🔥📱 Flutter x Firebase CRUD Masterclass • Create / Read / Update / Delete
Summary
TLDRIn this Flutter and Firebase master class, the instructor guides viewers through creating a simple notes app to demonstrate the four essential database operations: Create, Read, Update, and Delete (CRUD). The tutorial starts by setting up a Firebase project and connecting it to a new Flutter app. It then covers adding the Firebase Core and Firestore packages, initializing Firebase in the app, and setting up Firestore rules. The instructor shows how to create a Firestore service for CRUD operations, implement a UI with a floating action button, and use a StreamBuilder to display notes. The video concludes with adding, updating, and deleting notes, providing a comprehensive introduction to using Flutter and Firebase for database interactions.
Takeaways
- 🚀 The video is a Flutter and Firebase masterclass focused on teaching CRUD (Create, Read, Update, Delete) operations for databases.
- 🔑 The tutorial demonstrates creating a simple notes app to explain CRUD operations, allowing users to manage notes stored in a Firebase database.
- 💻 The process starts by setting up a new Firebase project and connecting it with a Flutter project using the Firebase CLI tools.
- 📝 Firebase Firestore is used as the database, and rules are configured to allow read and write access for demonstration purposes.
- 🛠️ The video guides through adding the Firebase core and Cloud Firestore packages to the Flutter project for database interactions.
- 📱 A Flutter UI is created with a floating action button to add new notes, and a stateful widget is used to manage UI state changes.
- 📑 CRUD operations are encapsulated in a `FirestoreService` class, with methods for creating, reading, updating, and deleting notes.
- 🔄 The read operation uses a stream to listen to real-time updates from the database, ensuring the app UI reflects the latest data.
- ✍️ The update and delete operations require the document ID, which is used to identify the specific note to modify or remove.
- 🔗 The video concludes by demonstrating the complete functionality of the notes app, showcasing the successful implementation of CRUD operations with Flutter and Firebase.
Q & A
What are the four most important operations when it comes to databases?
-The four most important operations when it comes to databases are Create, Read, Update, and Delete (CRUD).
How do you create a new project in Firebase?
-To create a new project in Firebase, go to the Firebase console, click on 'Add project', and follow the on-screen instructions.
What is the purpose of the 'flutterfire_cli' tool?
-The 'flutterfire_cli' tool is used to configure Firebase in a Flutter project, including setting up dependencies and initializing Firebase services.
How do you connect a Flutter project to Firebase?
-To connect a Flutter project to Firebase, use the 'flutterfire_cli' tool with the 'configure' command, select the Firebase project, and follow the prompts to set up the necessary configurations.
What does the 'Firebase.initializeApp' method do in a Flutter app?
-The 'Firebase.initializeApp' method in a Flutter app initializes Firebase services and ensures that Firebase is set up and ready to use.
How do you add a new note to the Firestore database in the example app?
-To add a new note to the Firestore database, use the 'add' method on the collection reference, passing in a map containing the note content and a timestamp.
What is a StreamBuilder and how is it used in the Flutter app?
-A StreamBuilder is a Flutter widget that listens to a stream and rebuilds its child based on the latest snapshot from the stream. In the app, it's used to listen to changes in the Firestore database and update the UI with the latest notes.
How do you update a note in the Firestore database?
-To update a note in the Firestore database, use the 'update' method on the document reference, passing in a map containing the fields to update.
How do you delete a note from the Firestore database?
-To delete a note from the Firestore database, use the 'delete' method on the document reference corresponding to the note you want to remove.
What is the purpose of the 'FloatingActionButton' in the Flutter app?
-The 'FloatingActionButton' in the Flutter app serves as a UI element that, when clicked, opens a dialog for the user to input a new note, which can then be added to the Firestore database.
