Professional Expense Tracker in Python
Summary
TLDRIn this tutorial video, the host guides viewers through building a command-line expense tracking tool using Python and SQLite3. The project is designed for beginners, focusing on creating a database, establishing a connection, and implementing a user interface with a menu for entering new expenses and viewing summaries. The script covers SQL statements for inserting data and querying expenses by month and category, concluding with a demonstration of the tool in action.
Takeaways
- 😀 The video is a tutorial on building an expense tracking tool in the command line using Python.
- 🛠️ The project utilizes SQLite3 for database management to store expense data persistently.
- 📝 It starts by creating a Python file named 'create_DB.py' to set up the database and table structure.
- 🔑 The table 'expenses' has columns for ID, date, description, category, and price, with ID as the primary key.
- 🔍 The script includes a main menu for the user to choose between entering a new expense or viewing expenses.
- 📅 When entering a new expense, users are prompted to input the date, description, and category, with the option to create new categories.
- 💰 The price input is handled with prepared statements to prevent SQL injection.
- 📊 There are options to view all expenses or view monthly expenses by category, providing summaries of spending.
- 🔄 The application includes a loop for continuous use, allowing users to add multiple expenses and view summaries repeatedly.
- 🔒 The connection to the database is properly closed after operations to ensure data integrity.
- 📚 The tutorial is aimed at beginners, providing a practical project to practice Python and database skills.
Q & A
What is the main purpose of the video?
-The main purpose of the video is to guide viewers on how to build an expense tracking tool in the command line using Python and SQLite database, which is suitable for beginners to practice their coding skills.
What is the first step in creating the expense tracking tool?
-The first step is to create a new Python file called 'create_DB' to establish a connection with the SQLite database and set up the initial database structure using SQL commands.
What does the 'expenses' table in the database consist of?
-The 'expenses' table consists of columns for 'ID' as an integer and primary key, 'date', 'description' as text, 'category' as text, and 'price' as a real (decimal) data type.
How does the video script handle the user input for the date of the expense?
-The script prompts the user to enter the date of the expense in the format YYYY-MM-DD, which is a four-digit year, two-digit month, and two-digit day.
What is the method used to display existing categories to the user?
-The script uses a SQL query with 'SELECT DISTINCT category FROM expenses' to fetch unique categories and then enumerates them to create a menu for the user to select from or create a new category.
How does the script handle the insertion of a new expense into the database?
-The script collects the date, description, category, and price from the user, uses prepared statements to avoid SQL injections, and then executes an 'INSERT INTO' statement to add the new expense to the 'expenses' table.
What are the two main options provided in the user menu for the expense tracking tool?
-The two main options are to 'enter a new expense' and to 'view expenses summary', which includes viewing all expenses or monthly expenses by category.
How does the script display the summary of expenses?
-The script provides two options to display the summary: viewing all expenses or viewing monthly expenses grouped by category. It uses SQL queries to fetch and display the data accordingly.
What is the purpose of using prepared statements in the script?
-The purpose of using prepared statements is to securely pass user inputs into SQL queries, which helps to prevent SQL injection attacks by ensuring inputs are safely typecast and handled.
How does the script handle the user's choice to exit the tool?
-The script includes an 'if' condition to check if the user's choice is invalid or if they choose to exit by entering an option that is not recognized or by answering 'no' to the prompt 'would you like to do something else?'.
What is the final step in the script after all operations are completed?
-The final step is to close the database connection using 'connection.close()' to ensure all data is saved and the connection is properly terminated.
