C_82 What is Dangling pointer in C | C Language Tutorials
Summary
TLDRThis video discusses special pointers in C, focusing on the concept of dangling pointers. It explains what a dangling pointer is, the causes, and how to prevent issues related to them. Examples and code demonstrations are provided to illustrate how pointers can point to freed memory locations, leading to undefined behavior and potential bugs. The video also highlights good practices to avoid dangling pointers, such as setting pointers to null after freeing memory. Additionally, there's information about a scholarship test for GATE aspirants organized by An Academy.
Takeaways
- 📌 A dangling pointer occurs when a pointer still points to a memory location after it has been freed.
- 📌 Dangling pointers can lead to unpredictable behavior, including program crashes or garbage values.
- 📌 The `malloc` function dynamically allocates memory in C, and its memory should be freed using the `free` function to avoid memory leaks.
- 📌 Always set a pointer to `NULL` after freeing its memory to prevent it from becoming a dangling pointer.
- 📌 Checking if a pointer is `NULL` before dereferencing it is a good programming practice to avoid errors.
- 📌 A pointer becomes dangling if it points to a local variable that goes out of scope.
- 📌 Returning the address of a local variable from a function can create a dangling pointer because the local variable's memory is freed once the function returns.
- 📌 Static variables retain their memory throughout the program's execution, preventing them from becoming dangling pointers.
- 📌 The `sizeof` operator in C returns the size of a data type, which is useful for memory allocation.
- 📌 Using `static` keyword for variables ensures they have a global lifetime and do not get deallocated when going out of scope.
Q & A
What is a dangling pointer in C?
-A dangling pointer in C is a pointer that continues to point to a memory location after the memory has been freed or deallocated. Accessing this memory can lead to undefined behavior, such as program crashes or unexpected results.
What are the main causes of dangling pointers?
-The main causes of dangling pointers include deallocating memory using the free() function while still holding the pointer, using local variables' addresses outside their scope, and returning addresses of local variables from functions.
How can you prevent dangling pointers after freeing memory?
-To prevent dangling pointers after freeing memory, you can set the pointer to NULL immediately after freeing the memory. This ensures that the pointer does not point to an invalid memory location.
What happens if you dereference a dangling pointer?
-Dereferencing a dangling pointer can lead to undefined behavior, such as accessing invalid or garbage data, causing program crashes, or other unpredictable results.
How does dynamic memory allocation work with malloc()?
-Dynamic memory allocation using malloc() involves requesting a block of memory from the heap. The malloc() function returns a pointer to the beginning of the allocated memory block. The size of the memory block is specified in the malloc() call.
What is the purpose of typecasting the return value of malloc()?
-The malloc() function returns a void pointer, which is a generic pointer type. To use the allocated memory for a specific data type, you need to typecast the void pointer to the appropriate type, such as int*, float*, etc.
Why is it important to check if a pointer is NULL before dereferencing it?
-It is important to check if a pointer is NULL before dereferencing it to prevent dereferencing an invalid memory location. Dereferencing a NULL pointer typically leads to a program crash or segmentation fault.
What can happen if you use the address of a local variable outside its scope?
-Using the address of a local variable outside its scope can lead to dangling pointers because the memory for the local variable is deallocated when the function or block ends. Accessing this memory outside the scope can result in undefined behavior.
How can declaring a variable as static affect its scope and prevent dangling pointers?
-Declaring a variable as static extends its lifetime to the entire duration of the program. This means the memory for the static variable is not deallocated when the function or block ends, thus preventing dangling pointers when returning its address.
What is a good practice to follow when working with pointers to avoid bugs and crashes?
-A good practice when working with pointers is to always initialize pointers to NULL if they are not immediately assigned a valid memory address. Additionally, before dereferencing a pointer, always check if it is NULL to avoid accessing invalid memory locations.