Outlines
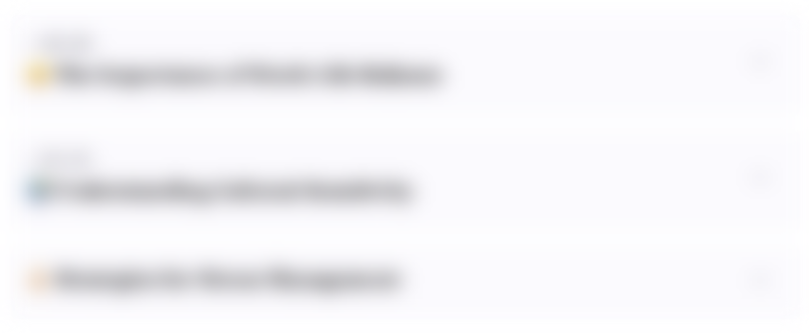
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
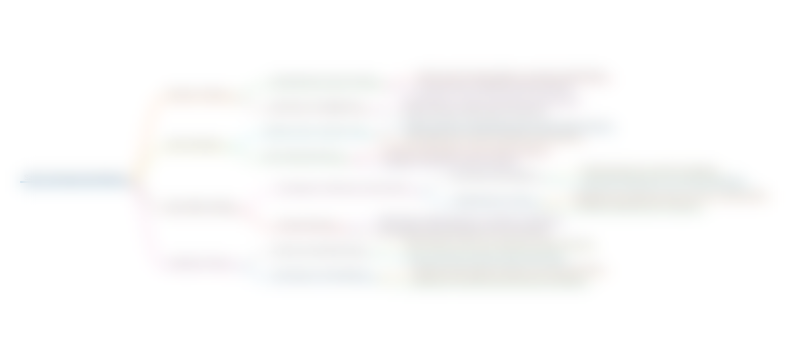
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
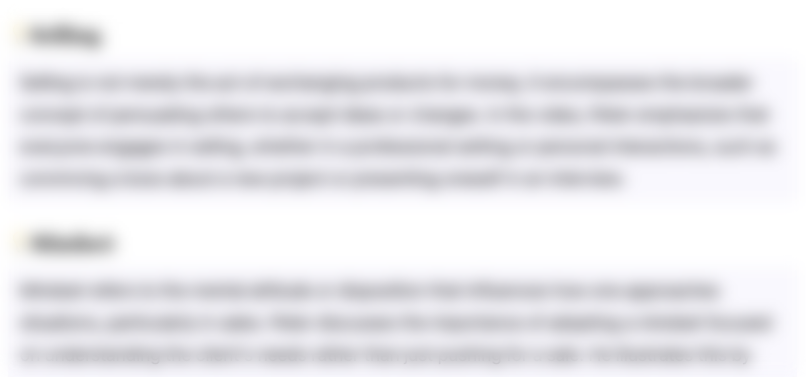
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
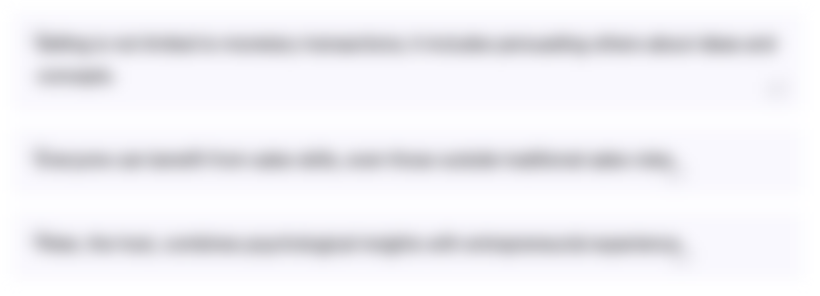
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
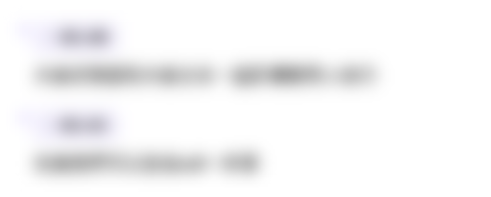
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
5.0 / 5 (0 votes)