Interface Segregation Principle
Summary
TLDRThis tutorial, part three of the SOLID design principles series, delves into the Interface Segregation Principle (ISP). It explains ISP's core idea of preferring multiple small interfaces to one large one, using the case study of Xerox's printer system to illustrate the principle. The video demonstrates how Robert C. Martin's solution to Xerox's software complexity involved creating specific interfaces for different functionalities, instead of a single, monolithic interface. It also highlights the problems of enforcing unnecessary implementation and the need for adaptability with technology advancements, advocating for ISP to address these issues effectively.
Takeaways
- π Interface Segregation Principle (ISP) is a part of SOLID design principles, emphasizing that no client should be forced to depend on methods it does not use.
- π¨βπ« ISP was formulated by Robert C. Martin, who used it to address issues at Xerox Corporation, where a single job class was being used for various tasks, leading to bloated and inflexible code.
- π¨οΈ Xerox's problem was that a single job class was handling multiple tasks like printing, stapling, and faxing, which resulted in a large class with many unused methods for different tasks.
- π ISP suggests creating multiple, smaller interfaces that are client-specific, rather than one large interface that clients must implement in full, even if they don't use all the methods.
- π οΈ The case study of Xerox led to the creation of separate interfaces for different printer functionalities, such as stapling and faxing, which were initially part of a single class.
- π ISP helps in reducing the impact of changes in the system by segregating the interface methods based on their usage, thus improving maintainability and flexibility.
- π The tutorial recommends referring to the previous session on Single Responsibility Principle, which is closely related to ISP, as both aim to reduce complexity and improve modularity.
- π In the provided example, a basic printer interface 'IPrint' was created with methods for printing, scanning, faxing, and photocopying, but not all printers need to implement all these methods.
- πΌοΈ The example of the Canon MG 2470 printer, which cannot fax, illustrates the problem of being forced to implement methods it does not use, highlighting the need for ISP.
- π ISP can also address the issue of new functionalities being added to an interface, which would then require all implementing clients to adapt to the changes, even if they are irrelevant to them.
- π§ The solution involves creating more granular interfaces that group related methods together, allowing clients to implement only the interfaces that are relevant to their functionality.
Q & A
What is the main topic of this tutorial session?
-The main topic of this tutorial session is the Interface Segregation Principle and Interface Aggregation Principle from the SOLID design principles.
What does the Interface Segregation Principle state?
-The Interface Segregation Principle states that no client should be forced to depend on methods it does not use. It advocates for many small, client-specific interfaces rather than one large, general-purpose interface.
Who formulated the Interface Segregation Principle?
-Robert C. Martin formulated the Interface Segregation Principle.
What was the problem with the software design at Xerox Corporation as described in the script?
-The problem at Xerox was that a single job class was used for all tasks, including printing, stapling, and faxing, which made the class very large and difficult to modify. This design resulted in classes knowing about methods they did not need to.
What solution did Robert C. Martin suggest to Xerox to address the software design problem?
-Robert C. Martin suggested segregating the large job class into multiple smaller interfaces, each tailored to specific tasks, thus implementing the Interface Segregation Principle.
How does the Interface Aggregation Principle relate to the Interface Segregation Principle?
-The Interface Aggregation Principle is a way to implement the Interface Segregation Principle by creating multiple smaller, relevant interfaces that clients can implement based on their specific needs, rather than being forced to implement a large, all-encompassing interface.
What is an example of a method that was added to the IPrint interface in the script?
-An example of a method added to the IPrint interface is 'printDuplexContent', which was introduced to handle the new duplex printing capability.
Why is it problematic for a printer that cannot fax to implement the IPrint interface as it was originally designed?
-It is problematic because the original IPrint interface included a fax method, and printers that cannot fax would still be forced to implement this method, even though they do not use it.
How does the script illustrate the application of the Interface Segregation Principle in practice?
-The script illustrates this by showing how interfaces for different printer capabilities (like faxing and duplex printing) are segregated into smaller, more specific interfaces, allowing clients to implement only the interfaces that are relevant to their functionality.
What is the next principle in the SOLID series that the tutorial will cover after discussing the Interface Segregation Principle?
-The next principle in the SOLID series that the tutorial will cover is the Open/Closed Principle.
