ALL 47 STRING METHODS IN PYTHON EXPLAINED
Summary
TLDRThis video script offers an extensive tutorial on Python's string methods, covering a total of 47 functions excluding dunder methods. It begins with common methods like 'capitalize' and 'upper', then delves into lesser-known functionalities. Each method is explained with examples, from simple case manipulations to complex string encoding and pattern searches. The script also clarifies the differences between methods that may seem similar, such as 'isdecimal', 'isdigit', and 'isnumeric'. The tutorial is rounded off with methods for string formatting, partitioning, and justification, providing a comprehensive guide for anyone looking to master string manipulation in Python.
Takeaways
- 📝 The lesson covers 47 string methods in Python, excluding the dunder methods, providing a comprehensive overview of string manipulation capabilities.
- 🔄 'capitalize' and 'casefold' methods are used to transform strings to start with a capital letter and to enable caseless comparisons, respectively.
- 📏 'center' allows for centering a string within a given width, optionally padded with a specific character.
- 🔢 'count' is utilized to determine the number of occurrences of a substring within a string.
- 🔠 'encode' converts a string into a byte representation, with options to handle encoding errors.
- 🔚 'endswith' checks if a string concludes with a specified character or tuple of characters.
- 📖 'expandtabs' is used to convert tab characters into spaces, based on a defined tab width.
- 🔍 'find' and 'index' locate the position of a substring within a string, with 'index' raising an error if the substring is not found.
- 📐 'format' and 'format_map' offer ways to insert values into a string with placeholders and dictionary mappings, respectively.
- 🔤 'isalnum', 'isalpha', 'isdigit', 'isdecimal', and 'isnumeric' are used to check for strings containing specific types of characters, with 'isalnum' being the broadest check.
- 🛑 'index' is a method that, unlike 'find', will throw an error if the specified substring is not present in the string.
- 🔗 'join' is used to concatenate elements of an iterable with a specified string as a separator.
- 🔄 'lower' and 'upper' convert strings to lowercase and uppercase, respectively, while 'swapcase' swaps the case of each character.
Q & A
What does the 'capitalize' method do in Python?
-The 'capitalize' method converts the first character of a string to uppercase and the rest to lowercase.
How does the 'casefold' method help in string comparison?
-The 'casefold' method returns a case-insensitive version of the string, which helps in comparing strings without worrying about case differences.
What is the purpose of the 'center' method?
-The 'center' method centers the string in a field of a specified width, optionally filling the surrounding space with a specified character.
How does the 'count' method work?
-The 'count' method returns the number of non-overlapping occurrences of a substring in the string.
What is the function of the 'encode' method?
-The 'encode' method encodes the string using the specified encoding and returns the encoded bytes object.
How can you check if a string ends with a specific suffix using Python?
-You can use the 'endswith' method to check if a string ends with a specified suffix or any of a tuple of suffixes.
What does the 'expandtabs' method do?
-The 'expandtabs' method replaces tabs in the string with spaces, using a specified tab size.
How do the 'find' and 'index' methods differ?
-Both methods return the lowest index of the substring if found, but 'find' returns -1 if the substring is not found, while 'index' raises a ValueError.
What is the use of the 'format' and 'format_map' methods?
-The 'format' method allows you to format strings using placeholders, while 'format_map' formats strings using a dictionary for the placeholders.
How do the 'isdecimal', 'isdigit', and 'isnumeric' methods differ?
-The 'isdecimal' method checks if all characters are decimal characters, 'isdigit' checks if all characters are digits, and 'isnumeric' checks if all characters are numeric, including digits, decimals, and other numeric characters.
