Curso de Programação C | Como inserir no início de uma Lista Simplesmente Encadeada? | aula 243
Summary
TLDRIn this tutorial, the instructor walks through the process of implementing a linked list in C, specifically focusing on inserting an element at the beginning of the list. The step-by-step explanation covers defining the node structure, allocating memory for a new node, and handling potential errors in memory allocation. The instructor demonstrates how to update the list’s head pointer and connect the new node to the rest of the list, ensuring a thorough understanding of the procedure. This lesson is part of a series on linked lists, making it an essential watch for anyone looking to master data structures in C.
Takeaways
- 😀 Linked lists are flexible and do not have strict rules like stacks or queues, making them a more generic data structure.
- 😀 The lesson focuses on constructing a linked list and implementing insertion at the beginning of the list.
- 😀 A node structure is defined using `typedef`, where each node contains an integer value and a pointer to the next node.
- 😀 The teacher emphasizes the importance of allocating memory dynamically for new nodes in a linked list.
- 😀 The process of inserting a new element at the beginning of the list involves updating the head pointer of the list.
- 😀 Memory allocation is checked to ensure the new node is properly created, with an error message shown if allocation fails.
- 😀 To insert at the beginning, the new node's `next` pointer is set to the current head of the list, and then the head is updated to point to the new node.
- 😀 The insertion process requires passing a pointer to the pointer of the list's head so that changes made within the function reflect outside.
- 😀 The lesson encourages students to watch previous videos in the playlist to fully understand the context of working with linked lists.
- 😀 At the end of the lesson, the teacher announces that the next class will cover insertion at the end of the linked list.
Q & A
What is the main topic discussed in the script?
-The main topic is the implementation of a singly linked list in C, specifically focusing on the procedure to insert a new node at the beginning of the list.
What is a singly linked list?
-A singly linked list is a data structure in which each element (node) contains a value and a pointer to the next node in the list, allowing efficient insertion and deletion of elements.
Why is memory allocation checked when creating a new node?
-Memory allocation is checked to ensure that the system has successfully allocated space for the new node. If memory allocation fails, the program will notify the user with an error message.
What does 'typedef struct' mean in the context of the code?
-'typedef struct' is used to define a new data type (struct) called 'no' which represents a node in the linked list. The structure contains an integer value and a pointer to the next node.
What is the significance of using a pointer to pointer in the insertion function?
-Using a pointer to pointer allows the function to modify the original list pointer (the head of the list). This is necessary because inserting at the beginning of the list changes the head of the list, so the function needs to update it directly.
How does the 'inserir no início' function work?
-The function creates a new node, checks for successful memory allocation, assigns the new node's value, links it to the current head of the list, and then updates the head of the list to point to the new node.
What is the role of the 'novo->proximo' pointer in the insertion process?
-The 'novo->proximo' pointer is used to link the new node to the current first node of the list. It is set to point to the current head of the list, which becomes the second node after the new insertion.
Why is the function defined as 'void'?
-The function is defined as 'void' because it does not return a value. Its purpose is to modify the list directly by inserting a new node at the beginning.
What will happen if the memory for the new node is not successfully allocated?
-If memory allocation fails, the program will print an error message 'Error allocating memory' and the insertion process will not continue.
What is the next topic that will be covered in the following lesson?
-The next lesson will cover the process of inserting a node at the end of the linked list.
