JV M_22
Summary
TLDRThis tutorial introduces the concept of using Java to manage date and time, demonstrating how to create date objects, format them, and implement a delay in program execution using the Thread.sleep method. It explains the significance of milliseconds in programming and illustrates practical applications by printing the current date before and after a specified sleep period. Additionally, the tutorial covers error handling using try-catch blocks, ensuring robust program execution. Users will gain hands-on experience in managing time-related functions in Java, enhancing their coding skills through practical examples and iterative loops.
Takeaways
- 😀 The program demonstrates how to use the Thread.sleep() method in Java to pause execution for a specified time.
- ⏰ Sleep duration is set in milliseconds, where 1000 milliseconds equals 1 second.
- 📅 The current date and time are printed before and after the sleep period using the Date class.
- 🛑 Exception handling is implemented with a try-catch block to manage potential errors during execution.
- 💻 The class TimeDelay contains the main method where the sleep functionality is demonstrated.
- 🔄 A for loop can be used to print the current date and time repeatedly at specified intervals.
- 📈 The loop initializes a counter, checks a condition, and increments the counter to control the number of iterations.
- 📝 Using system.out.println(), the program outputs the current date and time both before and after the sleep call.
- 🔍 The example shows how to manipulate the sleep duration by changing the value in Thread.sleep().
- 💡 The script illustrates practical applications for pausing execution in Java programs, such as creating delays or scheduling tasks.
Q & A
What is the primary purpose of the Java program discussed in the video?
-The primary purpose of the program is to demonstrate how to print the current date and time, pause the execution of the program for a specified duration, and then print the updated date and time.
How does the program achieve the sleep functionality?
-The program uses the `Thread.sleep(milliseconds)` method to pause the current thread for a specified number of milliseconds.
What is the significance of using the `try-catch` block in the program?
-The `try-catch` block is used for error handling, allowing the program to catch and manage exceptions that may occur during execution, such as an `InterruptedException` when the thread sleep is interrupted.
What unit of time is used in the `Thread.sleep()` method?
-The `Thread.sleep()` method takes an argument in milliseconds, meaning that 1000 milliseconds corresponds to 1 second.
Can you explain how the current date and time are printed in the program?
-The current date and time are printed by creating a new instance of the `Date` class, which captures the current moment, and then outputting it using `System.out.println()`.
What happens if an exception occurs during the execution of the program?
-If an exception occurs, it is caught by the catch block, which then prints an error message describing the exception using `System.out.println()`.
How can the program be modified to print the date and time repeatedly?
-The program can be modified by using a `for` loop to iterate through a specified number of times, printing the date and time with a pause after each iteration, effectively printing it at regular intervals.
What is the expected output format of the date and time when printed?
-The expected output format of the date and time will be the default format provided by the `Date` class, which typically includes the day, month, year, hour, minute, second, and timezone.
How does changing the argument in `Thread.sleep()` affect the program's execution?
-Changing the argument in `Thread.sleep()` affects how long the program pauses before executing the next line; for example, setting it to 2000 will pause for 2 seconds instead of 1.
What are some potential applications of using the `Thread.sleep()` method in real-world programming?
-Potential applications include creating delays in animations, polling data at regular intervals, implementing timeouts for operations, or controlling the pacing of game events.
