Using Python: Part 1: Console Input and Output
Summary
TLDRThis Python tutorial focuses on console input and output, essential for interacting with the command line interface. It introduces the 'input' function to capture user text and the 'print' function to display text back to the console. The video demonstrates how to use these functions to receive and show information, highlighting their importance in console-based Python programming.
Takeaways
- 💻 The video is about using Python for console input and output.
- 🔑 The console is a non-graphical user interface, also known as the command line.
- 📝 The 'input' function in Python is used to take textual input from the console.
- 📢 The 'print' function in Python is used to display text on the console.
- 🔍 The input function captures whatever is typed on the console and returns it.
- 📌 The print function takes an argument and displays it on the console.
- 💬 The video demonstrates how to use 'input' and 'print' functions in a Python script.
- 🎥 The presenter runs a Python script to show how input is echoed back using the 'input' function.
- 🔧 Both 'input' and 'print' are essential for working with the console in Python.
- 📚 The video concludes by emphasizing the importance of input and output in console-based Python programming.
Q & A
What is the primary purpose of using Python's console input and output?
-The primary purpose is to interact with users by taking input from the console and displaying output back to it, facilitating communication between the user and the Python program.
What is the function used in Python to get input from the console?
-The function used in Python to get input from the console is `input()`.
How does the `input()` function work in Python?
-The `input()` function in Python takes the text entered by the user on the console and returns it as a string, which can then be stored in a variable.
What is the function used in Python to display output on the console?
-The function used in Python to display output on the console is `print()`.
How does the `print()` function display information on the console?
-The `print()` function takes the arguments provided to it and displays them on the console as output, which can be text or values of variables.
What happens when you run a Python script that uses the `input()` function?
