kwargs for accepting a variable number of keyword arguments.
Why is the '__name__' attribute important in Python scripts?
-The '__name__' attribute is important in Python scripts because it determines if the script is being run directly or being imported as a module. It is commonly used to prevent certain code from executing when the module is imported, but only when the script is run directly.
What is the Global Interpreter Lock (GIL) in Python, and how does it affect multi-threading?
-The Global Interpreter Lock (GIL) is a mutex that protects access to Python objects, preventing multiple threads from executing Python bytecodes at once. This means that even with multiple threads, only one thread can execute at a time, which can limit the performance benefits of multi-threading in CPU-bound Python programs.
How can the 'if __name__ == '__main__' idiom be used in Python scripts?
-The 'if __name__ == '__main__' idiom is used to allow a Python script to determine if it is being run directly or being imported as a module. Code inside this block will only execute when the script is run directly, not when it is imported.
What is the significance of the 'Nord Pass' sponsorship mentioned in the video?
-The 'Nord Pass' sponsorship mentioned in the video signifies that the company provided financial support for the creation of the video content. The video includes a promotion for Nord Pass, highlighting its features and offering a discount code for viewers.
How can understanding Python concepts like mutable and immutable types help in writing production code?
-Understanding Python concepts like mutable and immutable types helps in writing production code by preventing bugs and unexpected behavior that can arise from improper handling of object mutability. It ensures that code is more predictable, maintainable, and efficient.
Outlines
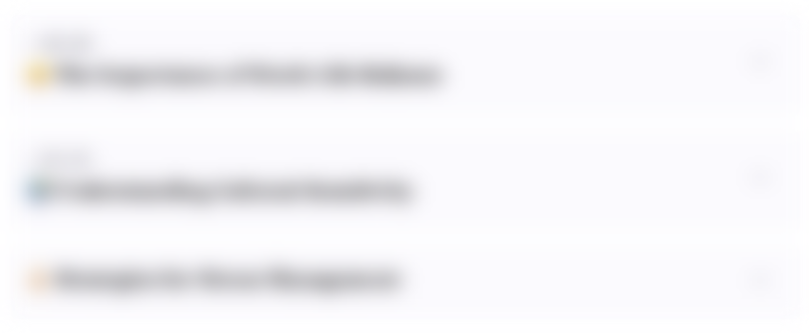
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
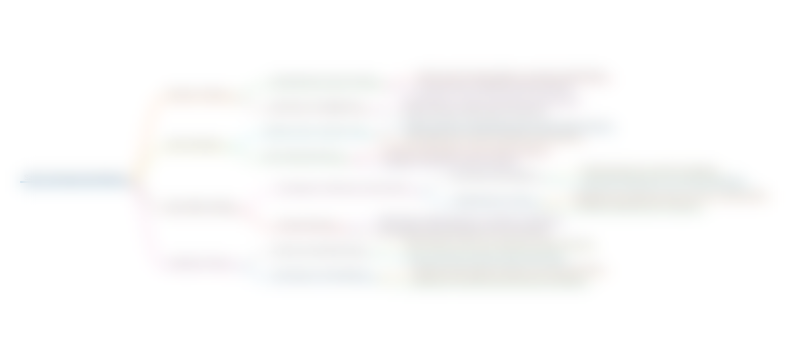
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
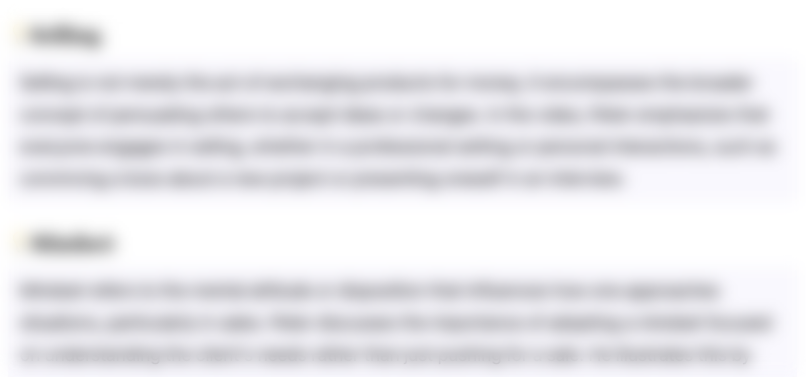
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
