1. How to Represent a Singly Linked List in Java | Data Structure using JAVA|APIPOTHI| DATASTRATURES
Summary
TLDRThis tutorial walks you through the process of creating a singly linked list in Java. It starts by explaining the fundamental concepts of nodes, where each node holds data and a reference to the next node. The video demonstrates how to create a basic `ListNode` class with data and next fields, along with a constructor to initialize the node. Although the tutorial focuses on creating a single node (head) for now, it sets the stage for future videos, where linking multiple nodes and performing operations like traversal and insertion will be covered in more detail.
Takeaways
- 😀 A singly linked list is a data structure where each node contains data and a reference (next) to the next node.
- 😀 The first node in a singly linked list is called the head, and the last node has a next reference set to null.
- 😀 Each node in a singly linked list is divided into two parts: data and the reference to the next node.
- 😀 The reference (next) of each node helps link it to the next node, forming a chain of nodes.
- 😀 In Java, a reference is used to connect nodes, and the address of the node changes dynamically across different executions and systems.
- 😀 For a singly linked list, the 'head' node represents the first node, and the 'next' reference connects nodes in the list.
- 😀 A private inner class is used to implement nodes in a linked list to ensure encapsulation and avoid external access or modification of the nodes.
- 😀 In Java, the 'next' reference of a node is initialized to null by default, which is updated when the nodes are linked.
- 😀 The constructor of a node initializes the node with the provided data and sets its next reference to null by default.
- 😀 To create a linked list, you need to instantiate nodes and link them together using the 'next' reference, forming a chain of nodes.
- 😀 The key focus in a singly linked list is on the data and linking nodes, not on the memory addresses, as they are managed by the JVM.
Q & A
What is a singly linked list?
-A singly linked list is a linear data structure where each element (node) contains two parts: the data and a reference (address) to the next node in the sequence. The last node's reference points to null, indicating the end of the list.
What are the key components of a singly linked list node?
-Each node in a singly linked list contains two main components: a 'data' field, which stores the node's value, and a 'next' field, which stores a reference (or address) to the next node in the list.
Why is the first node in a singly linked list called the head?
-The first node in a singly linked list is called the head because it serves as the entry point to the list. All operations typically begin from the head node.
What happens when a node is the last in a singly linked list?
-When a node is the last in the singly linked list, its 'next' field is set to null, indicating that there are no more nodes following it.
What is the purpose of the 'next' field in a node?
-The 'next' field in a node contains a reference (or address) to the next node in the list. It is essential for linking nodes together and maintaining the order of the list.
What happens if the 'next' reference in a node is null?
-If the 'next' reference in a node is null, it indicates that the node is the last node in the list, as there are no further nodes following it.
Why is the 'ListNode' class defined as private in the implementation?
-The 'ListNode' class is defined as private to encapsulate the node's structure and prevent external manipulation of the linked list's internal data. It ensures that only the linked list's methods can modify or access the nodes.
What does the constructor of the 'ListNode' class do?
-The constructor of the 'ListNode' class initializes a node with a given data value. It also sets the 'next' reference to null, which is the default value for a newly created node.
Why is the 'next' reference set to null by default?
-The 'next' reference is set to null by default because when a new node is created, it does not yet point to any other node. Only when nodes are linked together will this reference be updated.
What is the significance of using a static inner class for the 'ListNode'?
-A static inner class for the 'ListNode' allows the node to be part of the containing class (like 'SinglyLinkedList') without needing an instance of the outer class. It also ensures that the node class is not exposed outside of the containing class, enhancing encapsulation.
