Classes and Objects in Python | OOP in Python | Python for Beginners #lec85
Summary
TLDRThis Python programming tutorial focuses on Object-Oriented Programming (OOP), emphasizing the importance of classes and objects. It explains the drawbacks of procedural programming and how OOP simplifies complex projects. The video uses a YouTube channel analogy to illustrate classes as blueprints for creating objects. It discusses attributes and methods, differentiating them from variables and functions. The instructor clarifies that everything in Python is an object, and classes are necessary for defining custom data types. The video concludes with a practical demonstration of creating a class and objects, and assigns an exercise to create a 'CarDesign' class with two objects.
Takeaways
- 📚 Object-Oriented Programming (OOP) is preferred over the Procedure-Oriented Programming (POP) approach, especially for handling large real-world projects.
- 🏗 A class in Python serves as a blueprint for creating objects, which are instances of the class.
- 👤 Objects have attributes (data) and methods (functions) attached to them, distinguishing them from simple variables and functions.
- 🎥 The instructor uses a virtual YouTube channel example to explain how classes and objects model real-world entities.
- 💻 Everything in Python is an object, including basic data types like integers and strings.
- 📝 The class design is like a prototype, similar to an idli maker, which simplifies object creation just as an idli maker helps make idlis efficiently.
- 🚗 A class can create many objects, like a car blueprint that helps produce multiple cars efficiently.
- 🛠 The class keyword is used to define a class, and PascalCase is recommended for naming class names.
- 🆕 Objects are created by calling the class as a constructor, and the `pass` statement can be used as a placeholder when defining empty classes.
- 📦 Classes can be thought of as user-defined data types, and objects as instances of those types, helping to manage real-world complexity in programs.
Q & A
What is the main focus of the video script?
-The main focus of the video is to discuss object-oriented programming (OOP) in Python, particularly about classes and objects, how to create them, and why they are important.
What are the drawbacks of the procedure-oriented programming (POP) approach?
-Procedure-oriented programming (POP) struggles to model real-world problems and cannot efficiently handle large, complex projects. This is why OOP is used in industries for large-scale software development.
What analogy does the speaker use to explain the concept of a class?
-The speaker uses an 'idli maker' analogy, where the idli maker is the class (blueprint) and the idlis produced are the objects (instances). This analogy helps explain how a class is like a design for creating objects.
What is the purpose of a class in OOP?
-A class in OOP serves as a blueprint or design for creating objects. It allows developers to define attributes and methods, making it easier to create and manage multiple objects with similar properties and behaviors.
How does the speaker differentiate between attributes and methods in OOP?
-Attributes represent the information or data associated with an object (e.g., name, address), while methods represent the actions or functions an object can perform (e.g., teaching, preparing a quiz). Attributes are attached to objects, and methods are the actions the object can perform.
Why is everything in Python considered an object?
-In Python, everything is considered an object because all data types, such as integers, strings, and even functions, are instances of inbuilt classes (e.g., int, str). This object-oriented structure allows for consistent handling of data and operations.
Why do we need classes if objects are important in Python?
-Classes are needed because they provide a structured way to define the properties and behaviors of objects. Without classes, managing multiple objects and their attributes or behaviors would be chaotic and inefficient.
What is the syntax for creating a class in Python?
-To create a class in Python, you use the `class` keyword followed by the class name in Pascal case (e.g., `ClassName`) and a colon. Inside the class, you can define attributes and methods.
What is the difference between free-floating variables/functions and attributes/methods?
-Free-floating variables and functions are not associated with any object, while attributes and methods are tied to a specific object. In OOP, attributes hold data specific to an object, and methods define the object's behavior.
What assignment is given at the end of the video?
-The assignment is to create a class called `CarDesign` and then create two objects of that class. The task is to practice defining and using classes and objects in Python.