Outlines
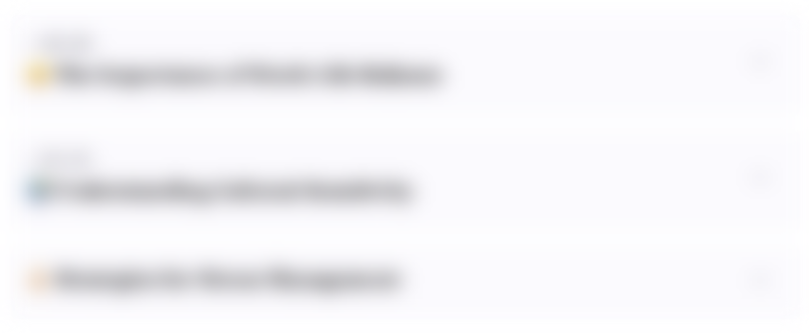
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
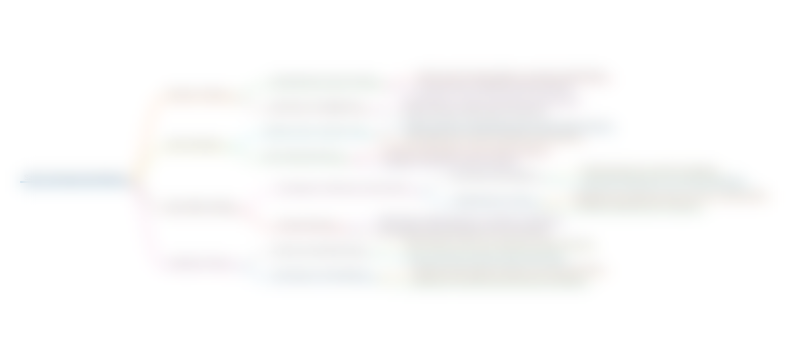
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
