C++ 11 | Operator Assignment | Tutorial Pemrograman C++
Summary
TLDRIn this tutorial, the concept of assignment operators in C++ is explained, with a focus on how they are used to assign values to variables and simplify arithmetic operations. The video covers the basics of the assignment operator (`=`) and its shorthand counterparts (`+=`, `-=`, `*=`, `/=`, `%=`). Practical examples demonstrate how these operators work, such as adding, subtracting, multiplying, dividing, and finding remainders. The tutorial is designed for beginners, aiming to simplify C++ coding by showing how to use these operators effectively for more efficient and readable code.
Takeaways
- 😀 The assignment operator (`=`) in C++ is used to assign values to variables, effectively 'giving a task' to the variable.
- 😀 The shorthand assignment operators like `+=`, `-=`, `*=`, `/=`, and `%=` allow you to combine assignment with arithmetic operations in one step.
- 😀 Using `+=`, you can add a value to a variable and assign the result back to the same variable, e.g., `bill += 5` is equivalent to `bill = bill + 5`.
- 😀 The assignment operator simplifies code by reducing repetition and improving readability.
- 😀 The operator `-=` subtracts a value from a variable and assigns the result to the same variable, e.g., `bill -= 5`.
- 😀 The operator `*=` multiplies a variable by a value and assigns the result, e.g., `bill *= 5`.
- 😀 The operator `/=` divides a variable by a value and assigns the result, e.g., `bill /= 5`.
- 😀 The modulus assignment operator (`%=`) assigns the remainder of a division to the variable, e.g., `bill %= 5` gives the remainder of `bill / 5`.
- 😀 Using shorthand operators makes code shorter and easier to maintain, especially when performing repetitive operations on variables.
- 😀 The script emphasizes that understanding and using the assignment operator effectively can help in writing more efficient and readable code.
Q & A
What is an assignment operator in C++?
-An assignment operator in C++ is used to assign a value to a variable. The most basic assignment operator is '=', which sets a variable's value, such as 'int x = 10;'
How does the shorthand assignment operator '+= ' work in C++?
-'+=' is a shorthand operator that combines the assignment and addition operations. For example, 'x += 5;' is equivalent to 'x = x + 5;', which adds 5 to the current value of x.
What is the difference between 'bil = 10' and 'bil += 5'?
-'bil = 10' assigns the value 10 to the variable 'bil', while 'bil += 5' adds 5 to the existing value of 'bil'. For example, if 'bil' starts at 10, after 'bil += 5', its new value will be 15.
Can you use other arithmetic operators with the assignment operator?
-Yes, C++ supports shorthand operators for various arithmetic operations, such as '-=' for subtraction, '*=' for multiplication, '/=' for division, and '%=' for modulus (remainder of division). For example, 'x -= 5;' subtracts 5 from 'x'.
What does the 'modulus' operator '%=' do?
-The modulus operator '%=' calculates the remainder of the division of the left-hand side by the right-hand side. For example, 'x %= 5;' will give the remainder when 'x' is divided by 5.
What is the advantage of using shorthand operators like '+=', '-=' in C++?
-Shorthand operators like '+=', '-=' make the code more concise and easier to read. They allow you to perform an operation on a variable and assign the result back to that variable in a single step, reducing redundancy.
What happens when you use the assignment operator '= ' in C++?
-The assignment operator '=' assigns the value on the right-hand side to the variable on the left. For example, 'x = 10;' sets the value of the variable 'x' to 10.
What is the purpose of the 'using namespace std;' in the C++ code?
-'using namespace std;' allows you to use standard C++ library functions like 'cout' and 'cin' without needing to prefix them with 'std::'. This makes the code cleaner and easier to write.
Can you explain the sequence of operations when using multiple assignment operators in a single statement?
-In C++, operators like '+=', '-=', '*=', '/=' are applied in the order they appear, affecting the value of the variable directly. For example, if 'bil' starts at 10, 'bil += 5;' changes its value to 15. If followed by 'bil *= 2;', 'bil' would then become 30.