Outlines
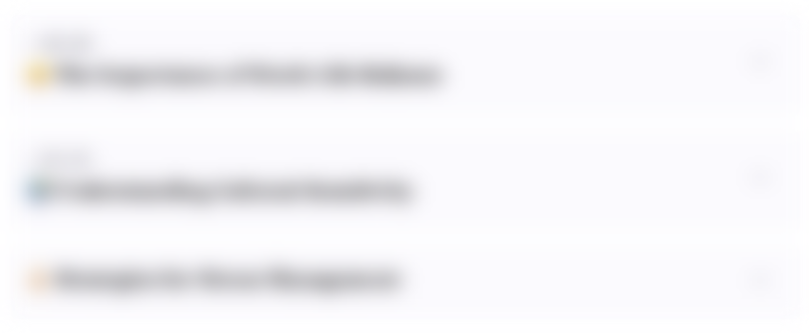
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
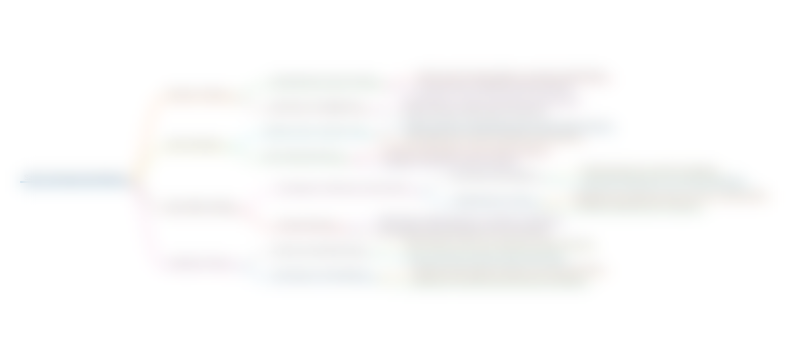
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
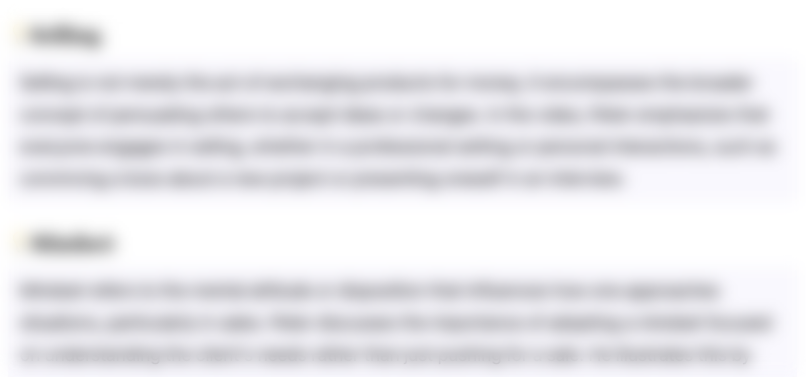
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
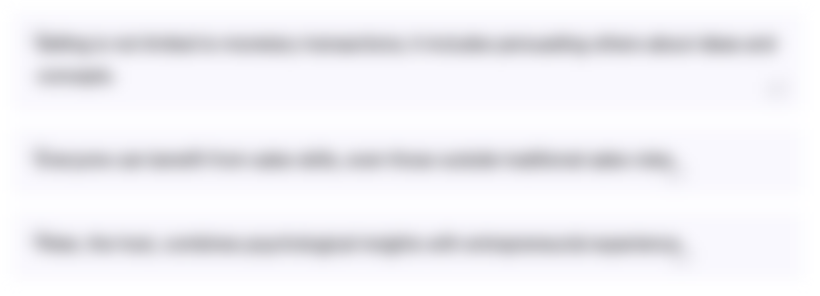
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
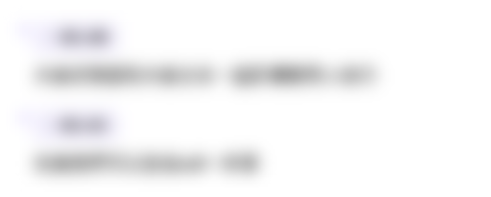
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
5.0 / 5 (0 votes)