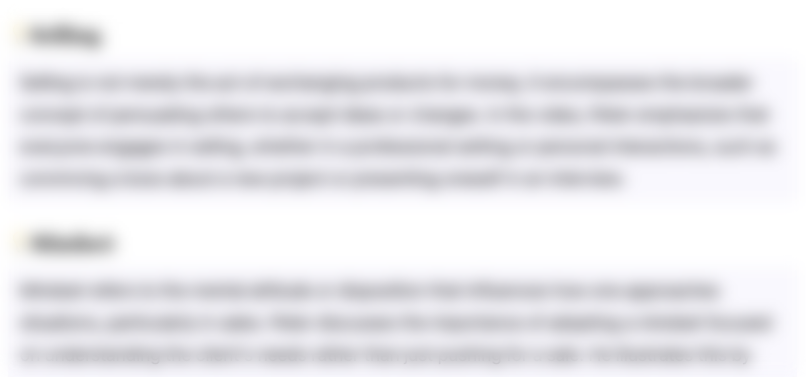
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
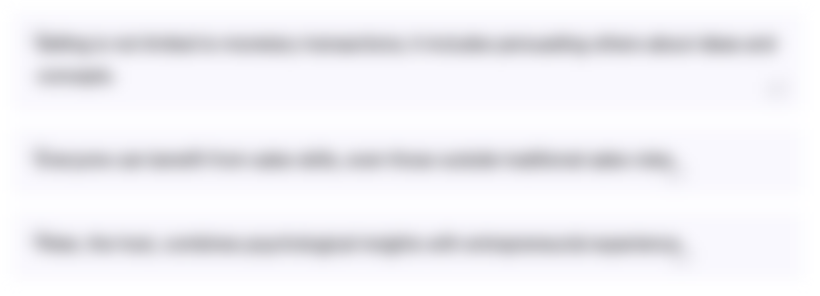
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
