Kelas Python 3 - Fungsi Rekursi - Mencari Nilai Faktorial #24
Summary
TLDRIn this tutorial, the instructor introduces the concept of recursion in Python by demonstrating how to calculate a factorial using both loops and recursion. The script explains the factorial concept, provides code for both iterative and recursive solutions, and highlights the advantages of recursion. Through the recursive implementation, the function calls itself until it reaches the base case, offering a more elegant and mathematically appealing approach. The instructor also emphasizes the importance of proper recursion implementation to avoid excessive memory use and infinite loops, ensuring the process terminates successfully.
Takeaways
- 😀 A recursive function is a function that calls itself within its body to solve problems iteratively.
- 😀 Factorial is the product of all positive integers up to a given number, e.g., 5! = 5 x 4 x 3 x 2 x 1 = 120.
- 😀 Factorial of zero is defined as 1, which is an important edge case to handle in a recursive function.
- 😀 A factorial function can be implemented using both loops and recursion, with recursion often resulting in more elegant code.
- 😀 If a user inputs a negative number, the program should reject the input, as factorial is only defined for positive integers.
- 😀 The program first asks the user to input a number and checks if the input is valid (positive integer or zero).
- 😀 A basic loop implementation of factorial involves initializing a variable and multiplying it by the sequence of integers.
- 😀 In a recursive factorial implementation, the function calls itself, reducing the number by 1 each time until reaching the base case (n = 0).
- 😀 Recursion works by breaking down a problem into smaller instances of the same problem, ultimately leading to a base case.
- 😀 A common pitfall of recursion is improper termination or reaching the base case, which can lead to infinite recursion and memory issues if not handled carefully.
- 😀 Recursive functions should be implemented with caution, ensuring that memory and performance considerations are taken into account to prevent stack overflow.
Q & A
What is a recursive function in programming?
-A recursive function is a function that calls itself within its own definition to solve a problem. It continues calling itself until a base case is reached, which prevents infinite recursion.
How is the factorial of a number calculated?
-The factorial of a number is the product of all positive integers from 1 to that number. For example, 5! = 5 * 4 * 3 * 2 * 1 = 120. The factorial of 0 is defined as 1.
What is the base case for calculating the factorial of a number?
-The base case for calculating the factorial of a number is when the input is 0. The factorial of 0 is defined as 1.
What are the two methods to calculate the factorial in the script?
-The script demonstrates two methods to calculate the factorial: using a loop (iterative method) and using recursion (recursive method).
How does the recursive factorial function work?
-The recursive factorial function calls itself with the input value reduced by 1, multiplying the result with the current value. This continues until the base case (n = 0) is reached, at which point the function returns 1.
What happens when a user inputs a negative number for the factorial?
-If the user inputs a negative number, the script will reject the input and prompt the user to enter a positive number, since factorials are only defined for non-negative integers.
Why does the recursive factorial function call itself repeatedly?
-The recursive factorial function calls itself repeatedly to break down the problem into smaller subproblems, each calculating the factorial for progressively smaller values, until it reaches the base case where the factorial of 0 is returned.
What is the significance of adding '1' to the range in the for loop for the iterative factorial calculation?
-Adding '1' to the range ensures that the loop includes the final value of the input number. Without adding 1, the loop would exclude the last integer, resulting in an incorrect factorial calculation.
What are the potential downsides of using recursion for calculating factorials?
-The main downsides of using recursion include higher memory consumption due to the function calls being stored on the call stack, and the risk of a stack overflow if the recursion depth becomes too large. It also might not be as efficient as an iterative solution for very large numbers.
How can recursion be used more efficiently in Python?
-Recursion in Python can be used more efficiently by ensuring that the base case is properly defined, limiting recursion depth, and using techniques like memoization or tail recursion (if supported) to avoid redundant calculations.