Outlines
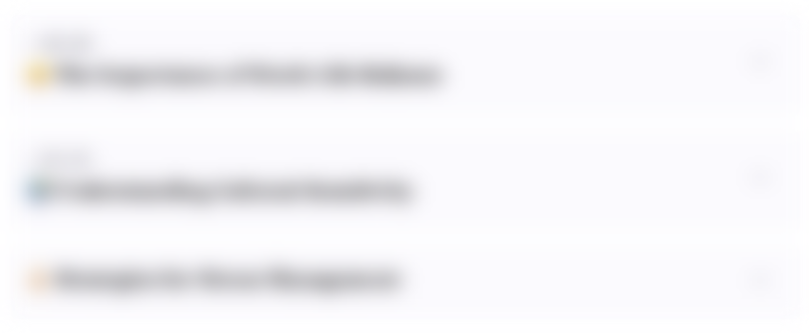
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
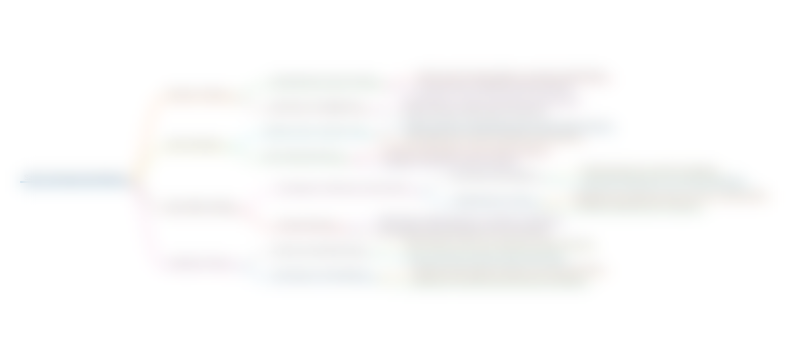
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
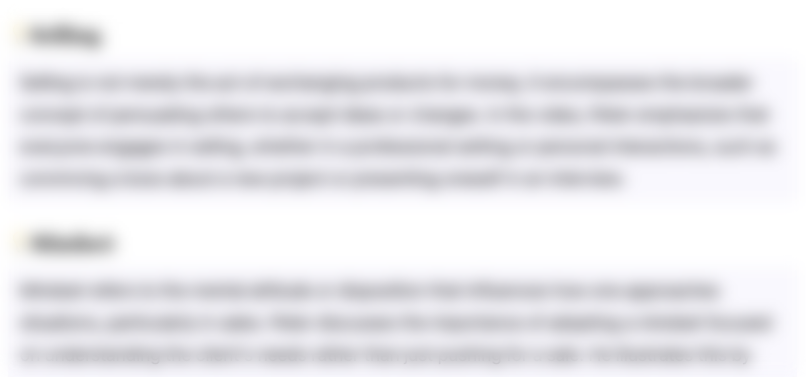
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
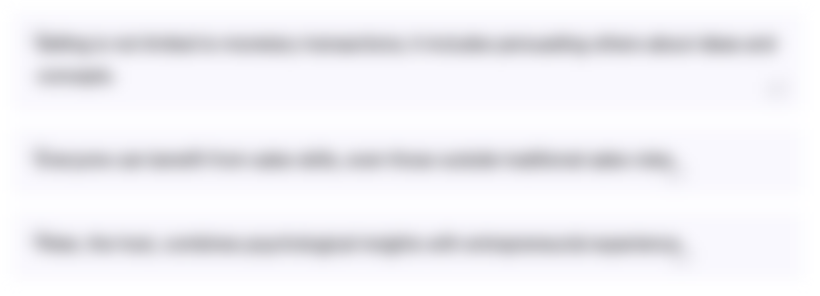
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
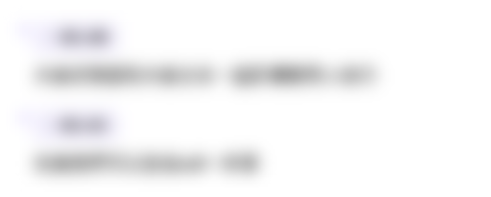
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Struktur Data Pertemuan 4

