Java Recursion
Summary
TLDRThis tutorial delves into the concept of recursion, explaining it as a method that calls itself, simplifying the problem with each call until it reaches a base case. The video demonstrates calculating triangular numbers and factorials using recursion, illustrating the process with visual examples and code snippets. Additionally, it provides a detailed walkthrough of the merge sort algorithm, showcasing its efficiency and organization through an animated execution, all aimed at helping viewers grasp these fundamental programming concepts.
Takeaways
- đ Recursion is a method where the function calls itself, simplifying the problem with each call until it reaches a base case where it no longer calls itself.
- đ The script explains how to find triangular numbers using recursion, which involves summing numbers from 1 to n, where n is the position in the sequence.
- đ The base case for the triangular number example is when the input number equals 1, at which point the method stops calling itself and returns 1.
- đ Triangular numbers are demonstrated visually as numbers arranged in triangles, with the nth triangular number being the sum of the first n natural numbers.
- đ€ The tutorial also covers a non-recursive method for calculating triangular numbers, which is a simple iterative process summing numbers from 1 to n.
- đ The script provides a step-by-step explanation of how to implement a recursive method to calculate triangular numbers, including printing intermediate results for clarity.
- đČ Factorials are introduced as another example of a calculation that can be performed using recursion, with the base case being factorial of 1 equals 1.
- đ The merge sort algorithm is explained, which uses recursion to sort arrays efficiently by dividing the array into smaller parts, sorting them, and then merging them back together.
- đ The merge sort process is visualized through the script, showing how it compares and swaps elements to sort the array incrementally.
- đ» The tutorial includes code examples and comments to aid understanding of recursive methods, triangular numbers, factorials, and the merge sort algorithm.
- đ The script encourages viewers to look at the provided code, which is heavily commented, to understand the inner workings of the merge sort algorithm.
Q & A
What is the main topic of this tutorial?
-The main topic of this tutorial is recursion, including its definition, how it works, and its applications in calculating triangular numbers, factorials, and in the merge sort algorithm.
What is a recursive method?
-A recursive method is a method that calls itself, simplifying the problem with each call until it reaches a base case where it no longer calls itself.
What are triangular numbers and how are they related to recursion?
-Triangular numbers are numbers that can be represented in a triangle pattern. They are related to recursion because the script demonstrates how to calculate them using a recursive method.
How is the nth triangular number calculated using recursion?
-The nth triangular number is calculated using a recursive method that adds the sequence of numbers from 1 to n, with each recursive call reducing the number by one until it reaches the base case of 1.
What is the base case in a recursive method?
-The base case in a recursive method is the condition that stops the recursion, typically when the problem is simplified enough that it no longer requires further recursive calls.
Can you explain the non-recursive approach to calculating triangular numbers?
-The non-recursive approach to calculating triangular numbers involves summing the sequence of numbers from 1 up to the desired number, without using method calls to itself.
What is a factorial and how is it calculated using recursion?
-A factorial is the product of an integer and all the integers below it. It is calculated using recursion by multiplying the number by the factorial of the number minus one, until the base case of 1 is reached.
What is the merge sort algorithm and how does it use recursion?
-The merge sort algorithm is a sorting technique that divides the array into smaller parts, sorts them, and then merges them back together in the correct order. It uses recursion to repeatedly divide the array until each part is a single element or empty, and then merge them back in sorted order.
How does the merge sort algorithm sort an array?
-The merge sort algorithm sorts an array by first dividing it into halves, sorting each half, and then merging the sorted halves back together. This process is done recursively until the entire array is sorted.
What is the significance of the merge sort algorithm in computer science?
-The merge sort algorithm is significant in computer science because it is an efficient, stable, and comparison-based sorting algorithm with a time complexity of O(n log n), making it suitable for large datasets.
