How to insert a new node in a linked list in C++? (at the front, at the end, after a given node)
Summary
TLDRThis video tutorial delves into the intricacies of linked lists, demonstrating three distinct methods for inserting nodes: at the front, at the end, and after a specific node. The presenter provides a clear, step-by-step guide through the process, complemented by C++ code examples. Viewers unfamiliar with linked lists are directed to an introductory video, while the current tutorial focuses on practical implementation, ensuring a comprehensive understanding of node insertion techniques.
Takeaways
- đ The video discusses three methods of inserting elements into a linked list: at the front, at the end, and after a specific node.
- đšâđ« For those unfamiliar with linked lists, the creator suggests watching an earlier video for a foundational understanding and implementation guide using C++.
- đ The code for a basic linked list implementation in C++ is provided, including the creation of nodes and a 'printList' function to display list contents.
- đ The 'insertAtFront' function is explained, detailing the steps to add a new node before the current head of the list, which involves creating a new node and adjusting pointers.
- đ To insert at the front, the new node's 'next' pointer is set to the current head, and then the head pointer is updated to the new node, effectively adding it to the front.
- đ The 'insertAtEnd' function involves checking if the list is empty and then finding the last node before inserting the new node after it, ensuring the list's integrity.
- đ The process of finding the last node in a non-empty list is demonstrated using a while loop that traverses to the node pointing to null.
- đ The 'insertAfter' function requires a reference to a specific node and a value to insert after it, and it involves checking for null references and updating pointers to maintain the list structure.
- đ When inserting after a specific node, it's crucial to first set the new node's 'next' pointer to the subsequent node to avoid losing any elements in the list.
- đ ïž The video provides practical examples of invoking the 'insertAfter' function to demonstrate how elements are added to the list after a given node.
- đ The video concludes with an invitation for viewers to like, share, and comment on the video, as well as to engage with the creator on social media.
Q & A
What are the three different ways to add an element into a linked list as discussed in the video?
-The video discusses inserting a node at the front of a linked list, at the end of a linked list, and after a specific node within the list.
What is the first step in inserting a node at the front of a linked list?
-The first step is to prepare a new node by allocating memory for it and assigning the new value to the node.
How does the 'insert at the front' function update the head of the linked list?
-The function updates the head of the linked list by making the new node the head, and then making the old head the next node in the list.
What is the purpose of the 'print list' function in the script?
-The 'print list' function is used to traverse and print out all the values stored in the linked list, starting from the head node.
What is the significance of the 'head' pointer in a linked list?
-The 'head' pointer is significant as it points to the first node of the linked list, and is used to traverse the list.
How does the video script ensure that the newly added node at the front of the list doesn't lose connection with the rest of the list?
-The script ensures this by first making the new node point to the current head (thus not losing the connection), and then updating the head pointer to the new node.
What are the steps involved in inserting a node at the end of a linked list?
-The steps are: 1) Prepare a new node, 2) Check if the list is empty (if yes, make the new node the head), 3) Find the last node, and 4) Make the last node point to the new node.
Why is it necessary to check if the previous node is null in the 'insert after' function?
-It is necessary to ensure that a valid node is provided after which the new node will be inserted, as inserting after a null node would not be meaningful.
How does the 'insert after' function handle the connection between the previous node and the node following it?
-The function first stores the address of the node following the previous node in the new node, and then updates the 'next' pointer of the previous node to point to the new node.
What happens if the linked list is empty when trying to insert a node at the end?
-If the list is empty, the new node becomes the head of the list, as well as the last and the only node in the list.
Can you explain the process of finding the last node in a linked list as described in the script?
-The process involves starting at the head node and iteratively moving to the next node until a node is found that points to null, which indicates it is the last node in the list.
