72. OCR A Level (H046-H446) SLR13 - 1.4 Primitive data types
Summary
TLDRThis video provides an introduction to common primitive data types used in programming, including integers, floats, booleans, characters, and strings. It explains how these data types differ in memory usage and emphasizes the importance of choosing the right one to optimize performance. The concept of casting, or converting one data type into another, is also discussed. The video highlights differences in data type syntax across languages like Python, Java, and C#. Viewers are encouraged to understand these core data types for exams and consider more complex types for advanced programming tasks.
Takeaways
- 💻 Data types refer to different kinds of data we store in computer programs, and different data types take up varying amounts of memory.
- 🔢 Primitive data types are basic data types provided by the programming language, used as foundational building blocks.
- 📐 Common primitive data types include integers, real (float), booleans, characters, and strings.
- 💡 Integers represent positive or negative whole numbers, while floats (real numbers) represent numbers with fractional or decimal components.
- 🔠 The character data type represents a single alphanumeric symbol, and the string data type represents a collection of characters.
- ✅ The boolean data type represents one of two possible values: true or false, and is often used in conditional programming statements.
- 🔄 Casting is the process of converting one data type to another, which is often necessary for operations like mathematical computations.
- 📜 Different programming languages have varied syntax for data types and casting. It's important to recognize these differences, especially for exams.
- 🧠 Understanding and selecting the appropriate data type for a specific task is crucial for optimizing memory usage and program performance.
- 📊 Beyond basic primitive data types, languages like C# support many more data types, especially for specific use cases like number storage.
Q & A
What is a data type in programming?
-A data type defines the kind of data a variable can hold, such as integers, floating-point numbers, characters, strings, or booleans. Different data types require different amounts of memory.
Why is it important to use the correct data type in programming?
-Using the correct data type optimizes program performance and memory usage. Selecting the appropriate data type ensures efficient use of memory and avoids potential errors when manipulating data.
What are the primitive data types mentioned in the video?
-The primitive data types mentioned in the video are integer, real or float, boolean, character, and string.
What is a primitive data type?
-A primitive data type is a basic data type provided by a programming language as a foundational building block, such as integers, booleans, and characters.
Can you explain the difference between an integer and a real (float) data type?
-An integer data type represents whole numbers, either positive or negative, while a real or float data type represents numbers with fractional or decimal components.
What is the purpose of the character data type?
-The character data type represents a single alphanumeric character or symbol, such as a letter, number, or punctuation mark.
What is the string data type and how is it different from the character data type?
-The string data type represents a collection of characters, which can include letters, numbers, symbols, or punctuation, whereas the character data type represents only a single character.
What is the boolean data type and where is it commonly used?
-The boolean data type represents one of two possible values: true or false. It is commonly used in conditional programming statements to control the flow of code based on logical expressions.
What is casting, and why is it important?
-Casting is the process of converting one data type to another. It is important when performing operations that require a specific data type, such as converting a character to an integer before doing mathematical calculations.
How do data types vary across different programming languages?
-While the concept of primitive data types exists in all programming languages, their names and syntax can vary. For example, the boolean data type is 'bool' in Python, 'Boolean' in VB, and 'bool' in Java and C#.
Outlines
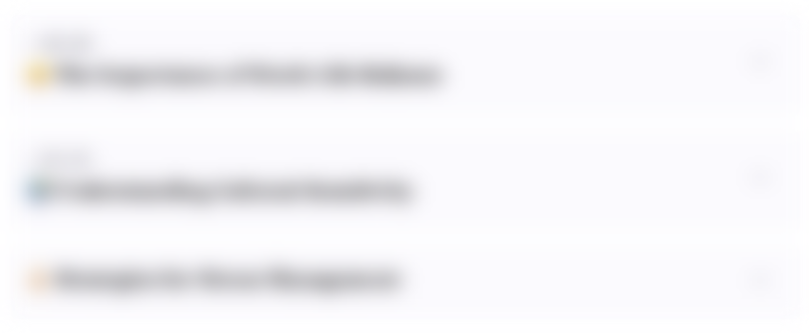
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
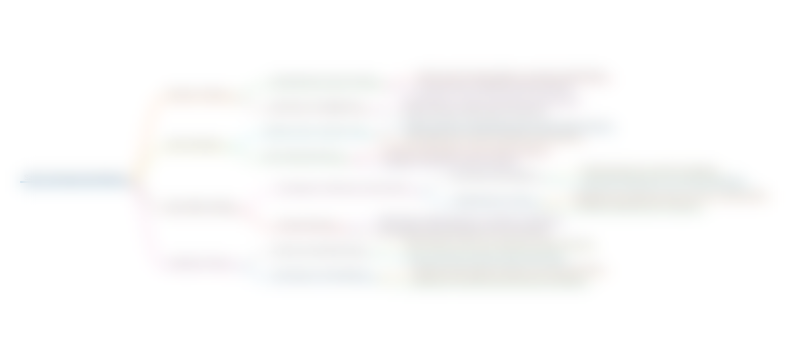
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
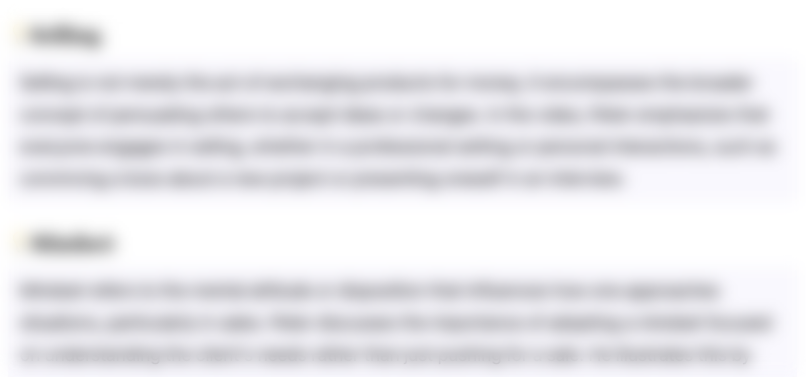
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
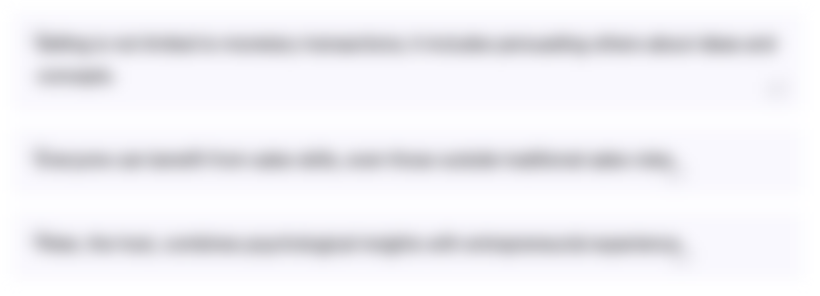
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
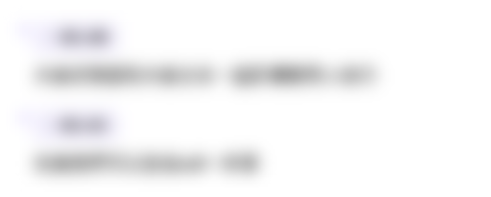
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
5.0 / 5 (0 votes)