Outlines
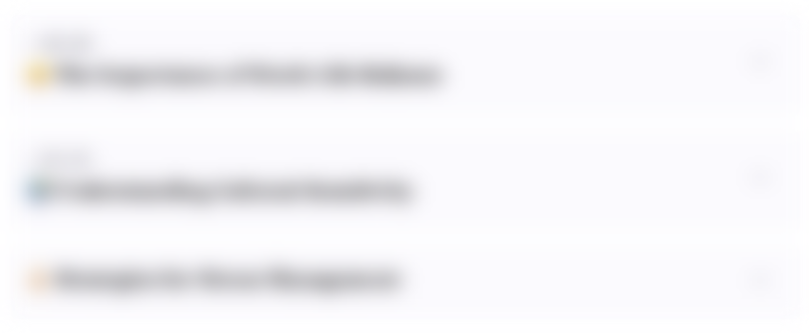
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
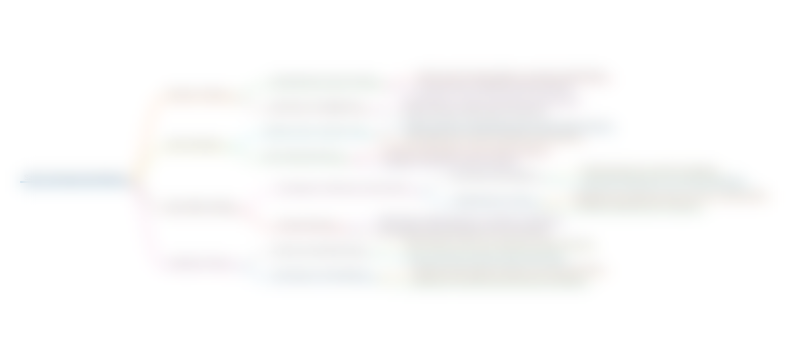
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
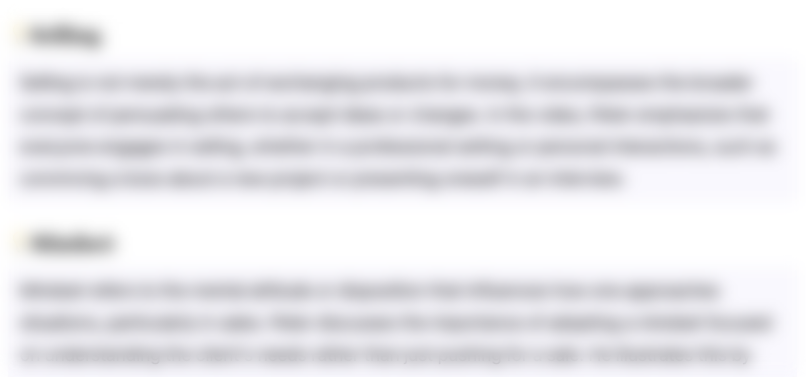
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
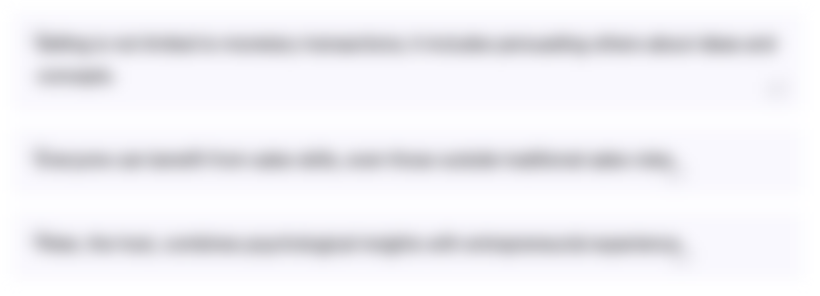