10. INF-ED | Lista doblemente enlazada: explicación de la estructura de datos.

Data Structures in Python: Doubly Linked Lists -- Append and Prepend
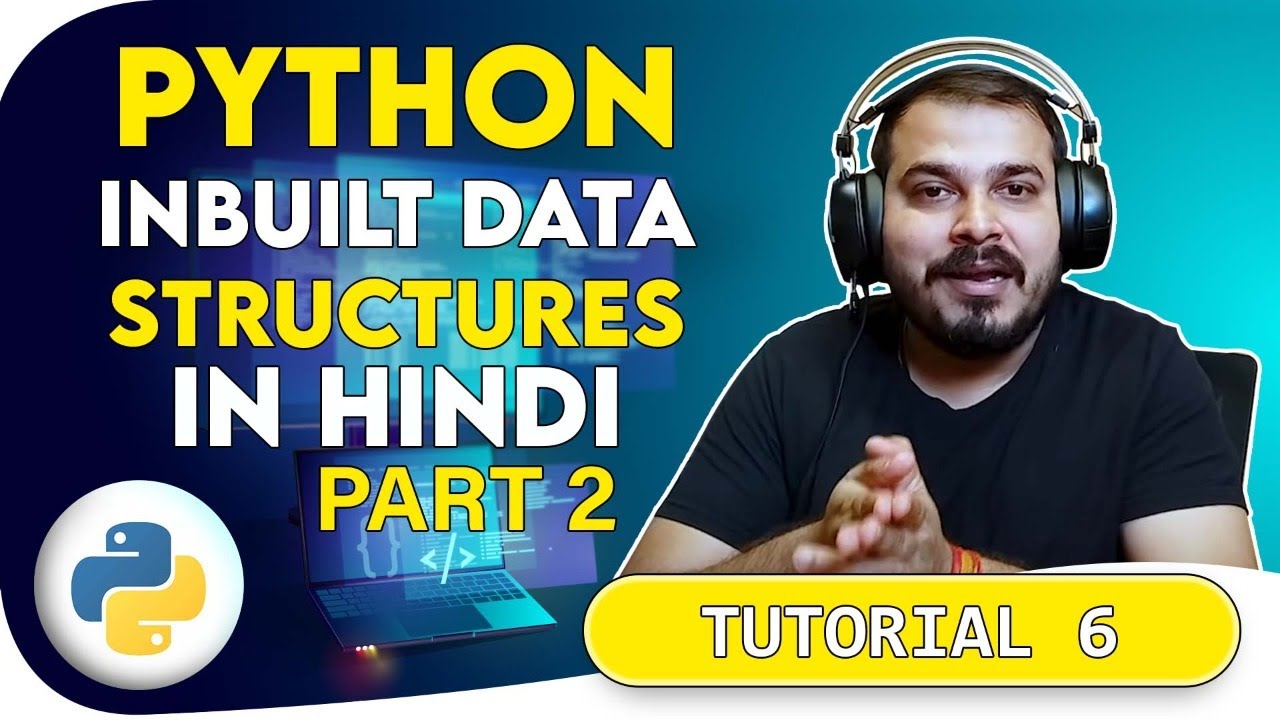