-When you run a Python script with the `input()` function, the program execution pauses, waiting for the user to enter text and press Enter before proceeding.
Can the `input()` function be used to get non-textual input from the console?
-No, the `input()` function is designed to receive textual input. If numerical input is needed, it must be converted from the string returned by `input()`.
What is the significance of the line number mentioned in the script when discussing the `input()` function?
-The line number mentioned in the script refers to the specific location in the code where the `input()` function is used to demonstrate how to capture user input.
Can the `print()` function be used to display multiple pieces of information on the console?
-Yes, the `print()` function can be used to display multiple pieces of information by passing multiple arguments separated by commas.
What is the practical application of using `input()` and `print()` functions in Python?
-The practical application includes creating interactive programs, taking user commands, processing data based on user input, and providing feedback or results to the user.
How can you ensure that the user input is of the expected type when using the `input()` function?
-To ensure the user input is of the expected type, you can use type conversion functions like `int()`, `float()`, or `str()` to convert the input string to the desired data type.
Outlines
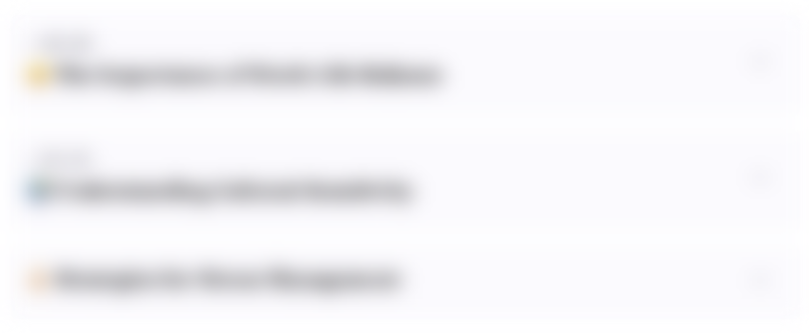
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
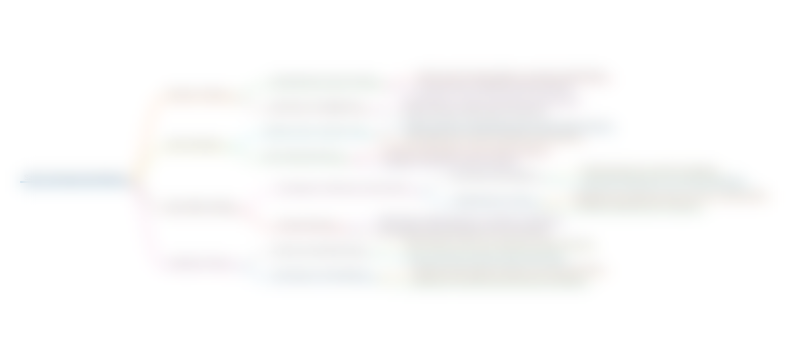
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
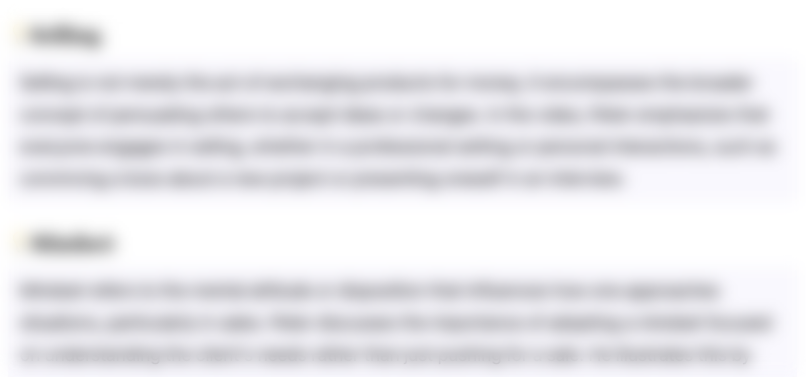
