Python an Immutable Object
Summary
TLDRThis video delves into the concept of object immutability in Python, using the example of an integer object with the value 2. It clarifies the misconception that variables can change by demonstrating that when 'first_number' is reassigned the value 5, a new object is created and the reference is updated, leaving the original object unreferenced and eligible for garbage collection. The video emphasizes the importance of understanding object references and the role of the garbage collector in managing memory, encouraging viewers to revisit these concepts as they advance in their Python journey.
Takeaways
- 🔑 The video assumes viewers have seen the previous one, which introduced key concepts for understanding this video's content.
- 📚 The previous video explained how assigning a value to a variable creates an execution space, an object reference, and an object, binding them together.
- 🔢 The video discusses the concept of an 'integer object', which is immutable, meaning its value cannot be changed once created.
- 🤔 The notion of immutability may seem counterintuitive when we can change the value of a variable in Python, but the video clarifies this by explaining the process of object reference reassignment.
- 🎯 When a variable is reassigned a new value, Python creates a new object for that value, and the object reference is updated to point to the new object, leaving the old object unbound.
- 🗑️ Unbound objects are eventually cleaned up by Python's garbage collector, which frees up memory space by removing objects that are no longer referenced.
- 🧩 The video uses the analogy of an execution space to illustrate how Python manages memory and object references, providing a deeper understanding of what happens 'under the hood'.
- 📈 The video encourages viewers to start with a simpler model of variables and objects and to revisit the concept of immutability as their understanding of Python deepens.
- 🔄 The process of variable assignment and reassignment in Python involves more steps than might be initially apparent, including object creation and reference updating.
- 🔗 The video suggests visiting a supporting website and subscribing to the YouTube channel for further updates and resources on Python programming.
Q & A
What is the key concept introduced in the previous video that is essential for understanding this video?
-The key concept introduced in the previous video is the process of assigning values to variables, which involves creating an execution space, generating an object reference, and binding the object reference to an object with a specific value.
What does it mean for an object to be immutable in Python?
-An object being immutable in Python means that once it is created, its value cannot be changed. This is in contrast to the common perception of variable assignment where it appears that the value of a variable can be changed.
How does Python handle the assignment of a new value to a variable that was previously assigned to an immutable object?
-When a new value is assigned to a variable that was previously bound to an immutable object, Python creates a new object with the new value and updates the object reference to point to this new object, leaving the old object unbound and available for garbage collection.
What is the role of the garbage collector in Python's memory management?
-The garbage collector in Python is responsible for identifying and removing objects that are no longer referenced by any variables or object references, thus freeing up memory space for other programs to use.
Why is it important to understand the difference between the simple model of variable assignment and the more complex reality of object references and immutability?
-Understanding the difference between the simple model of variable assignment and the complex reality of object references and immutability is important because it provides a deeper insight into how Python manages memory and how variables actually work under the hood, which can help in writing more efficient and error-free code.
What happens when an object reference is overwritten with a new address during variable assignment?
-When an object reference is overwritten with a new address, the previous binding to the old object is removed, and the object reference now points to the new object. The old object, if no other references point to it, becomes eligible for garbage collection.
How does the concept of immutability relate to the performance and efficiency of Python programs?
-The concept of immutability can contribute to the performance and efficiency of Python programs by preventing unintended side effects and allowing for certain optimizations, such as the reuse of immutable objects and the avoidance of unnecessary memory allocation.
What is the practical implication of understanding the difference between the simple model of variable assignment and the actual process in Python?
-The practical implication of understanding the difference is that it helps programmers to anticipate how their code will behave, especially in complex scenarios involving data structures and object-oriented programming, leading to better design and debugging strategies.
Why might a programmer initially be confused by the concept of immutability when they can seemingly change the value of a variable?
-A programmer might initially be confused by the concept of immutability because the act of changing a variable's value in Python actually involves creating a new object and updating the object reference, rather than changing the value of the existing object, which can seem counterintuitive to the common understanding of variable assignment.
How can the understanding of object references and immutability help in managing memory more effectively in Python?
-Understanding object references and immutability can help in managing memory more effectively by allowing programmers to write code that minimizes unnecessary object creation and takes advantage of Python's garbage collection, thus optimizing memory usage and performance.
