3 Constructor
Summary
TLDRIn this session, we discuss constructors, a special function in object-oriented programming that initializes properties when an object is created. Instead of setting each property individually, constructors allow for efficient and streamlined initialization using parameters like name, color, and price. The tutorial demonstrates how to create a constructor using the '__construct' function, and how to access it during object instantiation. By leveraging constructors, developers can simplify code and enhance clarity, making the process of object creation more intuitive and less error-prone.
Takeaways
- 😀 A contractor is a special function used to initialize properties of an object when it is created.
- 😀 Instead of initializing properties individually, a contractor allows for a more efficient setup.
- 😀 To create a contractor, define a new function with the exact keyword '__construct'.
- 😀 You can initialize all desired properties by passing them as parameters in the contractor function.
- 😀 Using 'this' in the contractor allows you to assign the passed parameters to object properties.
- 😀 You can add additional properties (like price) directly in the contractor as needed.
- 😀 Accessing the contractor happens during object instantiation, using parentheses to pass parameters.
- 😀 Example parameters can include values like name, color, and price when creating an object.
- 😀 Ensure to use proper syntax, like the dot operator, when concatenating strings in methods.
- 😀 The contractor simplifies object creation by allowing all properties to be set in a single line.
Q & A
What is the primary purpose of a constructor in programming?