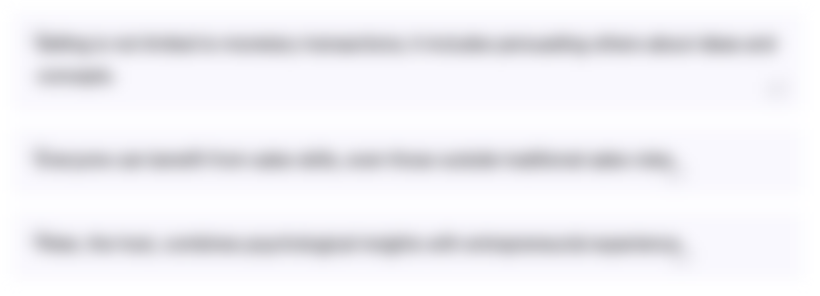
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
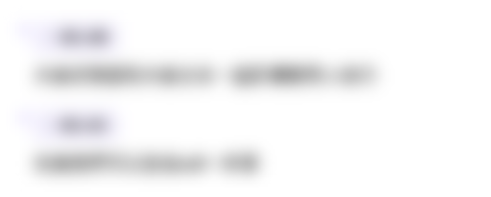
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
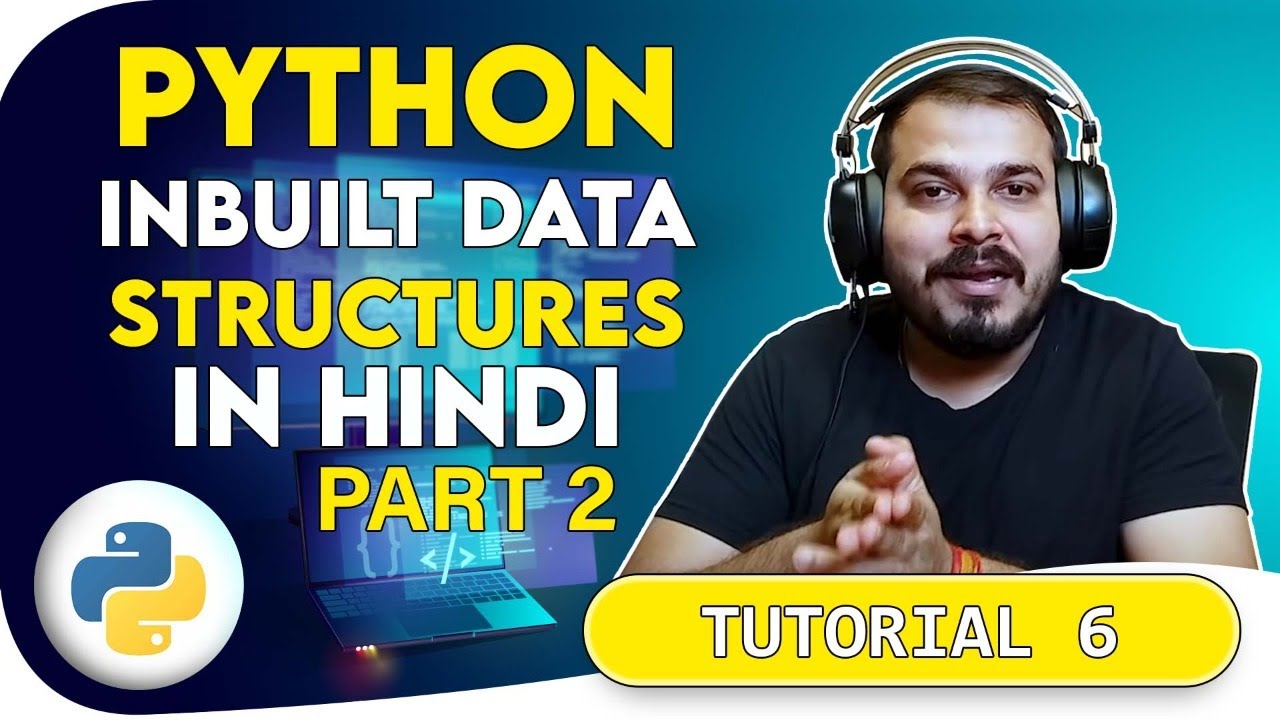
Tutorial 6- Python List And Its Inbuilt Function In Hindi
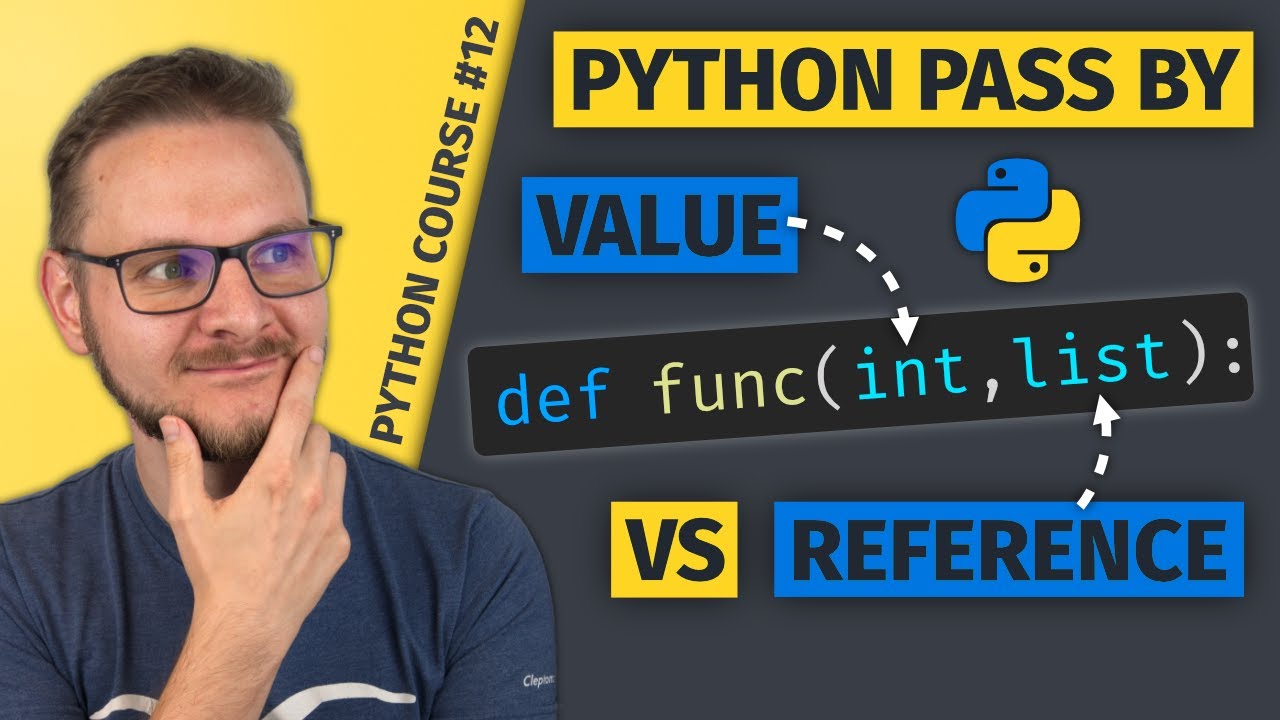
Pass by Value vs. Pass by Reference | Python Course #12

Top 10 JavaScript Interview Questions EXPLAINED! | Tanay Pratap Hindi

Photography Terminology Every Beginner Should Know
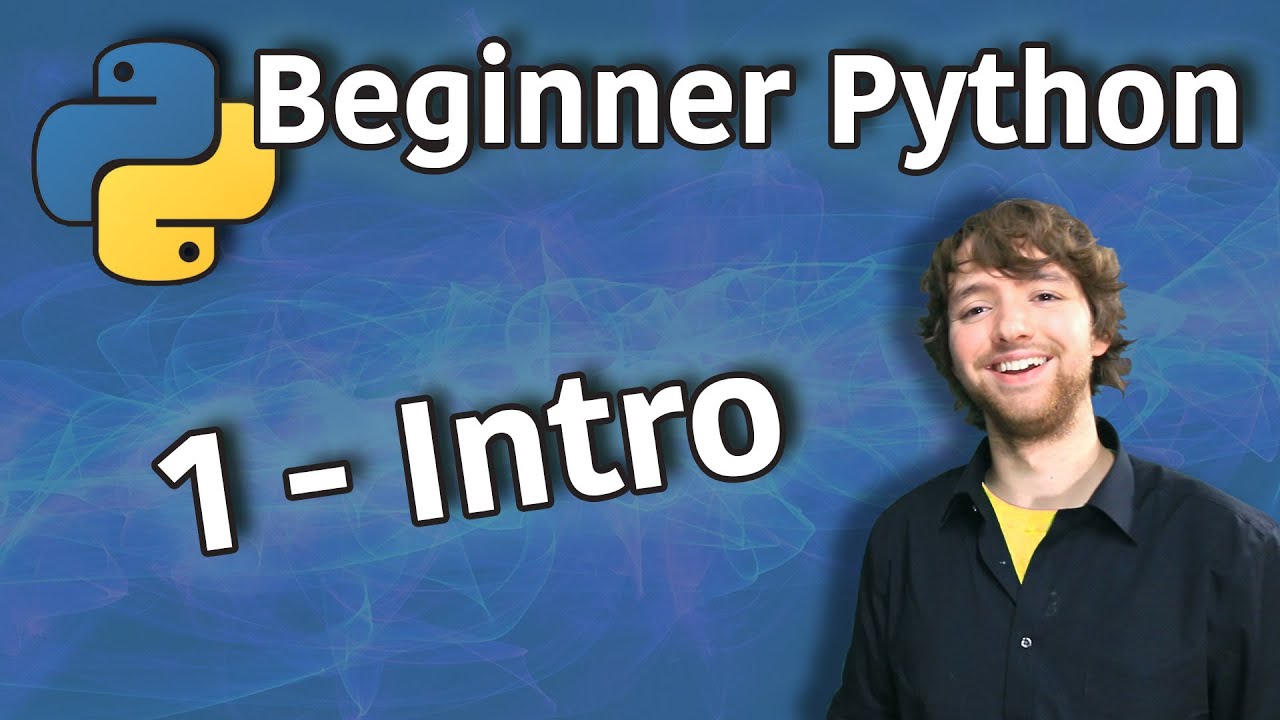
Beginner Python Tutorial 1 - Introduction
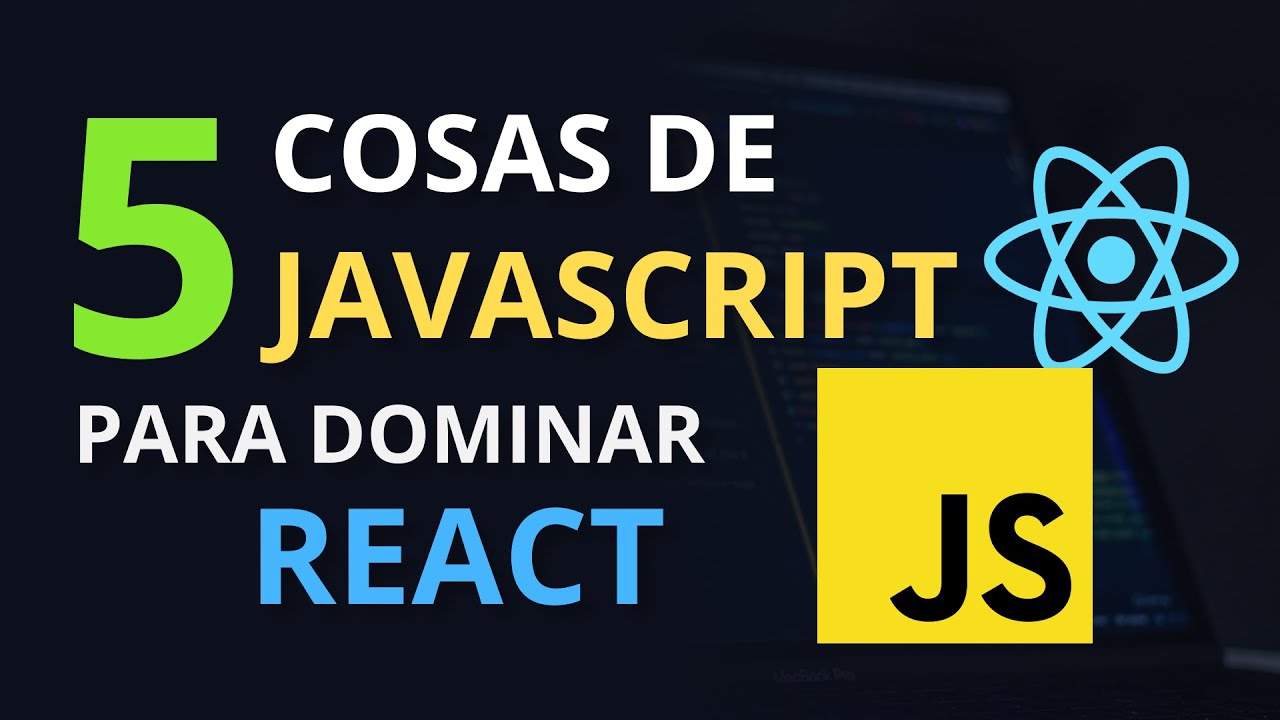
5 cosas de JAVASCRIPT que deberías dominar para aprender REACT JS
5.0 / 5 (0 votes)