Unity Endless Tutorial • 1 • Player Character [Tutorial][C#]
Summary
TLDRIn this tutorial, the instructor demonstrates how to create the core mechanics for an endless runner game in Unity. Starting with a basic scene setup, they guide viewers through creating a player using a capsule object, replacing its collider with a character controller, and writing a script to move the player forward. The movement is made frame-rate independent using `Time.deltaTime`, and a speed variable is introduced to control player velocity. The player is then saved as a prefab for reuse. Future episodes will cover additional mechanics like player movement control and gravity effects.
Takeaways
- 😀 Create a new scene and save it immediately to keep your project organized.
- 😀 Add a 'Scenes' folder to store all your scene files for better project management.
- 😀 Start by creating a simple player object (a capsule) and reset its position to the origin (0, 0, 0).
- 😀 Change the name of the capsule to 'Player' and assign it a 'Player' tag for easy identification in the project.
- 😀 Replace the default capsule collider with a 'CharacterController' to handle player movement and collisions more effectively.
- 😀 Create a custom C# script, 'PlayerMotor', to manage the player’s forward movement using the 'CharacterController'.
- 😀 Use the 'CharacterController.Move' function in the 'Update' method to move the player forward every frame.
- 😀 Adjust movement speed by multiplying the motion vector by a speed variable and 'Time.deltaTime' for frame rate-independent movement.
- 😀 Use the Unity Inspector to tweak the speed value for smoother gameplay (initial speed set to 5).
- 😀 Replace the capsule with a custom 3D model (either from the Asset Store or your own assets) for a more polished player character.
- 😀 Save the player object as a prefab for easy reuse and organization in your project, creating a 'Prefabs' folder to store all prefabs.
Q & A
What is the purpose of the `CharacterController` component in Unity?
-The `CharacterController` component allows an object to move and interact with the environment through physics-based collision detection, without using traditional physics (like Rigidbody). It also provides built-in functions for character movement, such as `Move()`.
Why does the script multiply the movement vector by `Time.deltaTime`?
-Multiplying by `Time.deltaTime` ensures that the movement is frame-rate independent. This means the player moves the same distance per second, regardless of how many frames are rendered per second (FPS).
How does the `PlayerMotor` script allow the player to move forward?
-The script uses the `CharacterController.Move()` method to move the player in the forward direction (`Vector3.forward`). This movement is multiplied by a speed factor and adjusted by `Time.deltaTime` to ensure consistent speed across different frame rates.
What is the purpose of using `Vector3.forward` in the movement calculation?
-`Vector3.forward` is a predefined unit vector (0, 0, 1) that points in the positive Z-axis direction. It represents forward movement in Unity’s 3D space, ensuring the player always moves in that direction.
What happens if you don't multiply the movement by `Time.deltaTime`?
-Without multiplying by `Time.deltaTime`, the movement would be tied to the frame rate. As a result, on systems with higher frame rates, the player would move faster, while on systems with lower frame rates, the player would move slower.
How does the script ensure the player moves at a consistent speed?
-By using a `speed` variable (e.g., 5 units per second) and multiplying it by `Time.deltaTime`, the script ensures the player moves at a constant speed, regardless of the frame rate.
What is the significance of creating a prefab for the player object?
-Creating a prefab allows you to save the player object with all its components and settings as a reusable asset. It makes it easier to manage and update the player object across the game, as changes to the prefab are reflected everywhere it's used.
What changes are made when replacing the capsule with a custom character model?
-When replacing the capsule with a custom character model, you need to ensure the new model has the necessary components (like `CharacterController` and `Animator`) for movement and animation. Other components, such as Rigidbody, may be removed if they are not needed.
Why is it important to set the tag of the player object to 'Player'?
-Setting the tag to 'Player' helps to identify and differentiate the player object from other objects in the scene, making it easier to reference and manage in scripts, such as for player-specific actions or interactions.
What are the next steps in the tutorial after completing the player setup?
