#9: If Else Statements in C | C Programming for Beginners
Summary
TLDRIn this educational C programming video, Padma from 'Programmies' introduces the concept of if-else statements, crucial for decision-making in code. The tutorial covers syntax, usage, and examples like checking voting eligibility based on age. It progresses to demonstrate else and else-if clauses for multiple conditions, enhancing logic with logical operators. The video concludes with a programming challenge to check if a number is positive, negative, or zero, encouraging viewers to apply their newfound knowledge.
Takeaways
- 📝 The video is a tutorial on 'if else' statements in C programming, focusing on decision-making in code.
- 🔍 It explains the syntax and usage of 'if' statements, which execute code based on a boolean condition being true or false.
- 👤 The tutorial uses a real-world example involving an election where the age of a person determines their eligibility to vote.
- 📈 The script demonstrates how to implement an 'if' statement to check if a person's age is 18 or above to print a message about eligibility.
- 📑 It shows the process of taking user input for the age variable and using 'printf' and 'scanf' statements in C.
- 🔄 The video covers the use of 'if' statements with 'else' clauses to handle scenarios where the condition is not met, like printing a message for ineligible voters.
- 🔧 The script introduces 'else if' clauses for handling multiple conditions, allowing the program to make decisions based on more than two options.
- 🔑 The tutorial provides a practical example of using 'else if' to check for invalid age values like being less than 0 or greater than 120.
- 💡 It offers a tip on simplifying 'if' and 'else' blocks by omitting curly braces when there's only one statement to execute.
- 📚 The video encourages viewers to practice creating test conditions and to review comparison and logical operators for building these conditions.
- 🎯 The script concludes with a programming challenge for viewers to create a program that checks if a number is positive, negative, or zero.
Q & A
What is the purpose of the 'if else' statement in C programming?
-The 'if else' statement in C programming is used for decision making, allowing a program to perform different sets of tasks based on whether a condition is true or false.
What is the basic syntax of an 'if' statement in C?
-The basic syntax of an 'if' statement in C is 'if (test condition)' followed by the body of the statement. The test condition is a boolean expression that evaluates to either true or false.
What happens if the test condition in an 'if' statement is true?
-If the test condition in an 'if' statement is true, the body of the 'if' statement is executed. If it is false, the body is skipped.
Can you provide an example of how to use an 'if' statement to check a person's eligibility to vote based on age?
-Yes, you can create a variable to store the person's age, ask the user to input their age using 'printf' and 'scanf', and then use an 'if' condition to check if the age is greater than or equal to 18. If true, print 'You are eligible to vote'.
What is the purpose of an 'else' clause in an 'if' statement?
-The 'else' clause in an 'if' statement is used to execute a different block of code when the test condition of the 'if' statement is false.
How can you modify the voting eligibility program to also handle cases where the age is less than 18?
-You can add an 'else' clause to the program that prints 'Sorry, you are not eligible to vote' when the age is less than 18.
What is the syntax for an 'if' statement with an 'else if' clause?
-The syntax for an 'if' statement with an 'else if' clause is 'if (test condition 1) { ... } else if (test condition 2) { ... }'. The program checks each condition sequentially and executes the block of code corresponding to the first true condition.
Why might you use 'else if' clauses instead of multiple 'if' statements?
-Using 'else if' clauses instead of multiple 'if' statements can make the code more efficient by avoiding repetitive checks of the same conditions and ensuring that only one block of code is executed.
Can you provide an example of using 'else if' clauses to handle multiple conditions in a program?
-Yes, in the voting eligibility program, you can use 'else if' clauses to check if the age is greater than 120 or less than 0, and print 'Invalid age' for both conditions.
What is the advantage of using logical operators in 'if else' statements?
-Using logical operators in 'if else' statements allows you to combine multiple conditions into a single test condition, making the code more concise and easier to read.
What is the final programming challenge presented in the video script?
-The final programming challenge is to create a program that checks whether a number is positive, negative, or zero using an 'if' statement based on user input.
How can you simplify an 'if else' block if it contains only one statement?
-If an 'if else' block contains only one statement, you can omit the curly braces, making the code less cluttered and easier to read.
Where can viewers find the answers to the programming challenge and additional resources for learning C programming?
-Viewers can find the answers to the programming challenge and additional resources on the GitHub repository mentioned in the video script, as well as on the website programmings.com.
