Tic Tac Toe Game In Python | Python Project for Beginners
Summary
TLDRIn this Python programming tutorial, Sagar introduces viewers to a project series where he teaches how to create a Tic-Tac-Toe game with a graphical user interface (GUI). The game involves two players, X and O, competing on a 3x3 grid. The first player to complete a line horizontally, vertically, or diagonally wins. Sagar demonstrates the game setup, including importing modules for GUI elements, defining functions to check for a winner, and handling button clicks. The script also covers creating buttons, arranging them in a grid, and updating the game state. The tutorial is interactive, encouraging viewers to try the game and share their feedback.
Takeaways
- 😀 The video is a tutorial on creating a Tic Tac Toe game in Python.
- 🎮 The game features a graphical user interface (GUI) for ease of play.
- 👾 Two players, X and O, take turns placing their symbols on a 3x3 grid.
- 🏆 The first player to complete a line horizontally, vertically, or diagonally wins.
- 🛠️ The 'Tkinter' module is used for creating the GUI elements of the game.
- 🔍 A 'check_winner' function is defined to verify if a player has won after each move.
- 🔑 The script includes a list of possible winning combinations for both horizontal, vertical, and diagonal lines.
- 💡 The 'button_click' function is used to handle player moves and update the game state.
- 🔄 The 'toggle_player' function alternates between players X and O after each turn.
- 🖥️ The 'root' window is created to house the game's GUI components.
- 🔢 A 'Label' widget is used to display whose turn it is and to show game status messages.
Q & A
What is the primary focus of the video series that Sagar is welcoming viewers to?
-The primary focus of the video series is a Python programming project series where Sagar is guiding viewers on how to create a Tic Tac Toe game.
What does 'GI' stand for in the context of the video?
-In the video, 'GI' stands for Graphical User Interface, which is used to make the game visually appealing and easier for players to interact with.
What is the basic gameplay of Tic Tac Toe as described in the script?
-Tic Tac Toe is a game played between two players, X and O, who take turns placing their symbols on a 3x3 grid. The player who first completes a line with their symbol, either horizontally, vertically, or diagonally, wins the game.
What module does the script mention for creating buttons and other graphical elements in Python?
-The script mentions the 'tkinter' module, which is used for creating buttons, labels, and other graphical elements in Python.
What function does the script define to check for a winner after every button click?
-The script defines a function called 'check_and_winner' to check for a winner after every button click by verifying if any of the possible winning combinations match the current state of the game board.
How does the script plan to indicate a win in the game?
-The script plans to indicate a win by changing the background color of the buttons that form a winning line to green and displaying a message to the user through a message box.
What is the purpose of the 'toggle_player' function in the script?
-The 'toggle_player' function is used to switch the current player from X to O or vice versa after each turn, ensuring that the game alternates between players.
How does the script handle the game's main loop to display the window and handle user input?
-The script uses the 'mainloop' method of the root window to handle the game's main loop, which is responsible for displaying the window on the screen and managing user input.
What is the significance of the 'current_player' variable in the script?
-The 'current_player' variable is used to keep track of whose turn it is in the game, alternating between 'X' and 'O' with each turn.
How does the script ensure that the game only progresses if the button is empty and the game is still ongoing?
-The script checks if the button is empty and the 'winner' variable is False (indicating the game is ongoing) before setting the current player's symbol on the button and calling the 'check_winner' function.
What is the role of the 'label' widget in the script?
-The 'label' widget in the script is used to display information to the user, such as whose turn it is, and to provide updates on the game's status.
