Learn React Hooks In 6 Minutes
Summary
TLDRThis video script discusses the evolution of React.js from class components to functional components with hooks. It explains how React hooks, introduced in version 16.8, enable state management and lifecycle handling in functional components. The tutorial demonstrates converting a class component to a functional one using useState and useEffect hooks, highlighting the benefits and efficiency of the new approach. The speaker also mentions plans for future videos on hooks' advantages and creating custom hooks.
Takeaways
- 🚀 React has evolved to favor function components over class components for simpler and more modern code.
- 🔄 React Hooks, introduced in React 16.8, are the solution to managing state and replacing lifecycle methods in function components.
- 🎯 The `useState` hook allows function components to maintain and update state without the need for a class component's `this.setState`.
- 🔧 Multiple pieces of state can be managed in a function component by using `useState` multiple times, each creating its own state variable and updater function.
- 🔏 The `useEffect` hook can be used to replicate the behavior of lifecycle methods like `componentDidMount`, `componentDidUpdate`, and even `componentWillUnmount`.
- 💡 By passing an empty array to `useEffect`, the function inside runs only once, similar to `componentDidMount`, while the lack of an array triggers the function on every render.
- 🛠 To persist state across page refreshes or visits, `useEffect` can be used to save and retrieve state from local storage, updating the component's state accordingly.
- 📌 React Hooks promote a more declarative programming approach, focusing on reacting to data changes rather than component lifecycle events.
- 📈 Using hooks can lead to more concise and potentially less error-prone code, as it abstracts away some of the complexities of class components.
- 🔜 Future React tutorials will increasingly focus on the use of hooks, reflecting the shift in best practices within the React community.
- 🤖 Creating custom hooks is a powerful feature that allows for the abstraction of reusable logic and state management patterns across different components.
Q & A
What has changed in React since the introduction of class components?
-React has evolved to favor the use of function components over class components. This shift has led to the introduction of React Hooks, which allow for state management and lifecycle handling in function components, replacing the need for class component methods like 'this.setState' and lifecycle events like 'componentDidMount' and 'componentDidUpdate'.
What are React Hooks and when were they introduced?
-React Hooks are a feature introduced in React version 16.8 that enables developers to use state and other React features without writing class components. Hooks provide a way to manage state and side effects in function components, making them more powerful and flexible.
How does the 'useState' hook work in function components?
-The 'useState' hook is used to manage state in function components. It returns an array with two elements: the first is the current state value, and the second is a function to update that state. Optionally, an initial state value can be provided. In the script, 'useState' is used to manage the text displayed and the count of button clicks.
How can you handle component lifecycle events using React Hooks?
-The 'useEffect' hook is used to handle lifecycle events in function components. It accepts a function that contains the side effects you want to occur during the lifecycle of the component. By passing an empty array as the second argument to 'useEffect', the function will only run once, similar to 'componentDidMount'. If you want to run the function whenever a specific state changes, you include that state variable in the array.
What is the role of the 'useEffect' hook in replacing lifecycle methods?
-The 'useEffect' hook replaces the need for lifecycle methods by allowing you to specify when you want certain side effects to happen. For example, you can replicate the behavior of 'componentDidMount' by running code in 'useEffect' with an empty array as the second argument. This makes it clear that the code should run once, when the component first mounts.
How can you save and retrieve state between component mounts using 'useEffect' and local storage?
-By using 'useEffect' with an empty array as the second argument, you can save the state to local storage when the component mounts. This ensures that the state is persisted even when the component unmounts and remounts. To retrieve this state on initial mount, you check local storage and set the state accordingly within the 'useEffect' hook.
What is the significance of the 'useEffect' hook in managing side effects?
-The 'useEffect' hook is significant because it provides a consistent and centralized way to manage side effects in function components. It allows developers to react to state changes and perform necessary actions, such as updating the DOM, fetching data, or interacting with other APIs, all in a clean and organized manner.
How does the 'useEffect' hook differ from 'componentDidUpdate'?
-While 'componentDidUpdate' is a lifecycle method that runs after updates to the component, 'useEffect' is a hook that can be used to handle similar behavior. 'useEffect' runs after every render by default, but you can control its behavior by providing a specific array as the second argument to the hook. This allows you to run code only when certain variables change, similar to the 'componentDidUpdate' method.
What is the role of the second argument in the 'useEffect' hook?
