SOLID Design Principles Introduction
Summary
TLDRIn this tutorial, Avish introduces the SOLID design principles, essential for managing software design problems. SOLID, an acronym for five principles—Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion—promoted by Robert C. Martin, aims to make software designs understandable, flexible, and maintainable. The tutorial explains each principle, their importance, and the potential issues arising from their neglect, such as tight coupling and code duplication. It emphasizes the benefits of adhering to these principles for code complexity reduction, increased readability, extensibility, and better testability. Avish promises further exploration of these principles with examples in subsequent sessions.
Takeaways
- 📘 SOLID is an acronym for five design principles that help manage software design problems, making designs more understandable, flexible, and maintainable.
- 🔠 The acronym SOLID stands for Single Responsibility Principle, Open/Closed Principle, Liskov Substitution Principle, Interface Segregation Principle, and Dependency Inversion Principle.
- 👷♂️ Single Responsibility Principle states that a class should have only one reason to change, encapsulating a single part of the software's functionality.
- 🔄 Liskov Substitution Principle asserts that objects should be replaceable with instances of their subtypes without affecting the correctness of the program.
- 🚀 Open/Closed Principle suggests that software entities should be open for extension but closed for modification, allowing new functionality with minimal changes to existing code.
- 🍽️ Interface Segregation Principle recommends using many client-specific interfaces over a single general-purpose interface, avoiding the implementation of unused interfaces.
- 🔗 Dependency Inversion Principle emphasizes depending on abstractions rather than concretions, with high-level modules not depending on low-level modules.
- 🚫 Ignoring SOLID principles can lead to tight coupling, making it difficult to implement new features or fix bugs, and potentially causing unknown issues.
- 🛠️ Following SOLID principles results in reduced code complexity, improved readability, extensibility, maintenance, error reduction, reusability, better testability, and reduced tight coupling.
- 🏗️ Successful application development relies on three factors: choosing the right architecture, adhering to design principles, and selecting appropriate design patterns.
- 🔍 The upcoming sessions will delve deeper into each SOLID principle with examples, helping to clarify their application in software development.
Q & A
What is the primary focus of this tutorial session?
-This session focuses on understanding the SOLID design principles, including what the SOLID acronym stands for and why these principles are important for software design.