Outlines
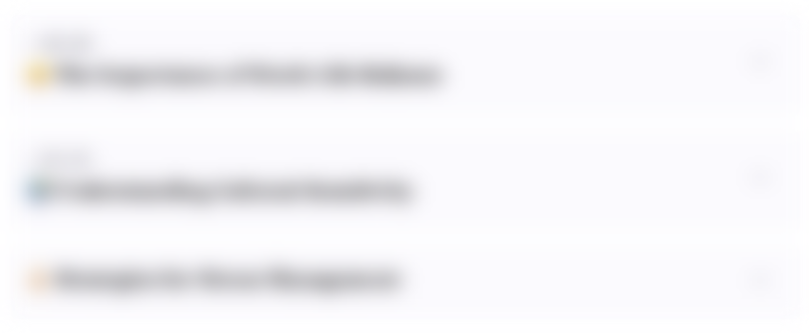
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
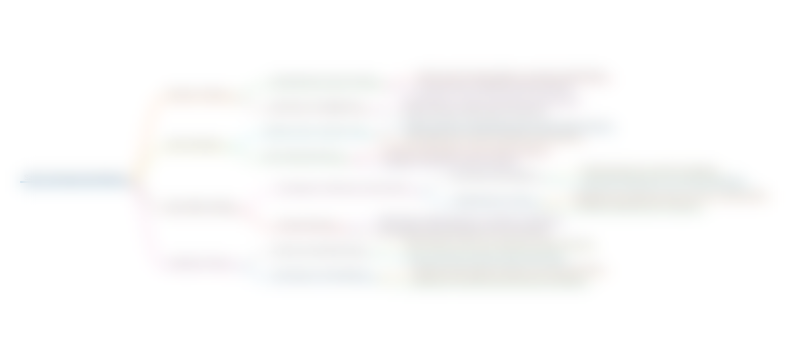
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
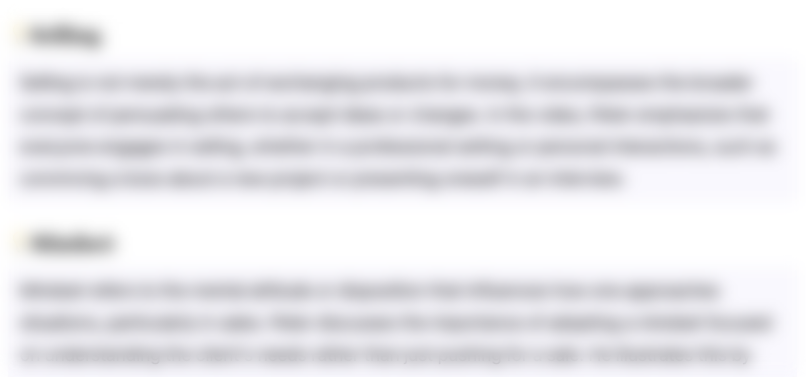
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
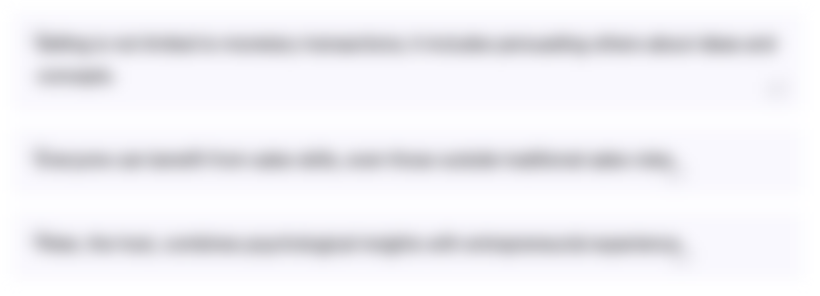