Outlines
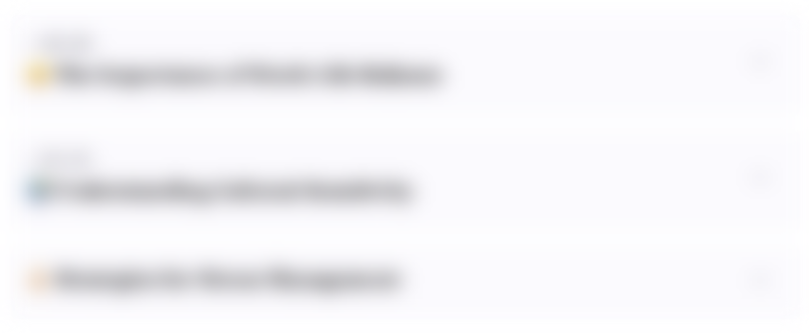
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
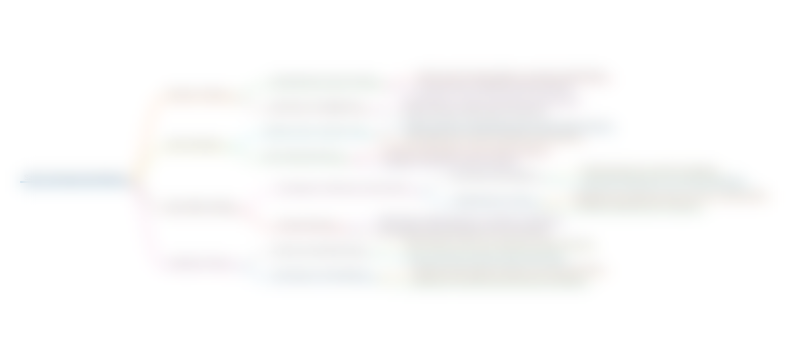
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
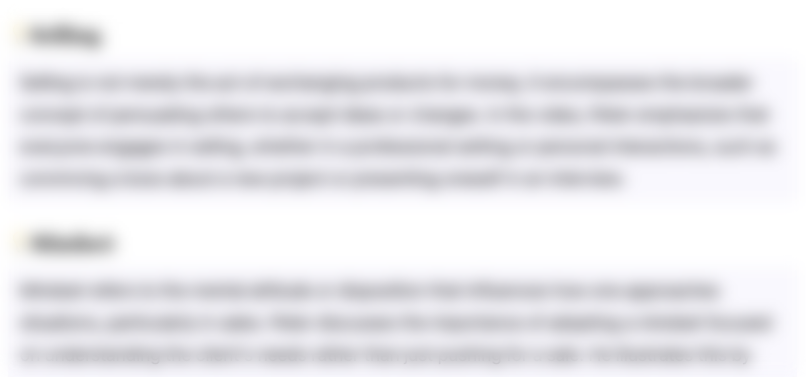
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
