#10 Stack Overflow and Other Pitfalls of Functions
Summary
TLDRThis video provides an insightful lesson on the pitfalls of using local variables in C programming, especially in embedded systems. It explains the issues that arise when returning pointers to local variables, which are destroyed upon function return. Through an example with the `delay` function, the instructor demonstrates how this leads to bugs, like incorrect argument values. The solution lies in using the `static` keyword to allocate variables outside of the stack, preserving their value even after the function returns. The lesson concludes with a preview of upcoming topics on data structures and hardware access using CMSIS.
Takeaways
- 😀 Always ensure that the pointers to local variables are not returned from a function to avoid accessing memory that is no longer valid after the function call.
- 😀 Local variables in functions are stored on the stack and go out of scope once the function returns, causing potential issues when accessing them outside of their scope.
- 😀 Returning a pointer to a local variable leads to undefined behavior, as the memory location can be overwritten by subsequent function calls.
- 😀 A simple way to avoid the above issue is by using the `static` keyword for local variables, which allocates the memory outside of the stack, making them persistent across function calls.
- 😀 Using `static` ensures that local variables retain their values even after a function returns, preventing memory corruption.
- 😀 The use of `static` for local variables is a common technique in embedded systems programming, where access to persistent data is crucial.
- 😀 The example demonstrates the effect of returning invalid pointers and how static variables can solve such issues in embedded systems.
- 😀 Debugging the issue with breakpoints and observing the stack shows how returning pointers to local variables can lead to incorrect program behavior.
- 😀 Proper use of memory allocation techniques, like `static`, ensures that variables can be safely accessed after a function exits without causing errors.
- 😀 The program runs correctly after the change to static variables, with the second function call receiving the expected argument and the LED blinking as intended.
Q & A
Why is returning a pointer to a local variable considered a bad practice in C?
-Returning a pointer to a local variable is dangerous because local variables are allocated on the stack, which is cleared when the function exits. Once the function returns, the memory for the local variable is no longer valid, leading to unpredictable behavior or crashes when trying to access it.
What happens to a local variable after a function returns in C?
-After a function returns, local variables that were allocated on the stack go out of scope and are deallocated. This means that their values are no longer accessible and the memory they occupied is reclaimed, which can cause issues if pointers to these variables are used after the function exits.
What is the purpose of the `static` keyword in C?
-The `static` keyword in C changes the storage duration of a variable. When applied to local variables, it ensures that the variable is stored outside the stack (usually in the data segment) and persists for the lifetime of the program. This prevents the variable from going out of scope when the function returns.
How does using the `static` keyword fix the issue of returning pointers to local variables?
-Using `static` ensures that the local variable is not allocated on the stack, but rather in a persistent memory location that outlives the function call. This makes the pointer returned from the function still valid, even after the function exits.
What specific problem does the code in the transcript address when using local variables?
-The code addresses the problem of returning a pointer to a local variable, which can lead to invalid memory access after the function returns. This problem is demonstrated by the incorrect argument being passed to a function due to the destruction of the local variable's value on the stack.
Why was the argument to the `delay` function incorrect after the first function call?
-The argument to the `delay` function was incorrect because the local variable used in the first function call was destroyed when the function returned. This caused the value to be overwritten, and the second call to `delay` received a value of zero instead of the expected 500,000.
How does using the `static` keyword prevent the issue with stack memory?
-By using the `static` keyword, the local variable is stored outside the stack, ensuring that it remains intact and accessible even after the function returns. This ensures that the correct value is passed to the second call to `delay`.
What does the code demonstrate about stack memory and function calls?
-The code demonstrates how local variables are managed in stack memory during function calls. It shows that when functions return, their local variables are deallocated, and any pointers to those variables become invalid, potentially leading to bugs or unexpected behavior.
What was the result after fixing the code by using the `static` keyword?
-After using the `static` keyword, the local variable persisted beyond the function call, and the correct argument of 500,000 was passed to the `delay` function during its second call. The program then ran as expected, and the LED blinked correctly.
What is the next lesson about, as mentioned in the transcript?
-The next lesson focuses on data structures in C and teaches how to use the Cortex Microcontroller Software Interface Standard (CMSIS) to access hardware, allowing students to work with hardware-level programming.