-The next steps involve implementing movement along the X-axis (left and right) and adding gravity to the player to create a more dynamic and realistic gameplay experience.
Outlines
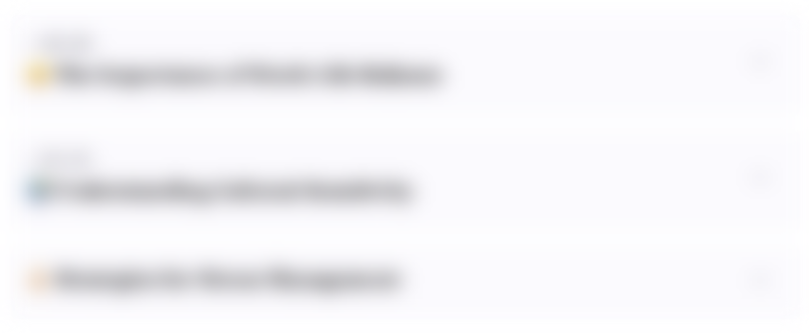
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
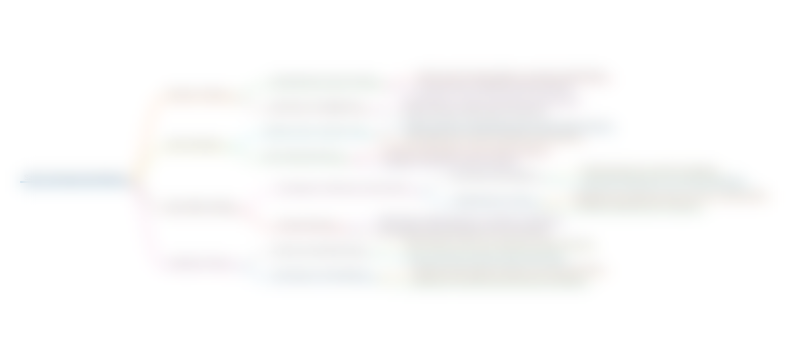
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
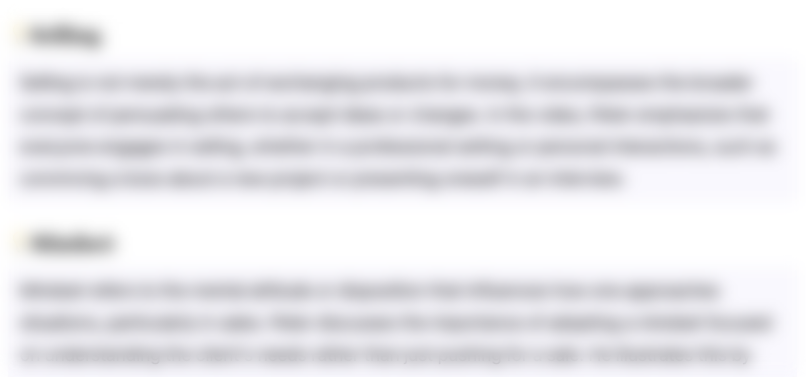
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
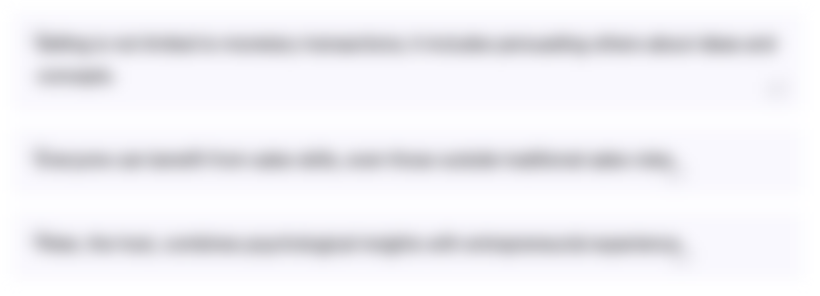