Outlines
💻 Introduction to Object-Oriented Programming in Python
The speaker begins by addressing the audience and checks on their well-being. They then dive into the topic of object-oriented programming (OOP) in Python, emphasizing the shift from procedural programming due to its limitations in handling complex, real-world projects. The previous video introduced the concept of OOP, explaining the need for it over procedural programming. This video focuses on classes and objects, explaining the syntax for creating classes and objects in Python. The speaker uses the analogy of a YouTube channel to illustrate how OOP simplifies relationships and reduces project complexity. They discuss the idea of a class as a blueprint for creating objects, using the example of instructors within a YouTube channel. Attributes and methods are introduced as ways to represent the information and actions of an object, respectively. The video concludes with a reminder that everything in Python is an object, and classes are necessary to define new data types that don't exist by default.
🏗️ The Importance of Classes as Blueprints
In this paragraph, the speaker uses the analogy of an idli maker to explain the concept of a class as a blueprint for creating objects. They argue that without a design or blueprint, it would be difficult to create objects efficiently. The idli maker simplifies and speeds up the process of making idlis, just as classes simplify the creation of objects in programming. The speaker also compares classes to other blueprints like car models and house sketches, emphasizing their importance in manufacturing and construction. They conclude by reiterating that classes are essential for defining new data types and creating objects, which are instances of those classes.
📝 Syntax for Creating Classes and Objects in Python
The speaker provides a step-by-step guide on how to create a class in Python. They explain the syntax, which involves using the 'class' keyword followed by the class name in PascalCase and a colon. The class body, where attributes and methods are defined, is indented after the colon. The speaker notes that the class name should follow the PascalCase convention, with the first letter of each word capitalized. They also mention that if a class is empty, the 'pass' statement should be used as a placeholder. The paragraph concludes with a practical example of creating an empty class named 'Instructor' and an object named 'instructor1' from that class. The speaker demonstrates that even though the class is empty, it can still be used to create objects, which can then be identified by their class type.
🚗 Assignment: Creating a 'CarDesign' Class and Objects
In the final paragraph, the speaker assigns a task to the audience: to create a class named 'CarDesign' and two objects from this class. This exercise is intended to reinforce the concept of defining classes and creating objects in Python. The speaker reassures the audience that understanding these concepts takes time and that further videos will cover additional details, such as adding attributes and methods to classes and introducing the constructor method. The video ends with a farewell, promising more information in the next installment.
Mindmap
Keywords
💡Object-Oriented Programming (OOP)
💡Class
💡Object
💡Attribute
💡Method
💡Procedure-Oriented Programming (POP)
💡Blueprint
💡Instance
💡Constructor
💡User-Defined Data Type
Highlights
Introduction to object-oriented programming (OOP) in Python, discussing its advantages over procedure-oriented programming (POP).
Explanation of why object-oriented programming (OOP) is used in industry for handling large, real-world projects.
Review of the previous video covering the basics of OOP, classes, and objects.
Example of a YouTube channel simulation, where instructors, editors, and social media marketers are modeled as objects in OOP.
Discussion of attributes and methods: attributes define the data (e.g., name, address) of an object, and methods define the actions (e.g., teach, prepare quiz).
Explanation that in Python, everything is an object, even variables like integers and strings.
Introduction to built-in Python classes like `int` and `str`, which are used to create objects.
Explanation of the importance of classes as blueprints for creating objects, using examples such as an idli maker, cars, and house blueprints.
Analogy of a class being like a design or blueprint, while objects are the real-world instances created from that design.
Clarification that a class in Python is a user-defined data type, allowing the creation of custom types.
Introduction to the syntax for creating a class and its objects using the `class` keyword.
Explanation of the Pascal case convention for naming classes in Python (e.g., first letter of each word capitalized).
Introduction to the concept of constructors and the importance of the `__init__` method in Python classes.
Explanation of the `pass` statement to temporarily define an empty class or function.
Assignment for viewers to create a class called `CarDesign` and instantiate two objects from it.