Outlines
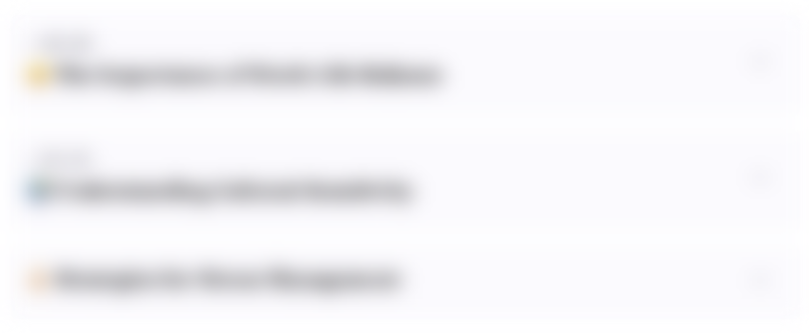
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
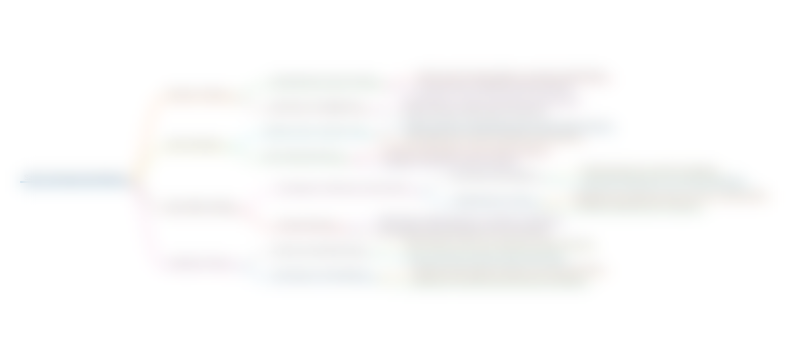
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
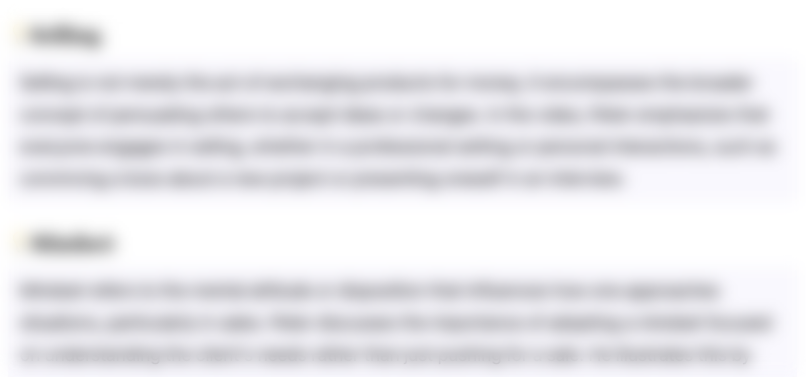
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
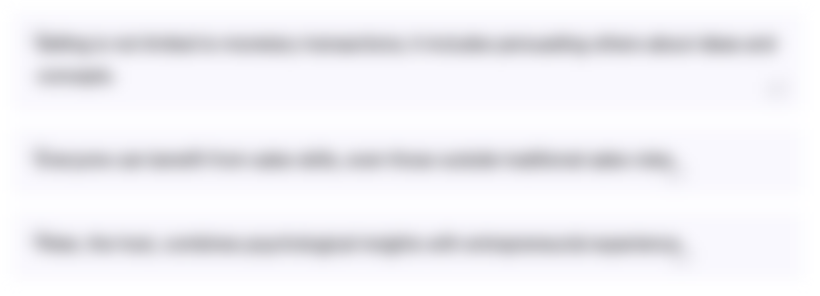