Outlines
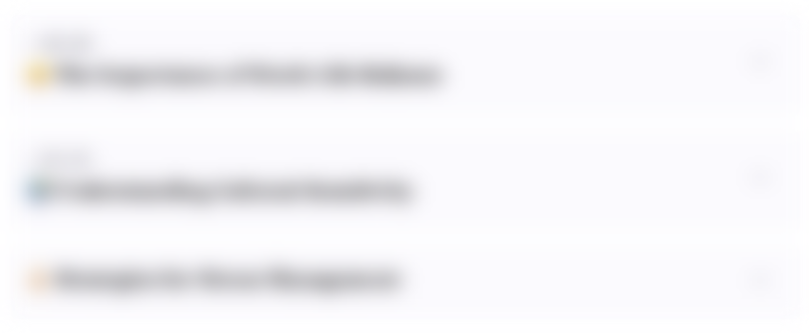
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
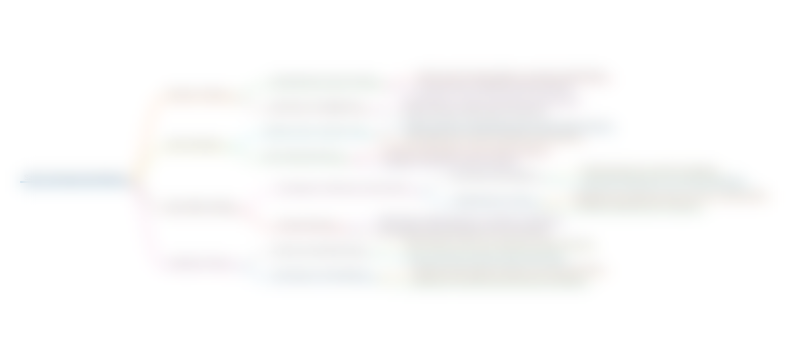
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
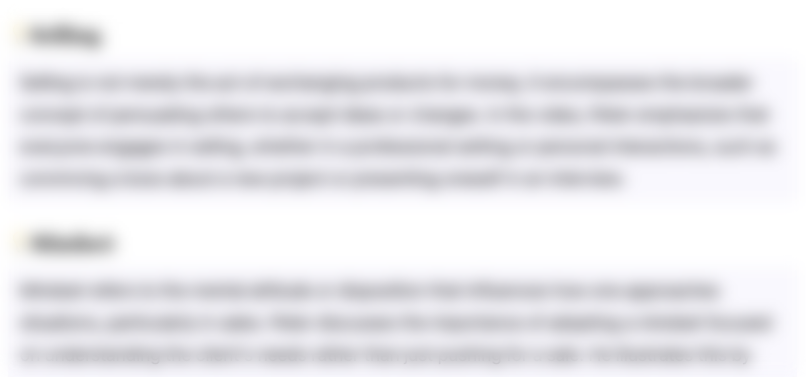