Why might you prefer to use 'bil += 5' instead of 'bil = bil + 5'?
-Using 'bil += 5' is preferred because it's more concise and easier to read. It reduces redundancy and avoids repeating the variable name, making the code cleaner, especially for larger operations.
Outlines
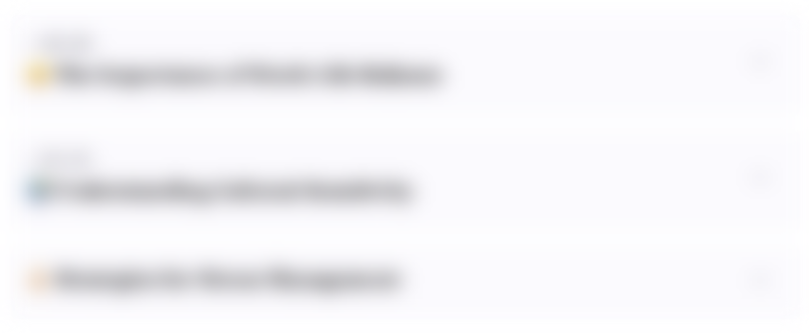
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
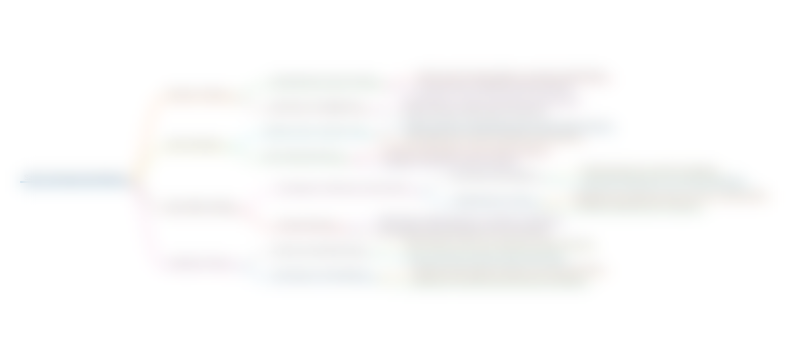
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
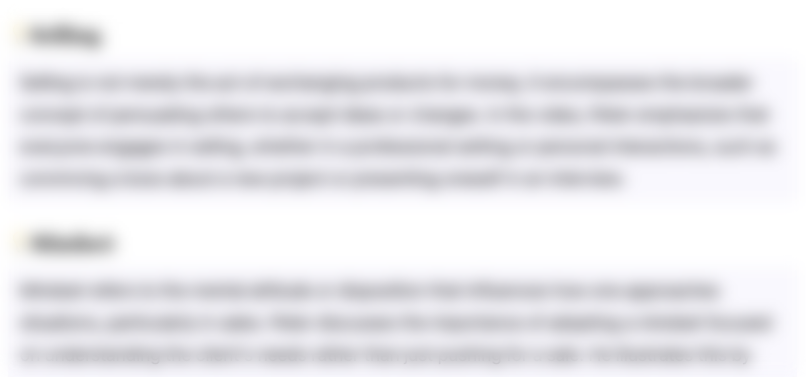
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