Outlines
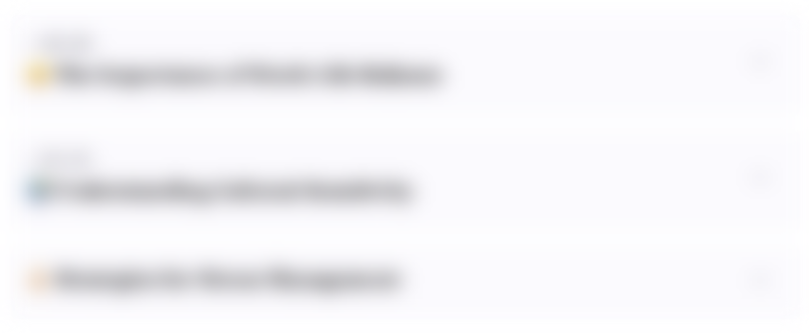
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
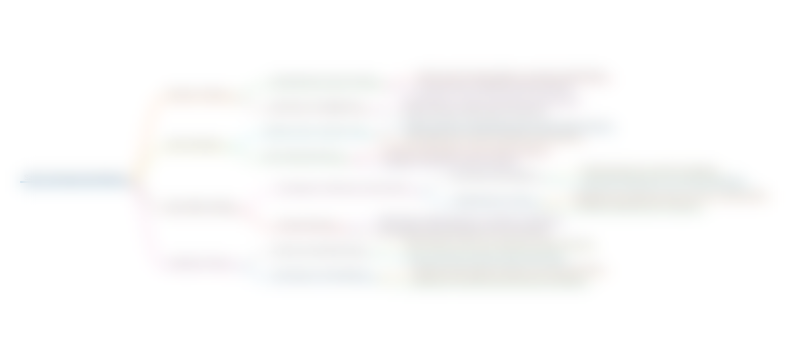
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
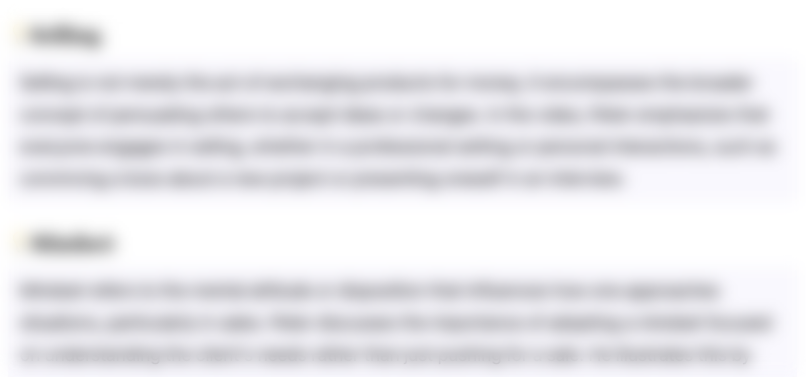