-The second argument to 'useEffect' is an array that determines when the function provided as the first argument should run. If no array is provided, the function will run after every render. If an empty array is provided, the function will run once, on mount. If the array contains specific variables, the function will run only when those variables change.
How can you create a custom hook in React?
-Custom hooks can be created in React by encapsulating a piece of state logic or side effect within a function that returns one or more hooks. These custom hooks can then be reused across different components, promoting code reuse and cleaner component structures.
What are the benefits of using React Hooks in function components?
-React Hooks provide several benefits, including simpler and more readable code by eliminating the need to create class components with lifecycle methods, better reusability of logic through custom hooks, and improved performance through more granular control over when effects run.
Why is the mindset shift when using React Hooks important?
-The mindset shift when using React Hooks is important because it encourages developers to think in terms of data changes and their effects, rather than component lifecycles. This leads to more maintainable and efficient code, as well as easier debugging and understanding of how components behave in response to state changes.
Outlines
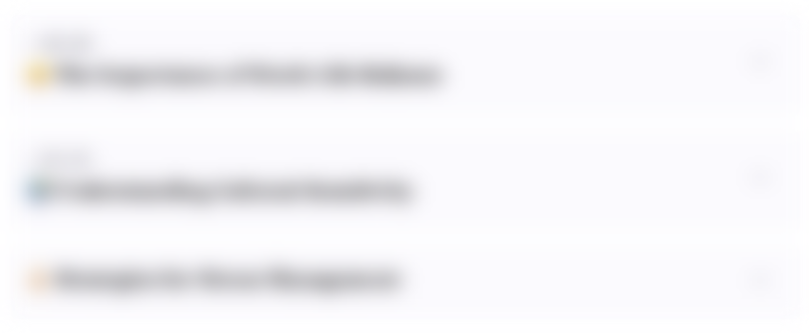
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
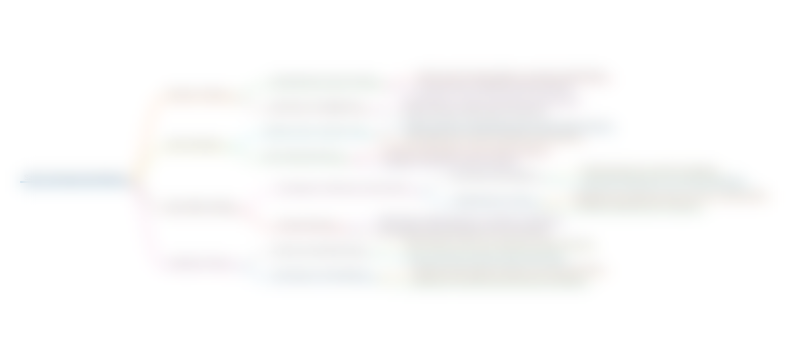
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
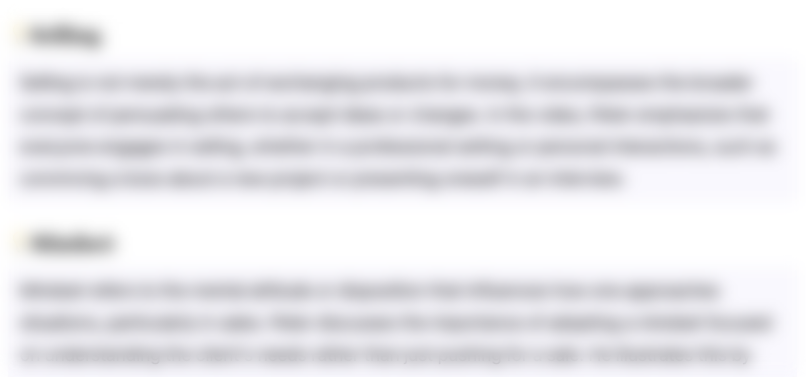
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
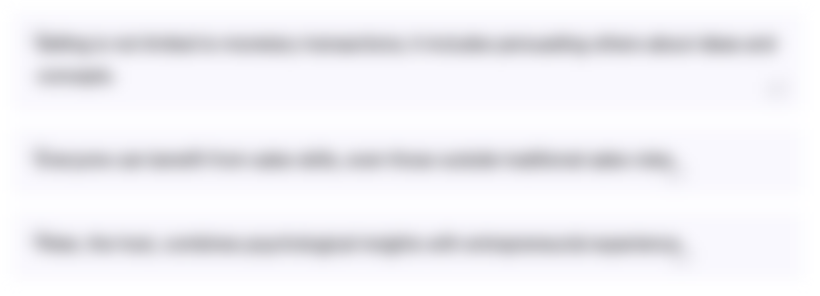
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
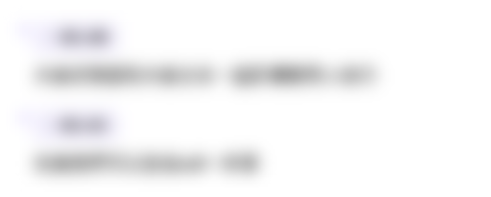
