For Loops in Python | Python for Beginners
Summary
TLDRThis video introduces Python for loops, explaining how they work to iterate over sequences like lists, tuples, strings, and dictionaries. The tutorial begins with a simple example, printing numbers from a list, and gradually builds to more complex scenarios, including manipulating loop variables, using dictionaries, and demonstrating nested for loops. The instructor explains each concept with code examples, showing how for loops can handle different data types and become more intricate with nesting. Viewers are encouraged to follow along to better understand the logic behind Python's looping mechanisms.
Takeaways
- 🔄 The for loop in Python is used to iterate over a sequence like a list, tuple, array, string, or dictionary.
- 📜 A for loop checks each item in the sequence and performs an action until it reaches the last element.
- 🖥️ The syntax of a for loop starts with 'for', followed by a temporary variable, then 'in', and the sequence being iterated over.
- 🧮 The loop assigns each item in the sequence to the temporary variable and executes the body of the loop.
- 🔢 You can print each element in the sequence, or even perform other operations with or without the temporary variable.
- 🧑💻 For loops can be used with dictionaries, where you can loop through the keys, values, or both (using `.items()` for key-value pairs).
- 🍨 A nested for loop allows iterating over two sequences, where one loop runs inside another, generating combinations.
- 📋 For loops can handle both simple tasks, like printing values, or more complex operations like summing elements or working with dictionary values.
- 👨🏫 Python's for loop is flexible and can work with a variety of data structures, from lists to dictionaries to even nested structures.
- 💡 Nested for loops allow for complex operations, like iterating through combinations of two lists, but can become harder to manage as complexity increases.
Q & A
What is a for loop in Python used for?
-A for loop in Python is used to iterate over a sequence, such as a list, tuple, array, string, or dictionary. It allows you to execute a block of code multiple times for each element in the sequence.
What are the key components of a basic for loop syntax in Python?
-A basic for loop in Python includes the 'for' keyword, a temporary variable to hold the current item from the sequence, the 'in' keyword, and the sequence being iterated over. This is followed by a colon and the body of the loop, which contains the code that runs during each iteration.
How does the iteration process work in a for loop?
-In each iteration, the for loop checks if it has reached the last element of the sequence. If not, it moves to the body of the loop, processes the current item, and then proceeds to the next item. This continues until all items in the sequence have been processed.
Can a for loop operate on other data structures besides a list?
-Yes, a for loop can iterate over various data structures in Python, including lists, tuples, strings, arrays, and dictionaries.
What happens if you don't use the loop variable inside the body of the for loop?
-If you don't use the loop variable inside the body of the for loop, the loop will still iterate over the sequence, but the variable won't affect the operations inside the loop. For example, you can just print a constant value in each iteration without using the variable.
Can the loop variable name be anything?
-Yes, the loop variable name can be any valid Python variable name. It doesn't have to be 'number' or any specific name. You can use any descriptive name like 'item', 'element', or even something unconventional like 'jelly'.
How do you iterate over a dictionary using a for loop?
-To iterate over a dictionary, you can use 'dictionary.values()' to loop through the values or 'dictionary.items()' to iterate over both keys and values. You can also use 'dictionary.keys()' to loop through just the keys.
What does a nested for loop do?
-A nested for loop is a for loop inside another for loop. The inner loop completes all its iterations for each iteration of the outer loop. It is often used to work with multidimensional data or for creating combinations of elements from multiple lists.
How does the example of the nested for loop with flavors and toppings work?
-In the nested for loop example, the outer loop iterates over the list of flavors, and for each flavor, the inner loop iterates over the list of toppings. This creates a combination of each flavor with each topping, printing out phrases like 'vanilla topped with hot fudge', 'vanilla topped with oreos', and so on.
What is the difference between 'dictionary.items()' and 'dictionary.values()' in a for loop?
-'dictionary.items()' returns both the keys and values of the dictionary, allowing you to access both in each iteration. 'dictionary.values()' only returns the values, so you can only access the values, not the keys.
