Python for Coding Interviews - Everything you need to Know
Summary
TLDRIn this coding tutorial, the host shares insights on using Python for coding interviews, highlighting its simplicity and conciseness. They discuss Python's dynamic typing, control structures, and various data structures like lists, sets, and dictionaries, emphasizing their practical use in interviews. The video also covers string manipulation, advanced data structures like heaps, and Python's syntax for functions and classes. Aimed at interview preparation, the host recommends neco.io for further resources, courses, and practice problems in multiple programming languages.
Takeaways
- 😀 The speaker learned Python specifically for coding interviews and found it helpful for its conciseness.
- 🔍 Python is a dynamically typed language, which allows for flexibility in variable assignment without declaring types.
- 🚫 Python does not support the '++' operator for incrementing variables, differing from some other programming languages.
- 💡 The 'None' keyword in Python represents null, and variables can be reassigned from other types to 'None'.
- 🔑 Python uses indentation rather than curly braces to define code blocks, which is a syntactical difference from languages like Java or C++.
- 🔄 The 'and' and 'or' keywords are used for logical operations instead of symbols, enhancing readability.
- 🔢 Python performs decimal division by default, unlike many languages that default to integer division.
- 📚 Lists (arrays) in Python are dynamic and provide various methods for manipulation, including append, pop, insert, and slice.
- 🔤 Strings in Python are immutable, meaning they cannot be changed after creation, but new strings can be created easily.
- 🔄 The 'zip' function is useful for iterating over multiple arrays simultaneously, combining their elements into pairs.
- 📚 Dictionaries (hashmaps) in Python are versatile and allow for key-value pair storage with efficient search and insertion.
Q & A
What is the main focus of the video?
-The main focus of the video is to cover everything one needs to know about Python for coding interviews.
Why did the channel creator learn Python specifically for coding interviews?
-The channel creator learned Python specifically for coding interviews because it is easier and more concise than most languages, which was helpful throughout all his interviews at Google.
What are some benefits of Python mentioned in the video?
-Python is mentioned to be beneficial for coding interviews due to its ease of use, conciseness, and dynamic typing, which allows for flexibility in variable assignments and types.
How does Python handle variable types differently from statically typed languages?
-Python, being a dynamically typed language, allows variables to be assigned values without declaring their types, and types are determined at runtime, enabling variables to change types.
What is the significance of the 'None' keyword in Python?
-In Python, 'None' represents the absence of a value, which is similar to 'null' in other languages, and it can be assigned to variables that are expected to hold other types of values.
How does Python syntax for if statements differ from other languages?
-Python syntax for if statements does not require parentheses for the conditional and uses indentation instead of curly braces to define the block of code associated with the conditional.
What is the default behavior of division in Python?
-In Python, division is decimal division by default, which means it will produce a floating-point result, unlike many other languages that use integer division by default.
How can one perform integer division in Python?
-To perform integer division in Python, one can use the double slash operator '//', which will round the result towards zero.
What is a key difference between Python lists and arrays in other languages?
-Python lists are dynamic arrays that can have items of different types and are easily manipulated with various built-in methods, such as append, pop, and sort.
How does Python handle string immutability?
-Python strings are immutable, which means that once a string is created, its characters cannot be changed. Any operation that appears to modify a string actually creates a new string.
What is a unique feature of Python sets?
-Python sets are collections of unique elements and are useful for membership testing, removing duplicates, and performing mathematical set operations.
How can one implement a Max Heap in Python?
-In Python, one can implement a Max Heap by using the negative values of the elements, pushing them onto a Min Heap, and then negating them again when popping from the heap.