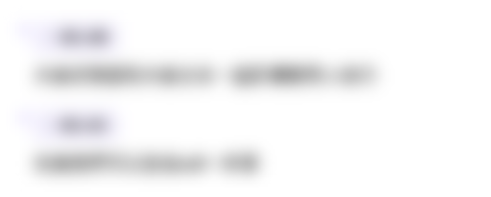
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen

Pointers and Dynamic Memory in C++ (Memory Management)
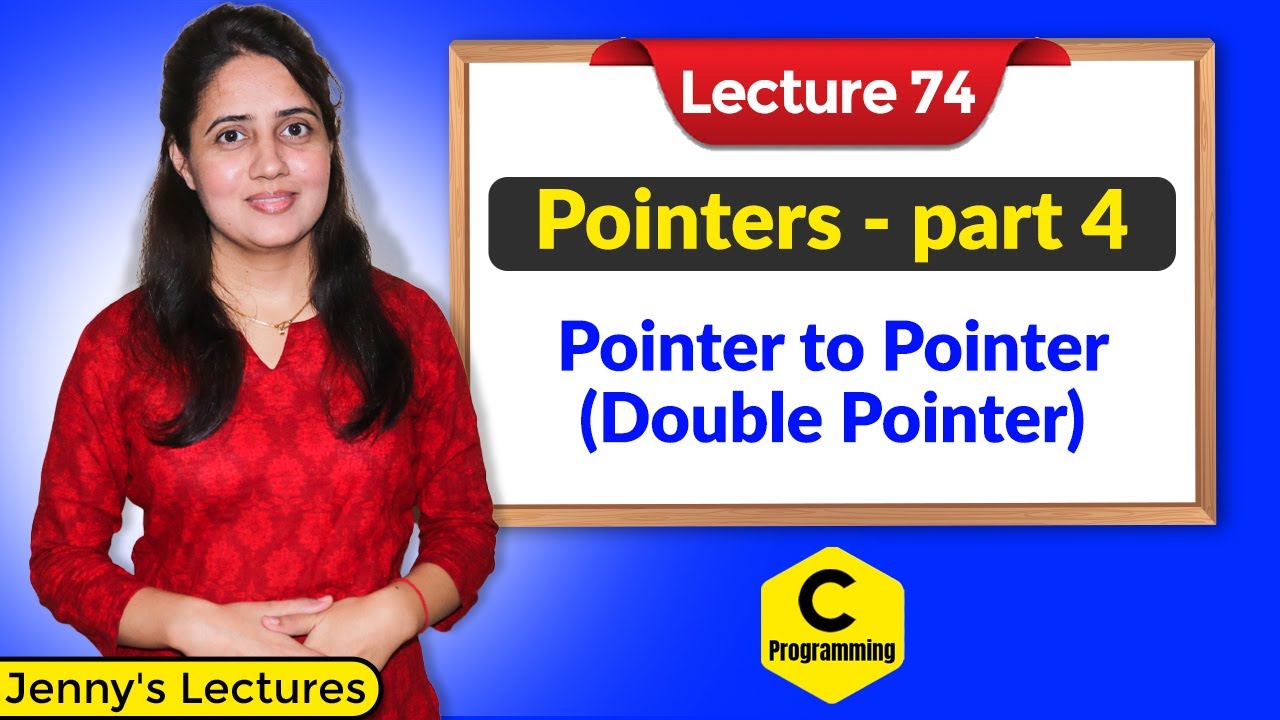