-The primary purpose of a constructor is to initialize the properties of an object at the time of its creation, making object instantiation more efficient.
How do you define a constructor in PHP?
-In PHP, a constructor is defined using the special function name '__construct', where you can specify parameters for the properties you want to initialize.
What syntax is used to initialize properties within a constructor?
-Within a constructor, properties are initialized using the syntax '$this->propertyName = parameterName;', where 'propertyName' is the object's property and 'parameterName' is the constructor parameter.
Can you give an example of properties that might be initialized in a constructor?
-Yes, examples of properties that might be initialized in a constructor include 'name', 'color', and 'price' for an object representing a product.
What is the advantage of using a constructor over setting properties individually after object creation?
-Using a constructor allows for all properties to be initialized in a single line, reducing the complexity of the code and improving readability and maintainability.
How are parameters passed to a constructor when creating an object?
-Parameters are passed to a constructor by including them in parentheses after the class name during object instantiation, like this: 'new ClassName(parameter1, parameter2, ...);'.
What happens if a property is not initialized in the constructor?
-If a property is not initialized in the constructor, it will be set to its default value (which is usually null for object properties in PHP) until it is explicitly set later.
Why is it important to use the '$this' keyword in constructors?
-The '$this' keyword is important because it refers to the current object instance, allowing you to access and assign values to the object's properties.
What type of errors might occur if there is a syntax mistake in the constructor?
-Common errors include syntax errors, such as missing commas or incorrect property names, which can lead to runtime errors when trying to instantiate the object or access its properties.
How can constructors improve the overall structure of a class?
-Constructors improve the overall structure of a class by encapsulating the initialization logic within the class itself, making it easier to manage and understand how objects of that class are created.
Outlines
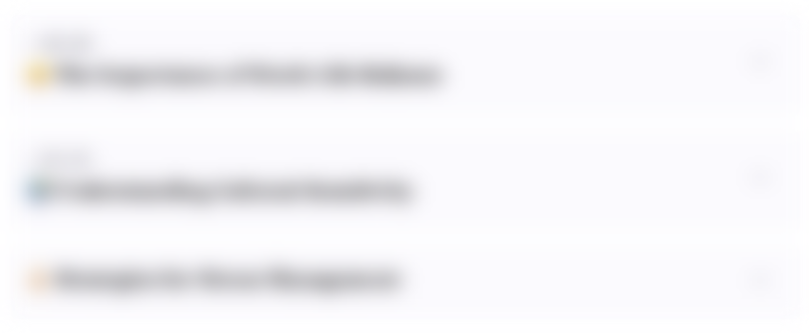
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
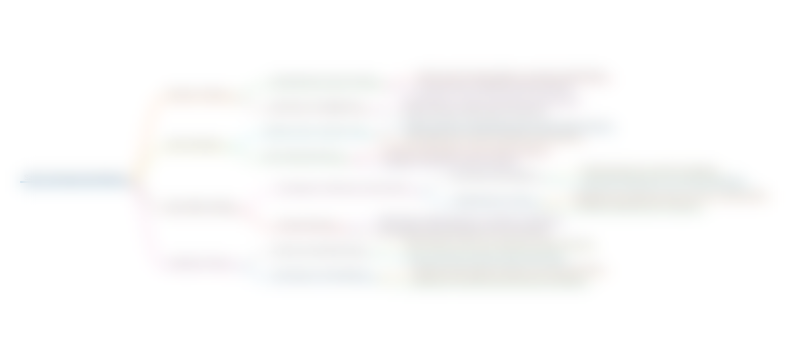
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
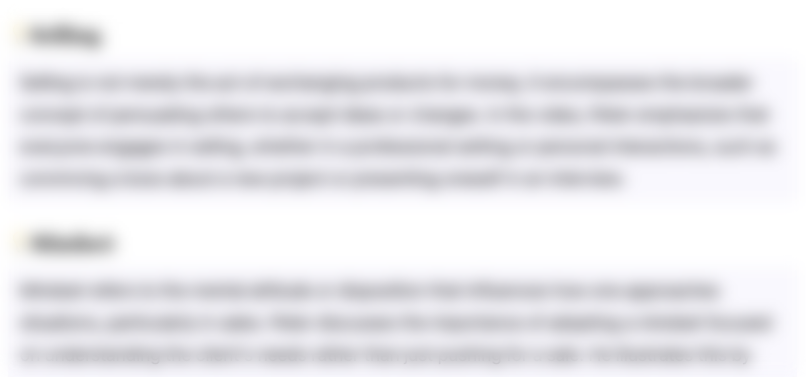
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
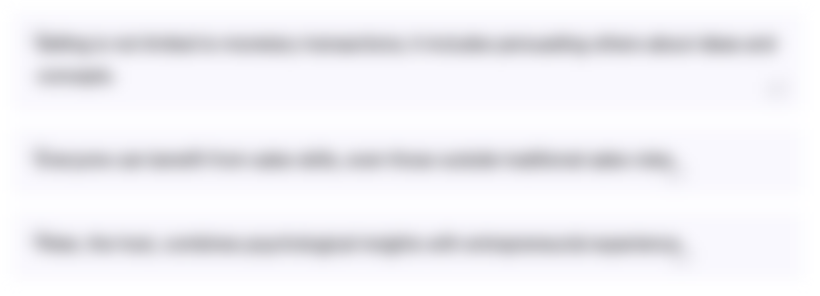
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
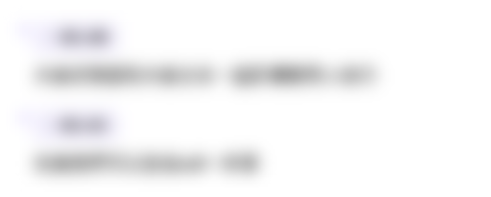
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen

Class Objects & Constructors | Godot GDScript Tutorial | Ep 16

Vlang a pragmatický přístup programování

ICS2O - Lesson 5 - Introduction to Alice

Object Oriented Programming Dasar pada C++ (Class, Object, Method, dan Constructor)
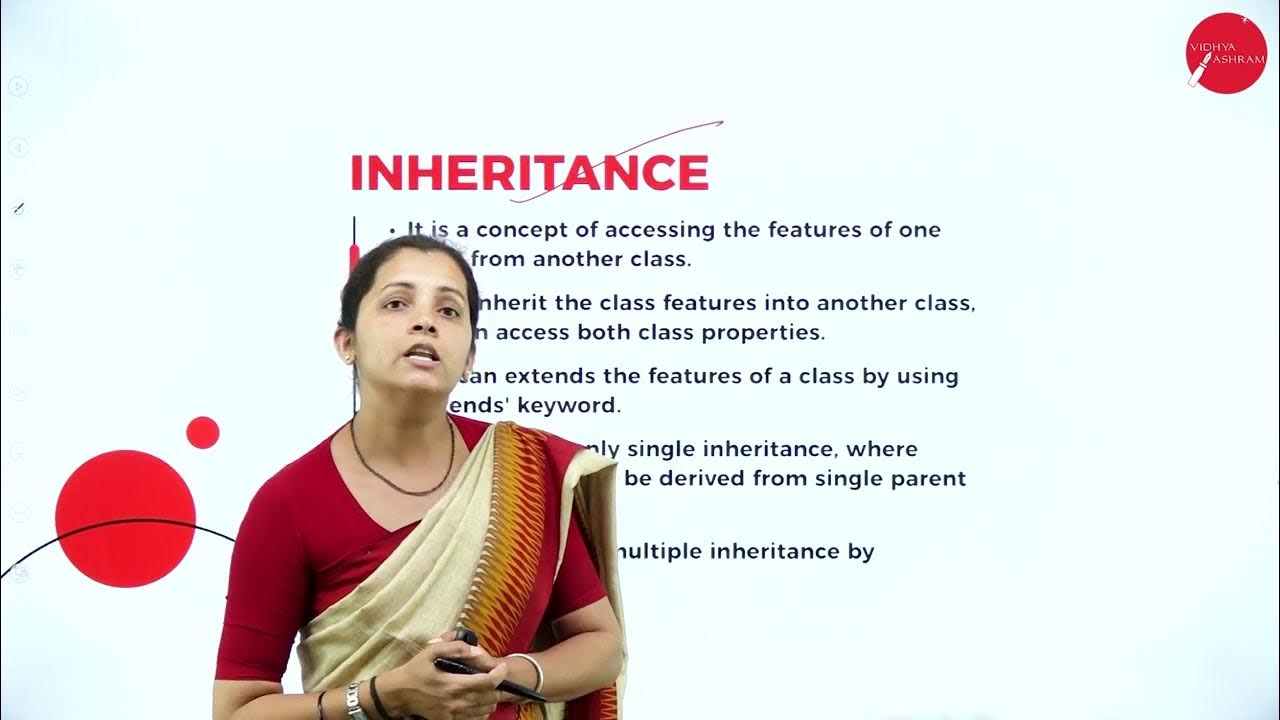
DAY 07 | PHP AND MYSQL | VI SEM | B.CA | CLASS AND OBJECTS IN PHP | L1
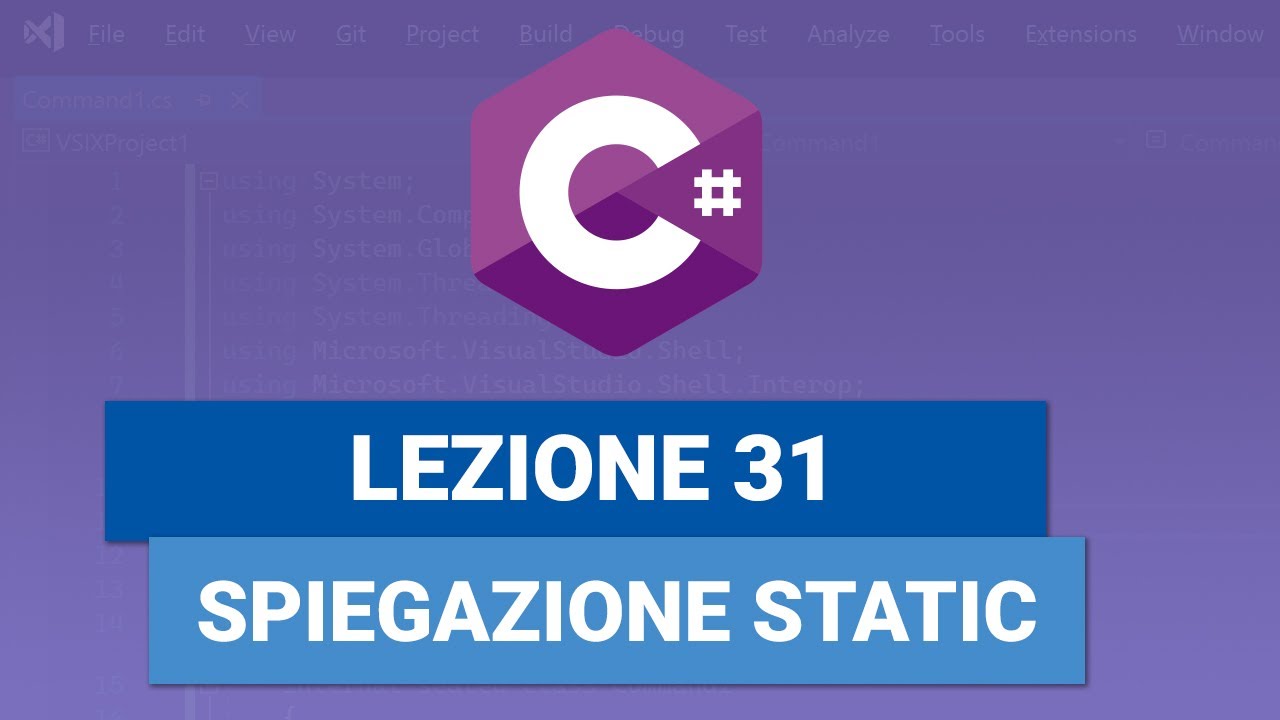
Parole chiave STATIC - C# TUTORIAL ITALIANO 31
5.0 / 5 (0 votes)