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
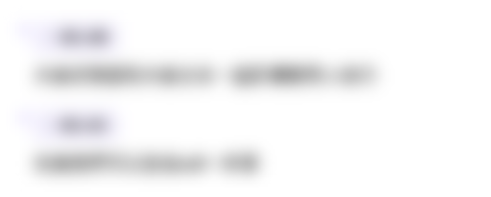
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
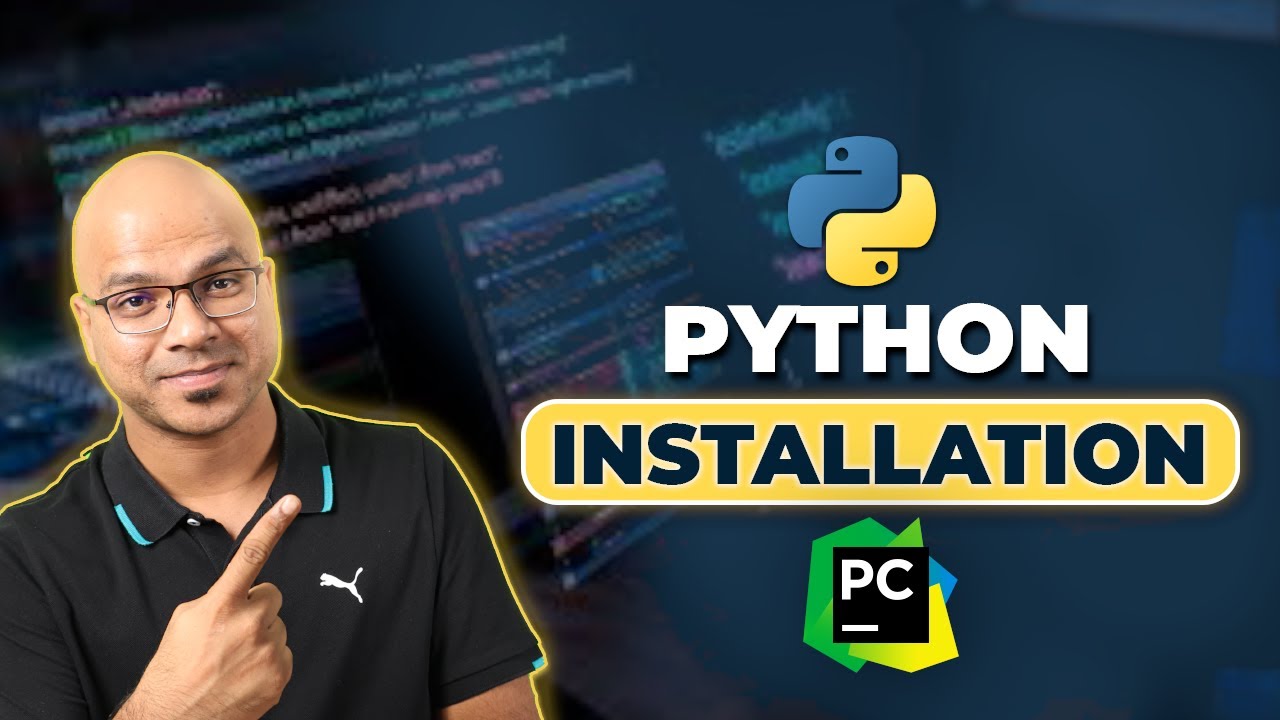
#2 Python Tutorial for Beginners | Python Installation | PyCharm
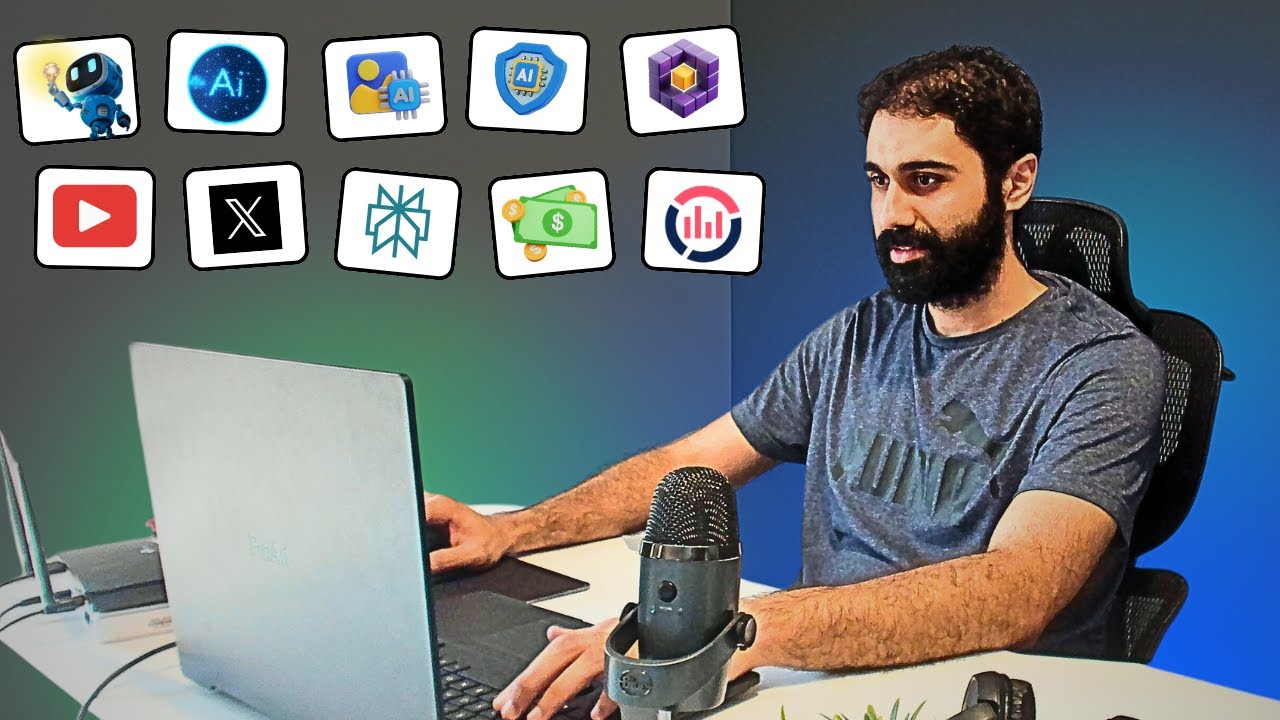
I built 10 AI Tools, and am giving it for free!