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
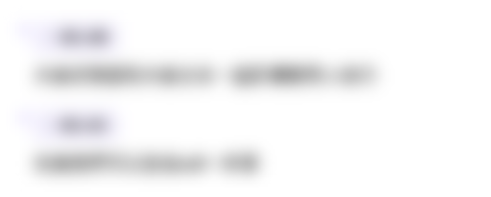
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
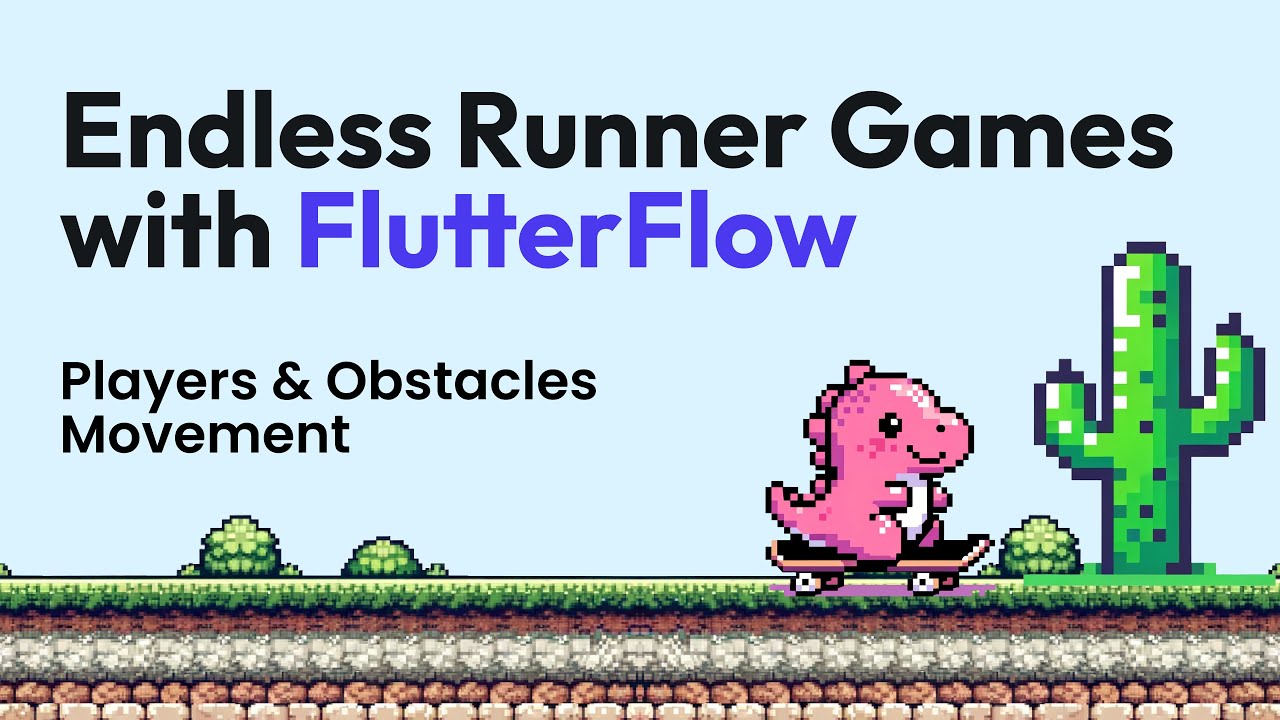
Endless Runner Games with FlutterFlow: Players & Obstacles Movement