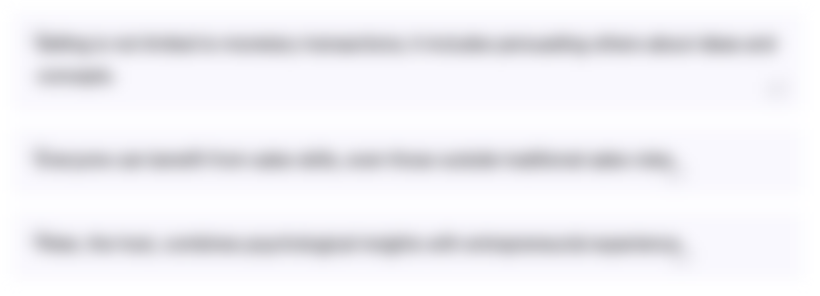
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
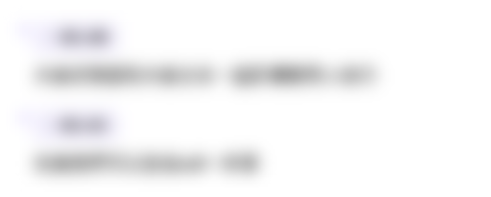
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
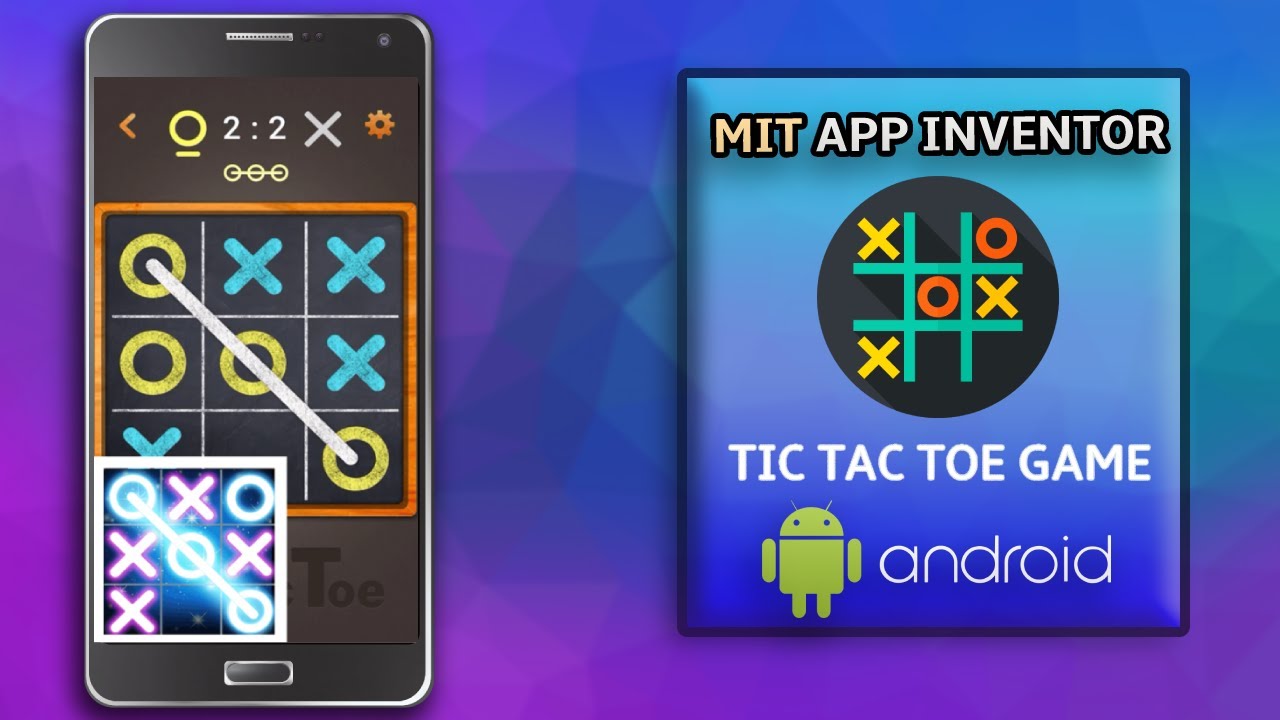
