#4: Get User Input in C Programming
Summary
TLDRIn this video tutorial on C programming, viewers learn how to take user input using the `scanf` function. The presenter covers various input types, including integers, doubles, and characters, and demonstrates how to store user inputs in variables. Through practical examples, the tutorial explains format specifiers and the importance of memory addresses. The video also introduces how to handle multiple inputs in a single `scanf` call. By the end, users are encouraged to write a program themselves and engage with a quiz to reinforce the learned concepts.
Takeaways
- 😀 The `scanf` function in C is used to take input from users and store it in variables.
- 😀 Format specifiers like `%d` (integer), `%lf` (double), and `%c` (character) define the type of input expected from the user.
- 😀 To store input in a variable, the `&` symbol is used to pass the memory address of the variable.
- 😀 A simple C program structure includes declaring variables, using `scanf` to take input, and printing the result using `printf`.
- 😀 It's essential to print clear messages for the user, such as ‘Enter input value,’ to guide them when entering data.
- 😀 You can take multiple inputs in one `scanf` call by using multiple format specifiers, e.g., `%lf %c` for a double and a character.
- 😀 Always ensure that the correct format specifier is used to match the type of data being inputted (e.g., `%d` for integers, `%lf` for doubles).
- 😀 The memory address of the variable is referenced using `&` in `scanf`, which is crucial for storing the user’s input in the variable.
- 😀 In case of confusion about input positions, you can add prompts or clear instructions to guide the user in providing input.
- 😀 After learning how to handle integers, doubles, and characters separately, you should also practice combining multiple inputs in a single `scanf` call.
Q & A
What is the purpose of the `scanf` function in C programming?
-The `scanf` function in C programming is used to take input from the user and store it in variables.
What role do format specifiers play in the `scanf` function?
-Format specifiers in `scanf` define the type of data expected from the user input, such as `%d` for integers, `%lf` for doubles, and `%c` for characters.
Why is `&a` used in the `scanf` function?
-`&a` is used in the `scanf` function to provide the memory address of the variable `a`, allowing `scanf` to store the input value at that memory location.
What would happen if `&a` was omitted in the `scanf` function?
-If `&a` were omitted, the `scanf` function would not know where to store the input value, leading to undefined behavior or errors.
How can we improve the user experience when using `scanf` for input?
-You can improve user experience by printing a prompt message before calling `scanf`, asking the user for input, making it clear what they need to enter.
What is the difference between `%d` and `%lf` in the `scanf` function?
-`%d` is used to read integer values, while `%lf` is used to read double (floating-point) values from the user.
Why is there a space before `%c` in the `scanf` function when reading a character?
-The space before `%c` in `scanf` ensures that any leftover newline characters (from previous inputs) are ignored, allowing proper input of a character.
Can we take multiple inputs in one `scanf` call? If yes, how?
-Yes, we can take multiple inputs in one `scanf` call by separating the format specifiers with spaces and passing multiple variables to store the input values.
What would happen if you tried to input a value that doesn't match the expected format specifier in `scanf`?
-If the input doesn’t match the expected format specifier, `scanf` will fail to assign the input value to the variable, and the program may not behave as expected.
How can we handle user input for different data types in a single program?
-You can handle different data types by using appropriate format specifiers for each type (e.g., `%d` for integers, `%lf` for doubles, `%c` for characters), and calling `scanf` multiple times for different variables or combining them into a single `scanf` call.
Outlines
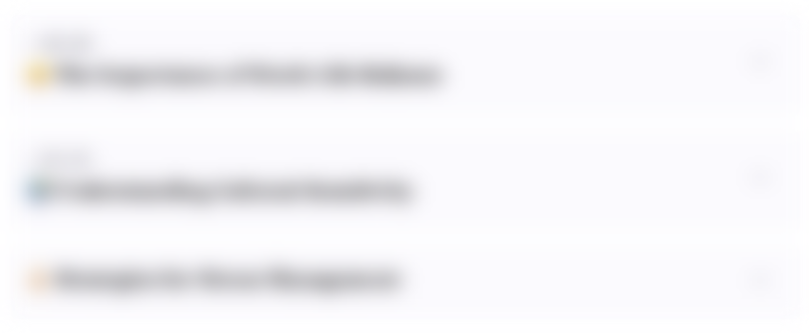
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
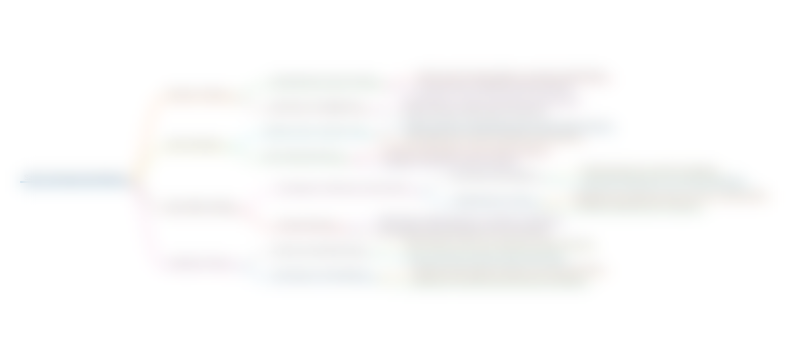
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
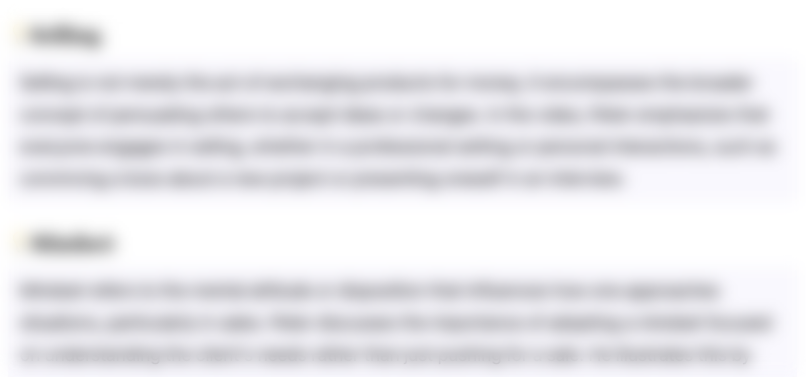
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
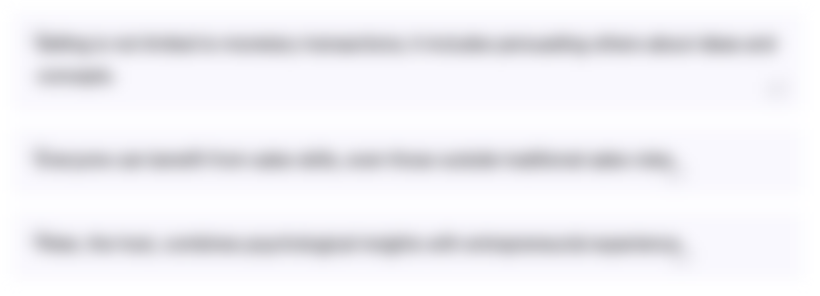
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
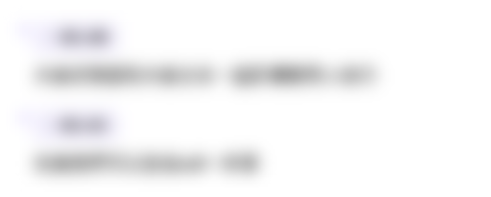
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
5.0 / 5 (0 votes)