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
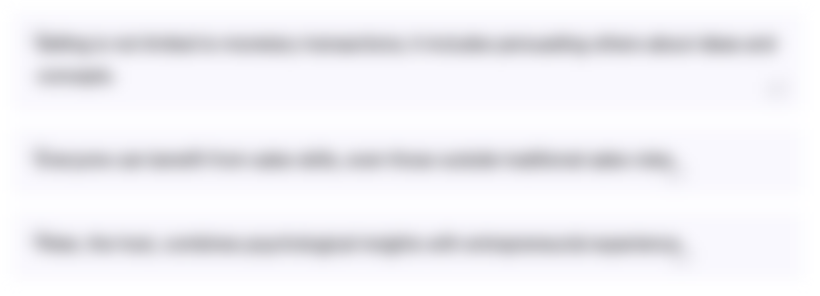
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
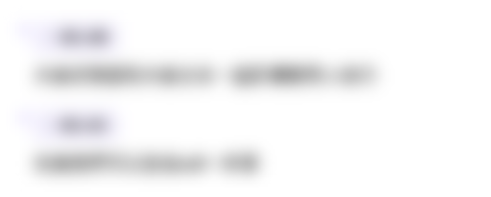
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
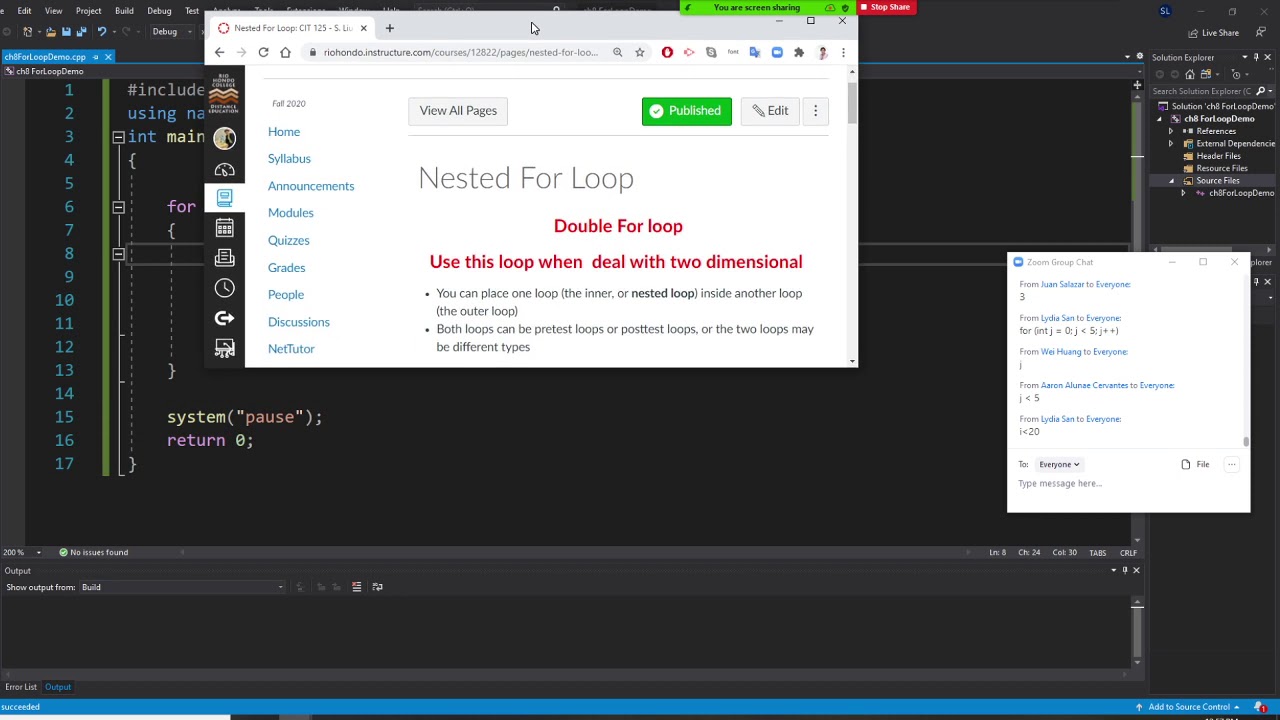