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
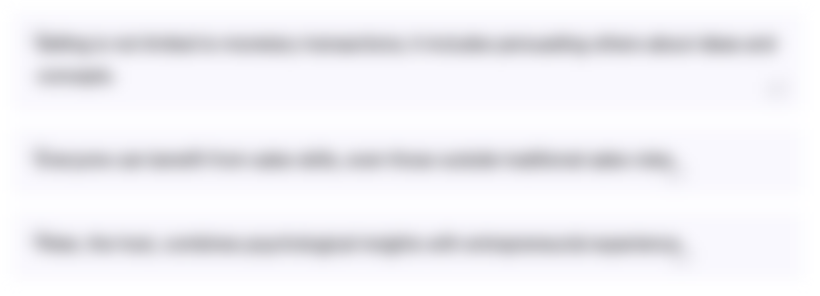
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
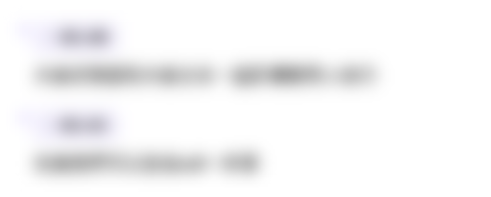
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

C_104 Recursion in C | Introduction to Recursion
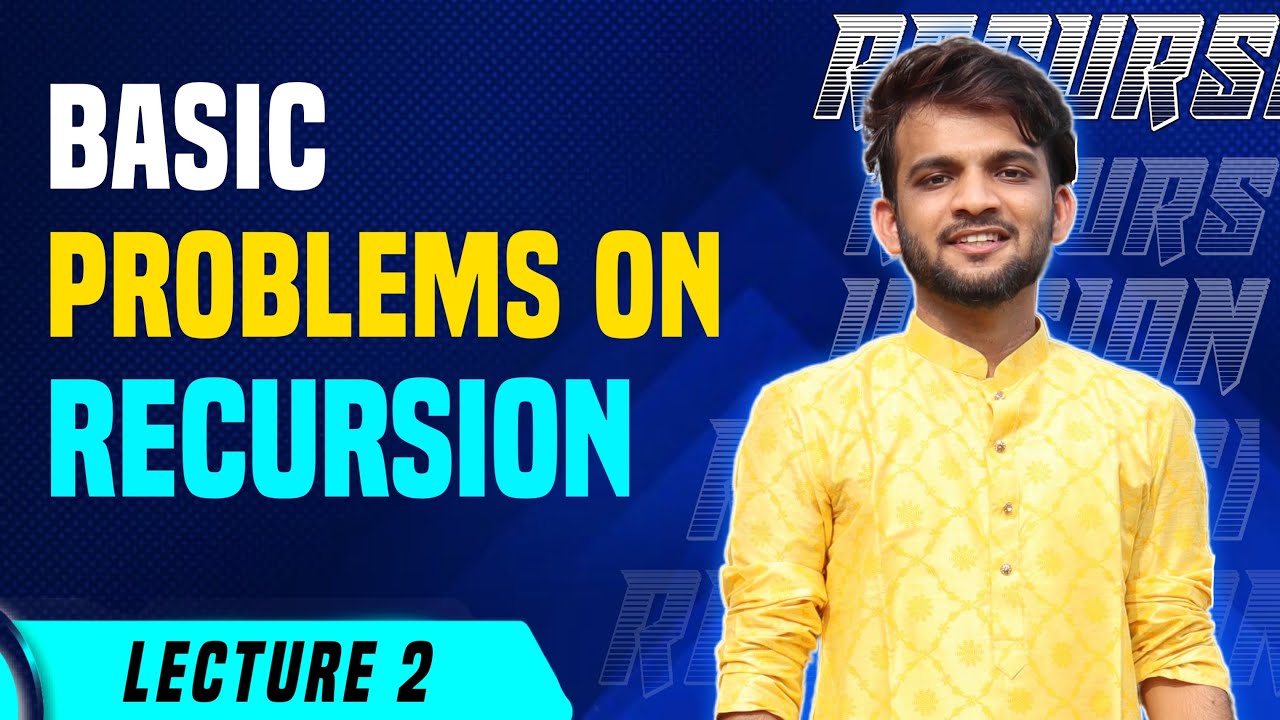
Re 2. Problems on Recursion | Strivers A2Z DSA Course
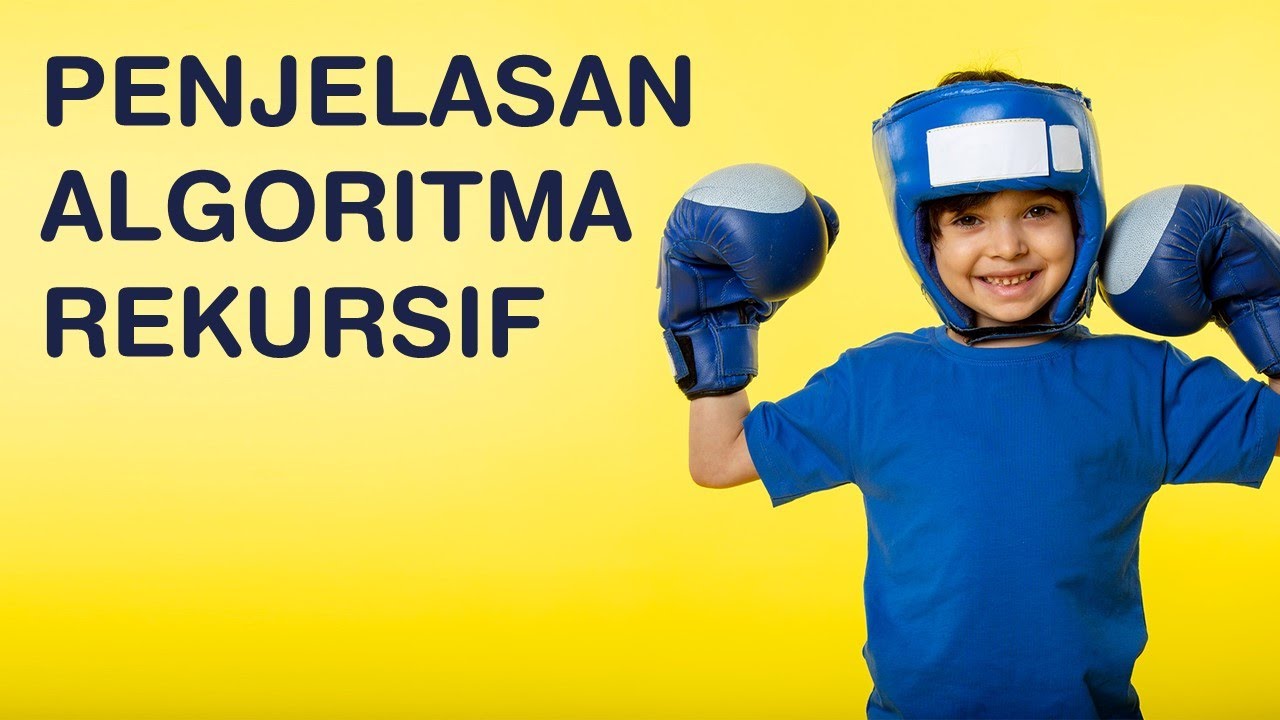
Penjelasan Algoritma Rekursif | Algoritma Faktorial dan Pangkat | Algoritma Pertemuan 57
