Tuples
Summary
TLDRThis lecture introduces Python tuples, emphasizing their immutability and ordered nature. It covers tuple creation, accessing elements through positive and negative indexing, and slicing. The script also touches on built-in functions like length, minimum, and maximum, and demonstrates tuple concatenation. However, it clarifies that once created, tuples cannot be modified, unlike lists, highlighting the key differences between the two data structures.
Takeaways
- 📚 A tuple is a data structure in Python that consists of an ordered collection of objects or elements.
- 🔒 Tuples are immutable, meaning once created, their contents cannot be modified.
- 📝 To create a tuple, use parentheses and separate elements with commas.
- 🔍 Indexing in tuples can be done using both positive (starting from 0) and negative (starting from the end) indices.
- 👀 To access elements in a tuple, use the square bracket notation with the specified index number.
- ❌ Attempting to modify a tuple, such as changing an element or using list methods like append or remove, will result in an error.
- 🔑 Slicing in tuples allows for the extraction of a range of elements using index numbers.
- 📏 The length of a tuple can be found using the `len()` function, which returns the number of elements.
- 📊 For tuples containing numerical data, the `min()` and `max()` functions can be used to find the smallest and largest values, respectively.
- 🔗 Tuples can be concatenated, or combined, using the `+` operator to create a new tuple.
- 🚫 Unlike lists, tuples cannot be modified after creation, emphasizing their use for storing data that should not change.
Q & A
What is a tuple in Python?
-A tuple in Python is an ordered collection of objects or elements, which is immutable, meaning once created, it cannot be modified.
How are tuples created in Python?
-Tuples are created by enclosing the elements separated by commas within parentheses.
What is the difference between positive and negative indexing in tuples?
-Positive indexing starts from 0 at the leftmost element, while negative indexing starts from -1 at the rightmost element, moving leftwards.
How can you access a specific element in a tuple?
-You can access a specific element in a tuple by using the index number with square brackets, e.g., `tuple_name[index_number]`.
What is slicing in the context of tuples?
-Slicing is used to access a subset of elements in a tuple by specifying a range of index numbers.
What happens if you try to modify an element in a tuple?
-Attempting to modify an element in a tuple will result in a TypeError, as tuples are immutable and do not support item assignment.
How can you determine the length of a tuple?
-The length of a tuple can be determined using the `len()` function, which returns the number of elements in the tuple.
What built-in functions can be used to find the minimum and maximum values in a tuple containing numeric data?
-The `min()` and `max()` functions can be used to find the minimum and maximum values in a tuple, respectively.
How can you combine two or more tuples in Python?
-Two or more tuples can be combined by using the plus (+) operator, which concatenates the tuples together.
What is the main advantage of using tuples over lists in Python?
-The main advantage of using tuples over lists is that tuples are immutable, which ensures that the data they contain cannot be changed, providing a level of safety for the data.
Can you provide an example of how to create a tuple with employee details?
-An example of creating a tuple with employee details would be `employee_details = ('P001', 'John', 35, 40000)`, where the tuple contains the employee's ID, name, age, and salary.
Outlines
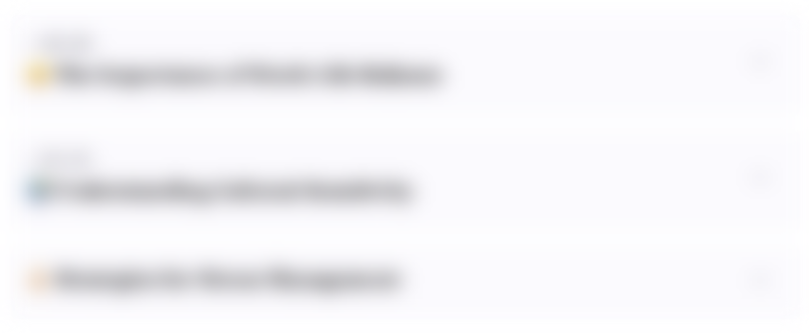
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
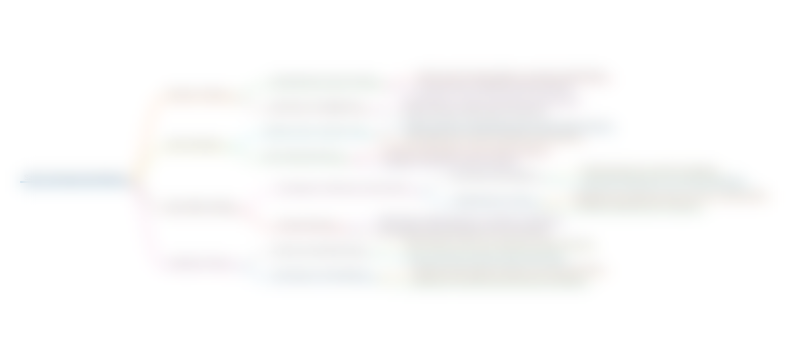
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
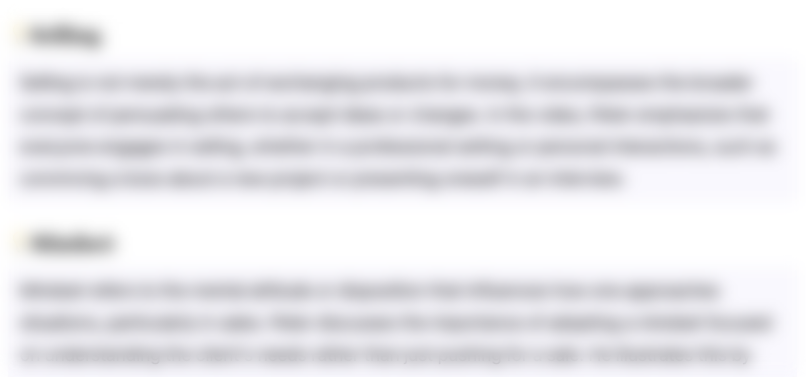
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
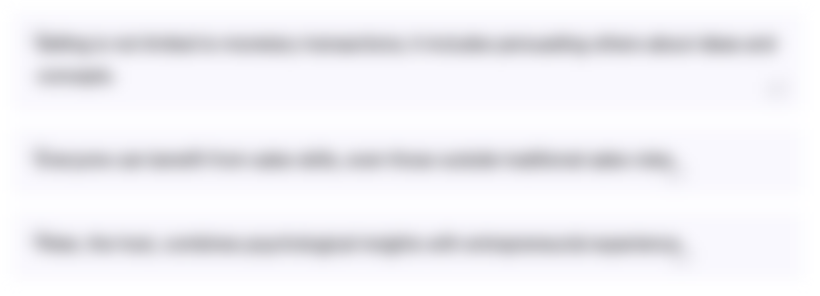
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
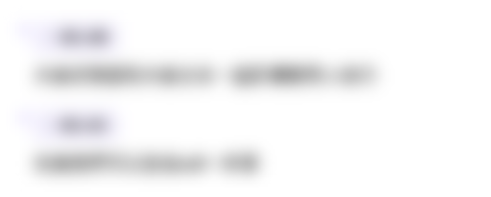
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
5.0 / 5 (0 votes)