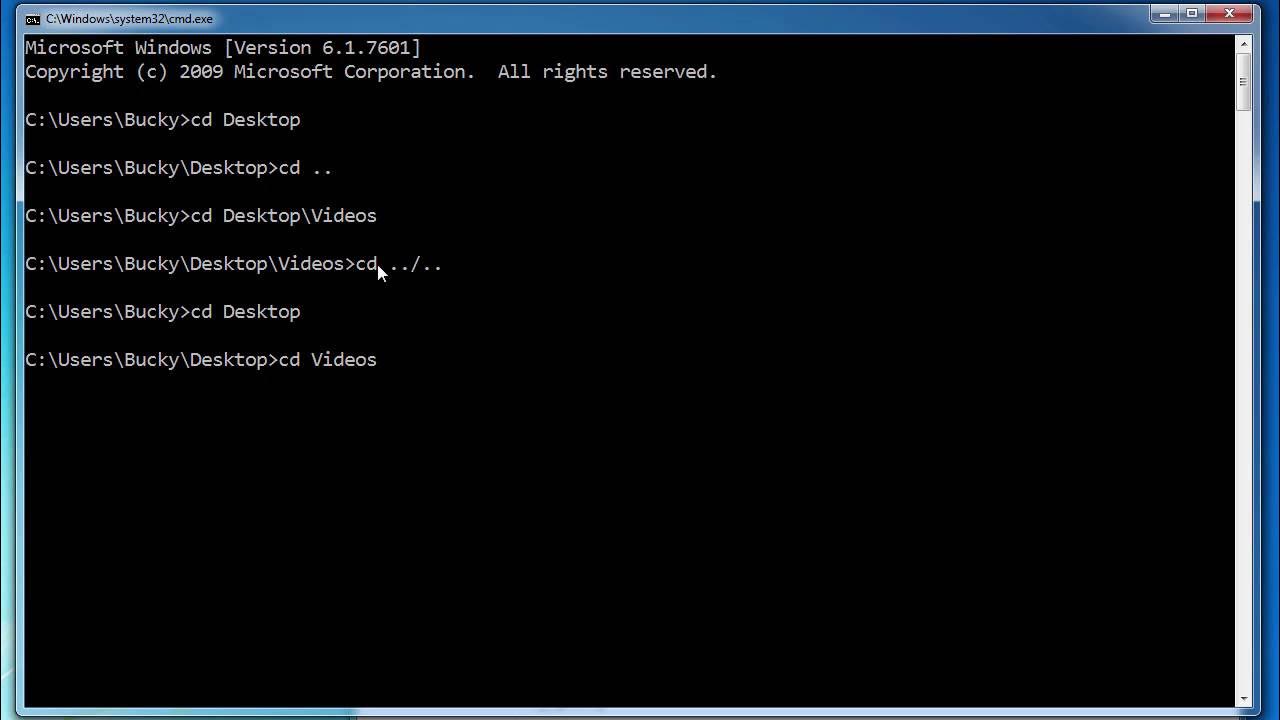
Windows Command Line Tutorial - 1 - Introduction to the Command Prompt
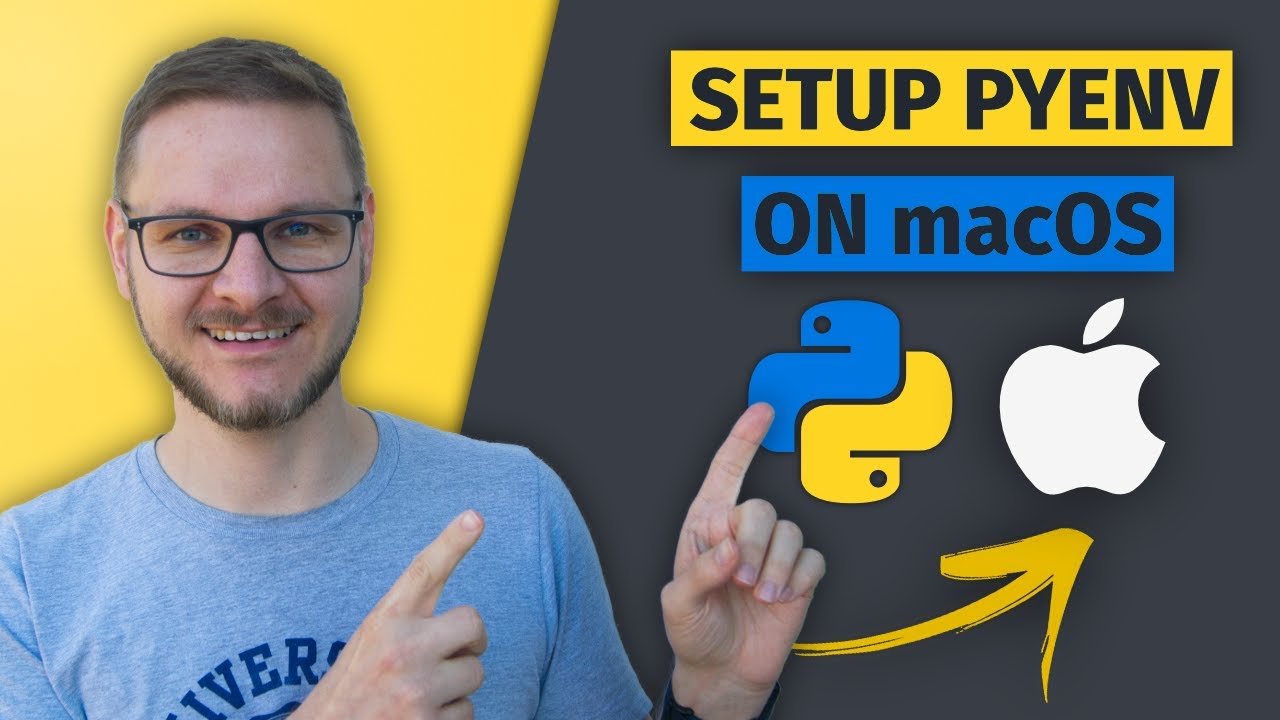