Outlines
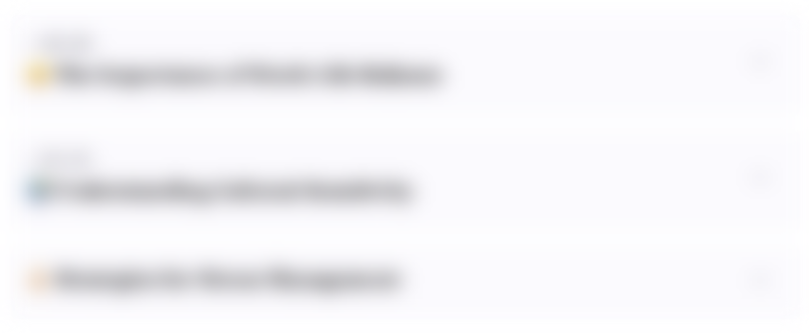
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
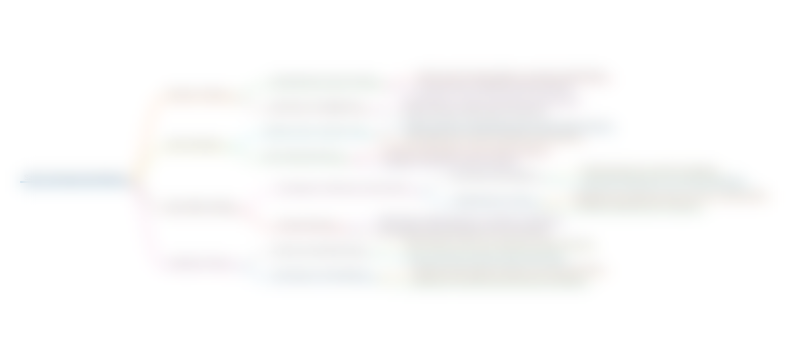
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
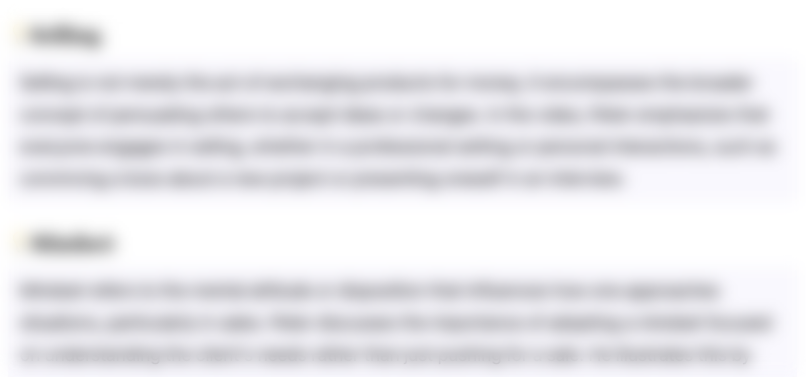