What does the SOLID acronym represent?
-The SOLID acronym represents five design principles intended to make software designs more understandable, flexible, and maintainable.
Who introduced the SOLID principles, and where did the acronym originate?
-The SOLID principles were promoted by Robert C. Martin, and the SOLID acronym was first introduced by Michael Feathers.
What does the Single Responsibility Principle (SRP) state?
-The Single Responsibility Principle (SRP) states that a class should have only one reason to change, meaning every module or class should have responsibility over a single part of the functionality provided by the software.
Can you explain the Liskov Substitution Principle (LSP)?
-The Liskov Substitution Principle (LSP) states that objects in a program should be replaceable with instances of their subtypes without altering the correctness of the program. Derived types must be substitutable for their base types.
What does the Open/Closed Principle (OCP) entail?
-The Open/Closed Principle (OCP) states that software entities should be open for extension but closed for modification, meaning new functionality can be added with minimal changes to existing code.
What is the Interface Segregation Principle (ISP)?
-The Interface Segregation Principle (ISP) states that many client-specific interfaces are better than one general-purpose interface, meaning clients should not be forced to implement interfaces they don't use.
What is the Dependency Inversion Principle (DIP) about?
-The Dependency Inversion Principle (DIP) states that one should depend on abstractions, not concretions. High-level modules should not depend on low-level modules, and abstractions should not depend on details.
What issues might arise if SOLID principles are not followed in programming?
-If SOLID principles are not followed, issues like tight coupling of code, difficulties in implementing new features, untestable code, code duplication, and new bugs emerging from fixing existing ones may arise.
How do SOLID principles contribute to software development?
-SOLID principles help reduce code complexity, increase readability, extensibility, and maintainability, reduce errors, improve reusability, and enhance testability, ultimately leading to a more robust application.
Outlines
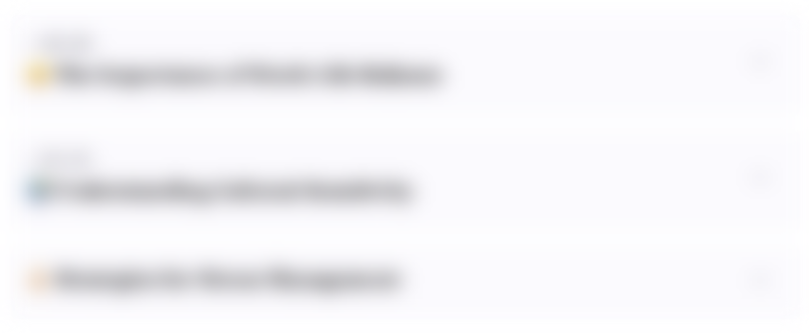
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
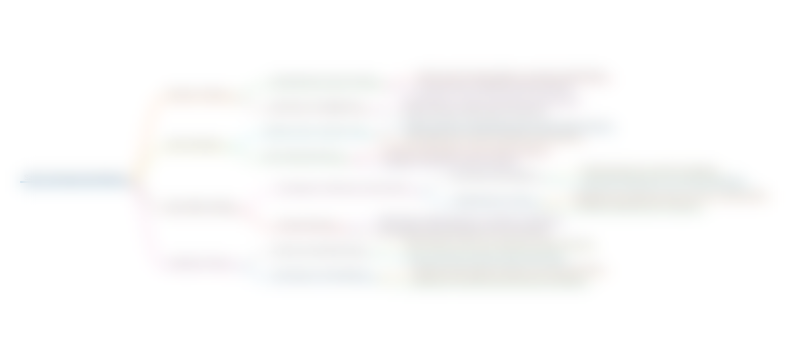
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
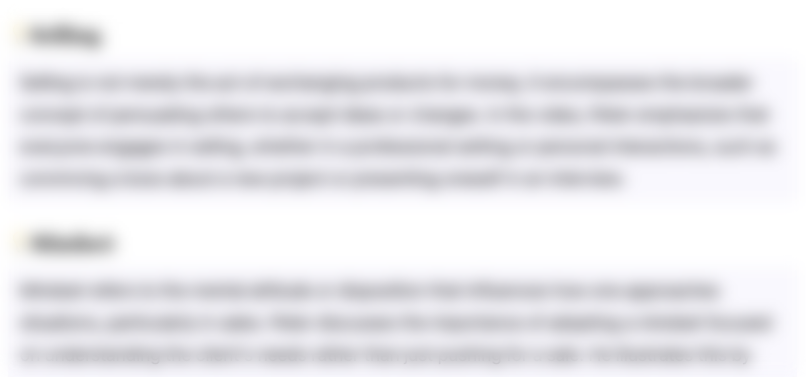
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
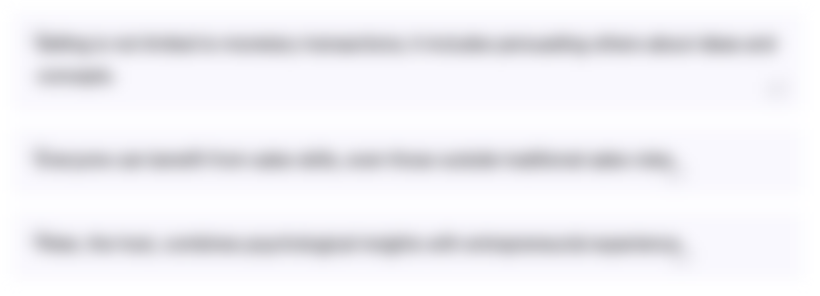
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
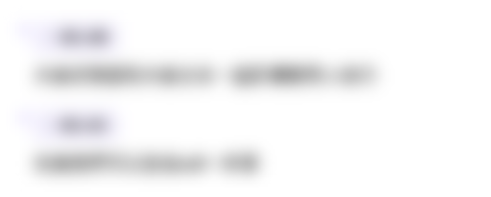
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen