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
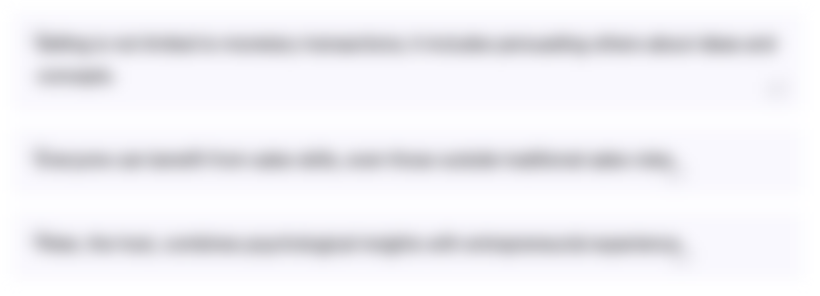
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
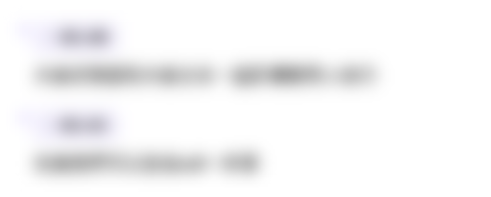
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
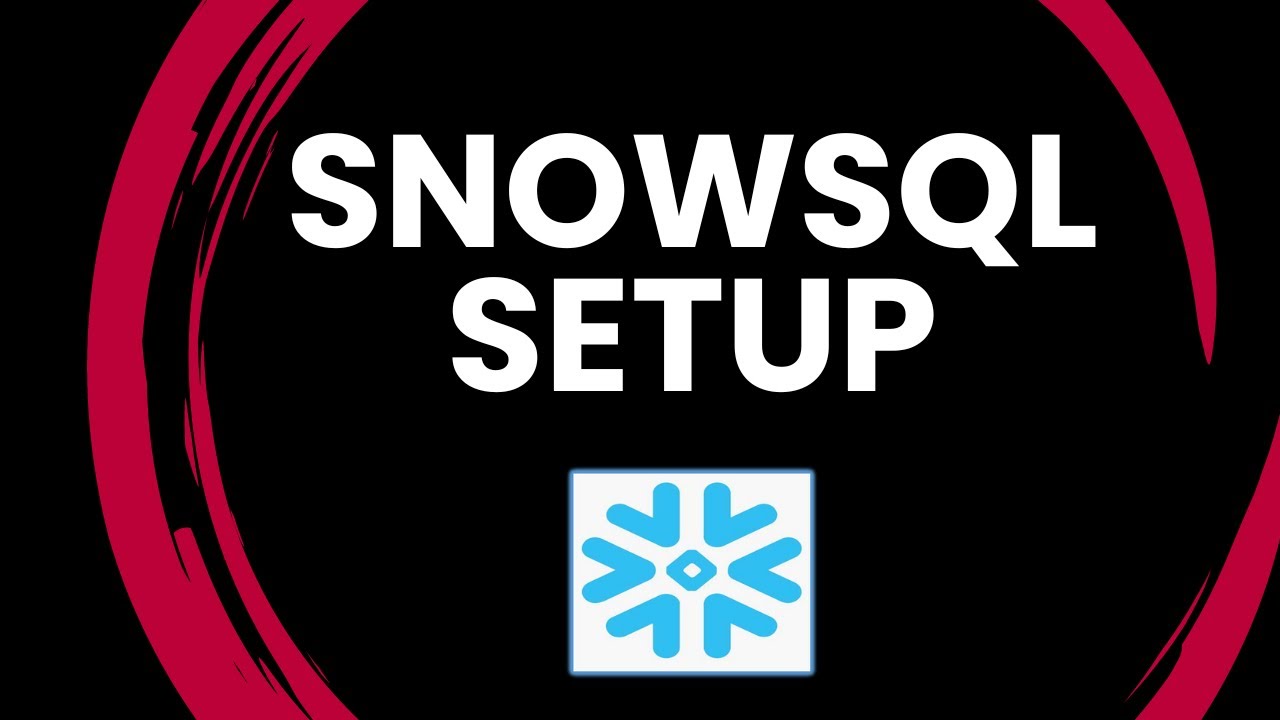
Snowsql Setup | How to login to snowsql | snowsql CLI
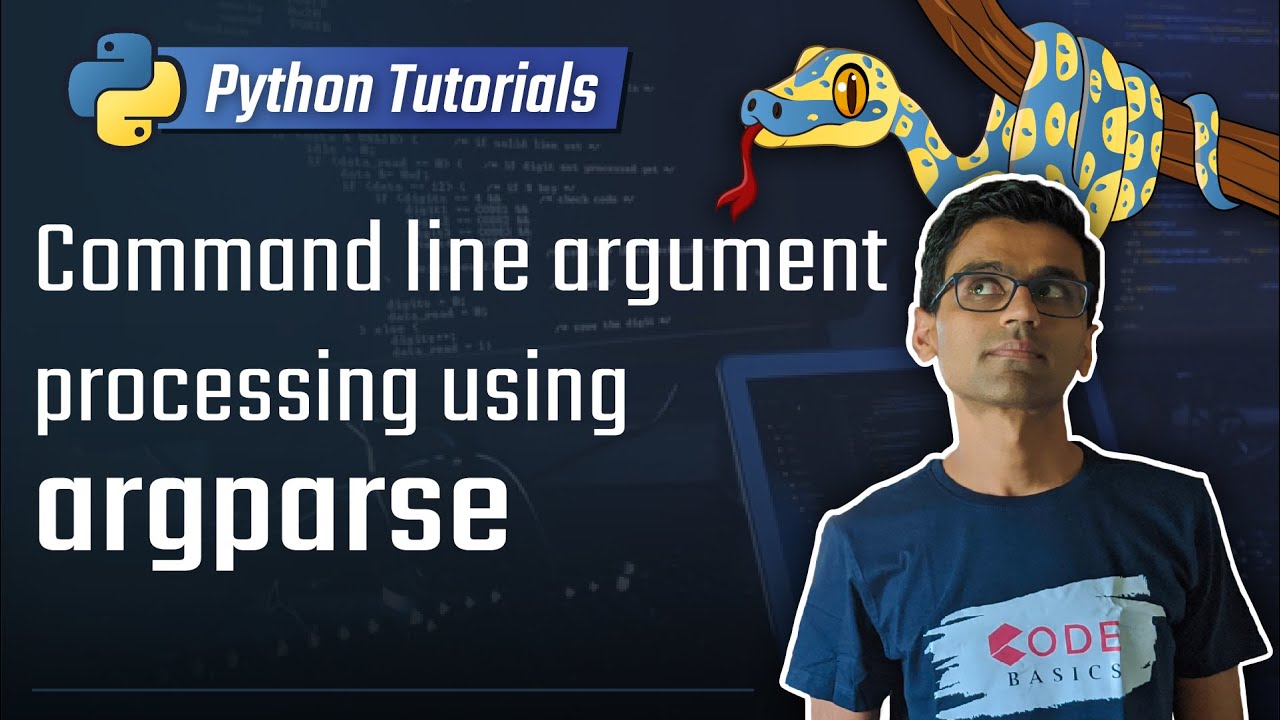
24. Command line argument processing using argparse [Python 3 Programming Tutorials]
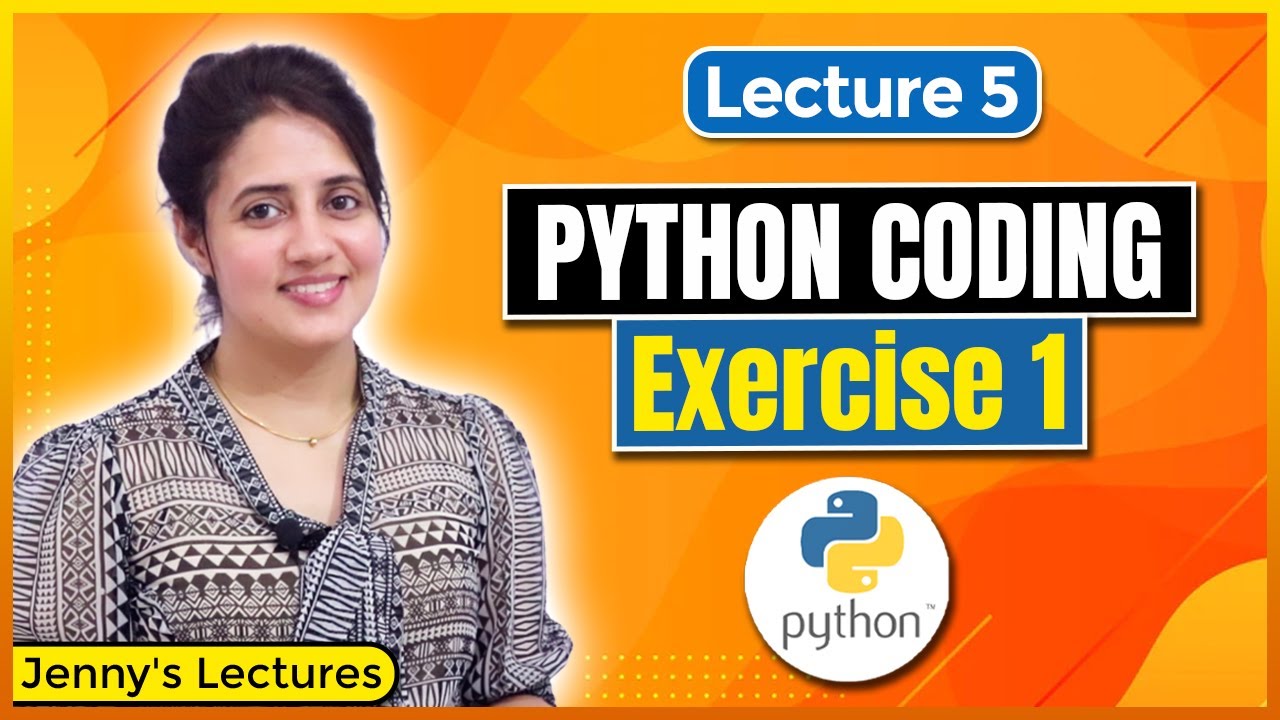
P_05 Coding Exercises for Beginners in Python - Exercise #1
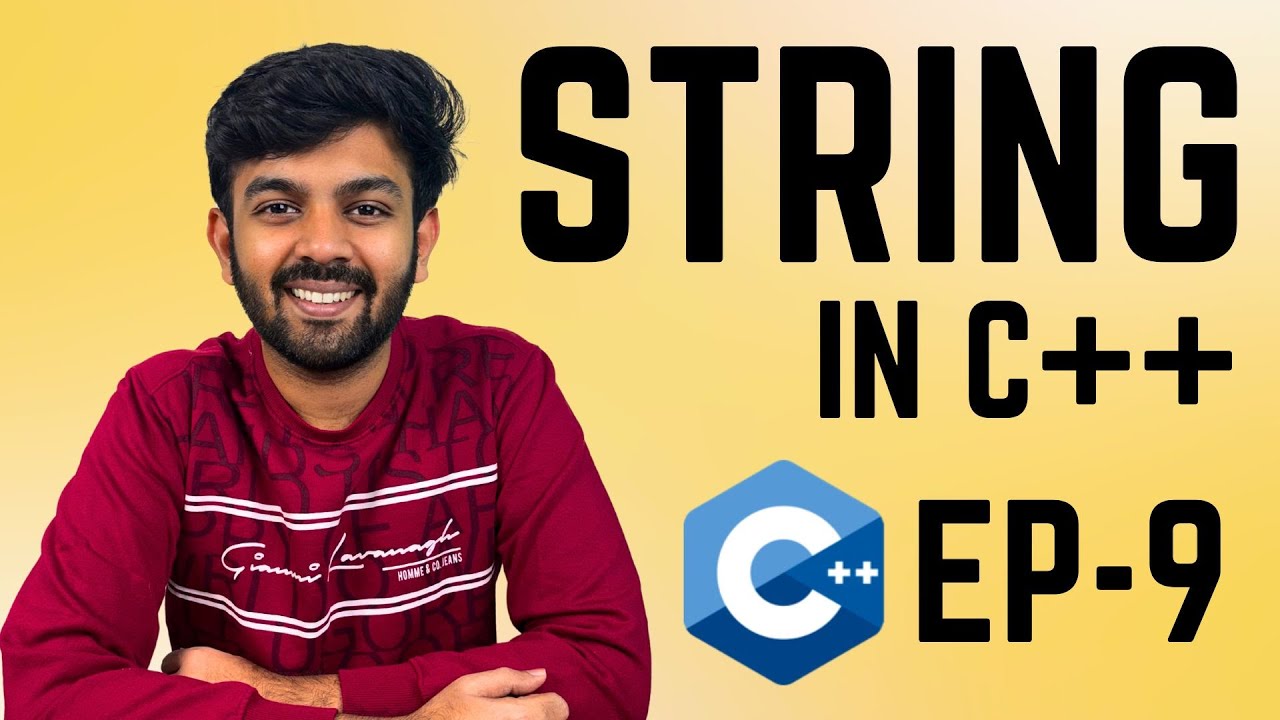
Strings | C++ for Beginners Ep-9 | code io - Tamil
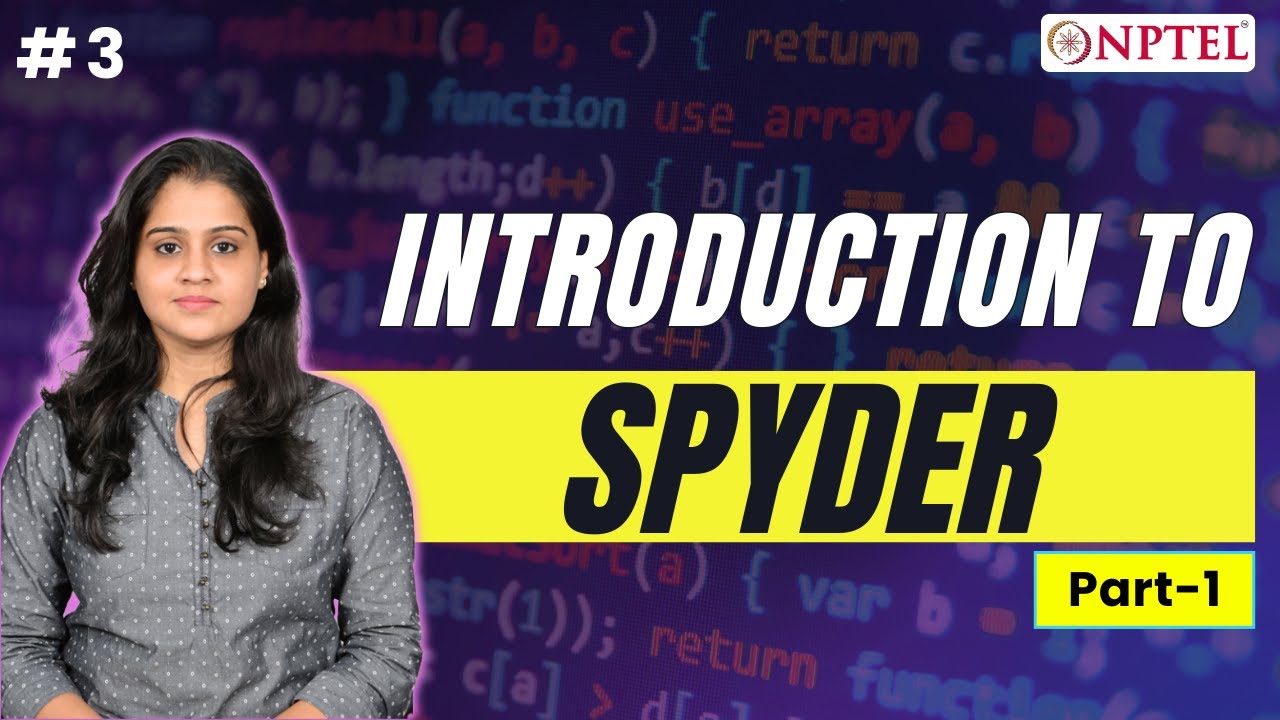
Introduction to Spyder - Part 1
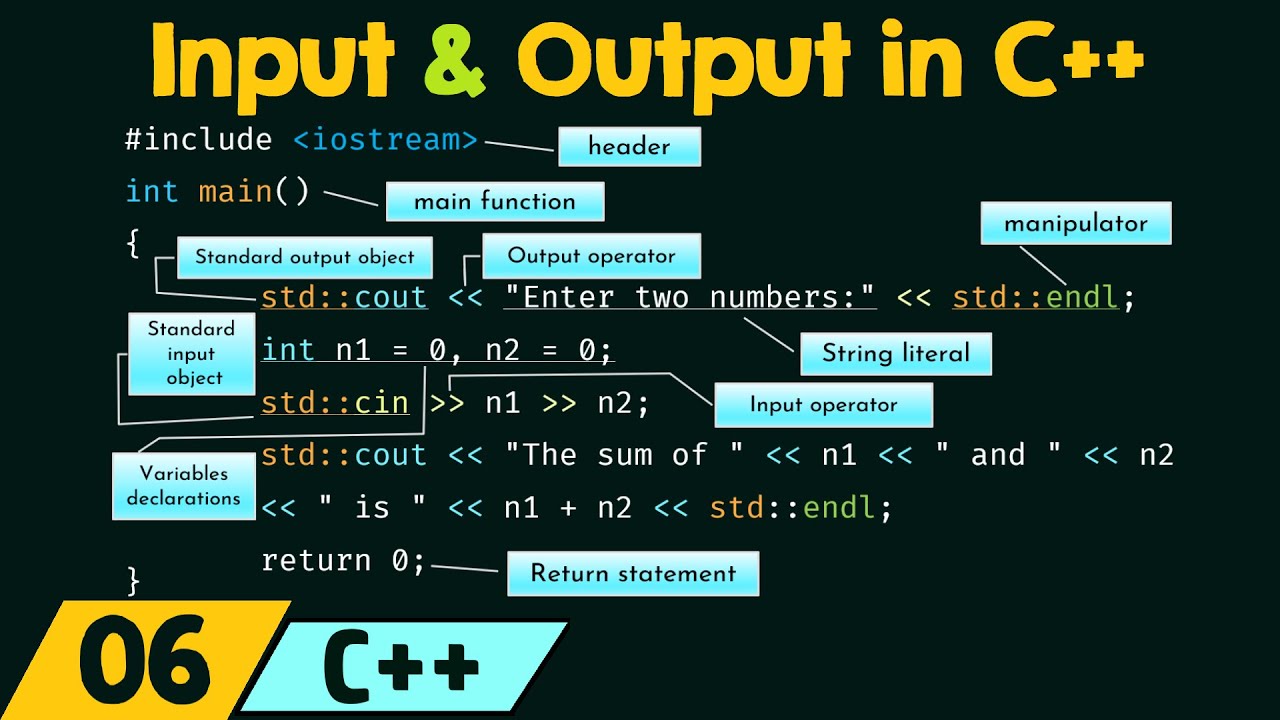
Input and Output in C++
5.0 / 5 (0 votes)