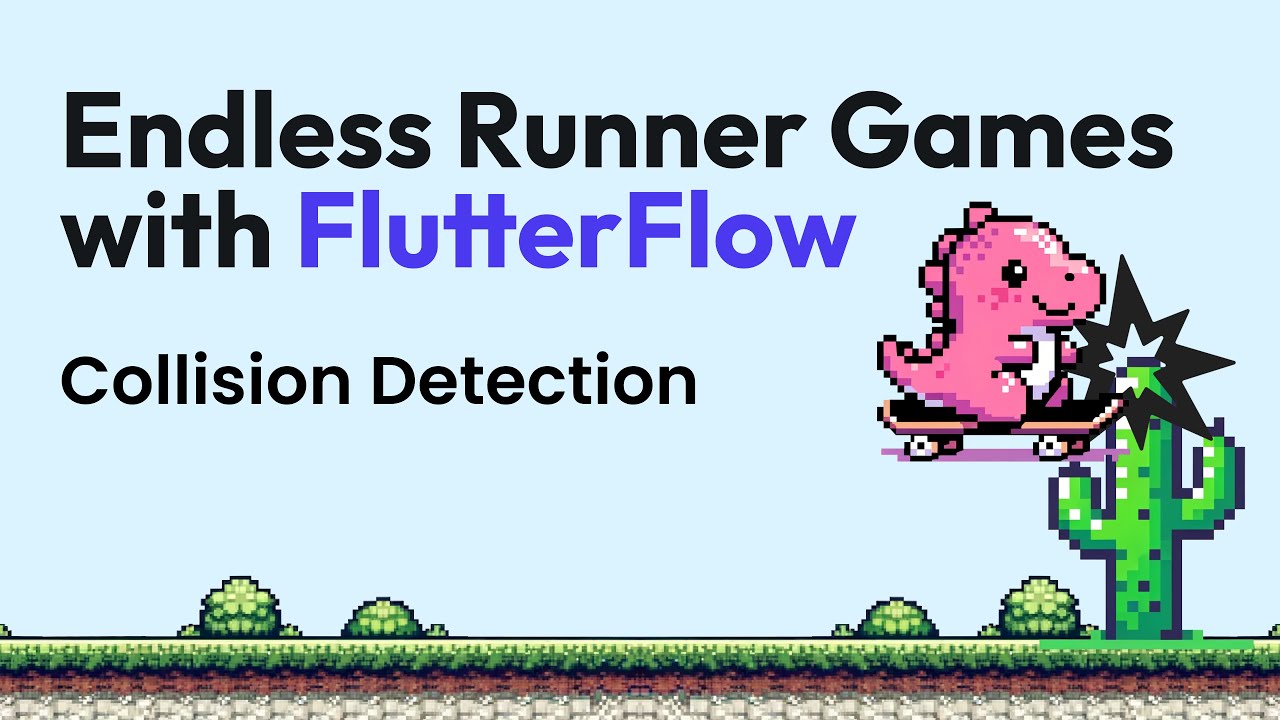
Endless Runner Games with FlutterFlow: Collision Detection & Moving Backgrounds
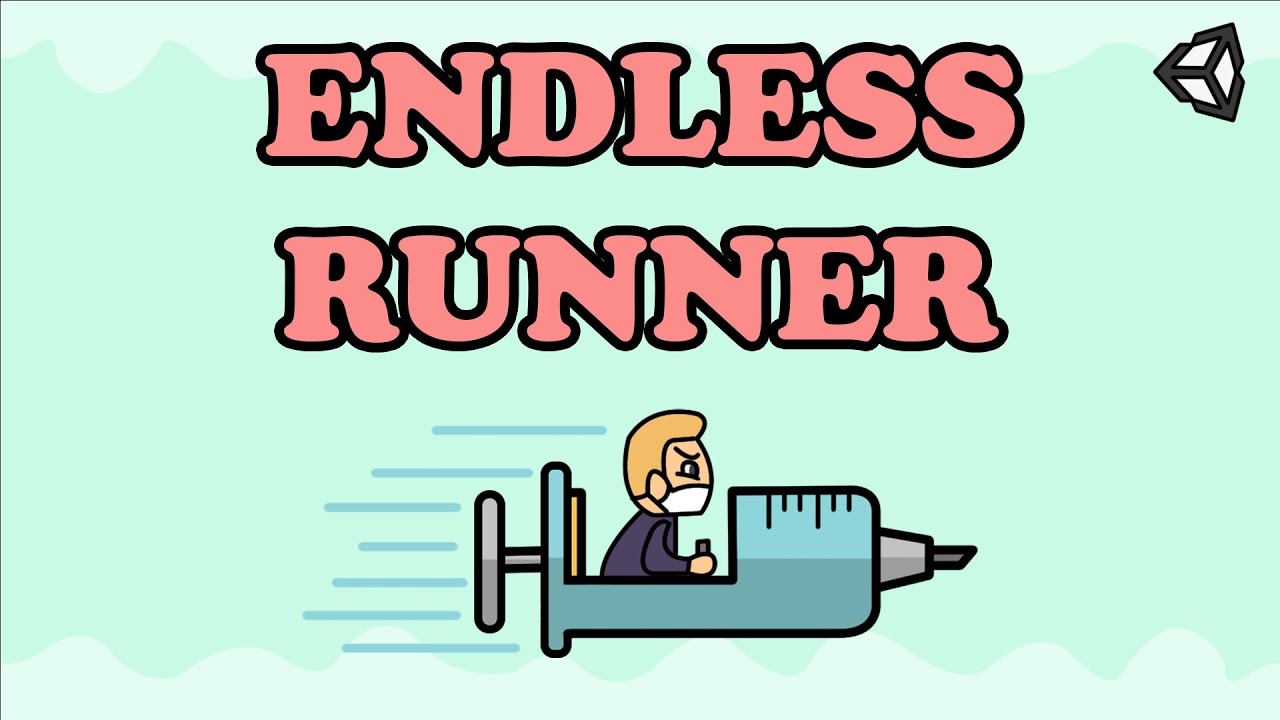
How To Make A 2D Endless Runner For Beginners - Easy Unity Tutorial
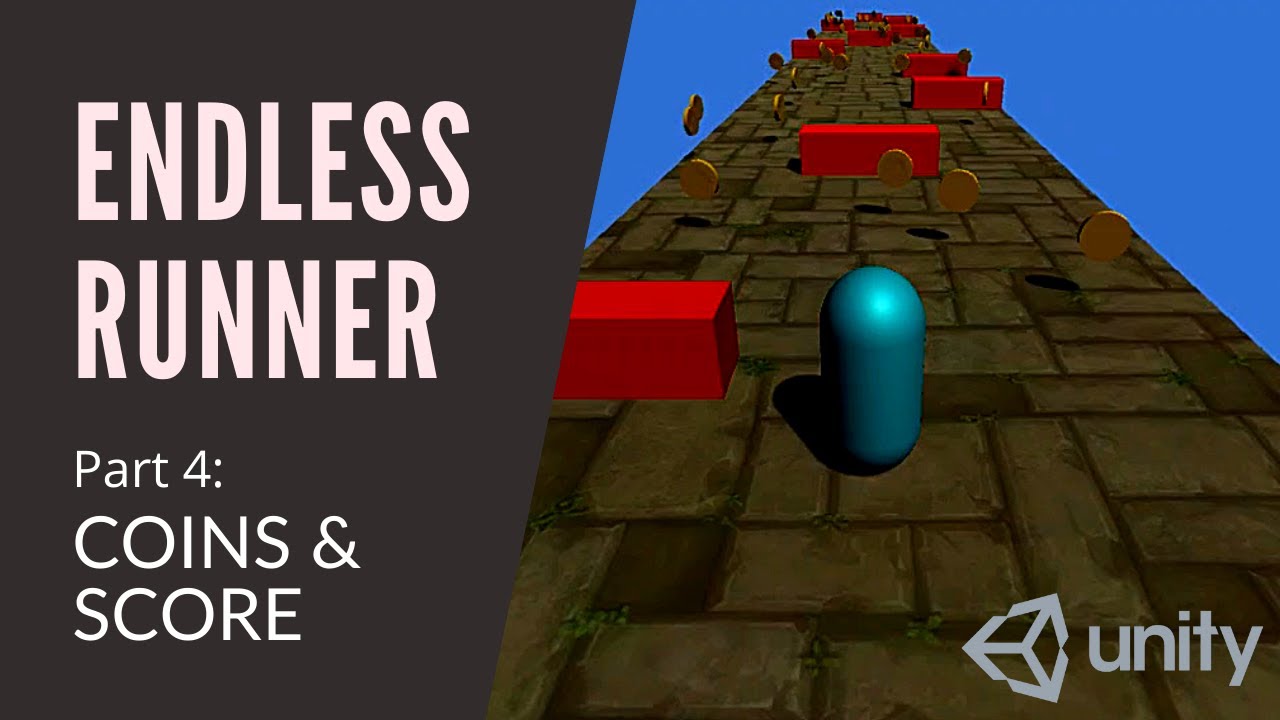