Transcripts
hey everyone I hope you all are safe and
doing good so in the series of learning
Python programming language we are
discussing object oriented programming
in Python
previous video was about like that was
introduction to oop what is an op and
why we need oop that we have discussed
in previous video because there are some
drawbacks in the procedure oriented
programming approach pop approach that
is why we we are using oop right and to
sum what we have discussed like what is
a class and what is an object in the
previous video so please watch out that
video first and then come to this video
then you will get in better right now
more about classes and objects we will
be discussing in this video and how to
create your own class the syntax of
creative class and object why basically
we need a class
that thing we'll discuss in this video
right so if you remember the previous
video then we have come to this thing
like we have taken an example of a
YouTube channel
right
so how you can simplify the relationship
and how you can you can you know reduce
the complexity of a larger project with
the help of this Paradigm with help of
this approach and that is object
oriented programming
in the previous video we have concluded
that like the pop approach is not able
to handle real world project we cannot
model real world project or real world
problems using pop approach pop approach
cannot handle large project very large
project
you know that is why at Industries in
companies The Coop approach is being
used while implementing the projects
right so now we are creating like a
virtual YouTube channel right so like I
was the manager and I have
instructor I have had instructor then I
have editor and social media marketer to
handle their respective job right now I
have hired like two instructor Jenny and
gr to teach different different subjects
right so these were objects and the
instructor was class so it was just a
blueprint to create objects
right
right so we know like object oriented
programming is used to model real world
problems Real World objects right so
every object is having some information
and will do something like I am an
object
so I'll have some information like name
phone number address and I'll do
something maybe I'm teaching
right so what an object have that we can
model with the help of attributes and or
you can say variables and what I do or
what an object do that we can model with
help of functions or methods in
programming using oop approach right
here like
and stuff that is having this thing this
information name address and has book
has camera has laptop and all and what
the instruction instructor will do teach
and prepare quiz so these are methods or
functions see we do we don't call these
simple variables and functions we call