Outlines
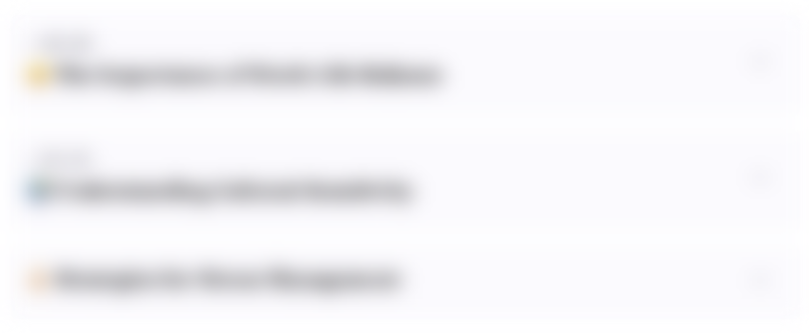
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenMindmap
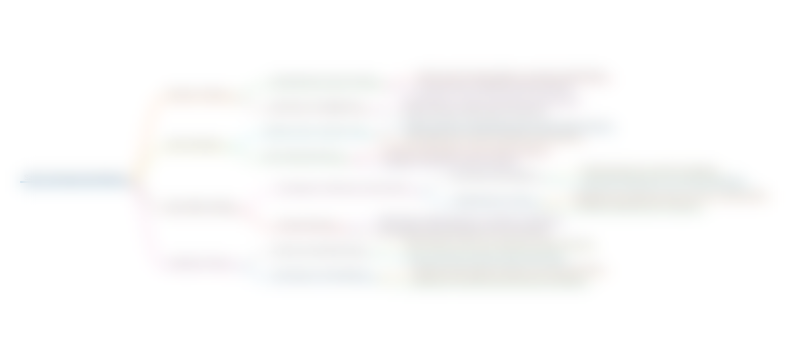
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenKeywords
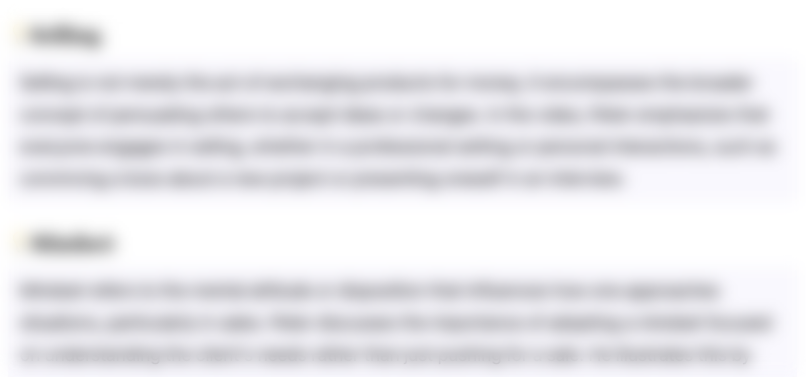
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenHighlights
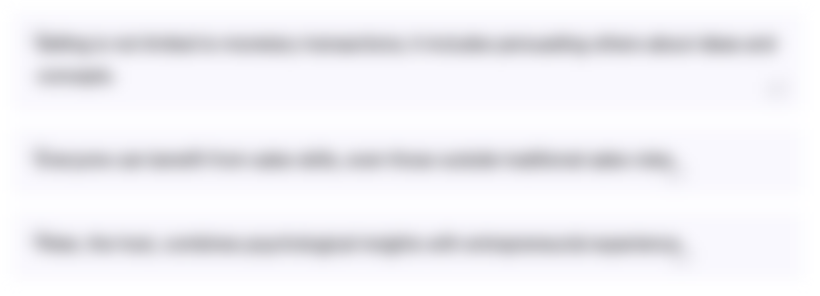
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenTranscripts
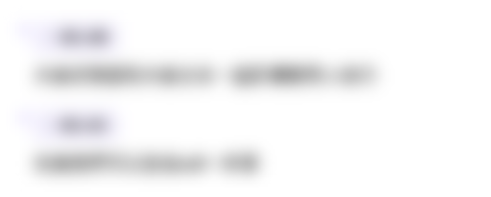
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenWeitere Ă€hnliche Videos ansehen
5.0 / 5 (0 votes)