Binary Search Trees (BST) Explained in Animated Demo
Summary
TLDRIn this video, Joe James explains binary search trees, a data structure with nodes connected by edges. Each node can have up to two children, forming a hierarchy with a root. Binary search trees maintain order, with each node greater than its left subtree and less than its right. Operations like insertion, deletion, and finding a node are efficient, taking O(h) time, where h is the height of the tree. The video also covers how to handle deletions with different child counts, emphasizing the speed benefits of balanced trees.
Takeaways
- 🌳 A binary search tree (BST) is a hierarchical structure where each node has at most two child nodes, arranged in a way that the left child node's value is less than the parent node's value, and the right child node's value is greater.
- 🔍 In a BST, searching for a node starts at the root and compares the target value with the current node's value, moving to the left or right child accordingly until the node is found or the target is determined to not exist in the tree.
- 🍃 Leaf nodes in a BST are nodes that do not have any children.
- 📚 The left and right children of a node are referred to as siblings.
- 📈 The property of a BST ensures that for any given node, all nodes in its left subtree are less than the node and all nodes in its right subtree are greater.
- 💼 Insertion in a BST involves starting at the root and moving to the left or right child depending on whether the value to insert is less than or greater than the current node's value until an appropriate position to insert the new node as a leaf is found.
- 🔑 The find operation in a BST is efficient because it eliminates half of the remaining tree with each comparison, making it much faster than a linear search.
- ❌ Deletion in a BST can be complex and depends on whether the node to be deleted is a leaf, has one child, or two children, each requiring a different approach to maintain the BST properties.
- 🔄 If a node to be deleted has one child, the child replaces the node in the tree, effectively removing the node without disrupting the BST properties.
- 🔄 If a node to be deleted has two children, the typical approach is to find the in-order successor (the smallest node in the right subtree) or the in-order predecessor (the largest node in the left subtree), replace the node with this successor or predecessor, and then delete the successor or predecessor.
- 🏃 The operations of insertion, deletion, and find in a BST are generally O(h), where h is the height of the tree, making them very efficient in balanced trees.
Q & A
What is a binary search tree?
-A binary search tree is a binary tree data structure where each node has at most two children, which are referred to as the left child and the right child. For each node, all elements in its left subtree are less than the node and all elements in its right subtree are greater.
What is the significance of the root node in a binary search tree?
-The root node is the topmost node in the tree and serves as the starting point for operations such as insertion, deletion, and searching.
What are the characteristics of leaf nodes in a binary search tree?
-Leaf nodes are nodes that do not have any children. They are the terminal nodes in the tree structure.
How are siblings defined in a binary search tree?
-Siblings in a binary search tree are nodes that share the same parent node.
What is the rule for ordering nodes in a binary search tree?
-In a binary search tree, each node is greater than every node in its left subtree and less than every node in its right subtree.
How does the insert operation work in a binary search tree?
-The insert operation starts at the root and compares the value to be inserted with the current node's value. Depending on whether the value is less than or greater than the current node's value, it moves to the left or right child, respectively, until it finds the correct position to insert the new node as a leaf.
Can you explain the find operation in a binary search tree?
-The find operation starts at the root and compares the target value with the current node's value. It traverses the tree, moving to the left child if the target is less and to the right child if the target is greater, until it either finds the node or reaches a null child, indicating the node is not present.
What are the three cases for the delete operation in a binary search tree?
-The three cases for the delete operation are: 1) Deleting a leaf node, which can be simply removed. 2) Deleting a node with one child, where the child replaces the node. 3) Deleting a node with two children, where the node is replaced with its in-order successor or predecessor, usually the smallest value in its right subtree or the largest in its left subtree.
Why is the next highest node used when deleting a node with two children?
-The next highest node (in-order successor) is used to maintain the binary search tree property after deletion. It replaces the deleted node and is either the smallest node in the right subtree (or the largest in the left subtree), ensuring that all nodes in the left subtree are still less and all in the right subtree are still greater.
What is the time complexity for insert, find, and delete operations in a binary search tree?
-The time complexity for insert, find, and delete operations in a binary search tree is O(h), where h is the height of the tree. This means that in a balanced tree, these operations can be performed very efficiently, often in logarithmic time.
Why are binary search trees considered efficient for certain operations?
-Binary search trees are considered efficient because they allow for fast lookup, addition, and removal of items. In a balanced tree, these operations can be performed in O(log n) time, where n is the number of nodes in the tree.