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
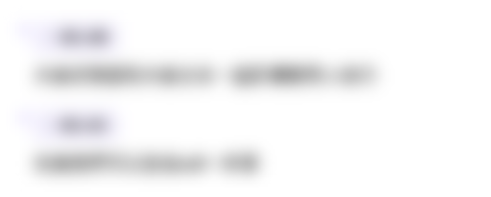
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen

Data Structures in Python: Doubly Linked Lists -- Append and Prepend
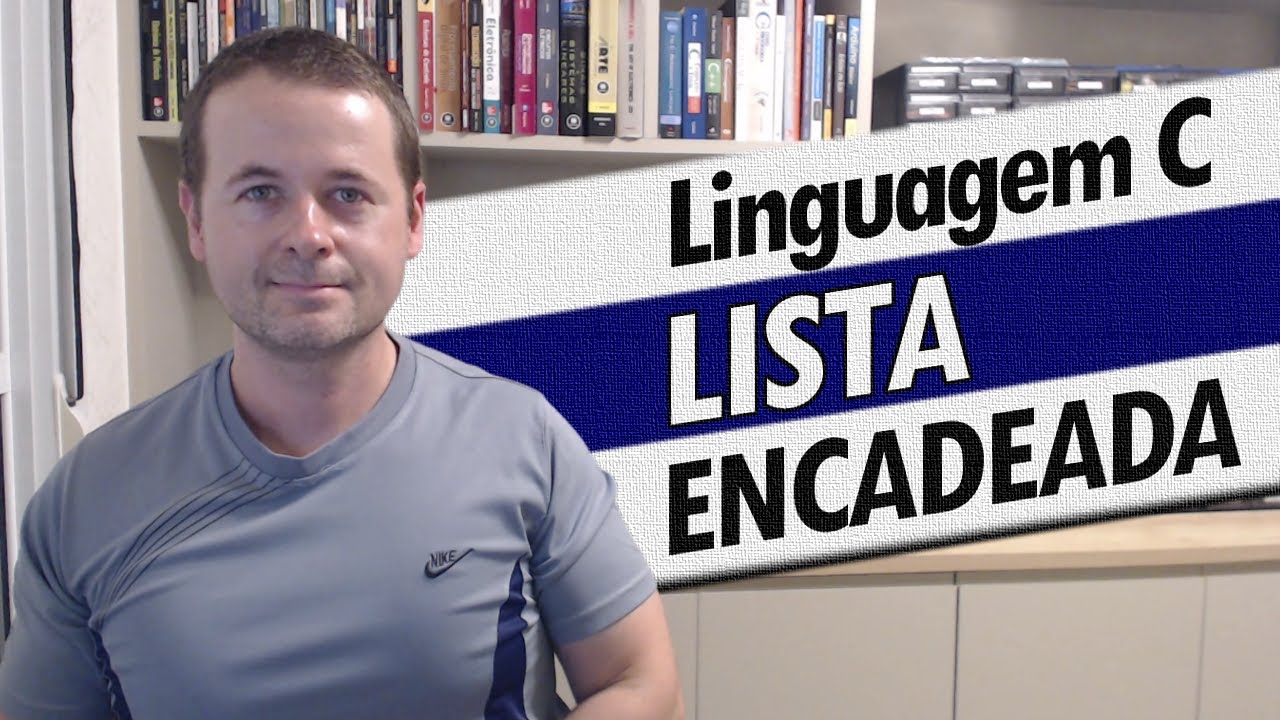
ENTENDA LISTAS ENCADEADAS EM C EM POUCOS MINUTOS!