Outlines
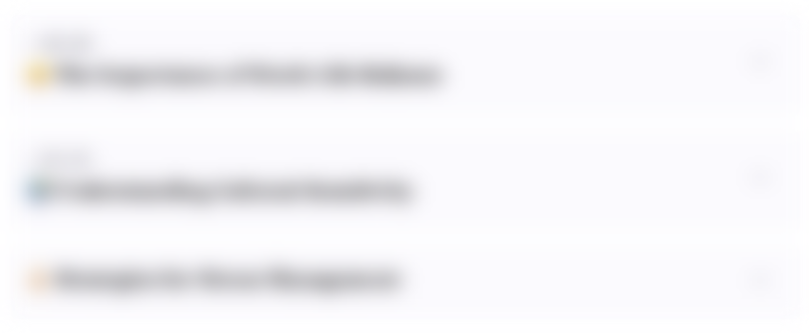
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenMindmap
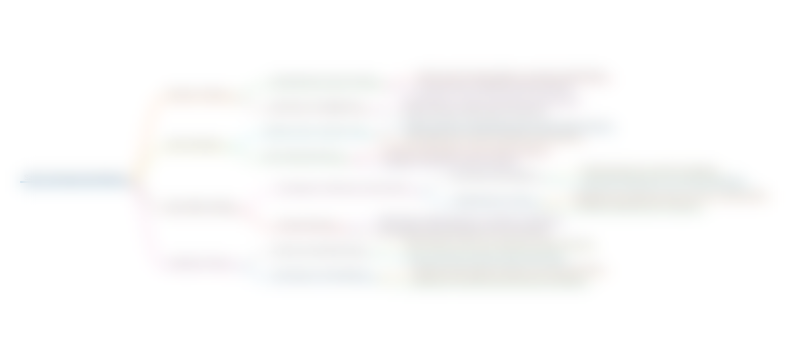
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenKeywords
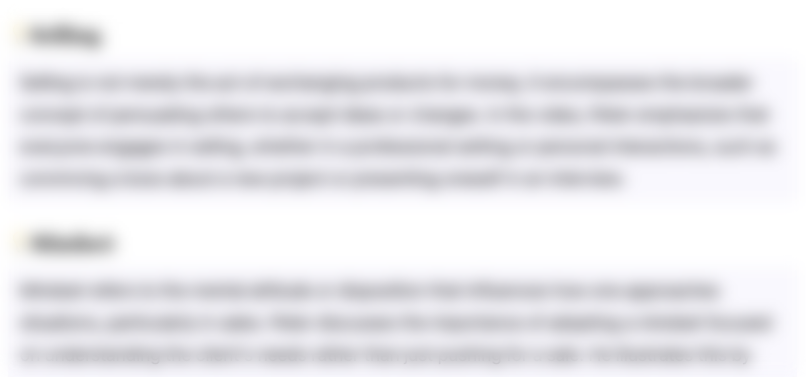
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenHighlights
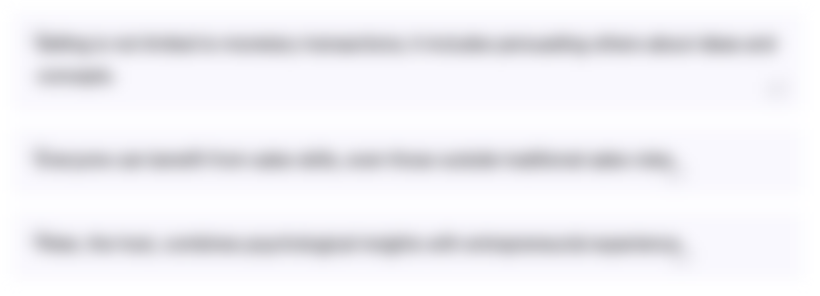
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenTranscripts
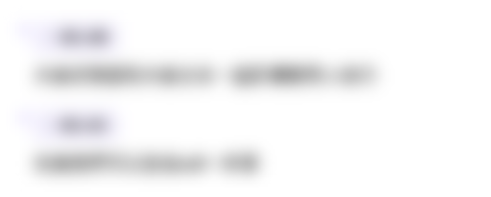
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenWeitere Ă€hnliche Videos ansehen
5.0 / 5 (0 votes)