How to Install and Run Multiple Python Versions on macOS | pyenv & virtualenv Setup Tutorial
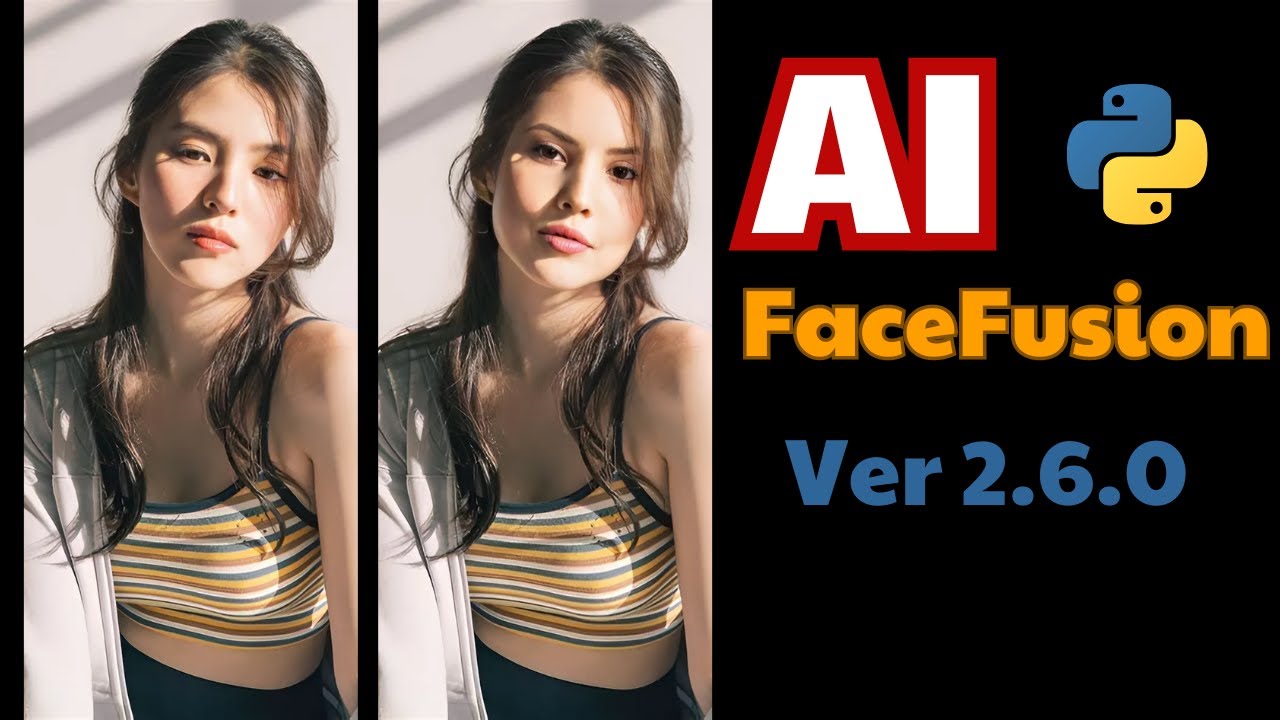
FaceFusion v2 6 0 update - Hoán đổi khuôn mặt cực