C++ programming, Nested For Loop Introduction
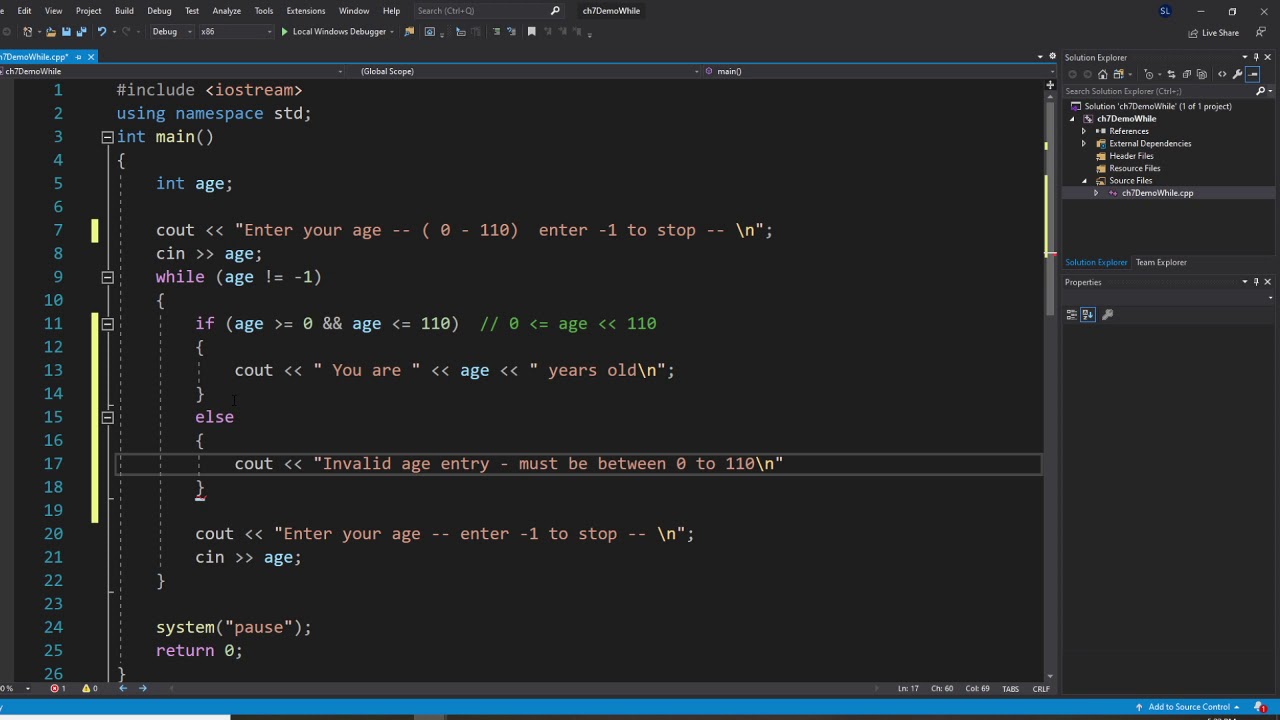
C++ read and display the age, use if else to prevent user enter invalid age. error handling
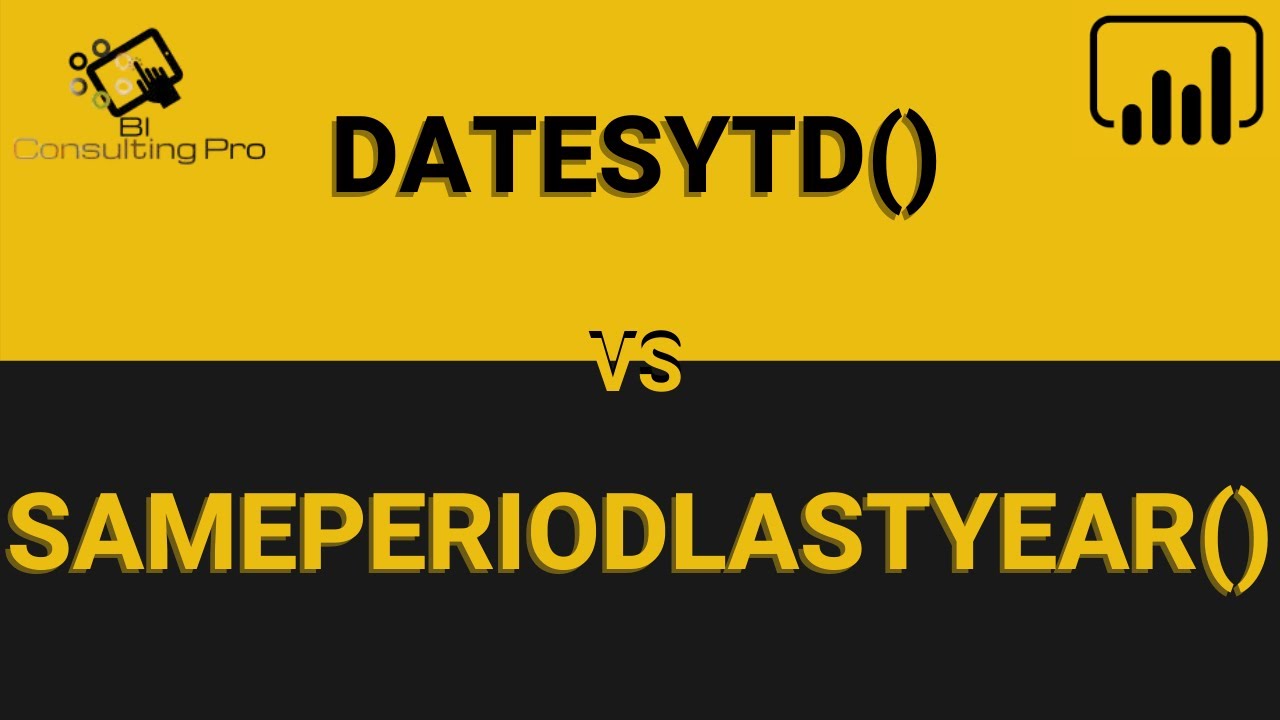
SAMPLEPERIODLASTYEAR Vs DATESYTD | DAX Sundays EP 4