these attributes and methods why so
because these are associated these are
attached
with the instructor
like name is equal to Jenny address I
have some address so these name and
address are attached to me these are not
free floating variables so these are
attributes that is why we call these
attributes and the functions like I
teach I prepare quiz these are also
attached to me if I am an object right
so that is why they are known as methods
not just simple functions that are free
floating in your program no right that
is why these are known as attributes and
methods
right now see everything in Python is an
object
in the previous video I have you know
given you one example also simply if I
write X is equal to 1 and if I print
type of this x then what you will get
class ends so this x is an object of
Class end the class is inbuilt class if
I want to find out like x in x I am
having hello
so this is the string so in this at this
time it will give class as Str so this
is string this hello or this x is an
object of class Str same with function
in the previous video I have shown you
this thing practically right so we have
inbuilt classes in Python and we use the
object so
objects are more important everything we
do with the help of objects right
because this x is an option X1 this is
an object if you create a function that
is also an object right so objects are
very important we do everything with the
help of objects in our python Python
Programming right then if objects are
important then why we need classes
right let's take let's understand this
thing with the help of this example see
suppose like you have an idli maker
right so this is an idli maker and you
put that better or that stuff here in
this early maker and your idli will be
ready in next maybe I don't know the
recipe proper maybe 10 to 15 minutes
within 10 to 15 minutes right
so the idli you are having
these are actual objects and the idli
maker what you have that is just a
design
to make to prepare idlis
right
and if you don't have this design then I
think it would not be easy for you to
prepare idli maybe you can prepare with
the help of some other method I don't
know but
if you have the steadily maker then it
is very easy for you guys to prepare
many idlis
just in just you know few maybe hours or
few minutes
right
so this idli maker is going to make your
thing to make your you know process of
making idli is very smooth and simple
and quick right so it is a design same
if you relate this thing with like of
example so class is just a design or
blueprint so obviously to create object
to create idli
we need idli maker so we need a class to
create object we need a class that is
why classes are important
same like if you have any car
it may be greater
so there are like suppose one million