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
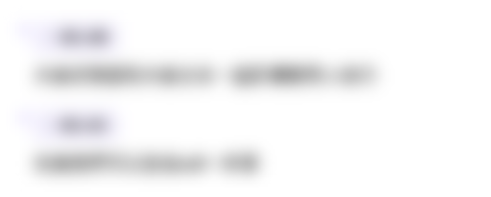
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
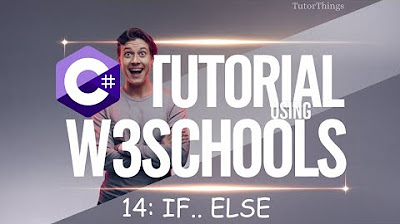
W3Schools | C# Full Course | W3Schools C# | C# Tutorial - Full Course for Beginners | C# Tutorial
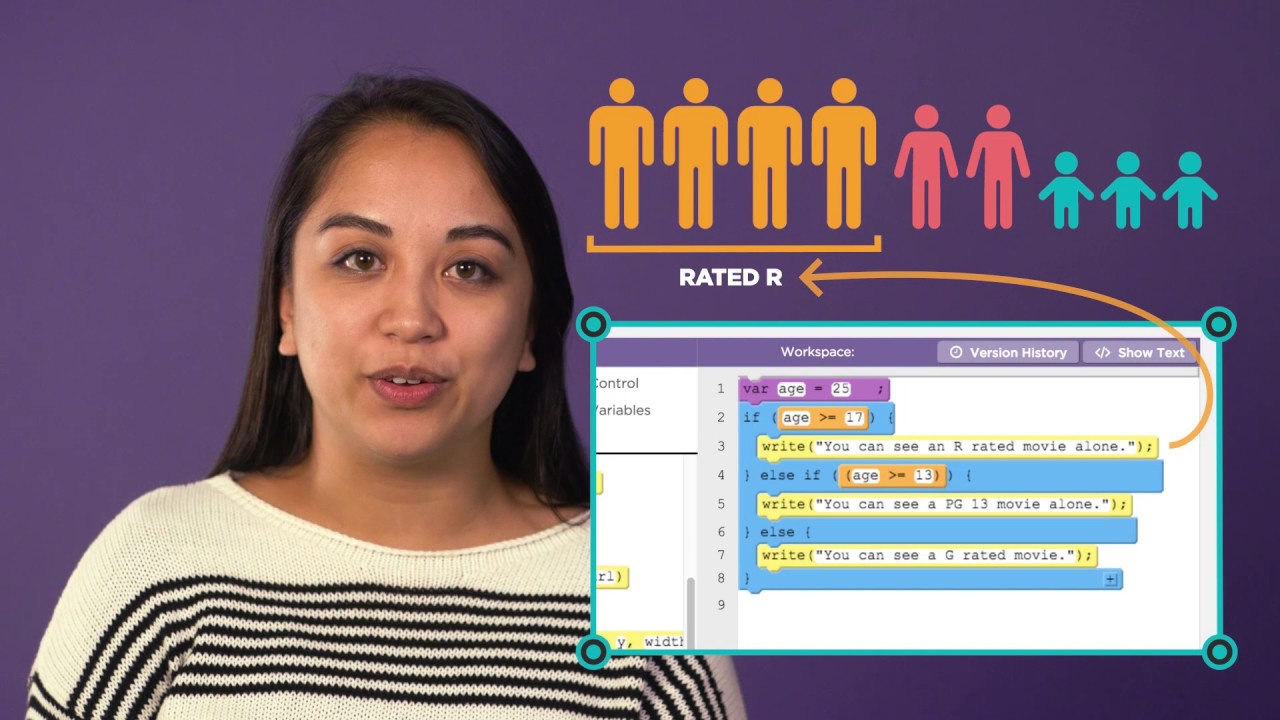