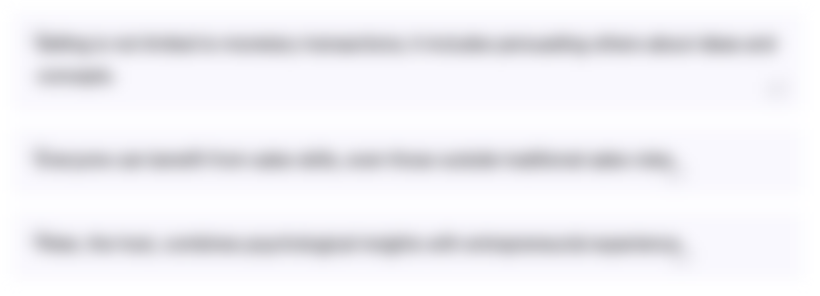
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
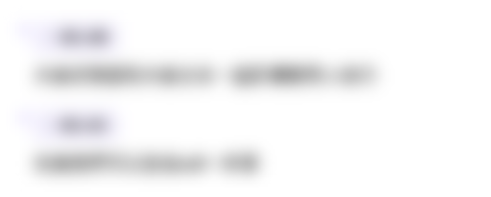
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
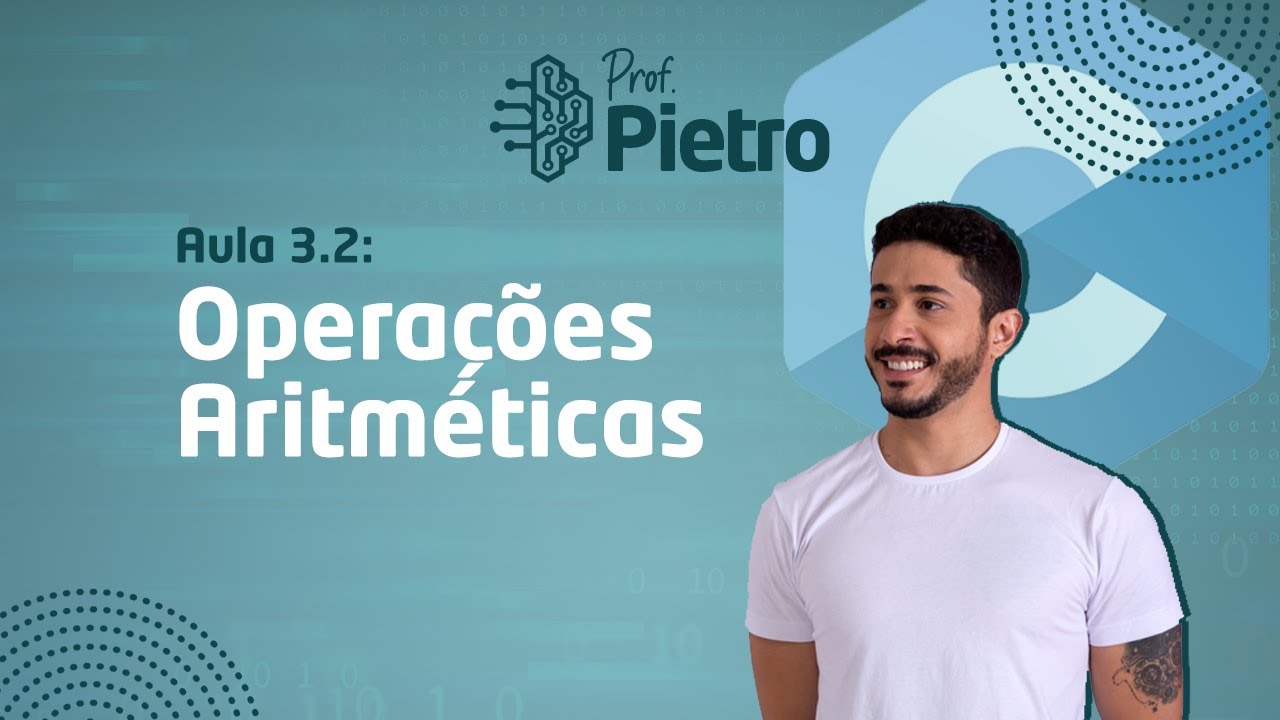