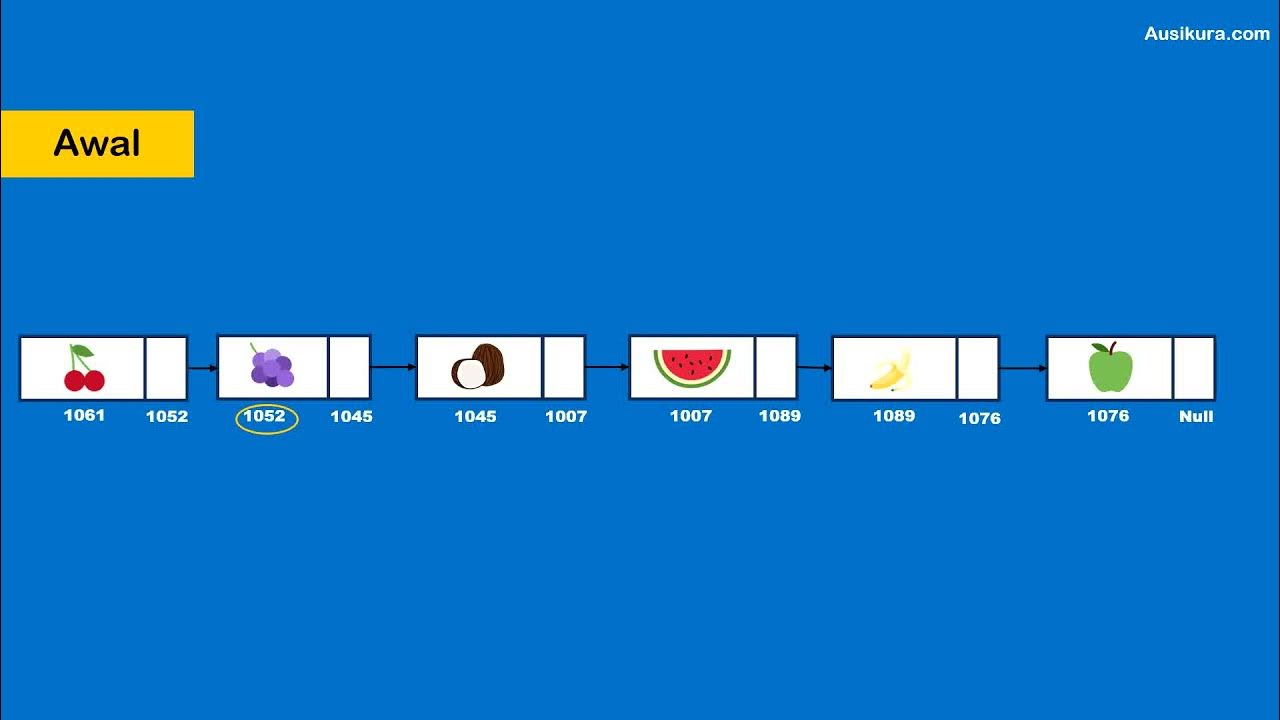
Pengenalan Linked List - struktur Data (Animasi)
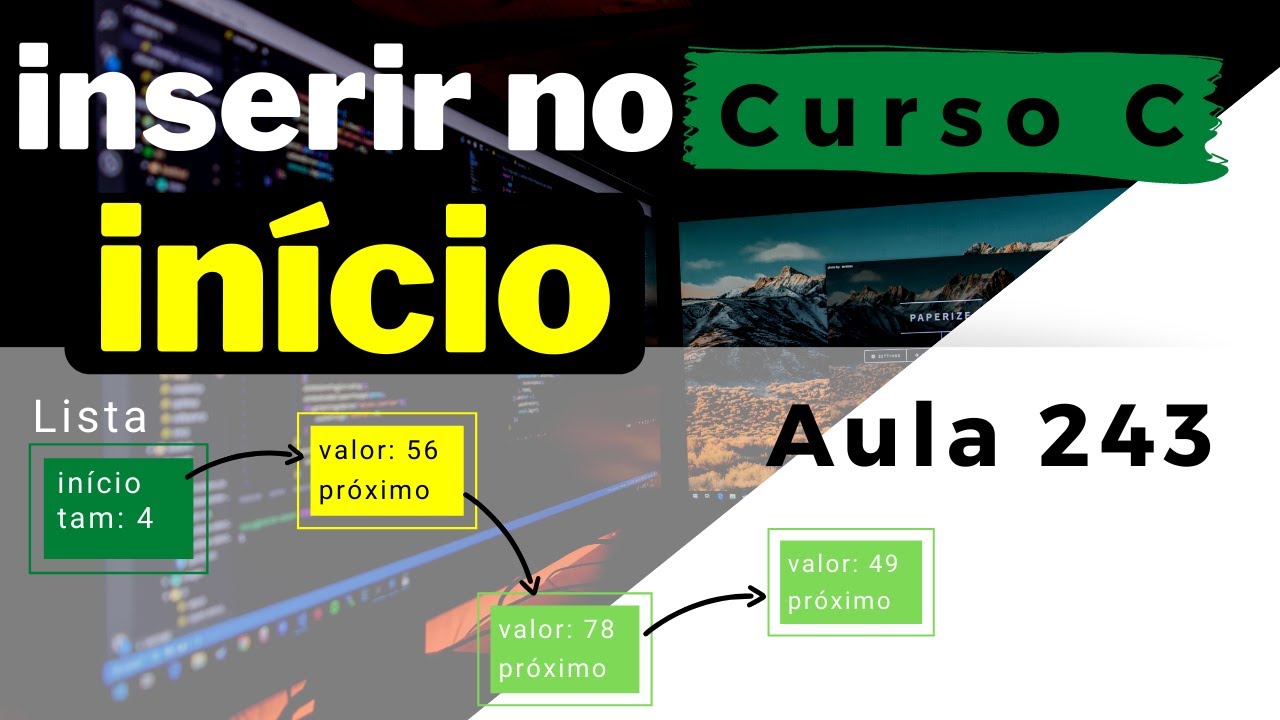
Curso de Programação C | Como inserir no início de uma Lista Simplesmente Encadeada? | aula 243

Como Criar um Aplicativo Passo a Passo?

Struktur Data Pertemuan 4
5.0 / 5 (0 votes)