krata in India right I don't know the
number but this I'm just supposing this
thing so there is a model there there is
a prototype there is a design of that
car crater and using that design they
are creating multiple craters
in less amount of time so that design is
important that blueprint is important
same like
third example we can take like a map of
a house or a sketch of a house
right using that sketch we can create
house houses many houses so that house
you create a object the real thing that
is an object and that map is just a
class or a blueprint but using that
blueprint you can create multiple house
multiple houses
many houses
right and if you don't have that map
then definitely you can
build or you can construct a house
but if you have map then it would be
easy and you know simple to build a
house to construct a house because there
are many people
working there and one who know like what
is the map exact planning like what to
do next then he or she can easily guide
the contractor or anyone he can he or
she can easily Guide to the members what
to do next if you don't have map then it
would be not that much easy
to communicate so relationship would be
tough
right so now I hope you got the idea of
class and object and why we need class
class is also important right so we can
say this class is just a blueprint or a
design to create object an object or you
can say instance we can also say object
is an instance of
that class or instance of a class
right
or we can say class is a user defined
data type user defined type
why I'm saying so because see when you
take X is equal to 1 the type of X is
end
when I say x is equal to hello
then the type of this is
it is of class Str it is of Class end so
these classes are inbuilt classes
right like in test year or many classes
we have Boolean also we have float
double
right
so if you want a variable something like
this so the type of this is already
defined like int but if I want like uh
if like Jenny an instructor
so this would be of
I like this is not any type like I'm not
providing it as a string but Jenny is
like an object it is having some
information like me as an actual person
real person some information I'm having
some task I do right
so for this we have to Define our own
type
right so that we can Define with the
help of like class keyword and whatever
class name you give right so class
simply we can say it is a user defined
data type also okay this thing class is
a user user defined a type that you will
get it at the end of this video don't
worry if you are not getting this right
so syntax of creating a class how to
create your own class just class keyword
and
class
name
and column
and here obviously the data what you
want to right here that reviewed and the
methods and that also we'll see
right the class name is in Pascal case