Outlines
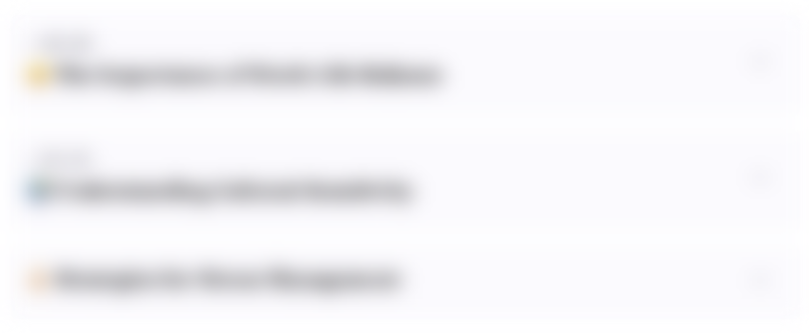
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
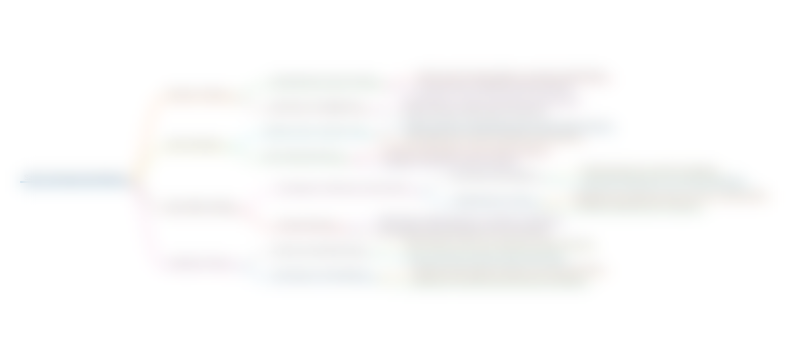
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
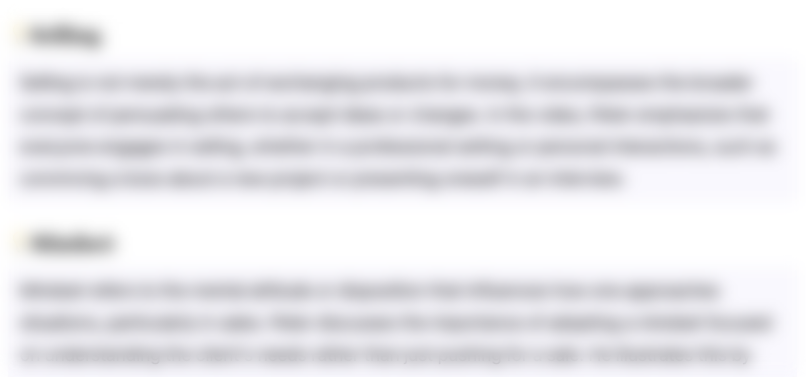
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
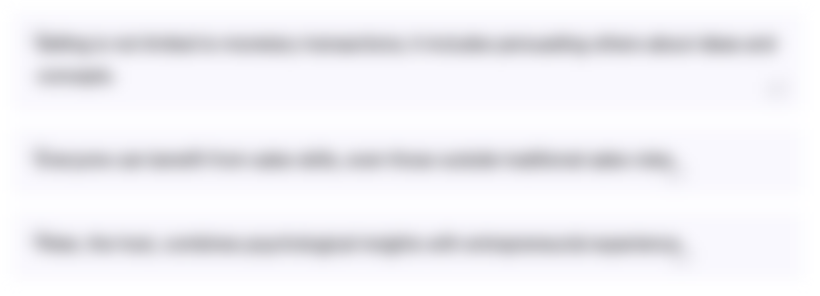