Outlines
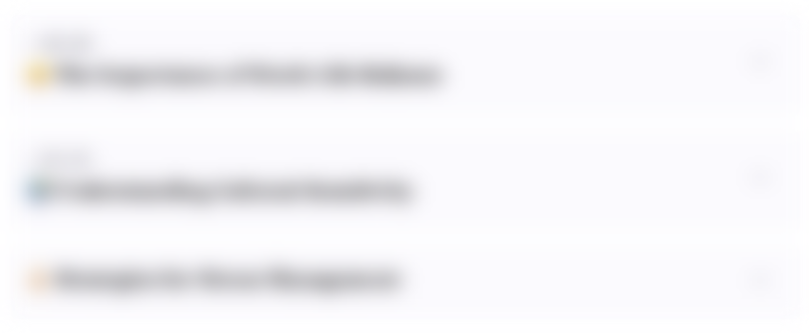
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
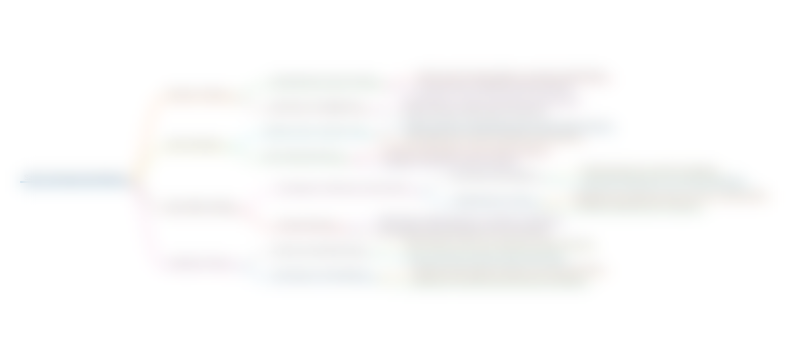
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
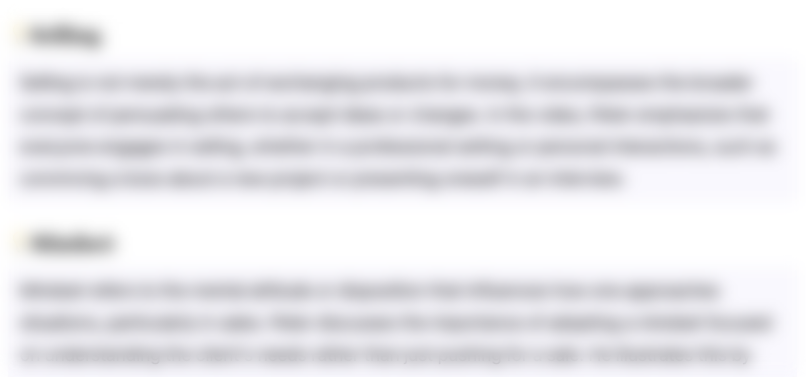
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