it means the first letter of each word
is capital letter like class the name n
like our name so J is capital K is
capital like this
so we use this
uh like no you can say the what I should
say this I'm not
I'm not getting the exact word it's okay
so this is you can say some kind of rule
you have to use a pascal case to write
down a class name
like for for creating variable name we
simply use like underscore
like maybe I don't know like user
underscore name if a variable then we
use underscore this is a
first
is what
first is letter is small and for each
other words
the first letter is capital this is the
only difference between Pascal and camel
keys right
so
this is how you write class class or if
you write like something like this first
is small there is also small that also
will be fine it will not give you any
error but that is some kind of
you know rule or you can say this kind
of thing right just to differentiate
between a class name a function name or
a variable name something like this
right
so
like this we write class name fine this
is the syntax simple
and here you will write whatever you
want to like attribute methods and all
that also we'll see now how to create
object if from this if suppose class
name is instructor
if I want to create object of this class
then
maybe I am like
writing instructor underscore 1 is equal
to just the object name then class name
last name is
instructor but this is not it
this you need to pass
the these brackets down brackets
so this is somewhat like Constructor now
what is Constructor that also we'll see
but this is simple syntax of creating an
object let's see this practically okay
so let's create a new file
op Concepts classes dot p y
so how to create your own class just
class keyword
and class name so I am
creating a class instructor
so chemical sorry Pascal Pascal cases
first is
capital and if the next if you want to
write them like information i n f o info
then I would be again Capital so this is
Pascal case every letter of the word
would be Capital so just instructor and
then column right now
okay I'm not passing anything in class
no attribute no method so maybe you
think this is an empty class right and
I want to create object of this class so
instructor 1 the name of the object is
instructor one and then equal to the
name of the class instructor and just
these brackets but see now can you see
this red line here so this is an error
like this indent expected
so same with function I think you have
seen if you create function then you
cannot you know leave that function
empty you have to write down something
but I'm not I don't want like I I don't
know how what to write in this class
right now I don't know the syntax how to
write attribute and methods so I have
told you one
statement we have passed