Create Tic Tac Toe Game in MIT App Inventor in Just 10 Minutes! || Free Extension || App Inventor 2
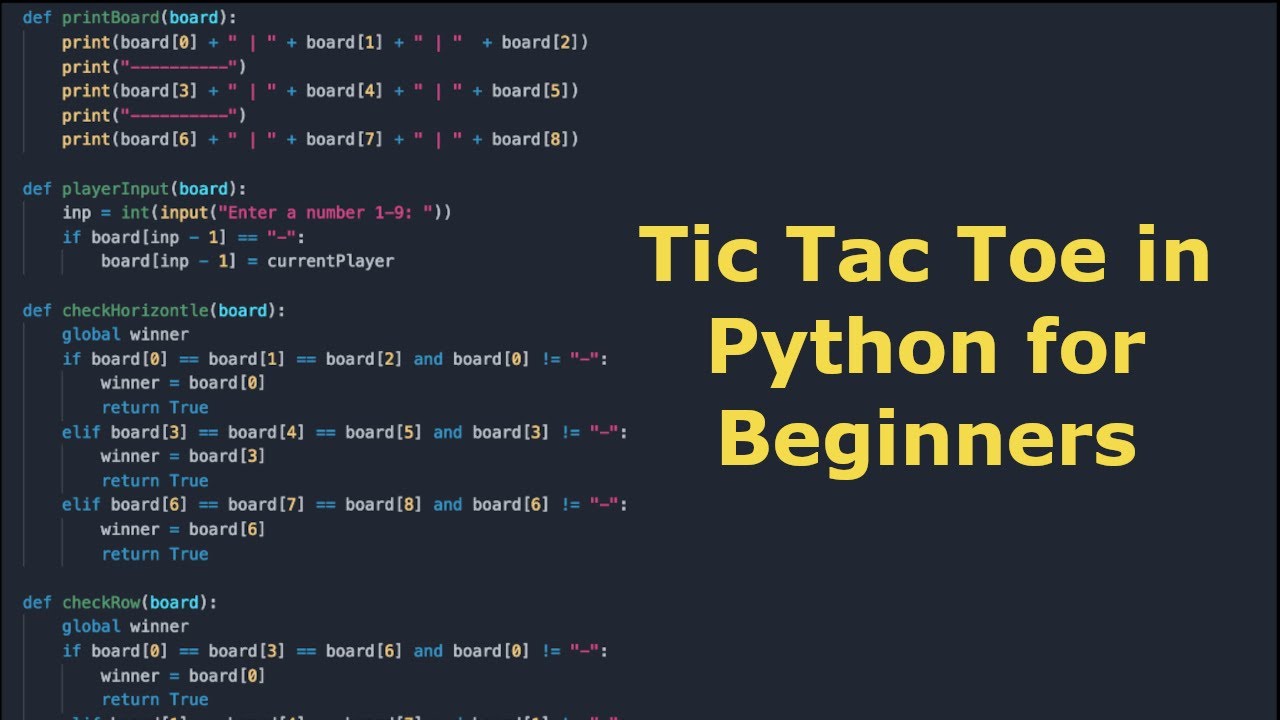
Python TIC TAC TOE Tutorial | Beginner Friendly Tutorial

Tic Tac Toe - Down the memory Lane 02
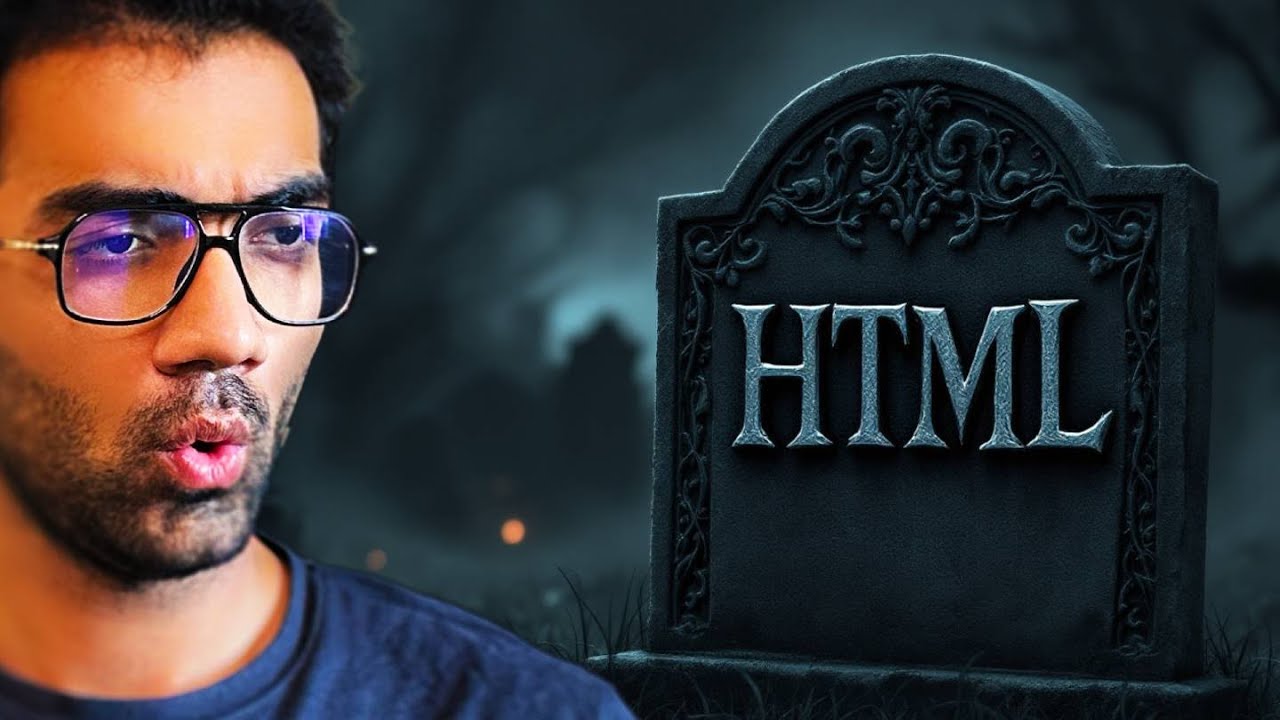