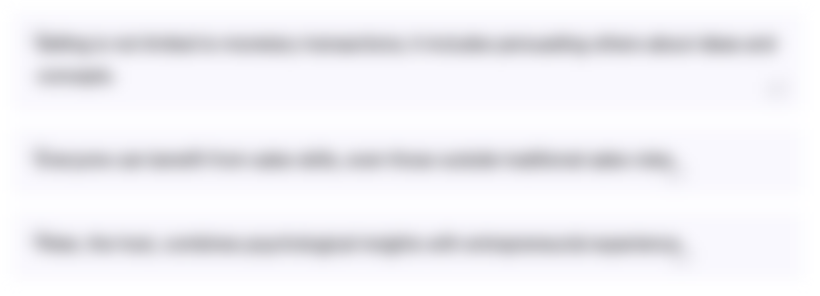
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
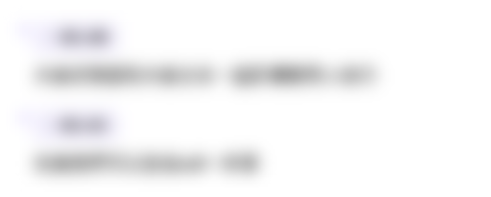
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen

Everything you need to know to become a quant trader (in 2024) + sample interview problem