Outlines
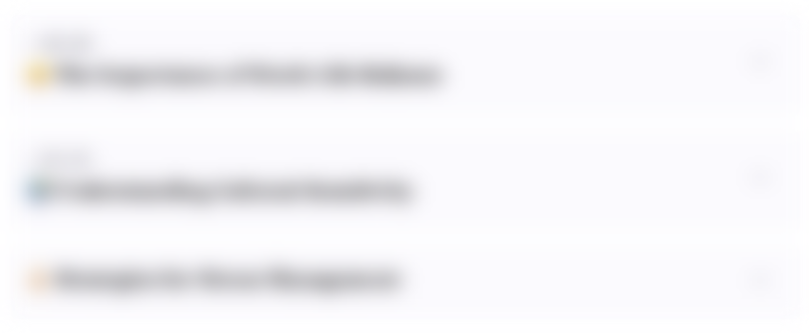
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
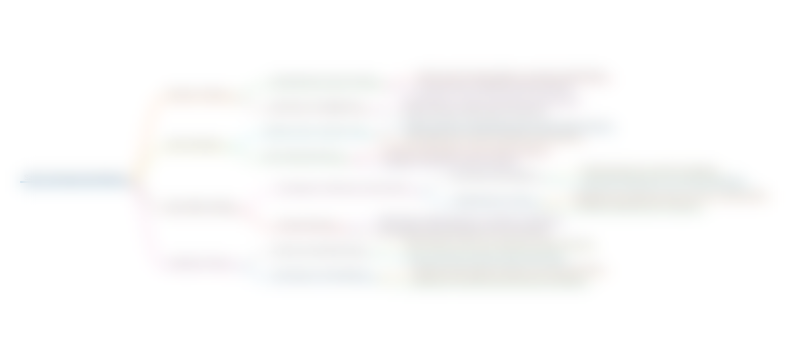
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
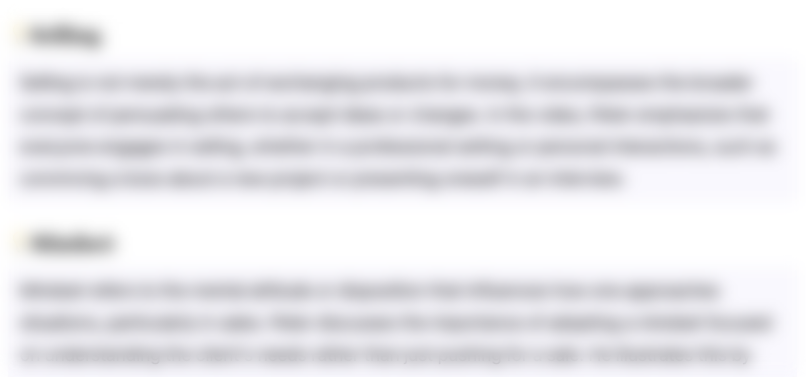
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
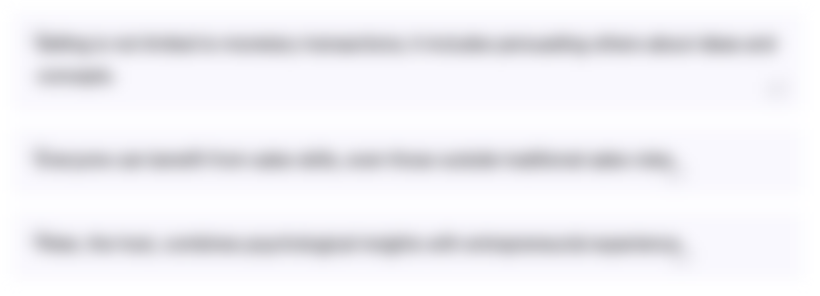
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
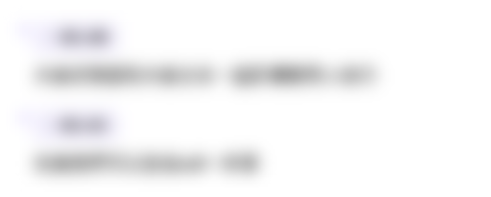
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführen5.0 / 5 (0 votes)