Leave HTML - You can code frontend with Python now
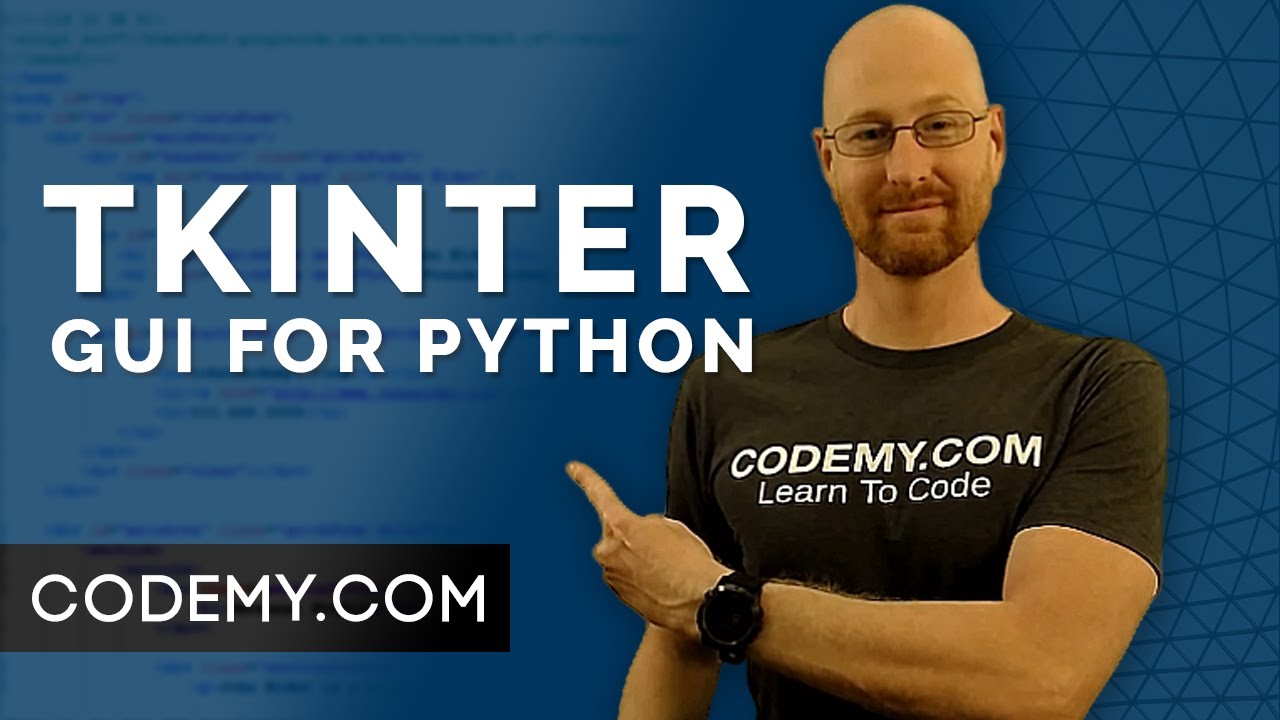
Create Graphical User Interfaces With Python And TKinter
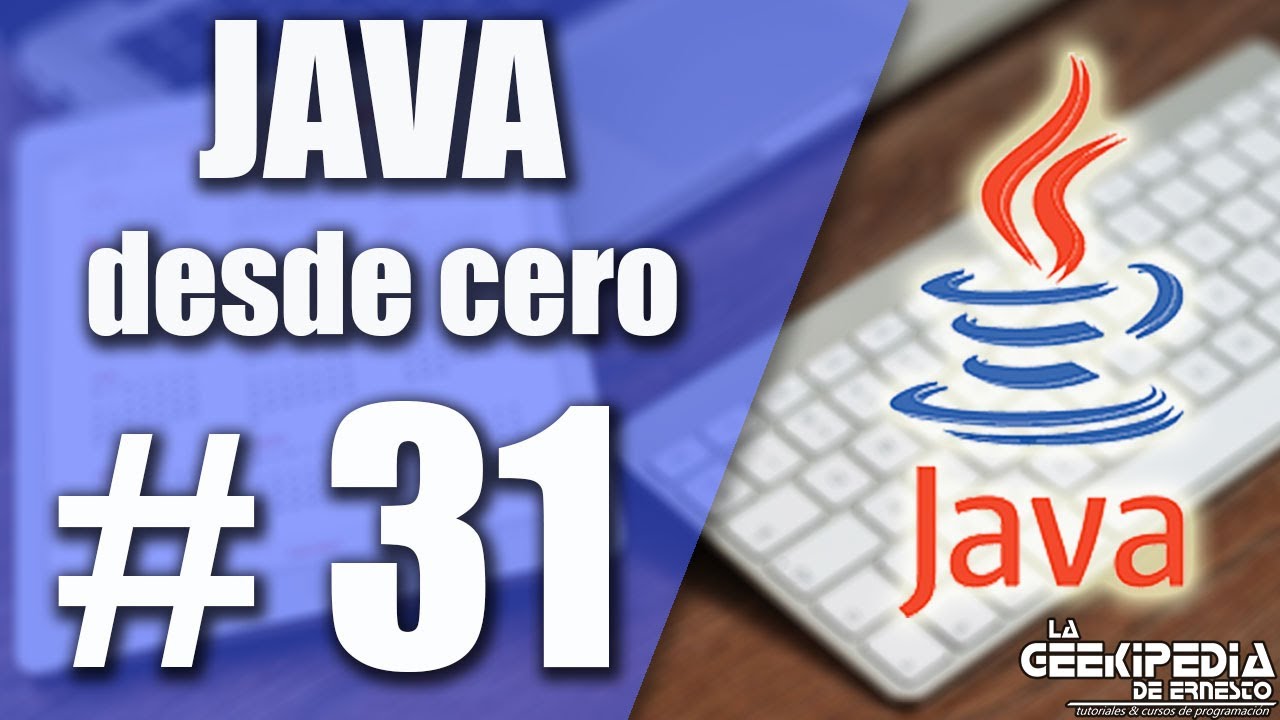
Curso Java desde cero #31 | Interfaces gráficas (Swing - JScrollPane)
5.0 / 5 (0 votes)