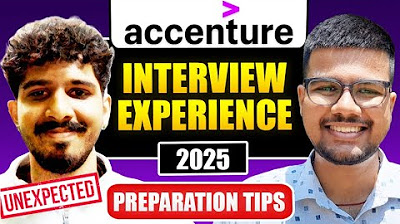
Accenture 2025 Actual Interview: What Really Happened (Unexpected)
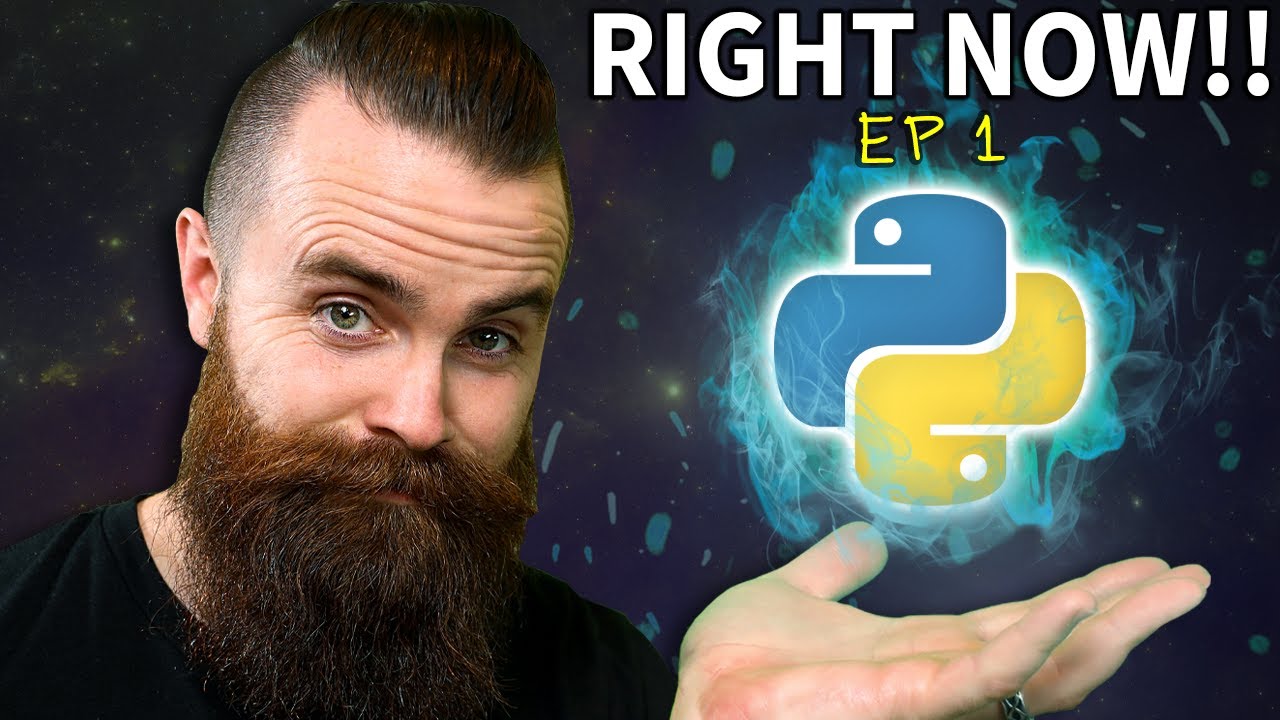
you need to learn Python RIGHT NOW!! // EP 1
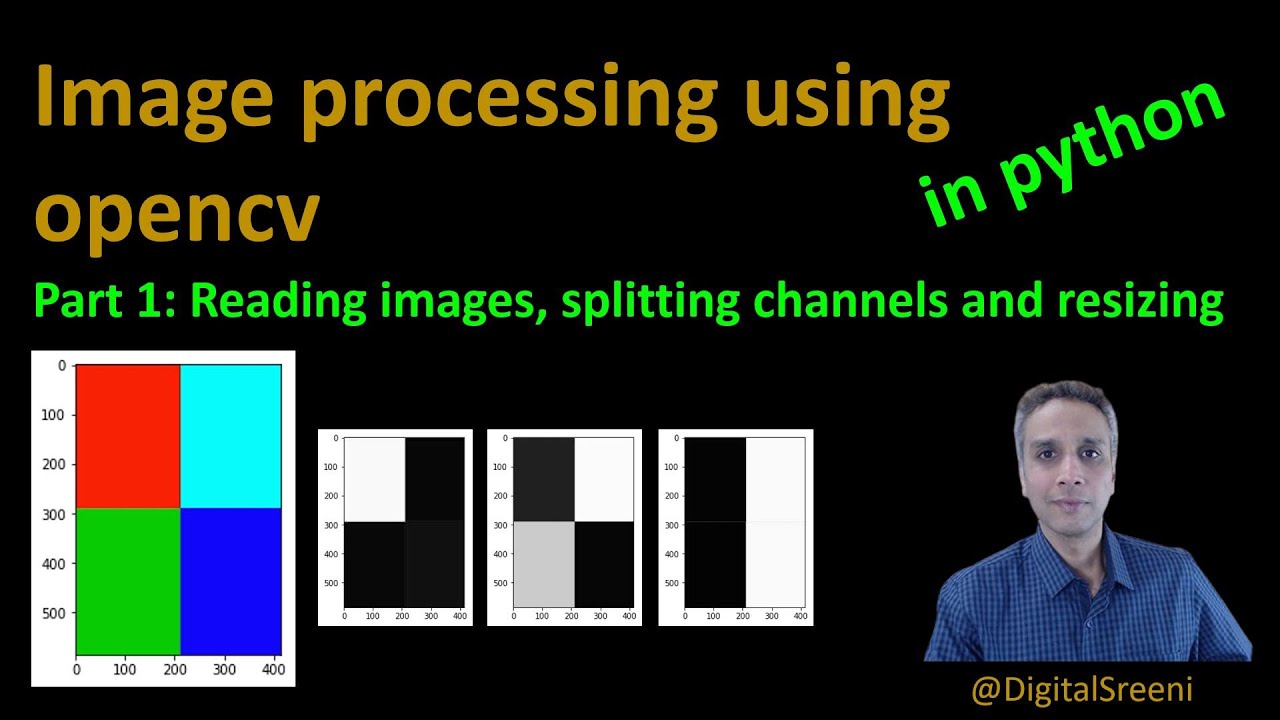