Tutorial 6- Python List And Its Inbuilt Function In Hindi
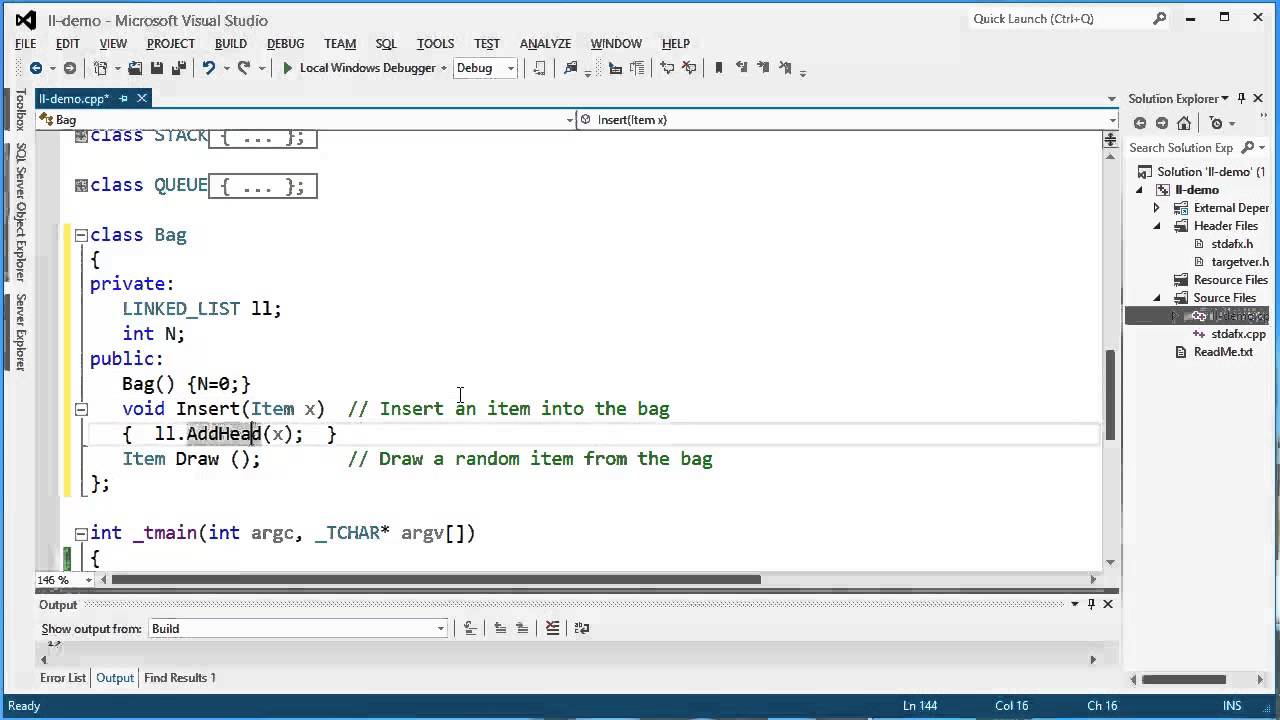
The Bag ADT
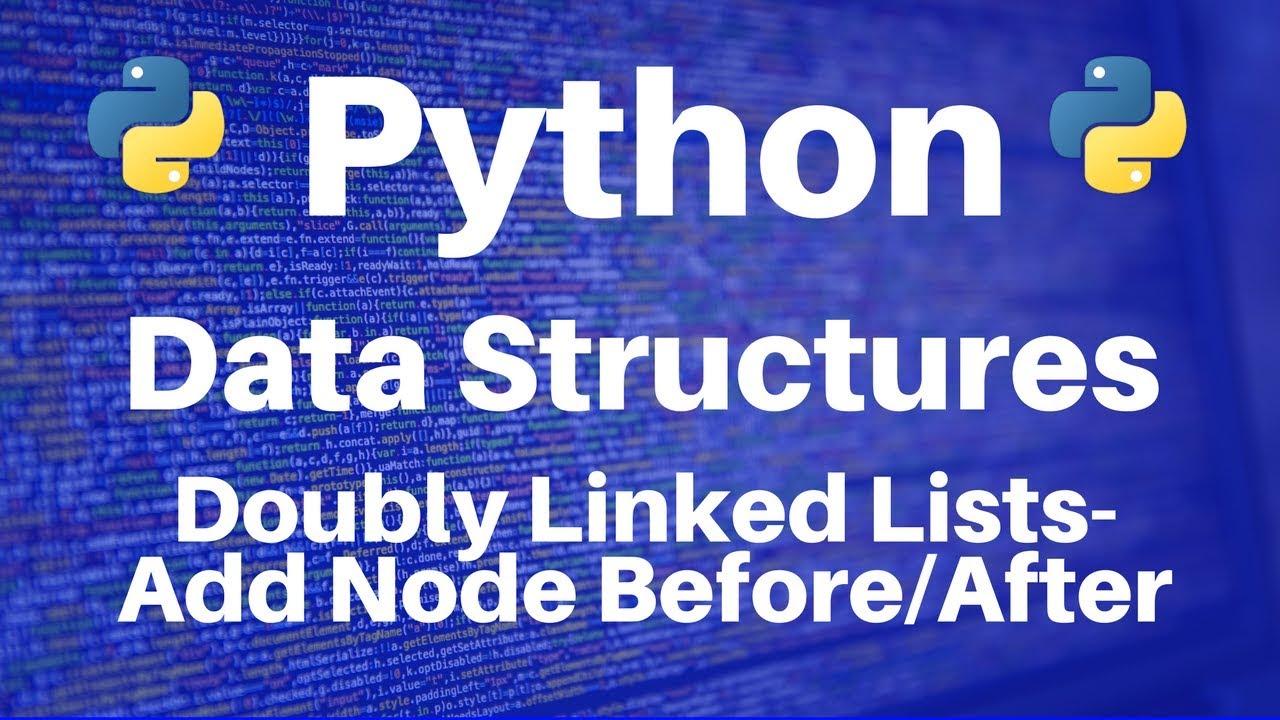
Data Structures in Python: Doubly Linked Lists -- Add Node Before/After
5.0 / 5 (0 votes)