Linguagem C - Aula 3.2 - Aprenda a realizar cálculos em C (2022)
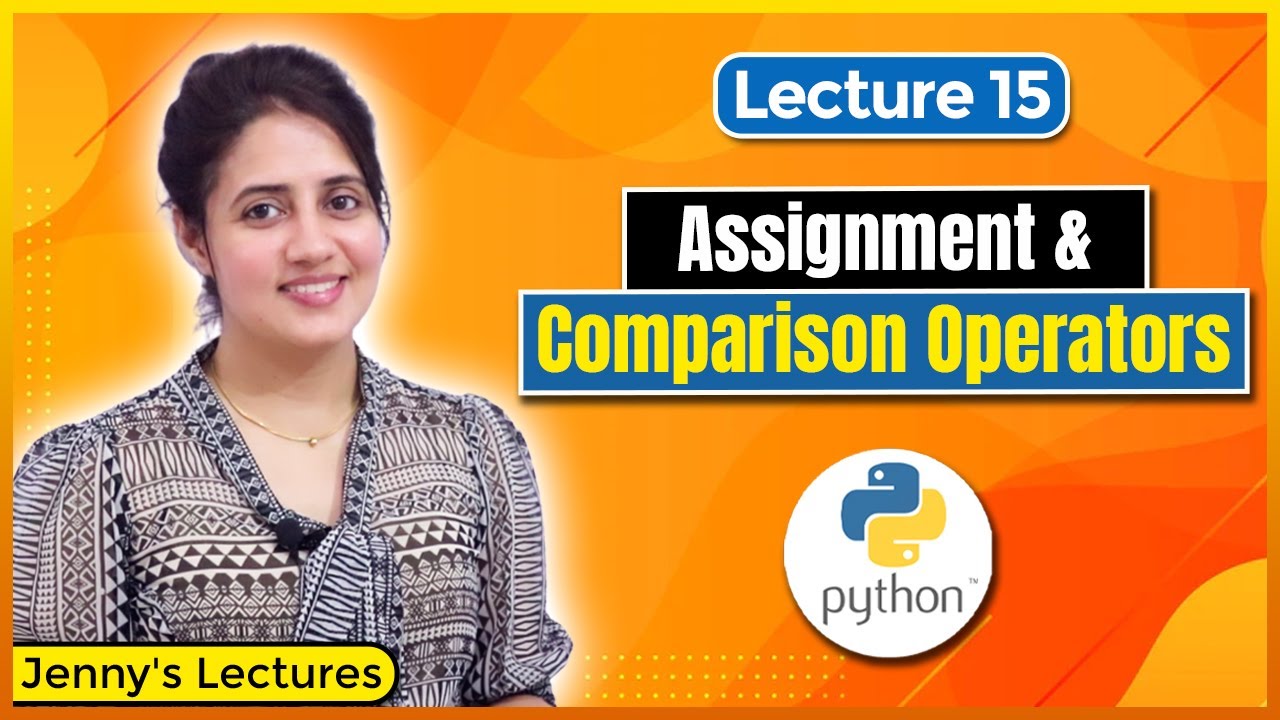
P_15 Operators in Python | Assignment and Comparison Operators | Python Tutorials for Beginners

#11 Python Tutorial for Beginners | Operators in Python
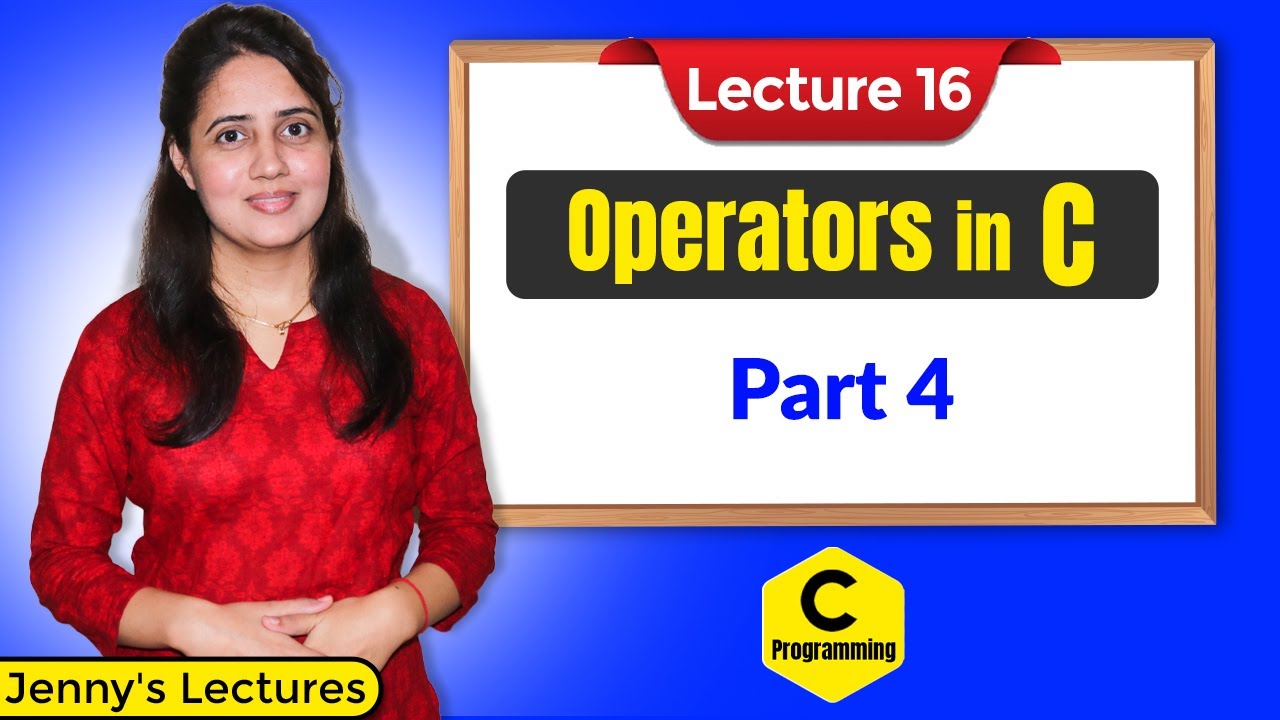