Outlines
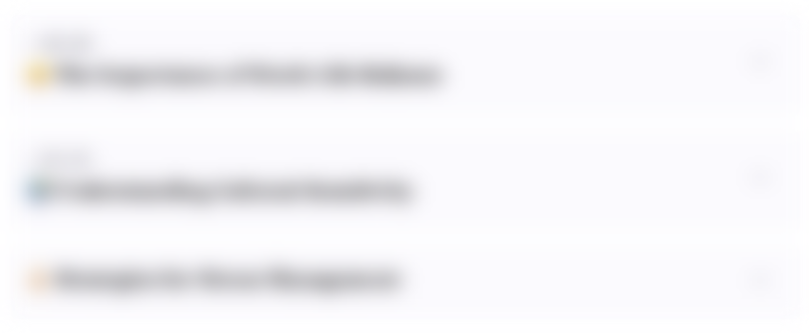
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
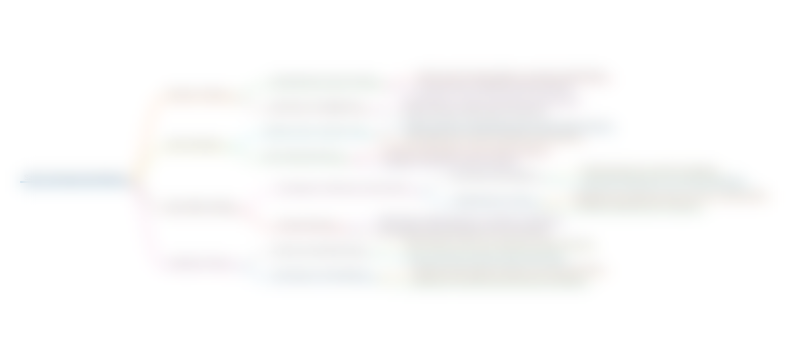
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
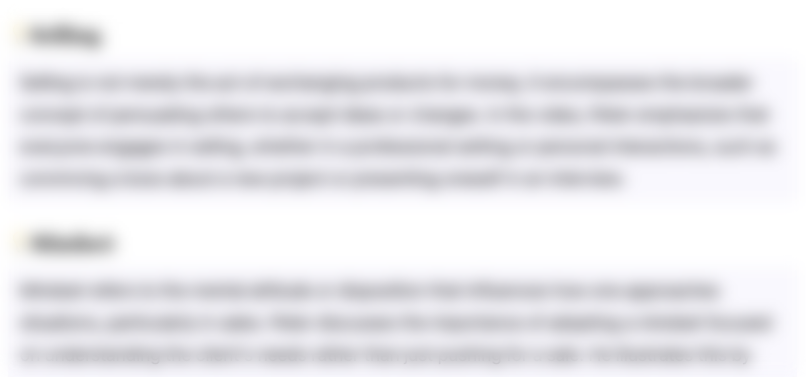
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
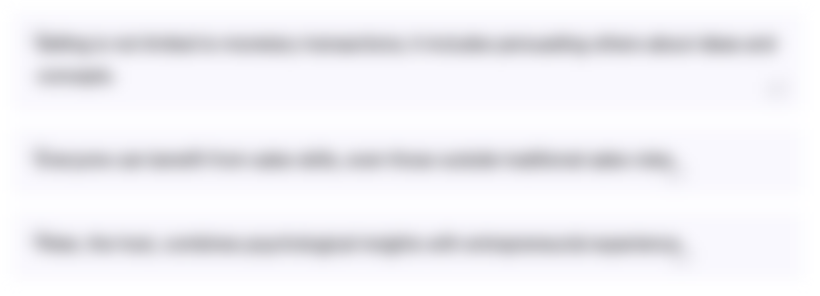
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
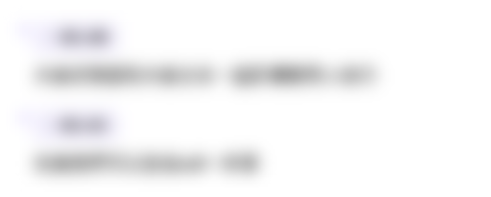
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
5.0 / 5 (0 votes)