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
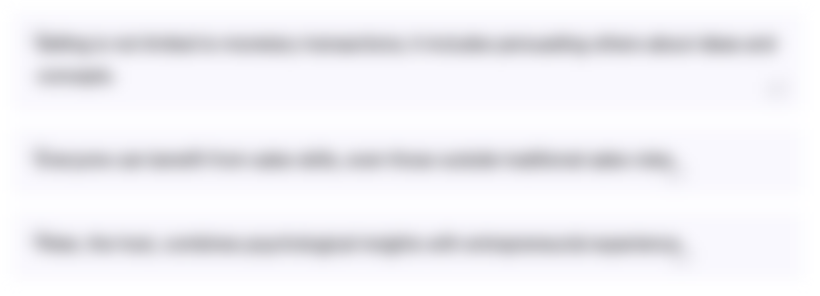
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
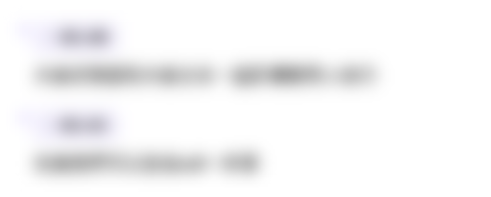
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
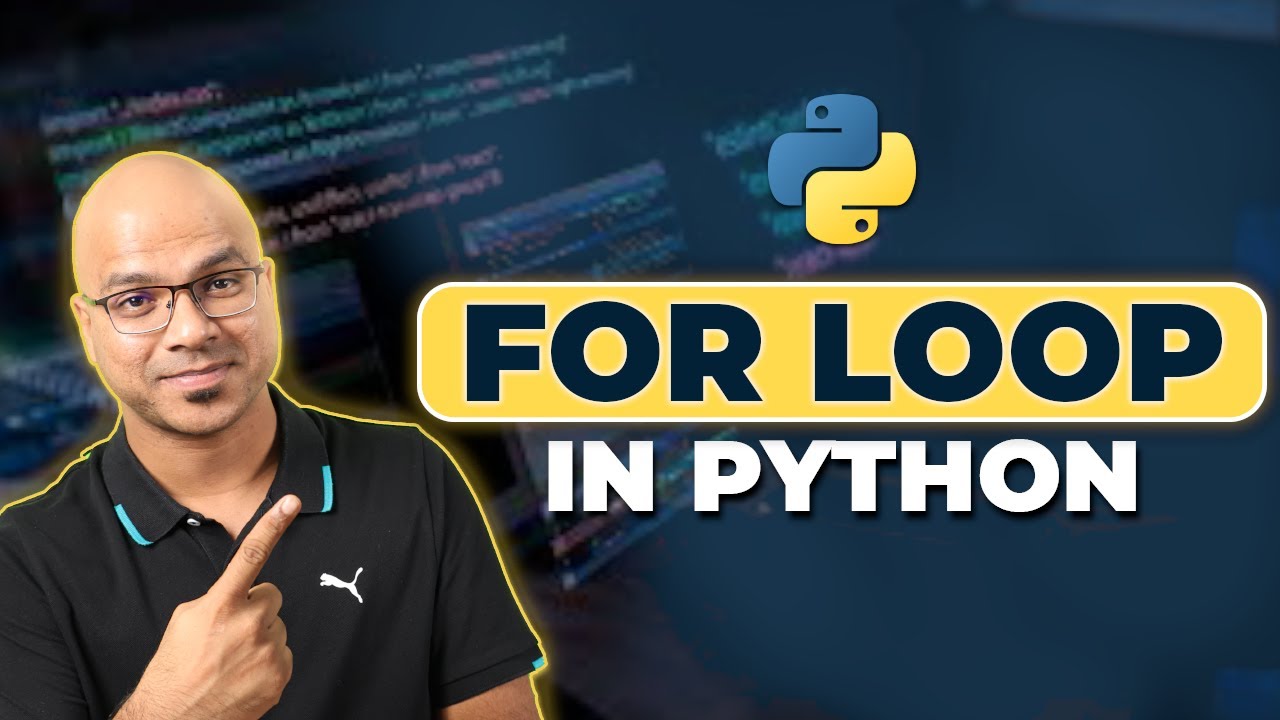
#21 Python Tutorial for Beginners | For Loop in Python
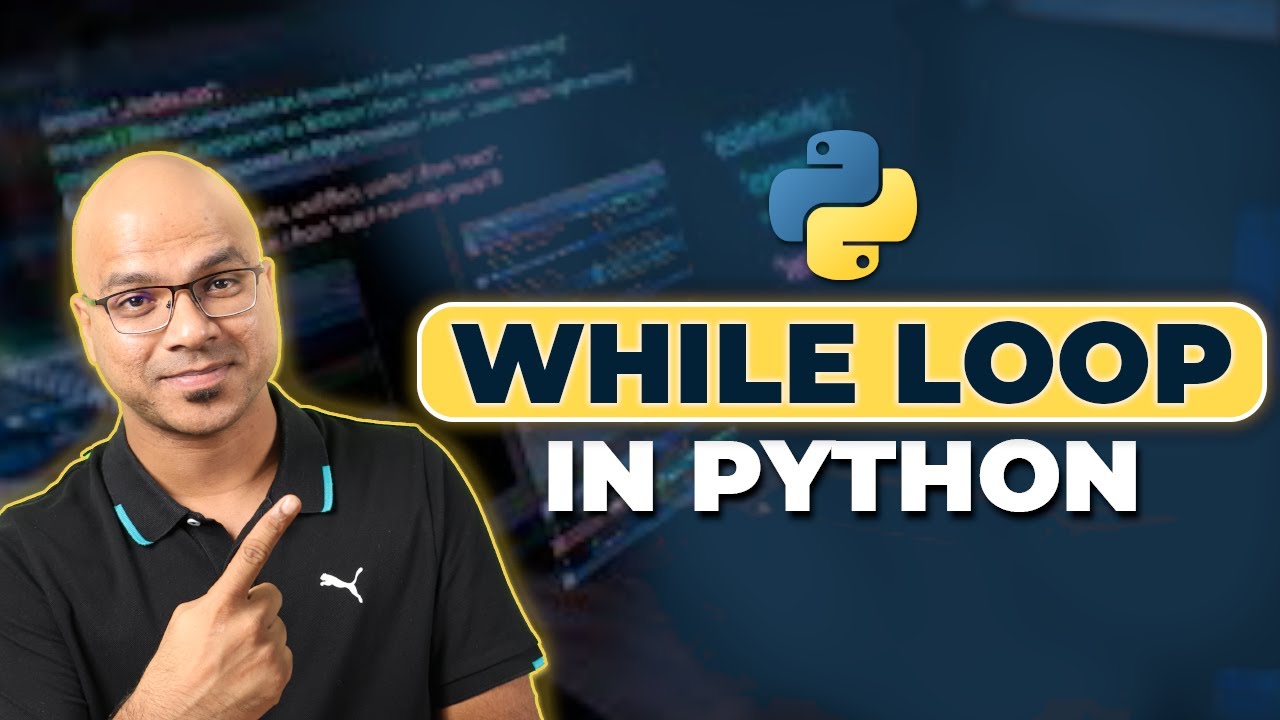
#20 Python Tutorial for Beginners | While Loop in Python
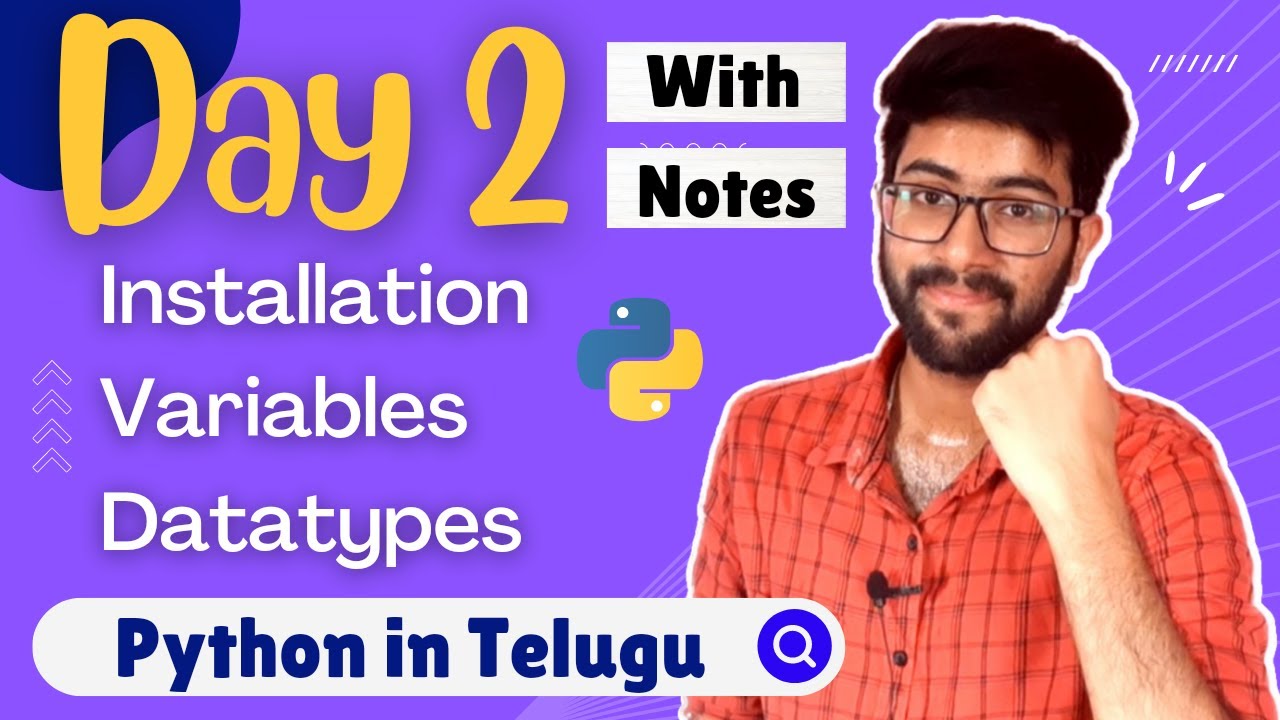