C_74 Pointers in C- part 4 | Pointer to Pointer (Double Pointer)
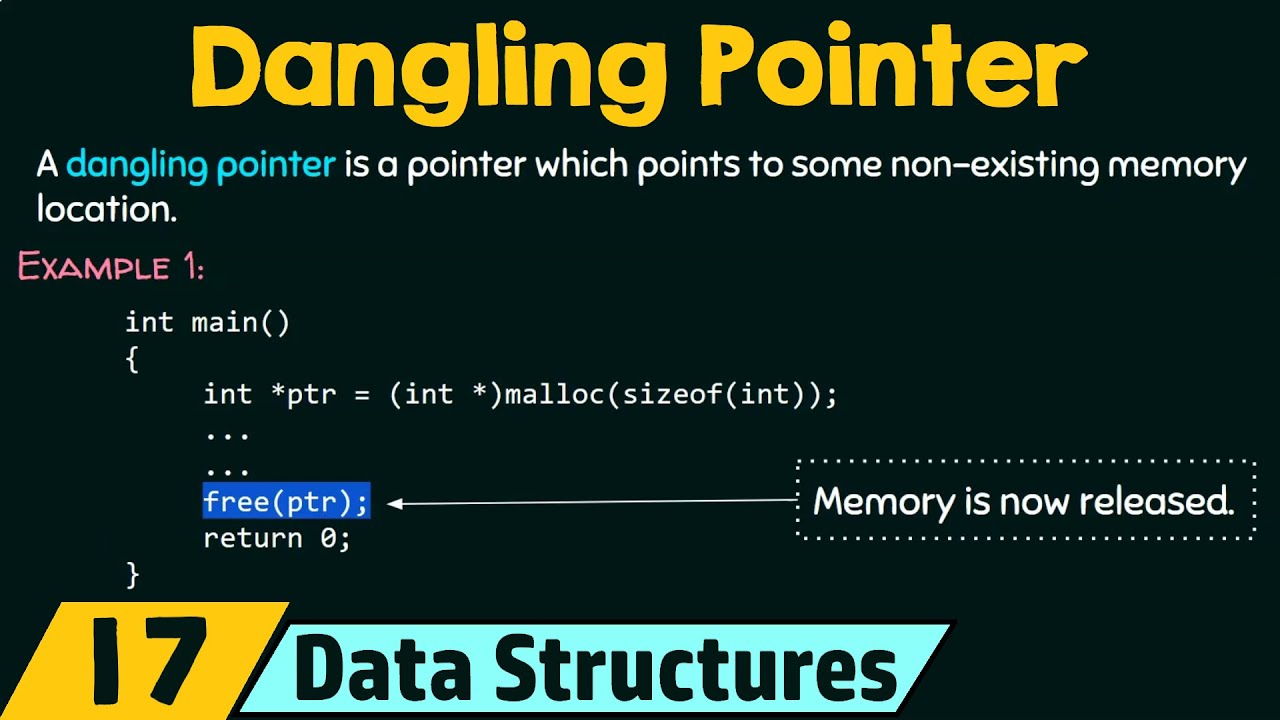
Understanding the Dangling Pointers
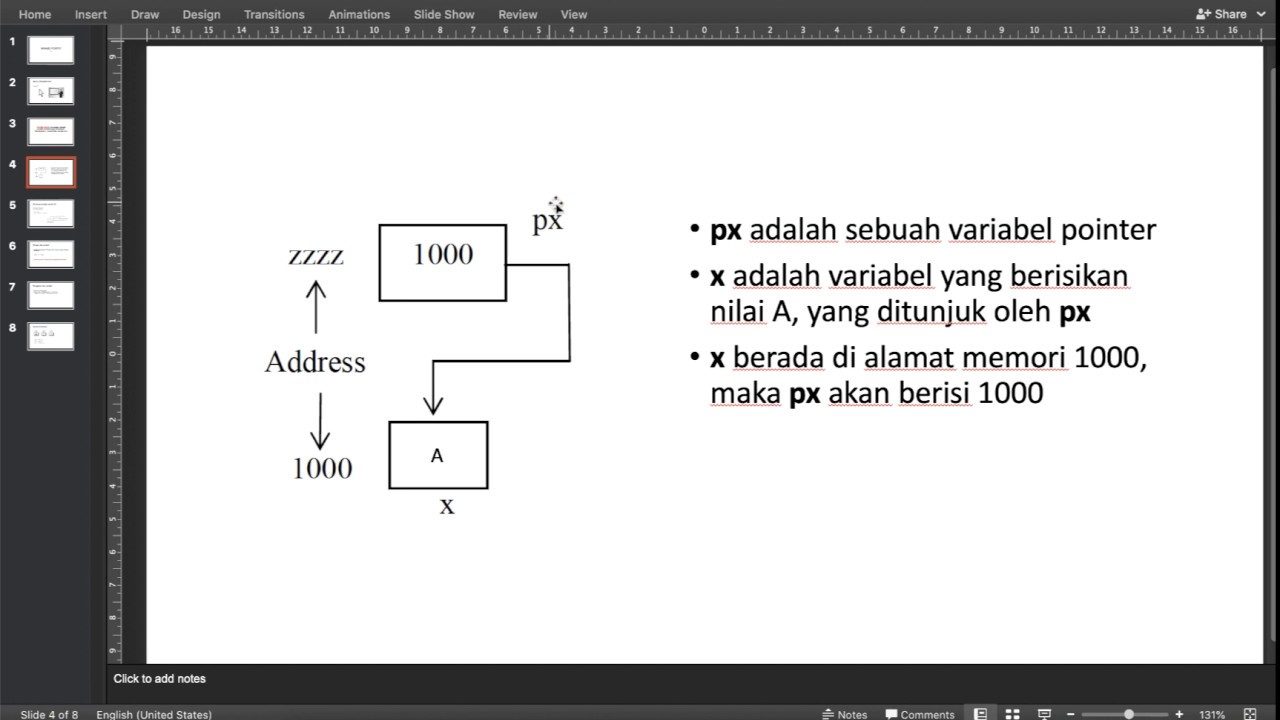
Variabel Pointer
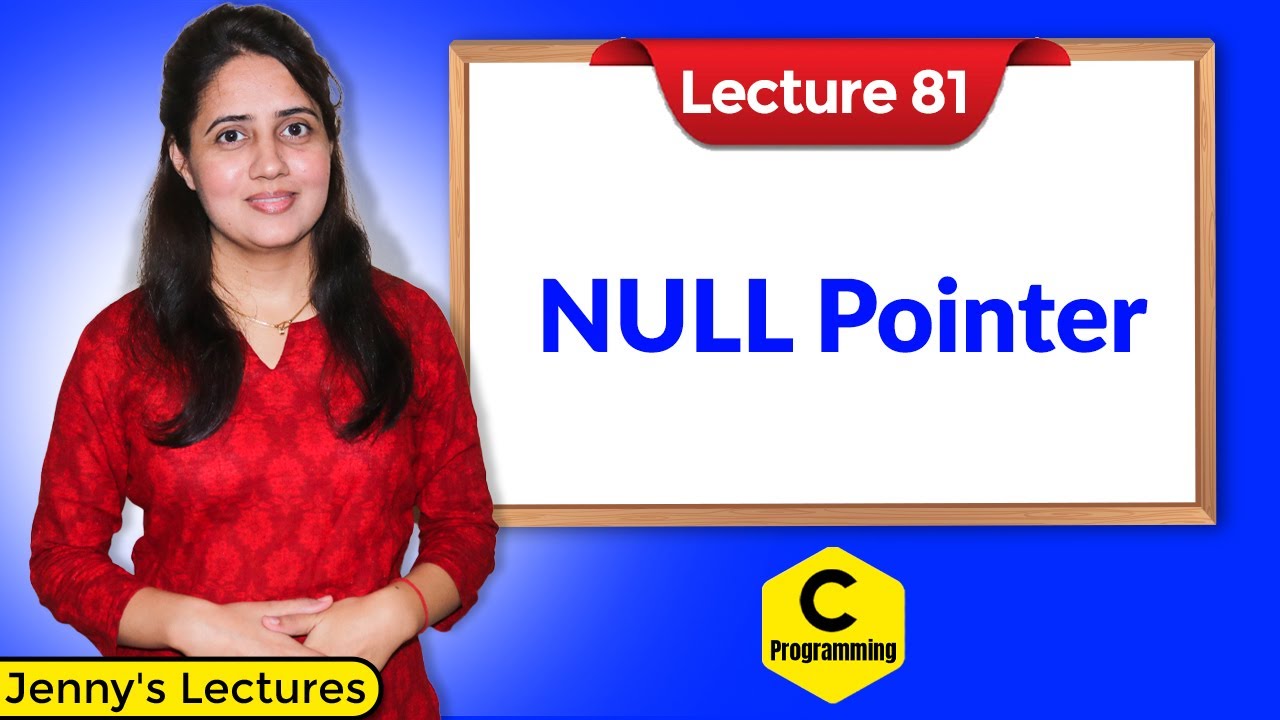
