Crack Your Java Interview With Most-Asked Questions | Java Fundamentals
Summary
TLDRThis video script offers an in-depth guide to mastering Java interviews, covering fundamental topics such as primitive and non-primitive data types, the importance of understanding object sizes, and the utilization of classes and methods. It delves into advanced concepts like auto-boxing, unboxing, and the use of final variables for immutability and thread safety. The script is designed to prepare viewers with a structured approach to Java interview questions, including topics on Spring Framework, security, and best coding practices, aiming to enhance their knowledge and confidence for technical interviews.
Takeaways
- 📚 The video covers Java fundamentals, aiming to help viewers crack Java interviews by addressing key interview questions and concepts.
- 🔑 It explains the difference between primitive data types and non-primitive data types in Java, emphasizing that primitives have fixed sizes and are not objects, while non-primitives are objects and their size is not fixed.
- 🧠 The video discusses the concept of pointers in Java, stating that Java does not provide support for pointers as seen in other languages due to its focus on security.
- 🔢 It addresses how to declare variables in Java for storing large numbers up to 2 billion, using the 'int' data type for smaller numbers and 'long' for larger values.
- 📈 The video talks about the difference between instance variables and local variables, highlighting that instance variables are class-level and retain their value for the life of the object, while local variables are method-level and do not retain their value after the method execution ends.
- 🏦 In the context of banking applications, it explains how customer account balances should be managed as instance variables to ensure each customer's balance is tracked and updated correctly.
- 🔄 The video touches on the importance of default values in Java, assigning default values to variables and instances based on their data type to prevent errors when variables are not properly initialized.
- 🔒 It discusses the 'final' keyword in Java, explaining its use for declaring constants, preventing method overriding, and class inheritance, thus contributing to immutability and thread safety.
- 📝 The video explains the 'static' keyword, detailing its use for indicating class-level variables and methods that belong to the class rather than any instance of the class.
- 🔍 The video also covers Java's string pool, a memory management feature that stores all string literals in the heap memory, allowing for efficient memory usage by reusing identical strings.
- 🛠️ Lastly, it provides insights into Java packages, explaining their role in organizing code by grouping related classes and interfaces, which helps in managing access control and logical grouping.
Q & A
What is the main topic of the video series?
-The main topic of the video series is Java fundamentals, focusing on real interview questions and answers.
What are the differences between primitive data types and non-primitive data types in Java?
-Primitive data types in Java are basic types with fixed sizes and are predefined by the language, such as int, double, and boolean. Non-primitive data types, on the other hand, are objects and classes that are not defined by Java itself but by programmers using the Java API to call methods to perform certain operations.
Why can't primitive data types in Java be null?
-Primitive data types in Java cannot be null because they have default values assigned to them, such as 0 for int values, 0.0 for double values, and false for boolean values.
What is the purpose of pointers in programming, and why are they not provided in Java?
-Pointers are used in programming to store the memory address of a variable or object, allowing for more secure manipulation of data. Java does not provide support for pointers because it is designed to be a more secure language, avoiding features like pointers that could lead to issues such as memory leaks or buffer overflows.
What is the difference between an instance variable and a local variable in Java?
-An instance variable in Java is a variable that belongs to an object of a class and retains its value throughout the lifetime of the object. A local variable, on the other hand, is declared within a method and is only accessible within that method, and its value is not retained after the method call ends.
Why is it not recommended to assign default values for local variables in Java?
-Assigning default values to local variables in Java is not recommended because Java requires that local variables be properly initialized before they are used. If a local variable is not initialized, the program will throw an error, helping to prevent mix-ups and keeping the program running smoothly.
What is the role of the 'static' keyword in Java?
-The 'static' keyword in Java is used to indicate that a particular member variable or method belongs to the class rather than any instance of the class. This means that static members can be accessed without creating an instance of the class.
Can static blocks throw exceptions in Java?
-Yes, static blocks in Java can throw exceptions, but if an exception is thrown, it must be handled within the block itself, and it cannot be declared using a 'throws' clause in the class.
What is the purpose of the 'final' keyword in Java?
-The 'final' keyword in Java is used to declare variables, methods, and classes that cannot be changed, overridden, or extended. It is used to make variables constant, methods non-overridable, and classes non-inheritable.
How does the 'final' keyword contribute to immutability and thread safety in Java?
-The 'final' keyword contributes to immutability by ensuring that the value of a variable cannot be changed once it is set. In terms of thread safety, it prevents unintended modifications, which can lead to concurrency issues.
What is the significance of the 'main' method in Java?
-The 'main' method in Java is the entry point of any standalone Java application. It is the first method that gets called when the program starts, and it must be declared as public, static, and void.
Why can't the 'main' method be overridden in Java?
-The 'main' method cannot be overridden in Java because it is a static method and is associated with the class itself rather than any object of the class. Overriding is based on dynamic binding at runtime, and static methods are bound at compile time.
What is the purpose of the String pool in Java?
-The String pool in Java is a place in the heap memory where all the String literals defined in the program are stored. It helps in memory management by reusing String objects with the same content, thus saving space and increasing efficiency.
How does the Java String pool benefit memory management?
-The Java String pool benefits memory management by ensuring that identical strings are not duplicated in memory. If a new string is created that already exists in the pool, the new object reference is shared with the existing one, reducing memory usage.