Day 2 : Python Installation, Variables, Datatypes | Python Course in Telugu | Vamsi Bhavani
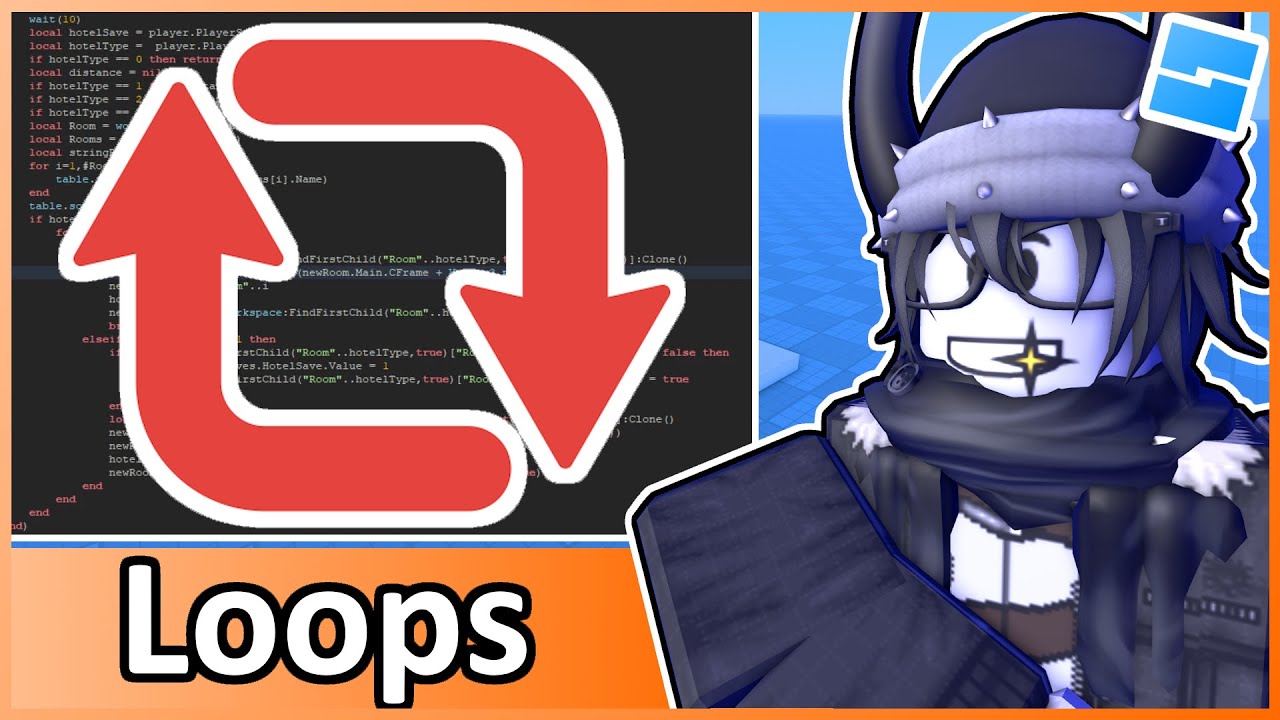
Loops - Roblox Beginners Scripting Tutorial #11 (2024)
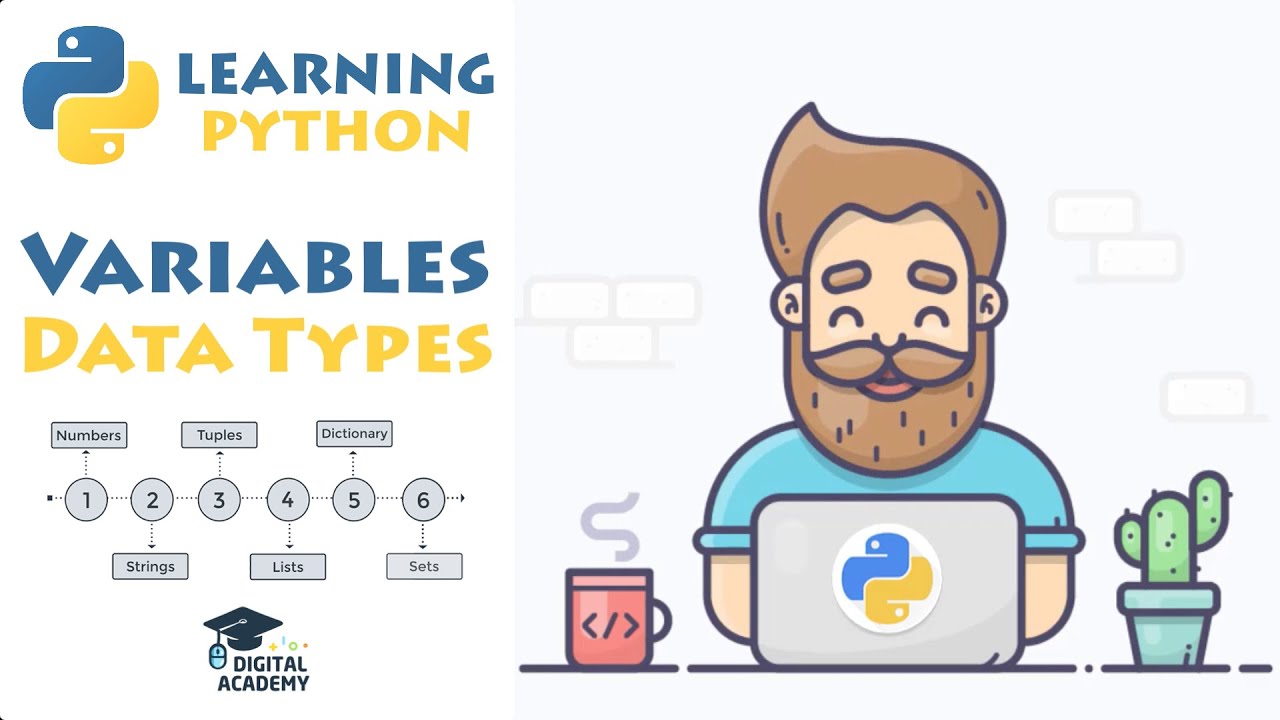