Cara Menampilkan Tanggal pada PHP yang harus dipahami Programmer | #8 - Belajar PHP Dasar

Eloquent Javascript: Error Propagation
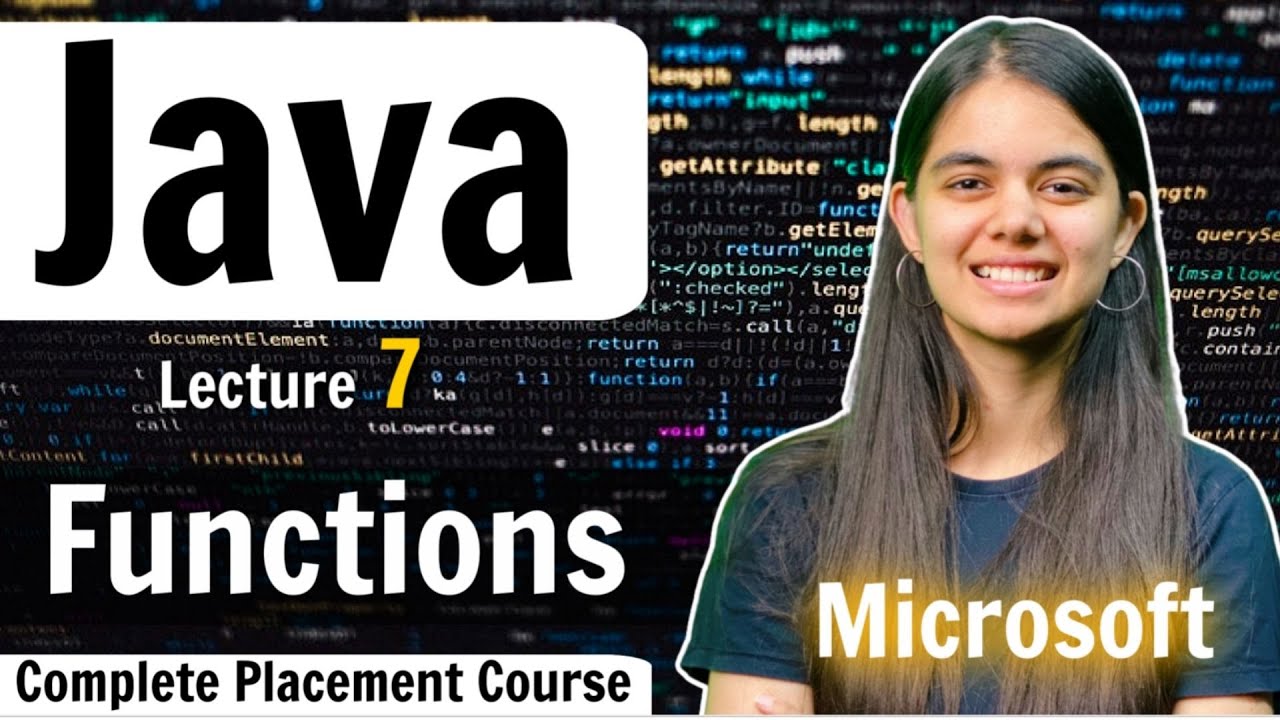
Functions & Methods | Java Complete Placement Course | Lecture 7
5.0 / 5 (0 votes)