Rekursi
Summary
TLDRThis lecture on algorithms and data structures focuses on the concept of recursion, explaining its significance in solving complex problems. Through the example of calculating factorials, the video highlights how recursion works by having a function call itself repeatedly until it reaches a base case. The differences between recursion and iteration are discussed, with recursion being noted for its elegance despite higher memory usage. The lecture also explores other applications of recursion, including prime number checks and the Tower of Hanoi problem, showcasing its broad utility in algorithm design.
Takeaways
- π Recursion is a concept where a function calls itself to solve problems, breaking down complex tasks into simpler steps.
- π Recursive functions are useful for solving problems that can be broken down into smaller, similar subproblems.
- π Unlike iteration, recursion requires more memory allocation because of the repeated function calls.
- π Factorial calculation is a classic example of recursion, where the function multiplies a number by the factorial of the previous number until it reaches 1.
- π The base case (or terminal condition) in recursion is critical to stopping the repeated function calls, preventing infinite loops.
- π In the recursive factorial example, the calculation process begins with n and multiplies by each smaller number until reaching 1.
- π Factorial (n!) can be defined recursively, where n! = n Γ (n-1)! until n = 1.
- π Recursion requires a return phase, where the results of the recursive calls are combined to return the final solution.
- π Recursive algorithms are typically more concise and easier to understand than their iterative counterparts, but they may use more memory.
- π Not all problems can be solved efficiently with recursion; the problem must be able to be divided into smaller, solvable subproblems.
- π Other problems that can be solved with recursion include checking prime numbers, calculating powers, and finding the greatest common divisor (GCD).
Q & A
What is the main topic discussed in the transcript?
-The main topic discussed is about recursive algorithms, specifically using recursion to solve problems like factorial calculations. It also touches on the difference between recursion and iteration in algorithms, including the concepts of memory allocation and the base case in recursion.
What is recursion in computer science?
-Recursion is a process in which a function calls itself in order to solve a problem. Each recursive call works on a smaller part of the problem, and the recursion terminates when it reaches a base case, which doesn't make a further recursive call.
How does recursion differ from iteration?
-Recursion differs from iteration in that recursion involves a function calling itself, whereas iteration uses loops to repeat a set of instructions. Recursion often uses more memory due to repeated function calls, while iteration may be more memory-efficient but can be harder to read or maintain in certain cases.
What is a base case in recursion?
-The base case in recursion is a condition that stops the recursion from continuing indefinitely. It provides a termination point where the function no longer calls itself, and the recursive calls begin to resolve.
What is the factorial problem mentioned in the transcript?
-The factorial problem involves calculating the product of all positive integers up to a given number 'n'. For example, 4! (4 factorial) is 4 Γ 3 Γ 2 Γ 1 = 24. This is used as an example to explain recursion in the transcript.
How is recursion used to calculate factorials?
-In recursion, the factorial of a number 'n' can be defined as n Γ factorial(n-1). The recursion continues until it reaches the base case, where factorial(1) = 1, stopping further recursive calls and beginning the process of returning results.
What are the steps involved in calculating the factorial of 3 using recursion?
-To calculate the factorial of 3 using recursion, the function calls factorial(3), which calls factorial(2), which calls factorial(1). When factorial(1) reaches the base case, it returns 1, and the recursion begins to resolve: factorial(2) = 2 Γ 1 = 2, then factorial(3) = 3 Γ 2 = 6.
What is meant by 'call stack' in the context of recursion?
-The 'call stack' refers to the memory structure that stores the function calls and their corresponding local variables. Each time a recursive function is called, a new entry is pushed onto the stack. When the base case is reached and recursion begins to resolve, the entries are popped off the stack.
Why does recursion often require more memory compared to iteration?
-Recursion requires more memory because each recursive call adds a new function frame to the call stack. This stack grows with each recursive call, and each frame holds the state of that particular function call, whereas iteration only requires memory for the loop control variable.
What is the importance of the terminal condition in recursion?
-The terminal condition, or base case, in recursion is critical to prevent infinite recursive calls. Without this condition, the recursion would never stop and could lead to a stack overflow or memory exhaustion, as each call would continue to use up memory without returning a result.