DATA TYPES in Python (Numbers, Strings, Lists, Dictionary, Tuples, Sets) - Python for Beginners
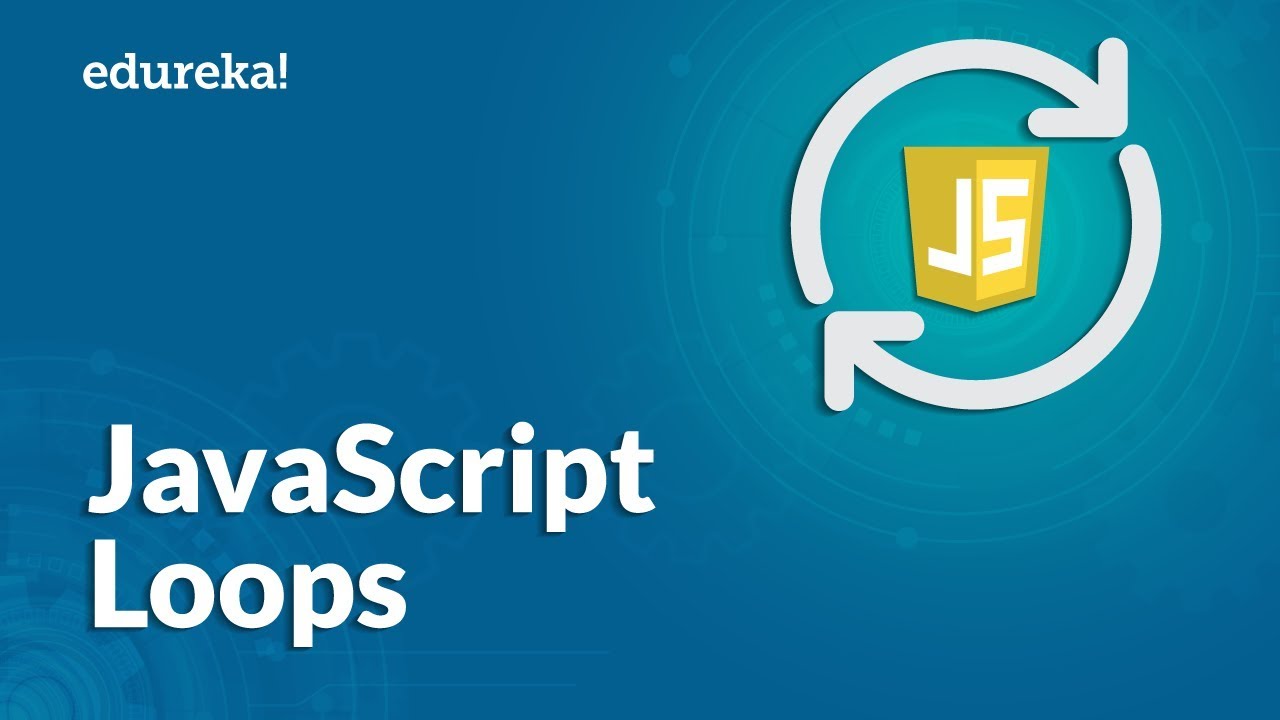
JavaScript Loops Explained | For Loop, While and Do-While Loop | JavaScript Tutorial | Edureka
5.0 / 5 (0 votes)