25 - Reading Images, Splitting Channels, Resizing using openCV in Python
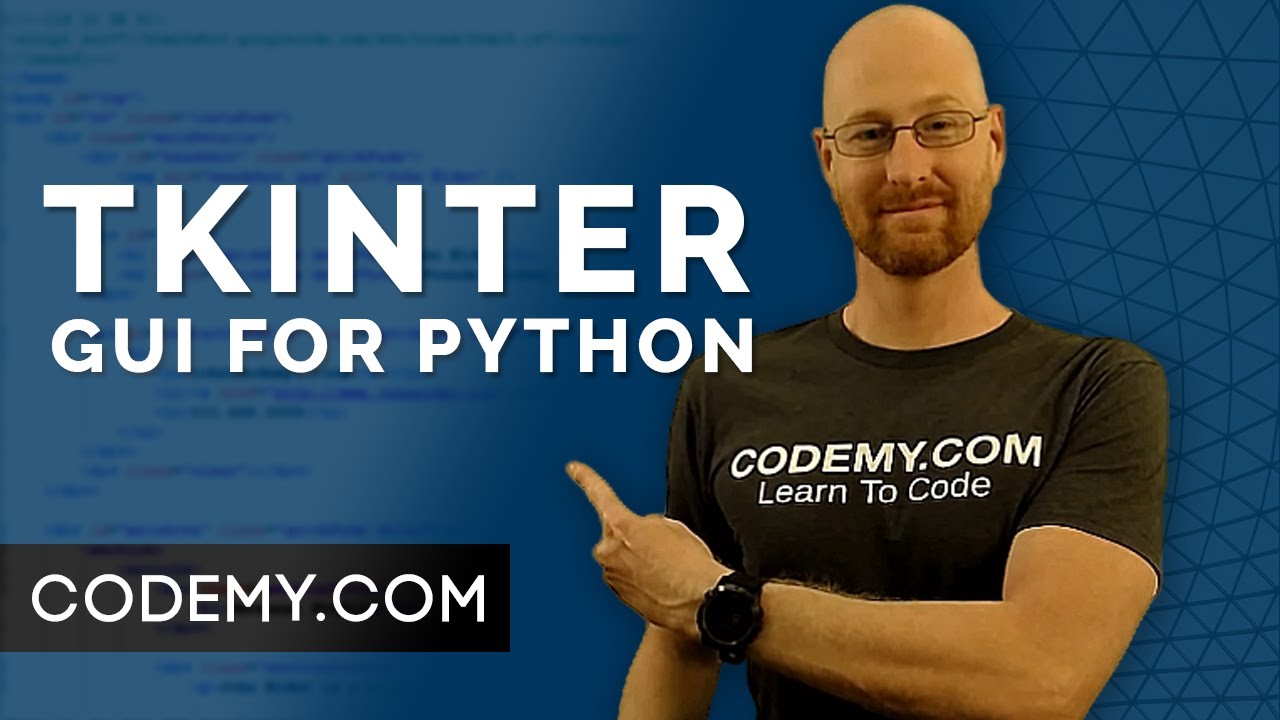
Create Graphical User Interfaces With Python And TKinter
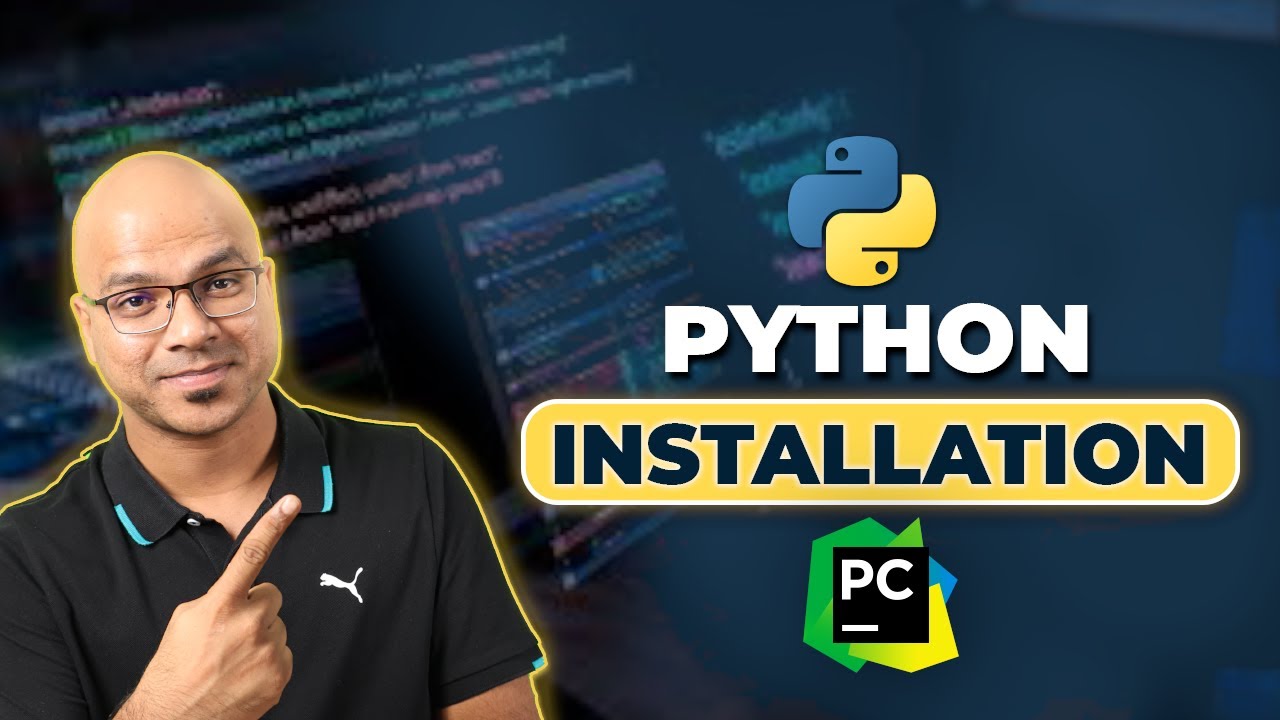
#2 Python Tutorial for Beginners | Python Installation | PyCharm
5.0 / 5 (0 votes)