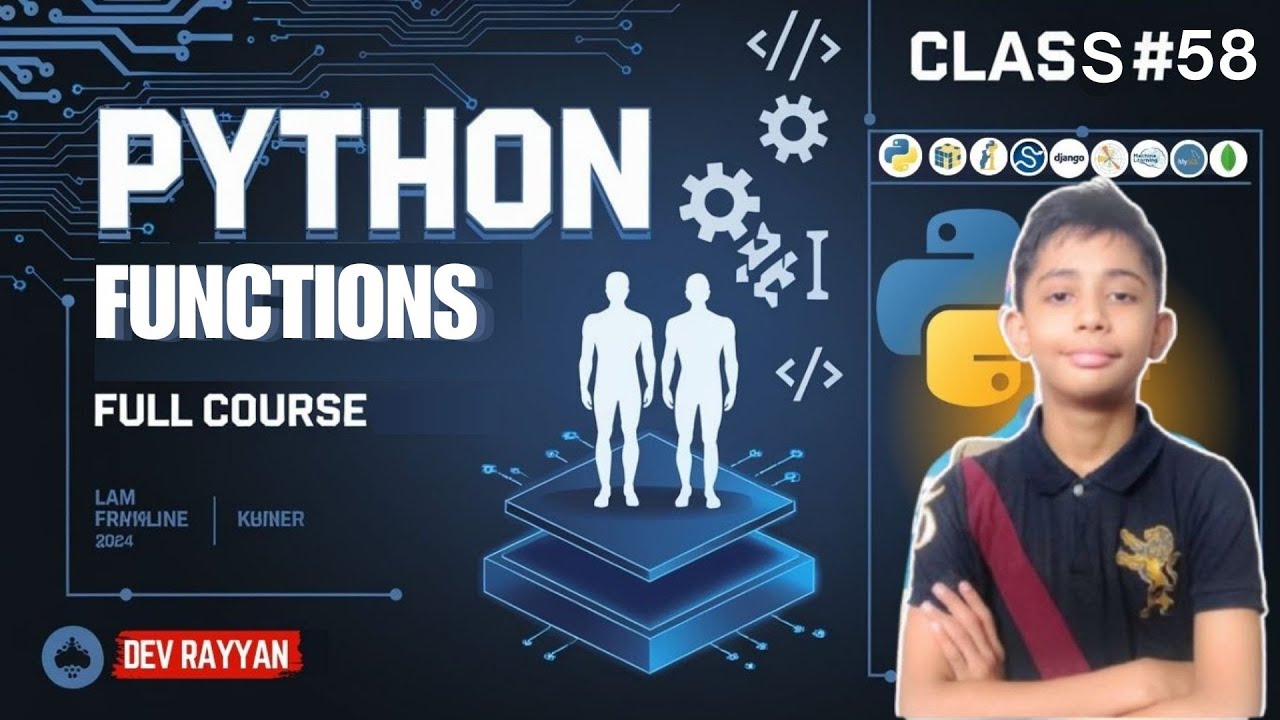
PYTHON FUNCTIONS | Python Tutorial - Lesson #58

Recursion in C
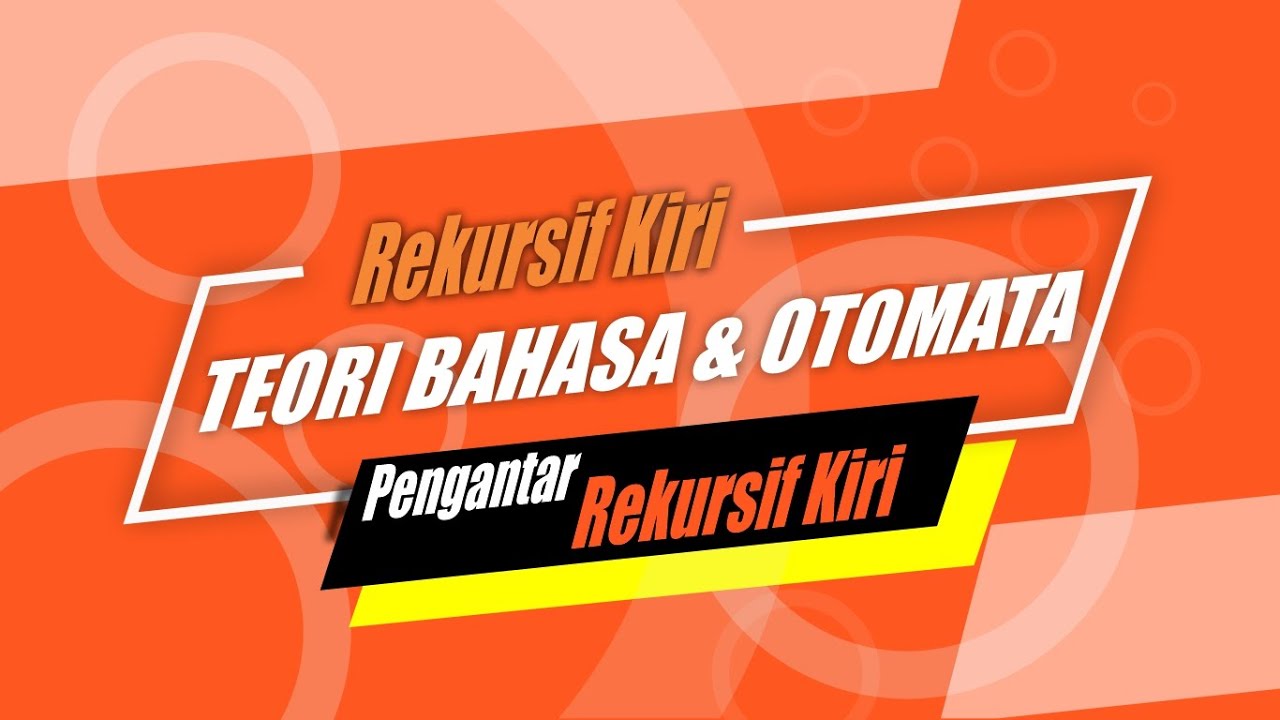
#12 Teori Bahasa & Otomata - Pengantar Aturan Produksi Rekursif Kiri
5.0 / 5 (0 votes)