Outlines
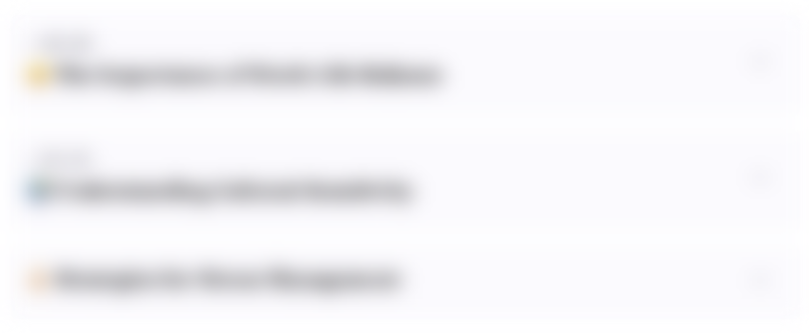
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
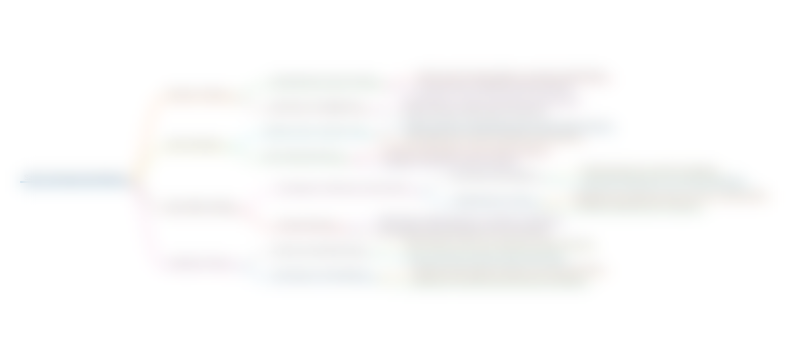
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
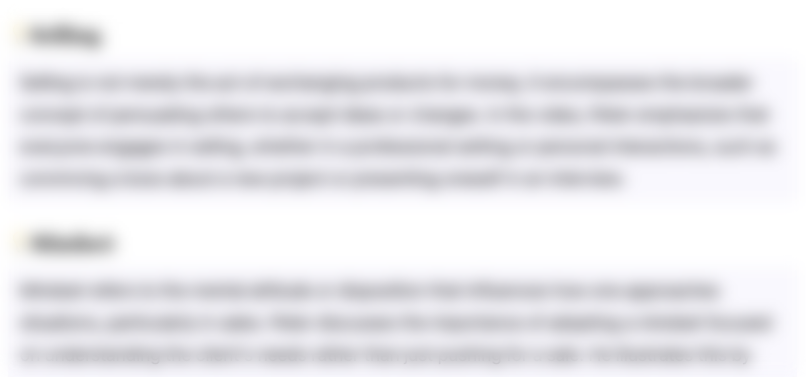
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
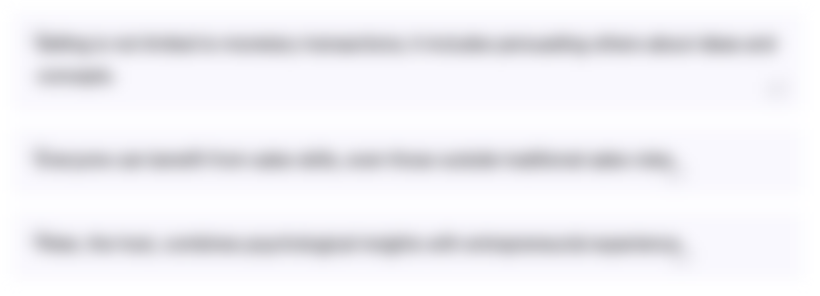
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
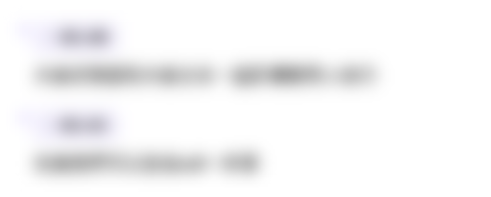
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