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
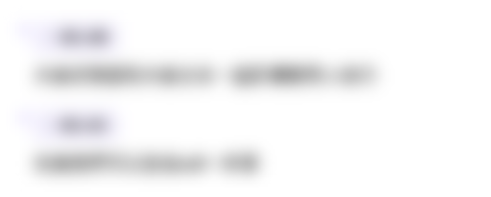
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
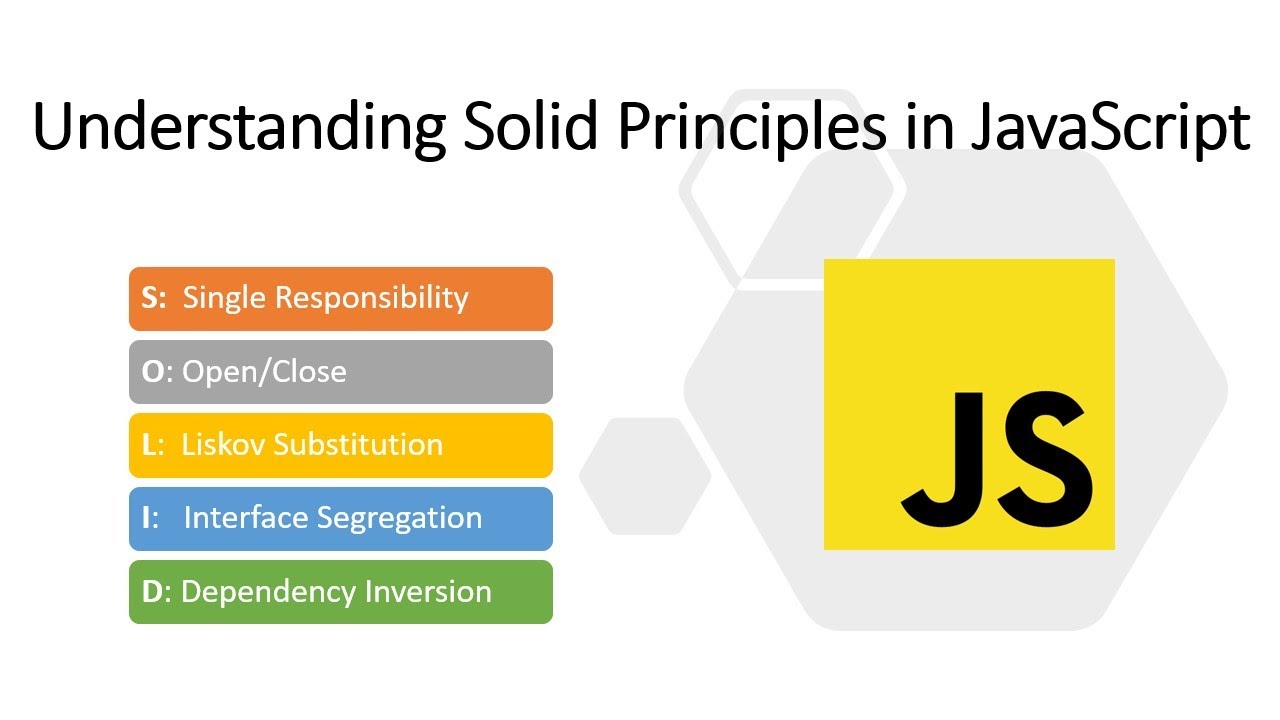
Understanding SOLID Principles in JavaScript
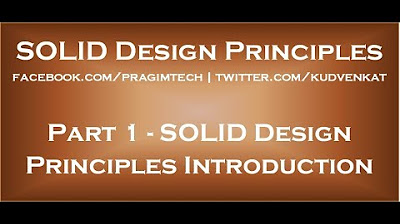
SOLID Design Principles Introduction

Single Responsibility Principle