mạnh & Các bước thiết lập cục bộ chi tiết
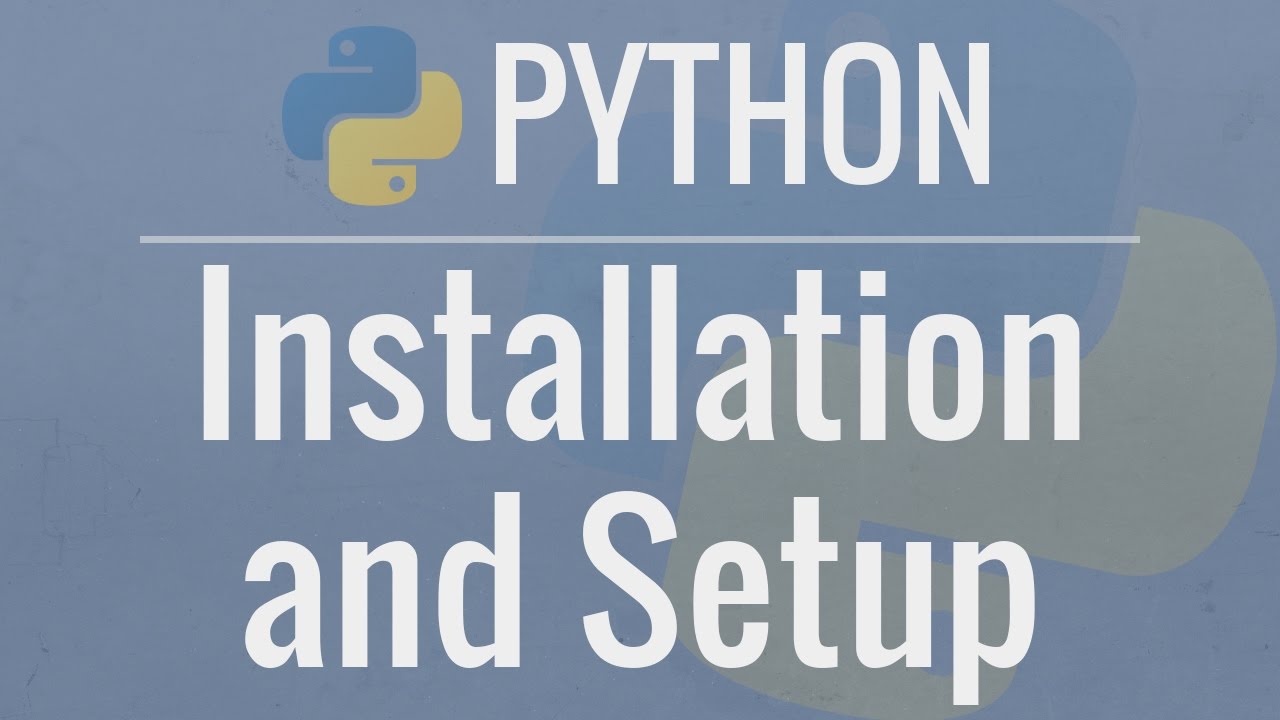
Python Tutorial for Beginners 1: Install and Setup for Mac and Windows
5.0 / 5 (0 votes)