TUTORIAL PHOTOSHOP #1 || Mengatur Area Kerja/Membuat Dokumen Baru || Photoshop 2023 Untuk Pemula

Single Responsibility Principle

RPL - 08 Konsep Perancangan (Design) Perangkat Lunak

Interface Segregation Principle
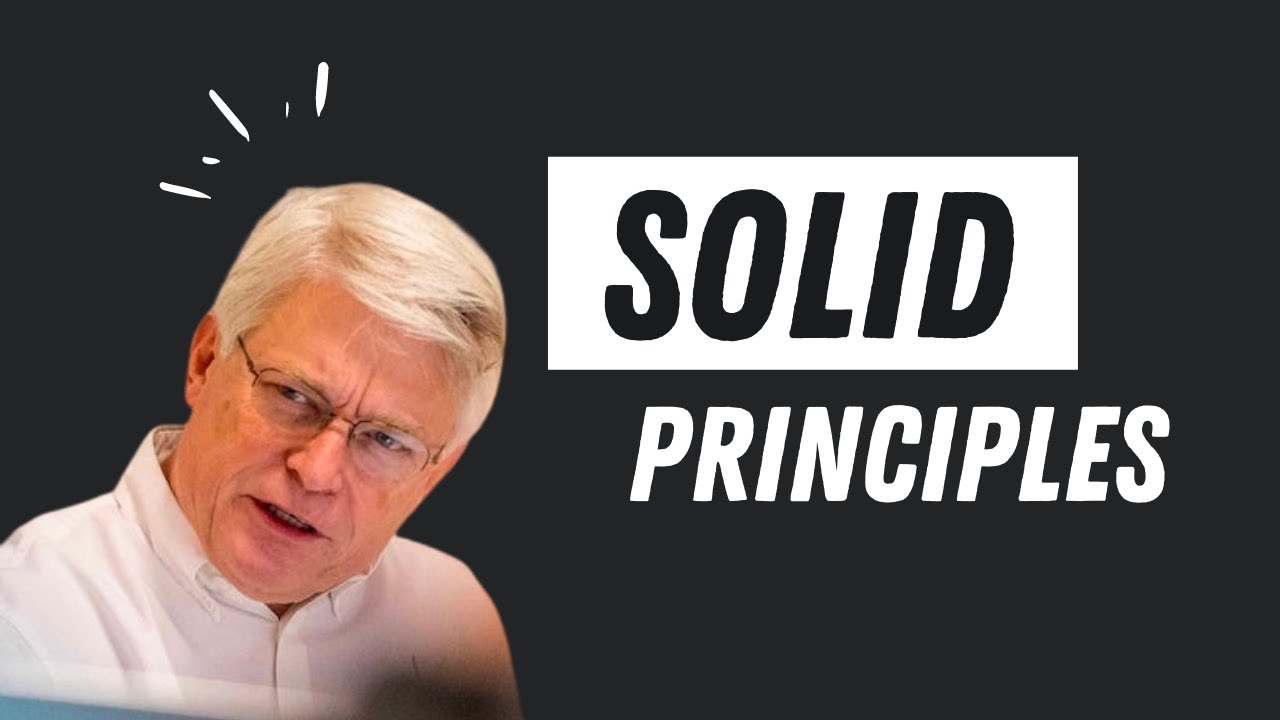
Learn SOLID Principles with CLEAN CODE Examples

Lecture 14 — Heuristic Evaluation - Why and How | HCI Course | Stanford University
5.0 / 5 (0 votes)