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
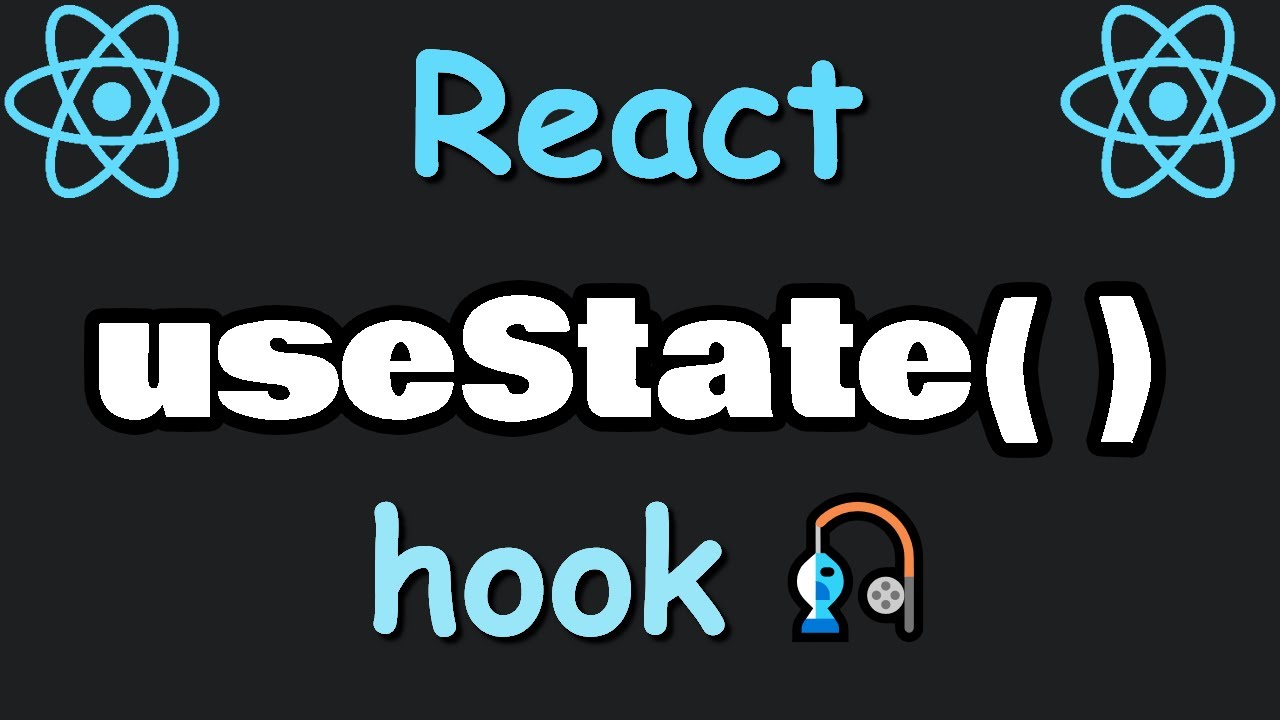
React useState() hook introduction 🎣
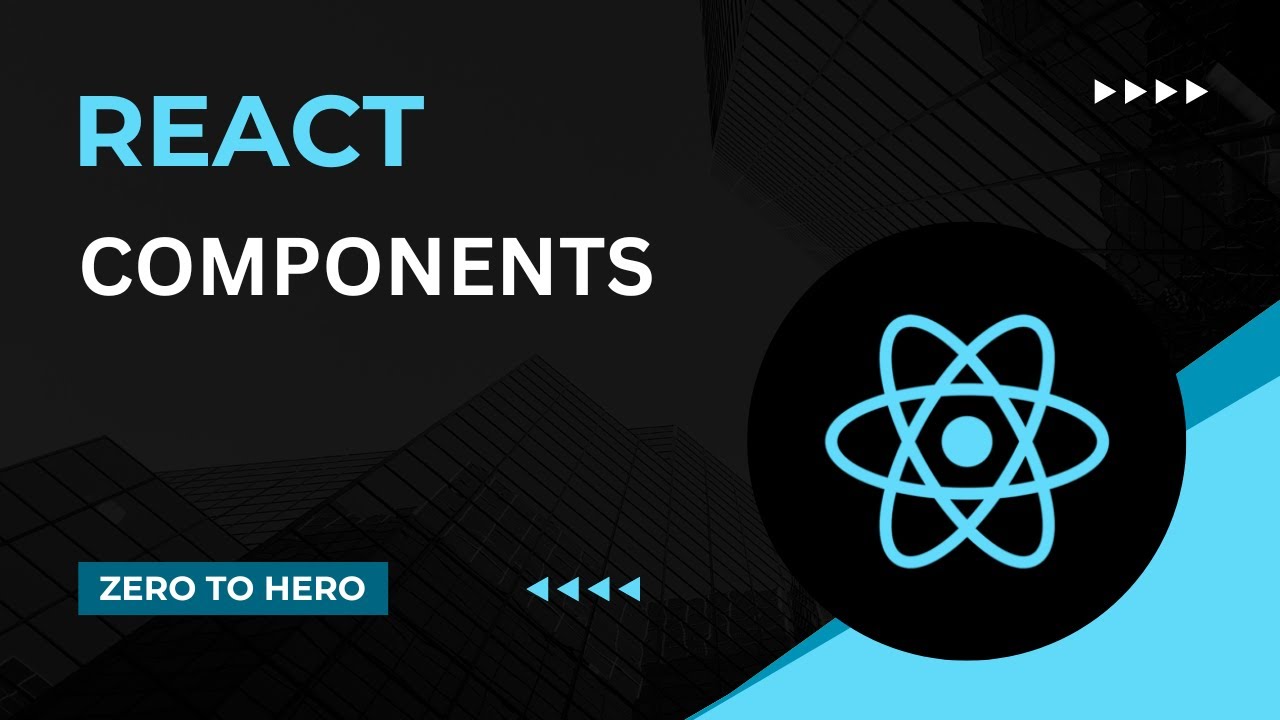
Components | Mastering React: An In-Depth Zero to Hero Video Series

React Hooks and Their Rules | Lecture 157 | React.JS 🔥
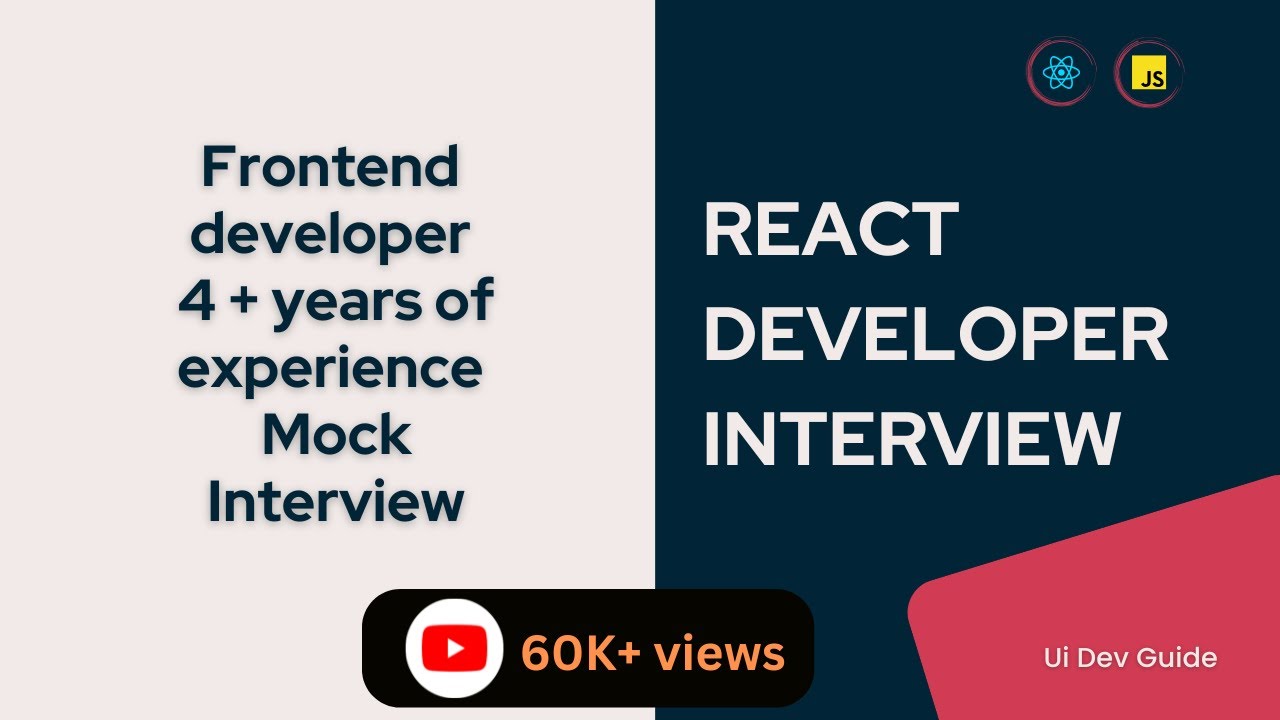
React Interview | Frontend Developer | React interview questions 5 to 8 years 2023 #reactjs

useState Hook | Mastering React: An In-Depth Zero to Hero Video Series
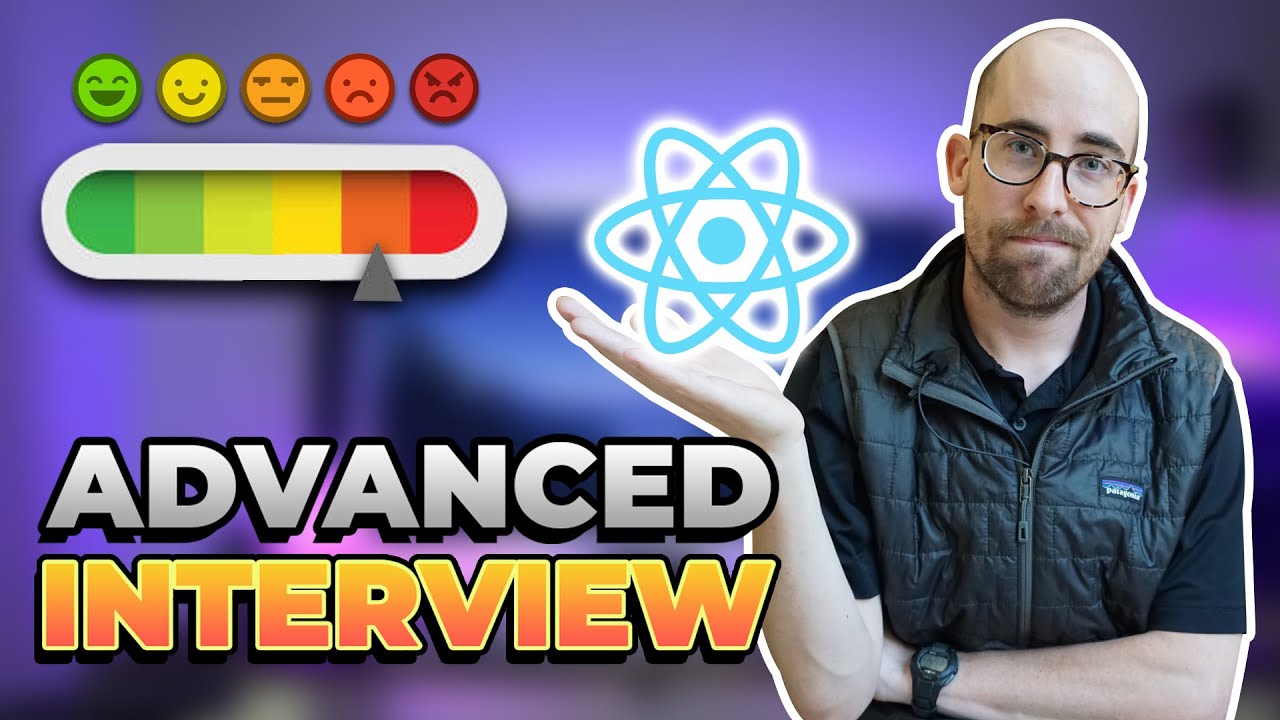
HARD React Interview Questions (3 patterns)
5.0 / 5 (0 votes)