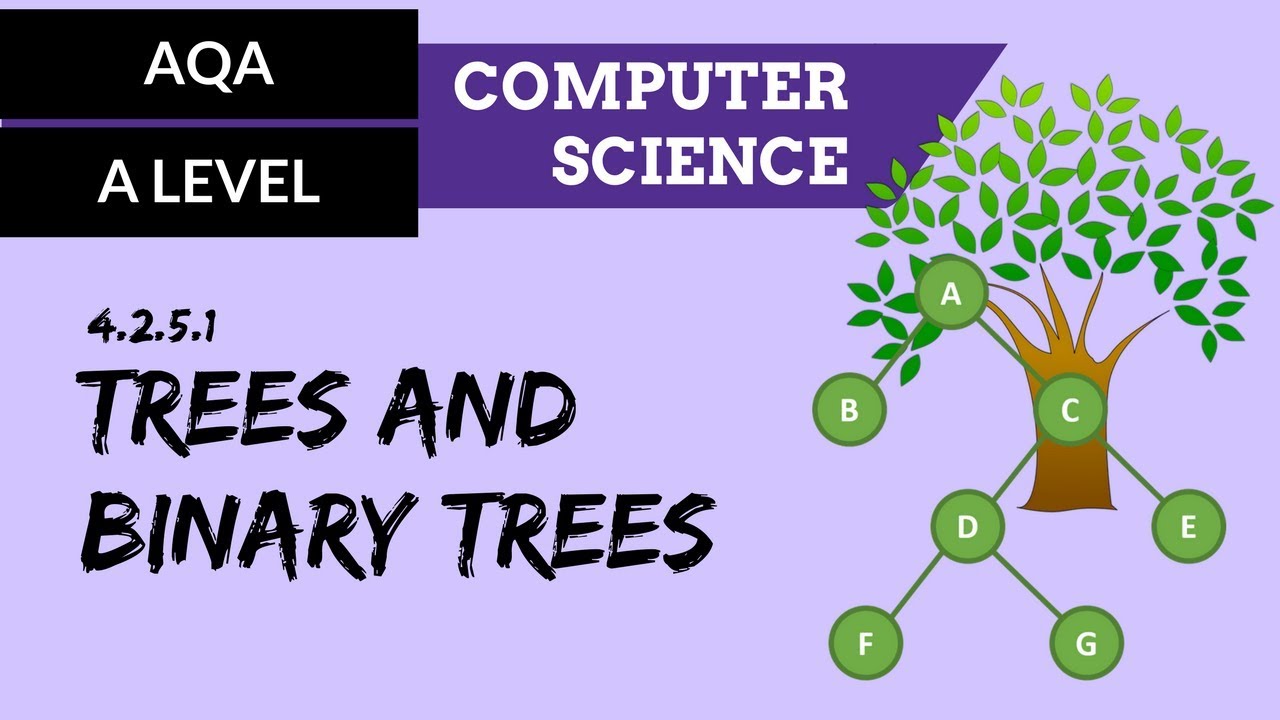
AQA A’Level Trees & Binary trees

L-3.7: Introduction to Trees (Binary Tree, Almost Complete Binary Tree, Full BT, Complete BT, BST)

90. OCR A Level (H446) SLR14 - 1.4 Data structures part 4 - Trees
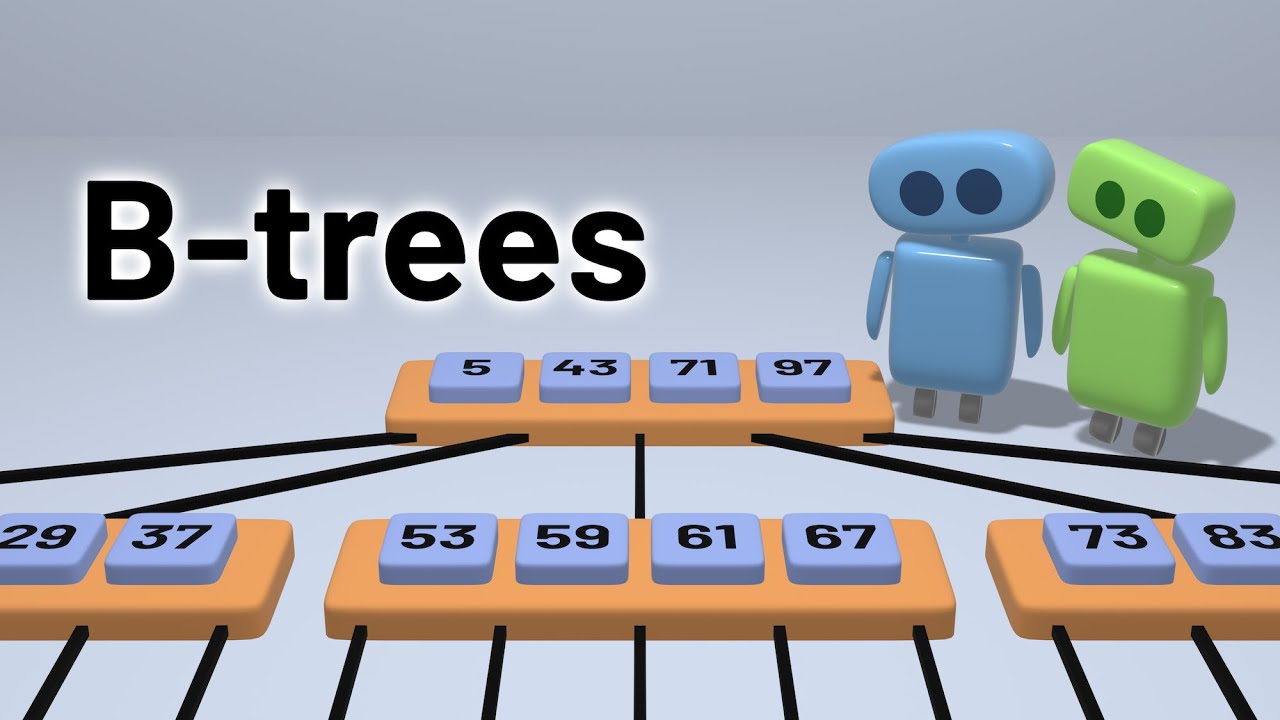
Understanding B-Trees: The Data Structure Behind Modern Databases

Aula 05.1 - Árvores Rubro Negras: Definição (AED2)

AQA A’Level Relationship between trees & graphs
5.0 / 5 (0 votes)