so that you can select just write down
here pass so maybe further I'll do
something here but right now I don't
know what to do here so just write down
their path so now see there is no error
right so this is how you can create your
class and this is how you can create an
object
so if you run this then there would be
no errand but no output you will yet
obviously
now if you want to find out the type of
this object
type of the object
instructor one because name of the
object is instructor one right this is
the name of the object we have created
so what would be the type of this object
let's run this
see the type is class is just focus on
the class instructor
right although we have underscore
underscore main underscore underscore so
that this thing I'll be talking in later
videos right but now you have to focus
only on this name instructor right so
now we have created our own type our own
data type that is why I have told you
that line like class is a user defined
data type as
now as many object as we want we can
create from this class from this time
instructor One Two Three or many objects
many instructors
right but all would be of this type
class instructor so this is a user
defined data type that actually acts as
a blueprint to create objects right and
object is just an instance of a class
right now one assignment for you is you
have to create a class and the class
name would be car design
car design this will be the name of the
class
right and you have to create two object
of that class
right so this is your assignment you can
write them that thing in comment section
so now I hope you got what is a class
what is an object what is objective
programming how to create your class how
to create an object right if you are not
getting this if you are still getting
like 50 then it's fine
not slowly you will get everything in
later videos right now in the next video
we will see like how to add attribute to
your class and there is a method like
init
that also we will see
in next video like underscore underscore
unit underscore underscore then in
bracket self so what is this method that
we will see in next video what is
Constructor and this kind of concept
right
so I'll see you now in the next video
till never bye take
تصفح المزيد من مقاطع الفيديو ذات الصلة
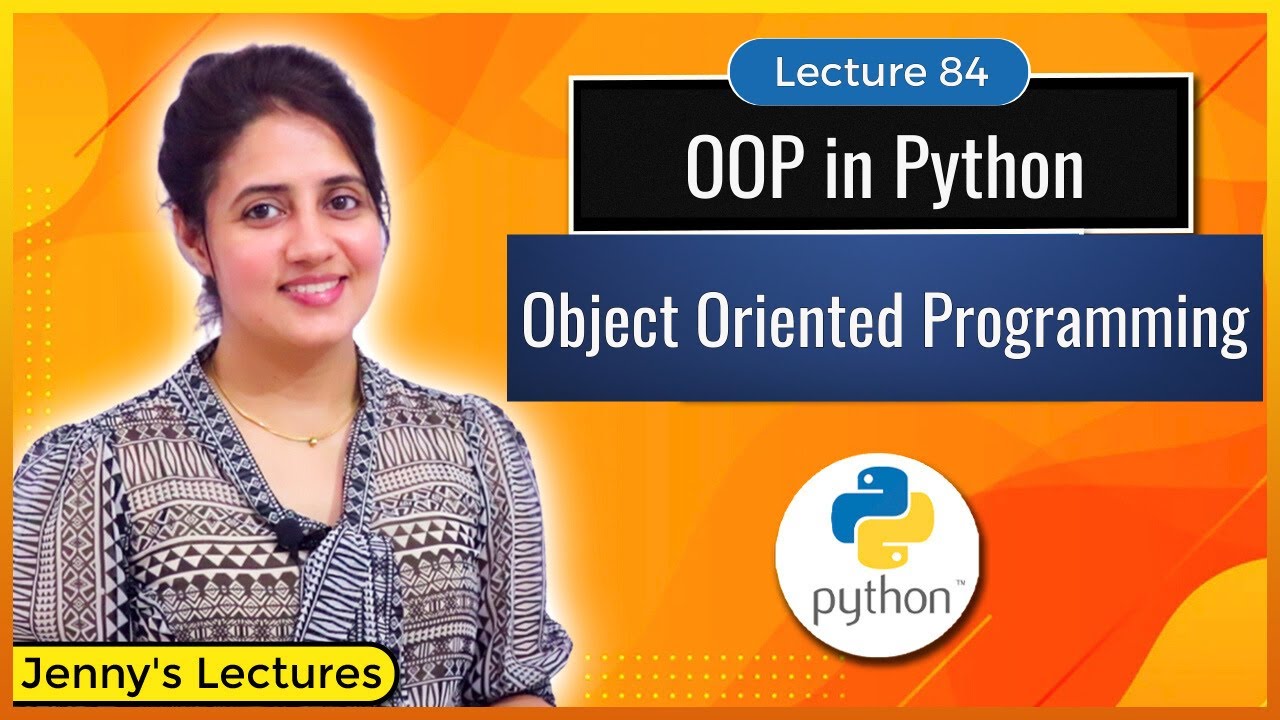
OOP in Python | Object Oriented Programming | Python for Beginners #lec84
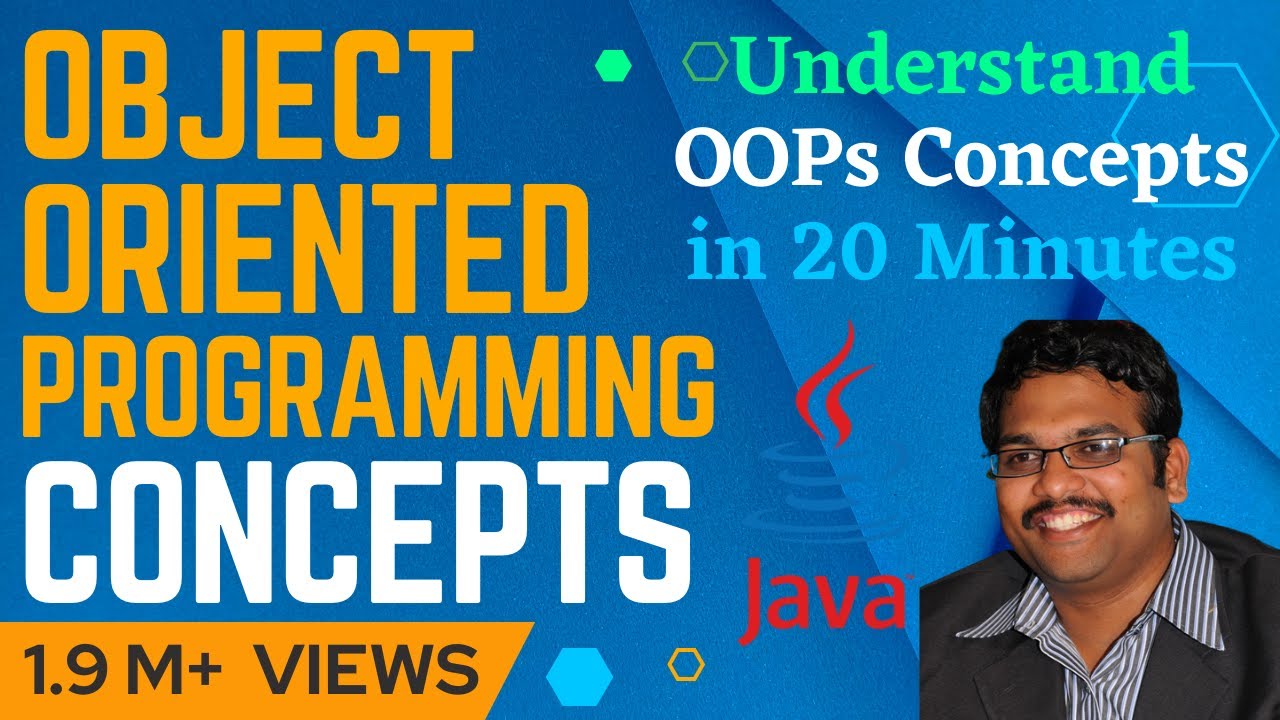
OOPS CONCEPTS - JAVA PROGRAMMING
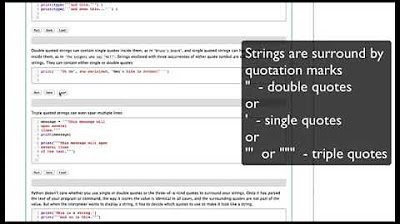
TypesNTypeConversion
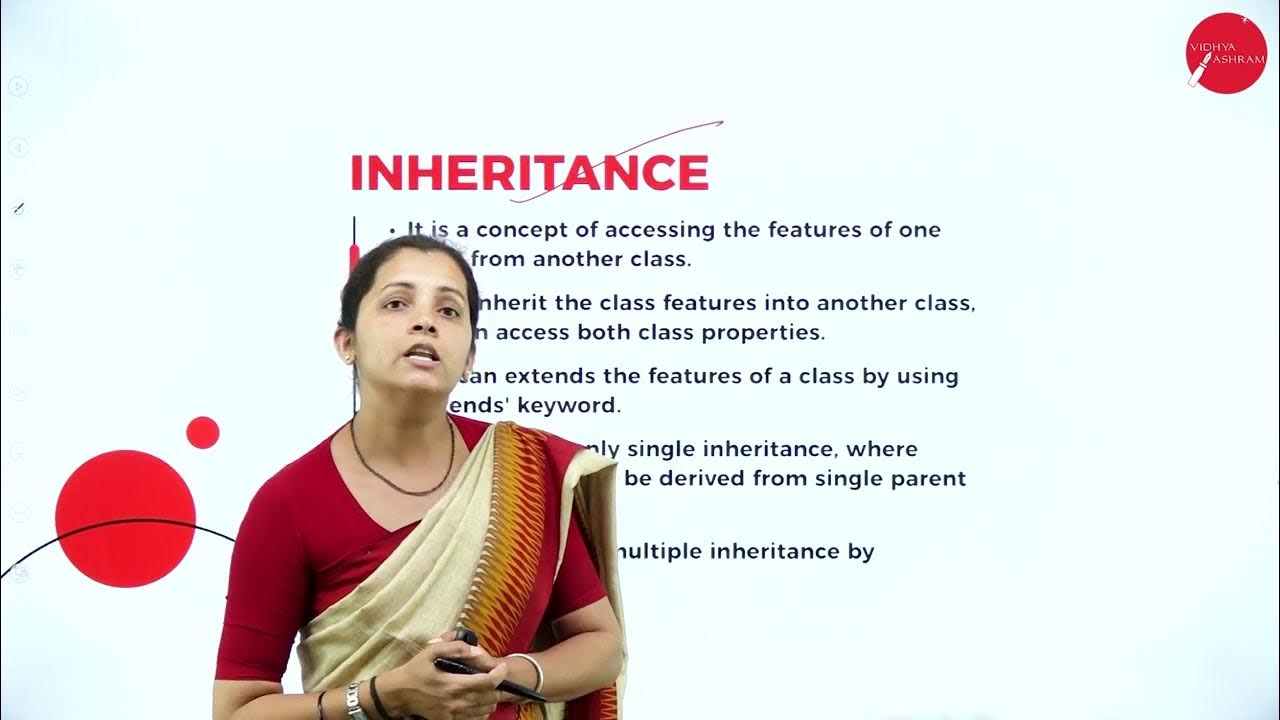
DAY 07 | PHP AND MYSQL | VI SEM | B.CA | CLASS AND OBJECTS IN PHP | L1

Java Basic OOP Concepts | Features of OOPs in Java | Learn Coding

Introduction to OOPs in Python | Python Tutorial - Day #56
5.0 / 5 (0 votes)