1. SOLID Principles with Easy Examples | OOPs SOLID Principles Interview Question - Low Level Design
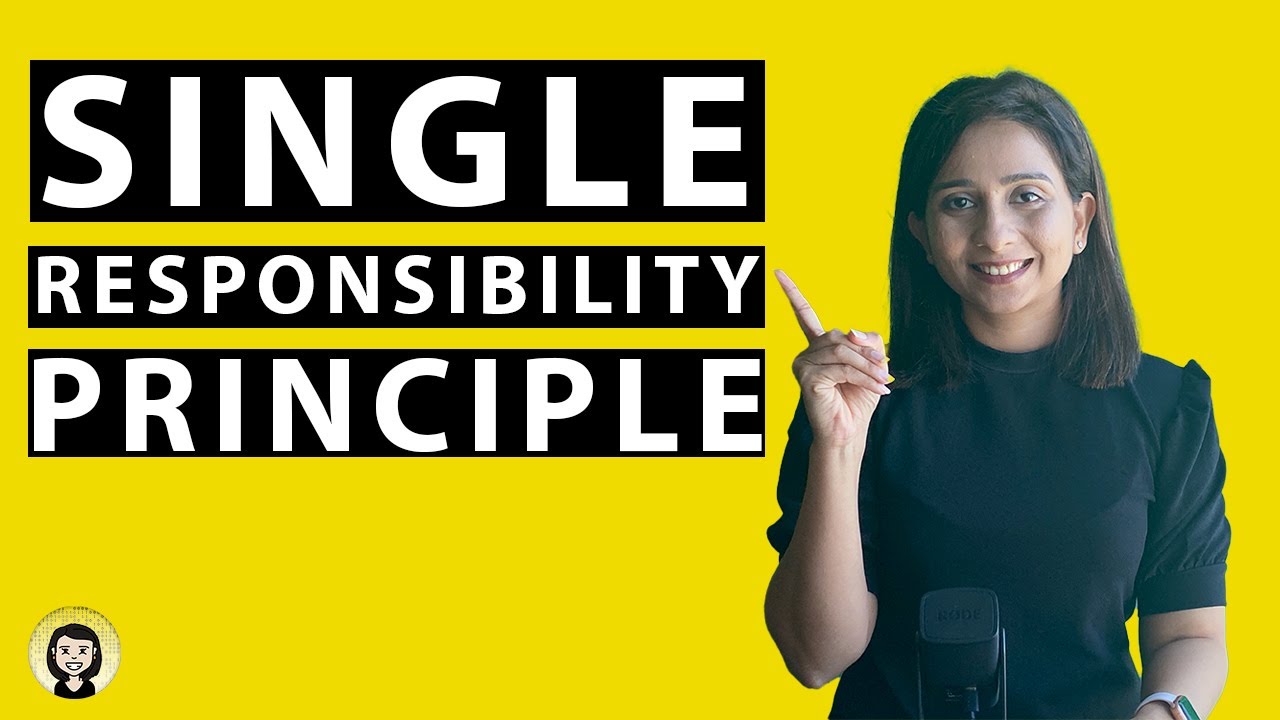
Low Level Design 105 | Single Responsibility Principle in SOLID | 2022 | System Design
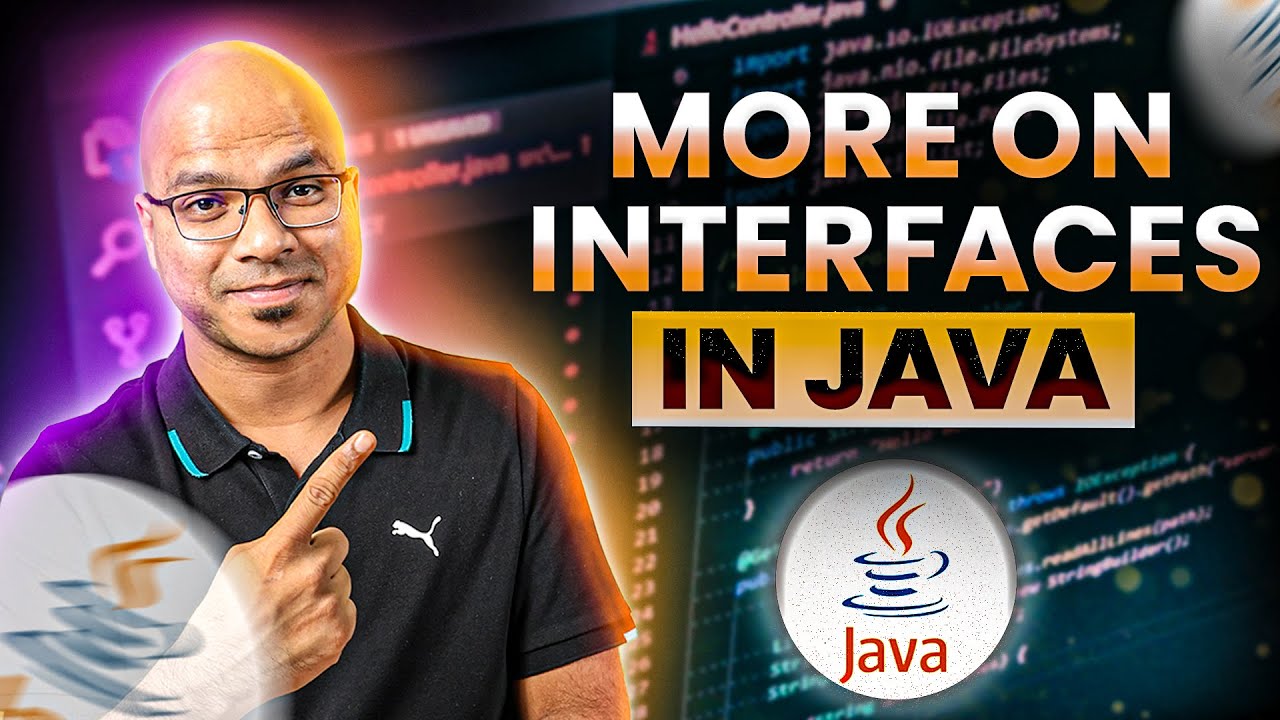
#67 More on Interfaces in Java
5.0 / 5 (0 votes)