CSP: Conditionals pt 2C - if/else if statements
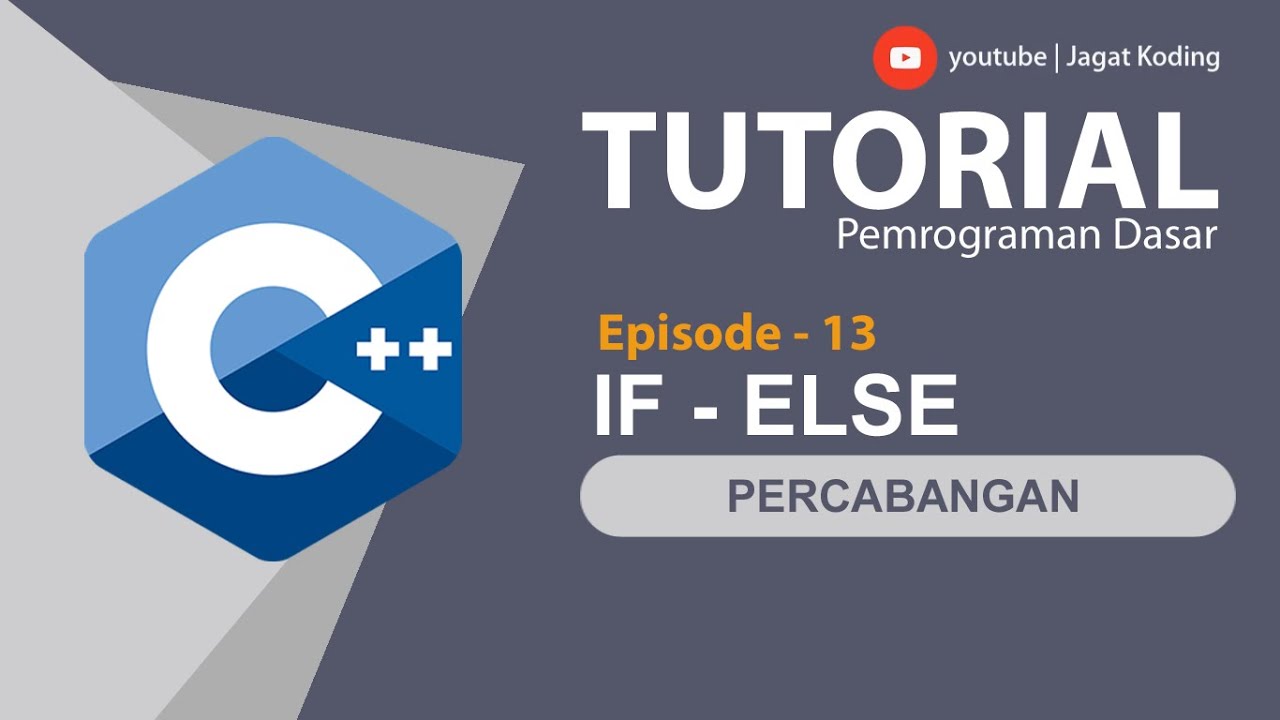
C++ 13 | If Else | Tutorial Percabangan C++

Svelte Tutorial - 9 - Conditional Rendering

Karel Python - if/else
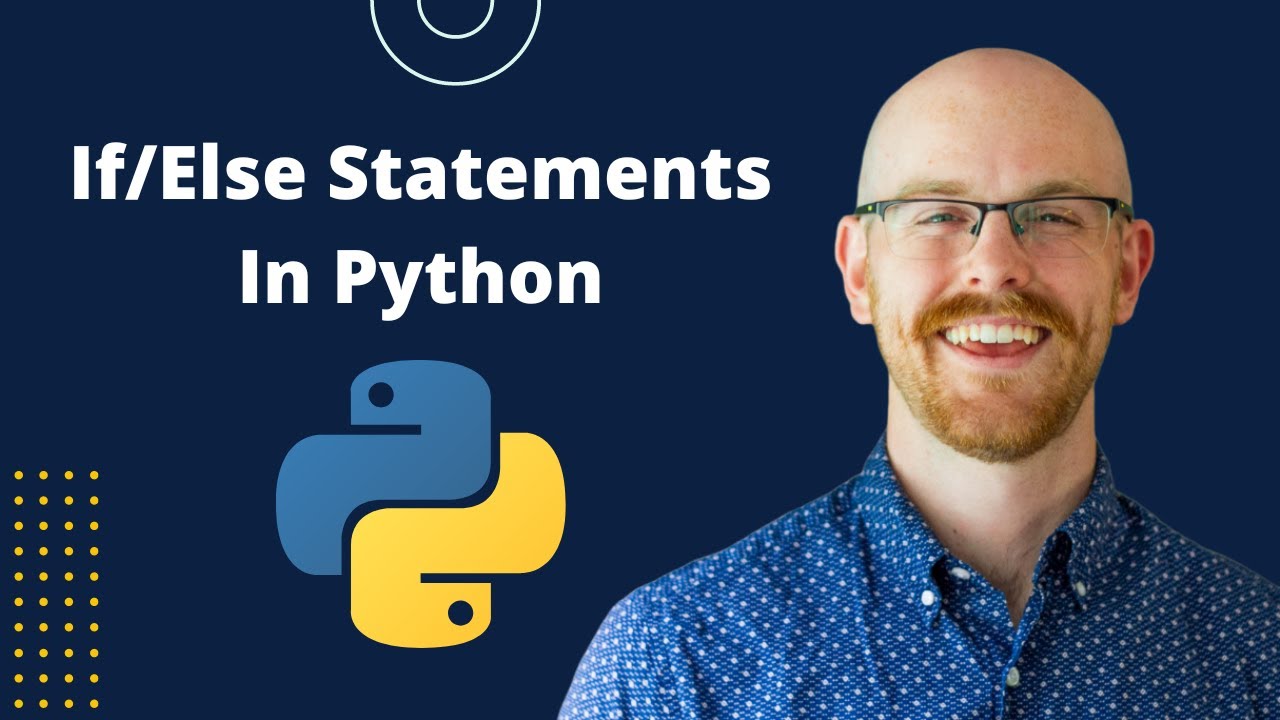
If Else Statements in Python | Python for Beginners
5.0 / 5 (0 votes)