3D ENDLESS RUNNER IN UNITY - COINS (Pt 4)
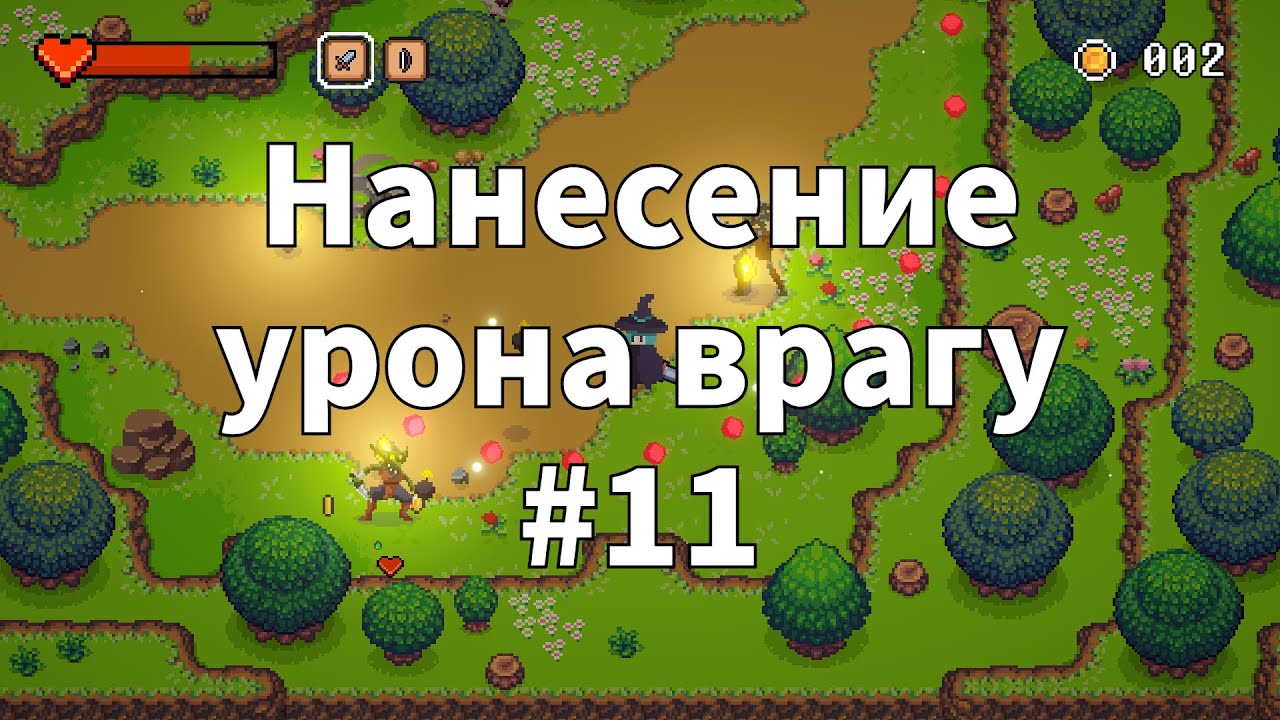
2D Top Down игра на Unity с нуля #11 | Коллайдер для меча, нанесение урона врагу

#32 Custom Route Constraint | Understanding Routing | ASP.NET Core MVC Course
5.0 / 5 (0 votes)