What is the difference between a String and a StringBuffer in Java?
-A String in Java is immutable, meaning its value cannot be changed after it is created. A StringBuffer, on the other hand, is mutable and allows for modifications to its content, making it suitable for use in multi-threaded environments where string manipulation is required.
Why is the 'super' keyword used in Java subclasses?
-The 'super' keyword is used in Java subclasses to refer to the superclass's instance or to access the methods of the parent class. It is particularly useful when you need to call a method from the superclass that has been overridden in the subclass.
Can the 'super' keyword be used in a static context in Java?
-No, the 'super' keyword cannot be used in a static context in Java because it refers to an instance of the superclass, which does not exist in a static context. Static methods belong to the class, not instances of the class.
What are packages used for in Java?
-Packages in Java are used to organize classes and interfaces into groups, providing a structured way to manage related classes together logically. They also prevent naming conflicts and manage access control.
How do packages help in organizing Java code?
-Packages help in organizing Java code by providing a namespace that groups related classes and interfaces, making it easier to locate and manage them. They also allow developers to create modular code, which can be easily maintained and reused.
Outlines
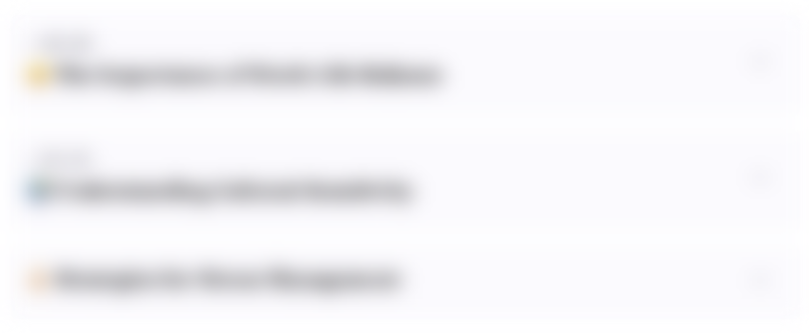
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
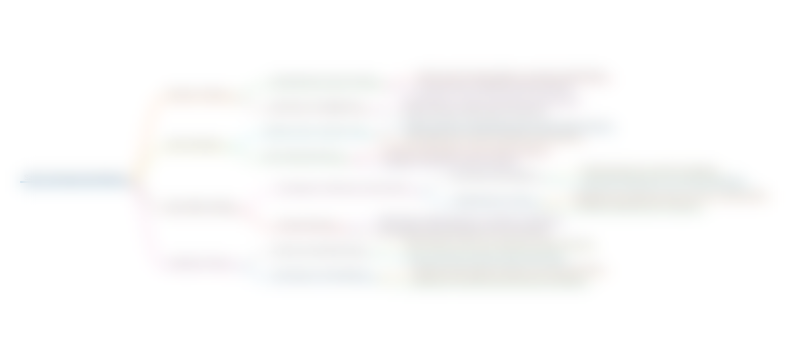
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
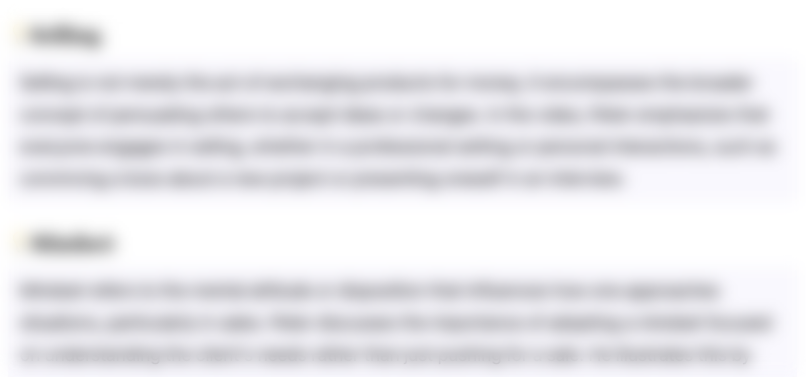
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
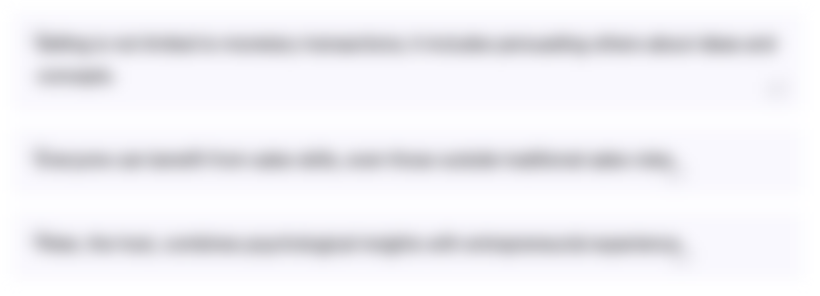
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
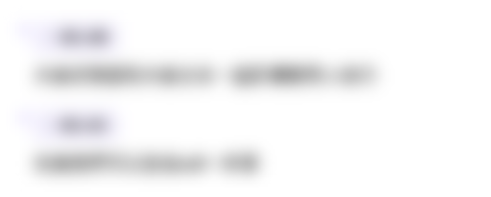
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführen5.0 / 5 (0 votes)