Outlines
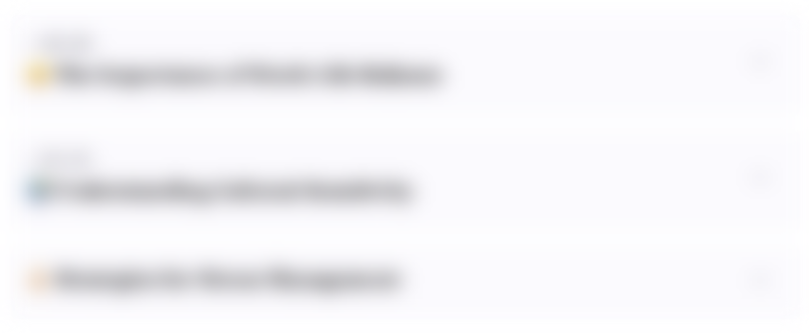
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
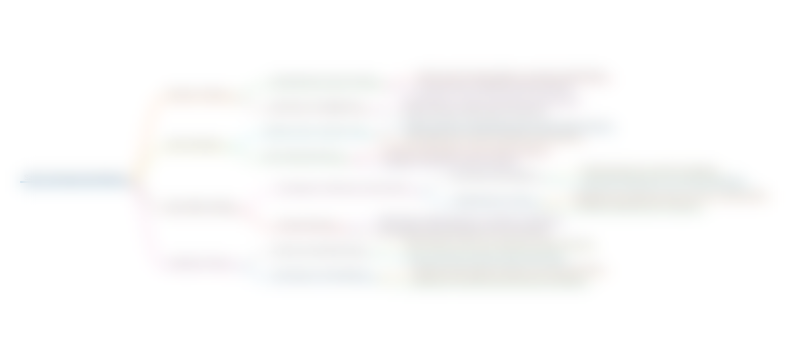
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
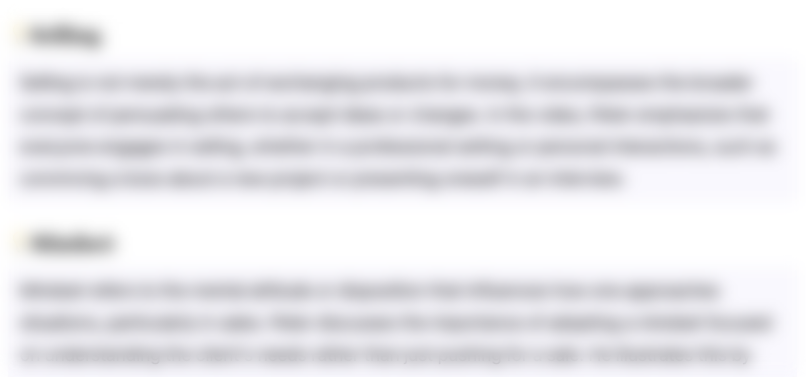
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
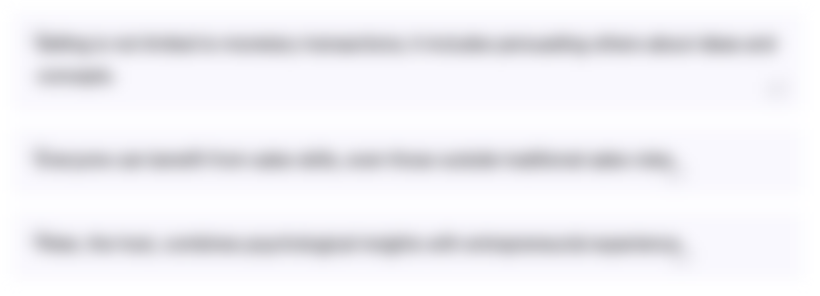
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