C_16 Operators in C - Part 4 | C Programming Tutorials
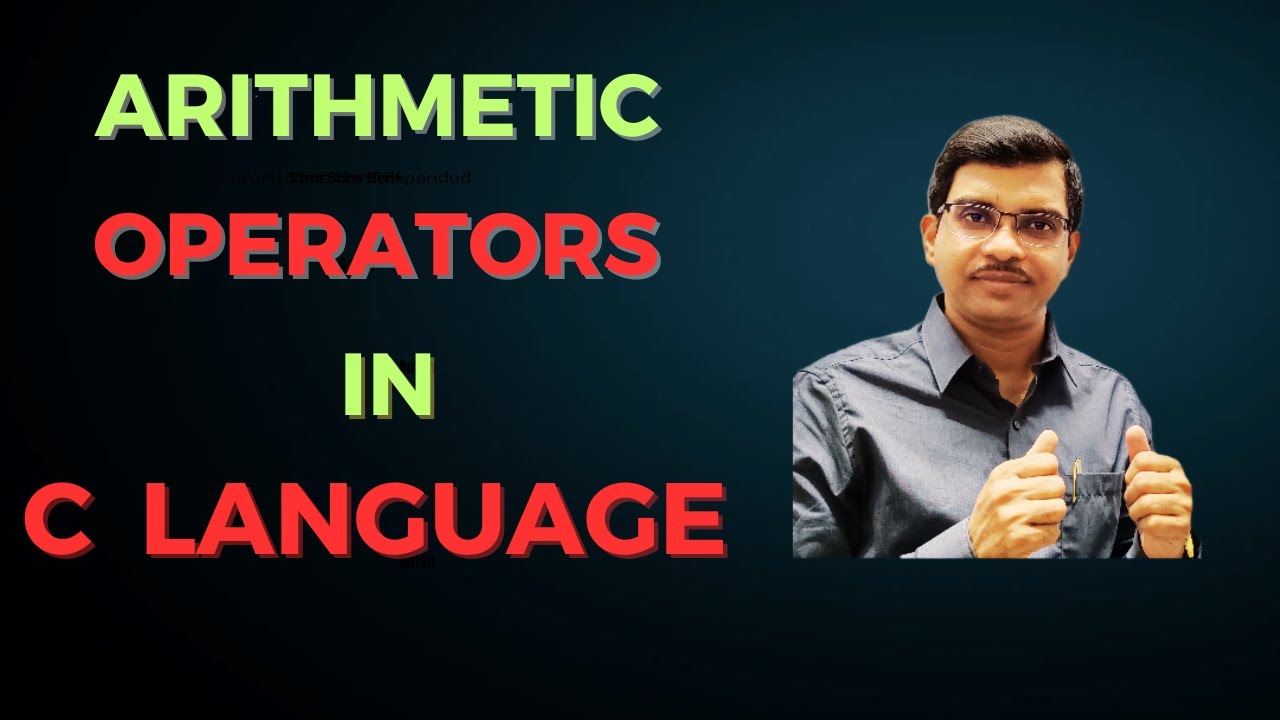
C_12 Arithmetic Operators in C Language | C Programming Tutorials
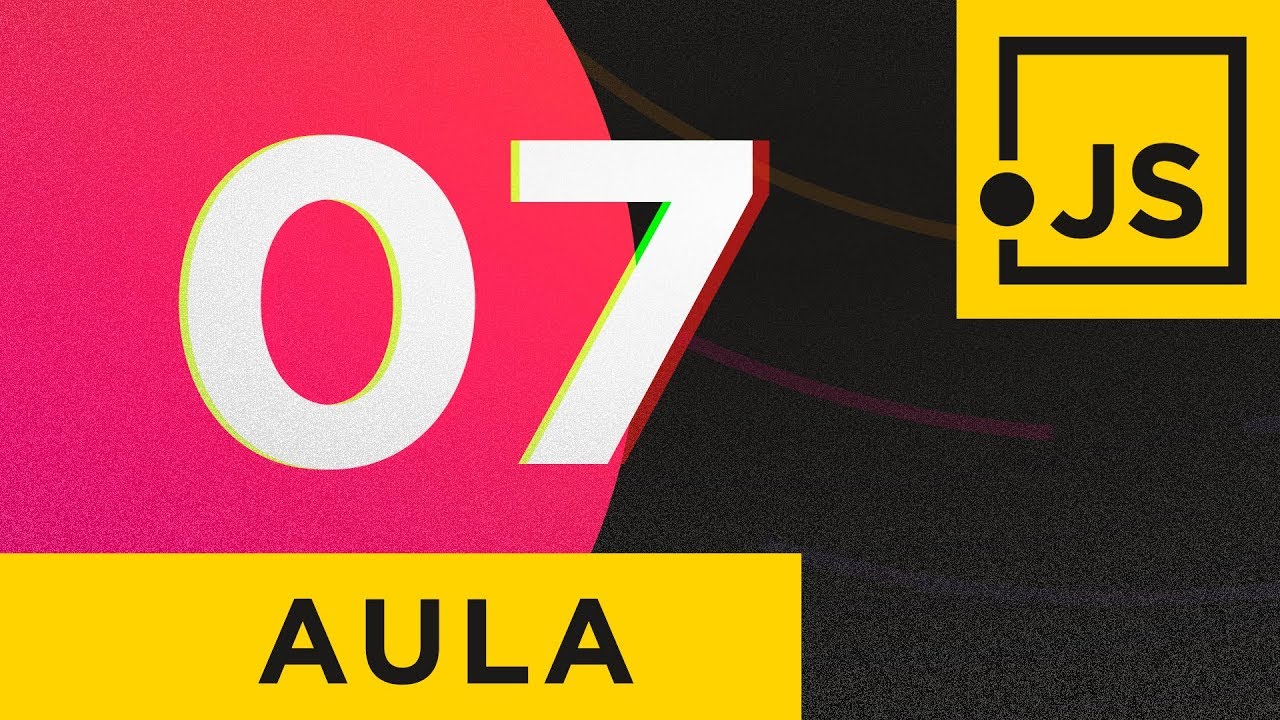
Operators (Part1) - JavaScript Course #07
5.0 / 5 (0 votes)