C_81 Null Pointer in C | C Programming Tutorials
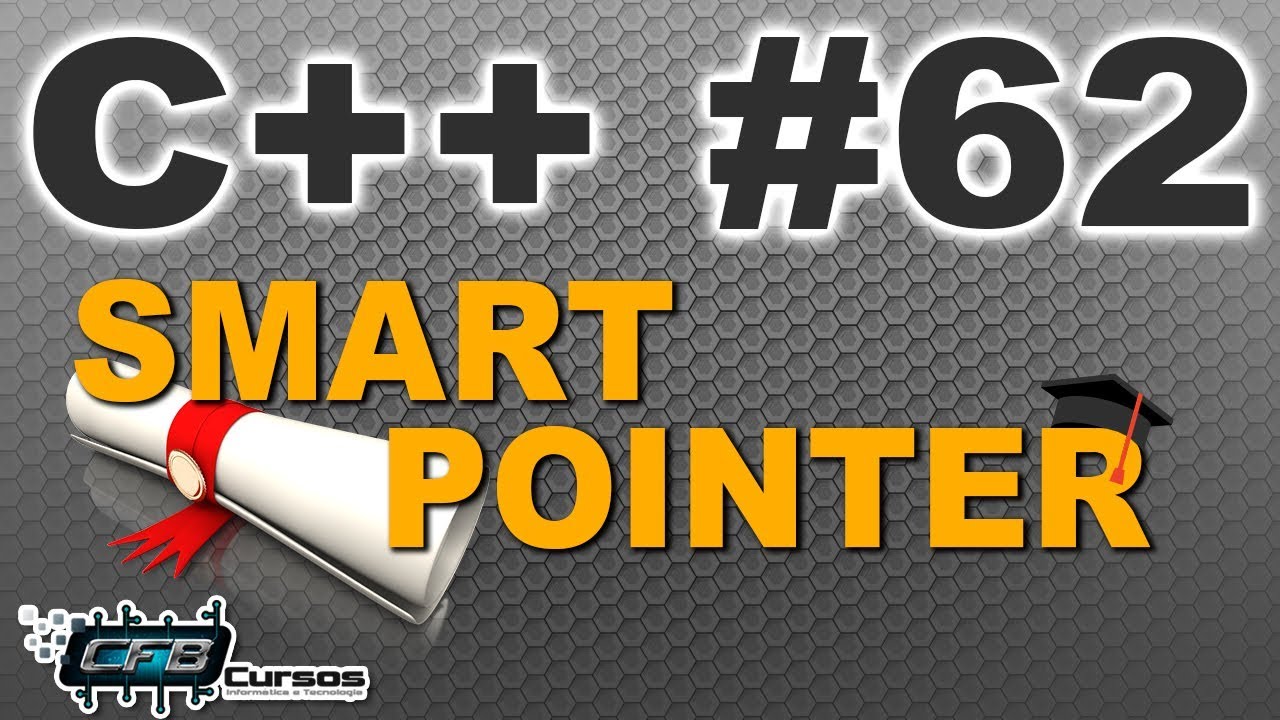
Curso de C++ #62 - Map - Smart Pointer / Ponteiro Inteligente - C++11 - (C++ Moderno)
5.0 / 5 (0 votes)