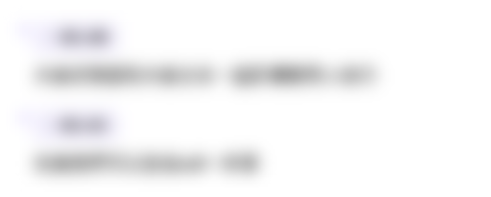
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
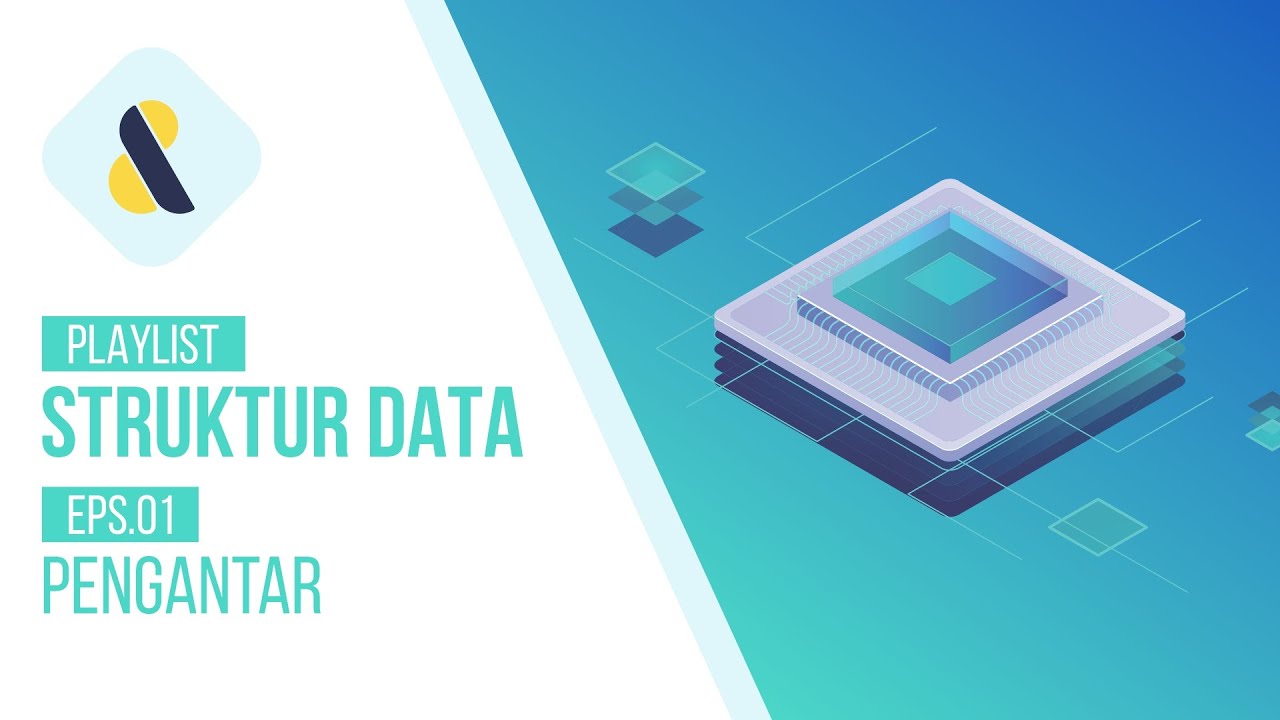
#1 Pengantar Struktur Data | STRUKTUR DATA
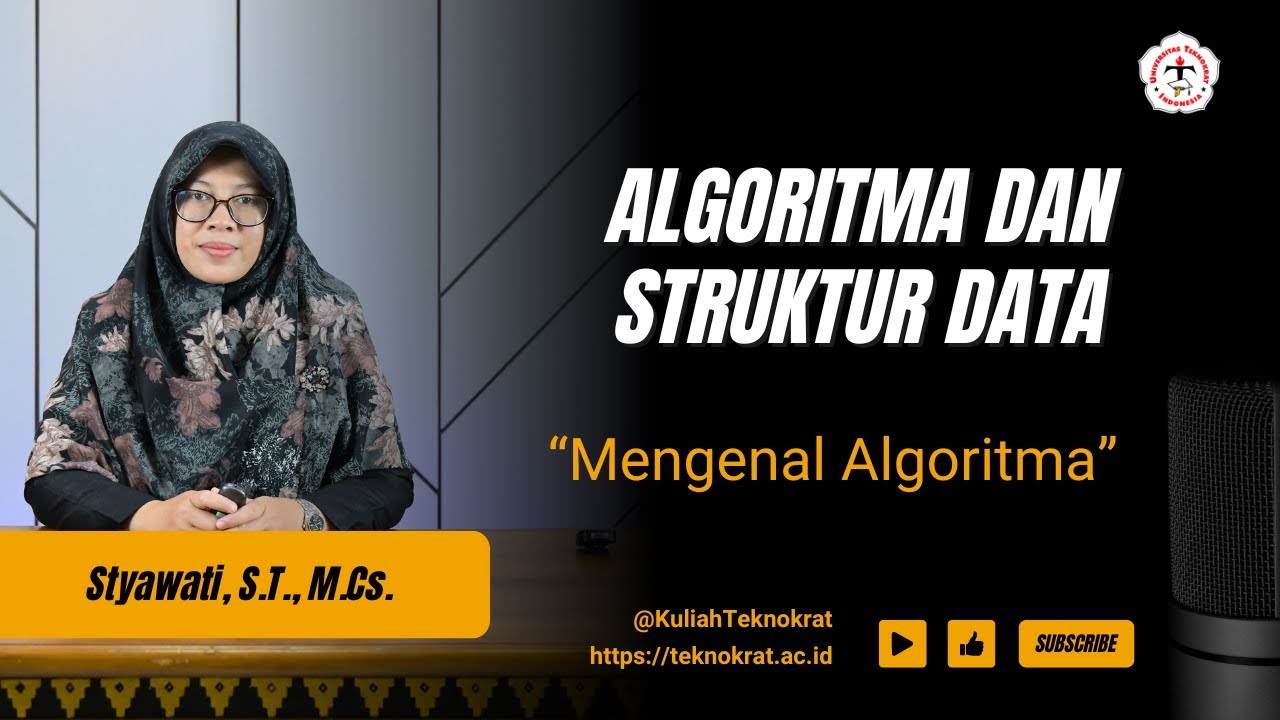
Mengenal Algoritma - Algoritma dan Struktur Data

93. OCR A Level (H446) SLR14 - 1.4 Data structures part 2 - Graphs (operations)

Can you really Complete DSA in 3 months?
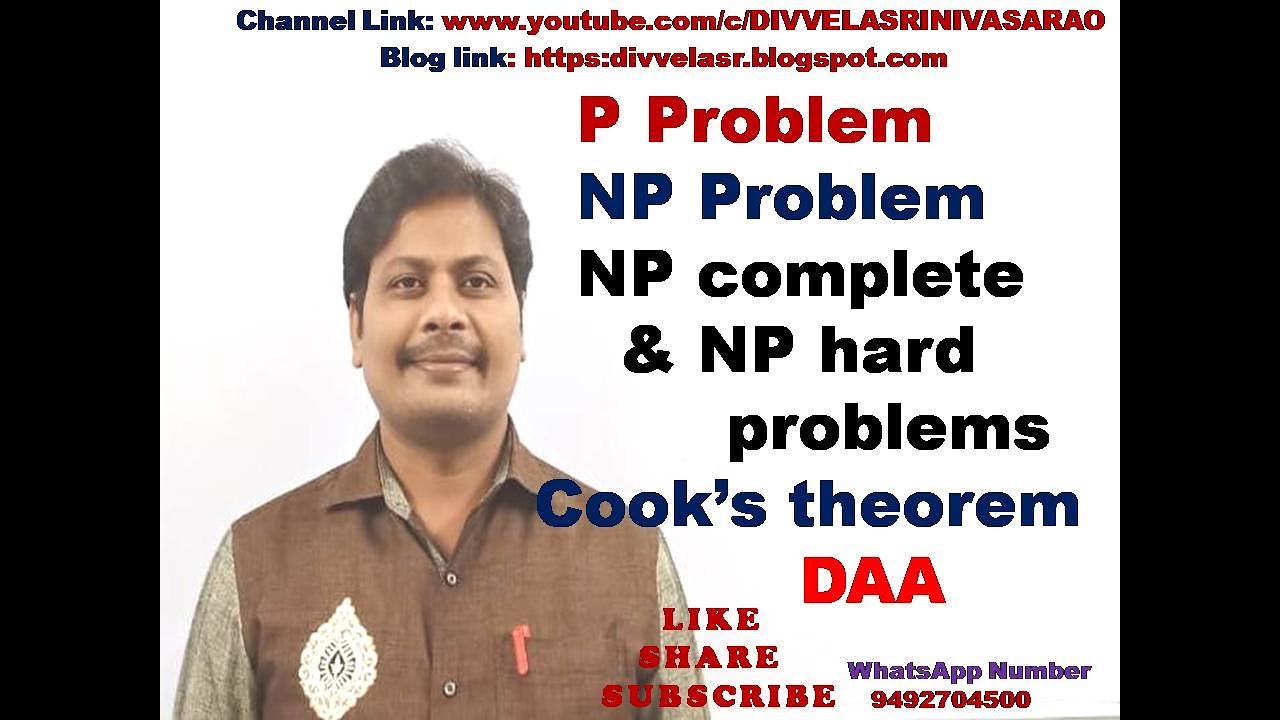
P NP NP-Hard NP-Complete problems || P Versus NP || Relationship between P NP & NP Complete Problems
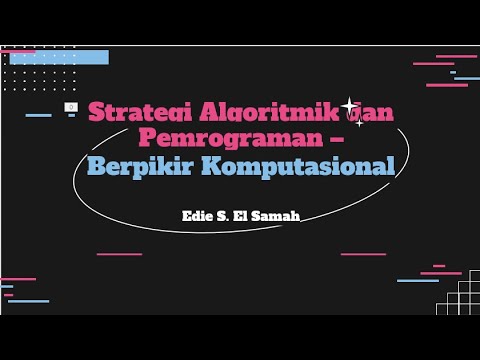
Berpikir Komputasional - Informatika Kelas XI
5.0 / 5 (0 votes)