Complete CRUD Operation in Asp.Net C# With SQL Server Step by Step
Summary
TLDRThis tutorial guide walks viewers through creating a complete CRUD (Create, Read, Update, Delete) operation in ASP.NET using C# with SQL Server. Starting with setting up a new project, designing the webpage, and creating databases and tables, it progresses to connecting SQL Server with Visual Studio and coding for CRUD functionalities. The video demonstrates inserting records into a database, updating them, and retrieving data to display in a grid view, ensuring a hands-on understanding of database interactions in web development.
Takeaways
- π The tutorial is focused on creating a CRUD (Create, Read, Update, Delete) operation in ASP.NET with C# and SQL Server.
- π οΈ The process starts with creating a new project in Visual Studio and designing the webpage for the CRUD operations.
- π The tutorial covers creating a database and table in SQL Server, including setting up the columns and data types for the 'student info' table.
- π It explains how to connect SQL Server to Visual Studio, which is essential for database operations within an ASP.NET application.
- π The script details the steps to design the user interface with HTML elements like div, table, label, text box, combo box, and button.
- π±οΈ The video demonstrates adding event handlers for the 'Insert' button to perform data insertion into the SQL Server database.
- π The 'Load Records' method is introduced to fetch and display records from the database in a GridView control.
- π The tutorial includes implementing 'Update' and 'Delete' operations with corresponding button click events and SQL command executions.
- π The script also covers how to retrieve and populate data into text boxes and combo boxes based on selected student IDs.
- ποΈ A confirmation message is added before performing delete operations to prevent accidental data loss.
- π The tutorial concludes with successfully demonstrating the CRUD operations and encourages viewers to subscribe for more content.
Q & A
What is the main topic of the tutorial video?
-The main topic of the tutorial video is to demonstrate how to perform a complete CRUD (Create, Read, Update, Delete) operation in ASP.NET using C# and SQL Server.
What are the three main steps mentioned in the video for setting up a project in ASP.NET?
-The three main steps mentioned are creating a new project, designing the webpage, and creating databases and tables.
How does one create a new project in Visual Studio according to the video?
-To create a new project in Visual Studio, one should click on the file menu, select 'New Web Site', choose 'Visual C#', select 'ASP.NET Web Site', set the location to save the website, and provide a project name.
What is the purpose of the GridView control in the context of this tutorial?
-The GridView control is used to display the inserted records from the SQL Server database table in the ASP.NET webpage.
What is the name of the database created in the video?
-The name of the database created in the video is 'programming db'.
What are the data types of the columns in the 'student info' table?
-The data types of the columns in the 'student info' table are Integer for 'student id', NVarChar(50) for 'student name', NVarChar(50) for 'address', and NVarChar(50) for 'contact'.
How is the connection to SQL Server established in the video?
-The connection to SQL Server is established by adding a connection in the Server Explorer of Visual Studio, providing the server name, authentication details, and selecting the database 'programmingdb'.
What is the purpose of the 'SqlCommand' object in the video?
-The 'SqlCommand' object is used to execute SQL commands, such as inserting records into the database.
How does the video demonstrate updating a record in the database?
-The video demonstrates updating a record by using an UPDATE SQL command within a button click event, setting the values for the fields based on user input from the webpage controls.
What is the method used in the video to display records in the GridView instantly after an insert operation?
-The method used to display records instantly is by calling a 'load records' method that fetches data from the database and binds it to the GridView control.
How does the video handle the deletion of records?
-The video handles the deletion of records by using a DELETE SQL command within a button click event, specifying the record to delete based on the 'student id' from a textbox.
What additional feature is added to the delete button in the video to prevent accidental deletions?
-A confirmation message box is added to the delete button using the 'confirm' JavaScript function, which prompts the user to confirm their intention to delete before executing the operation.
Outlines
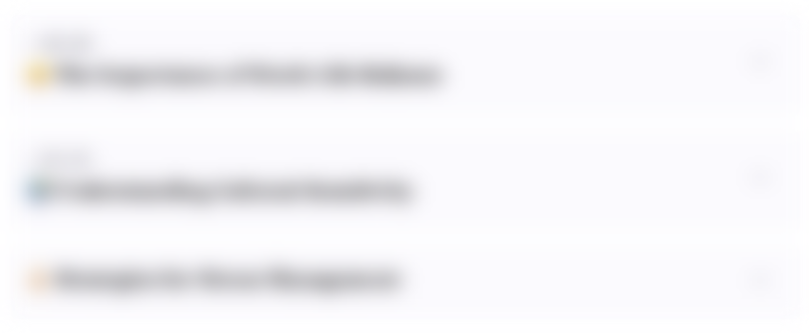
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
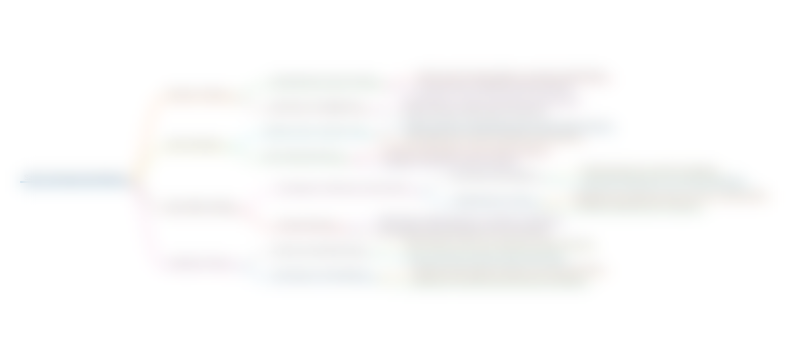
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
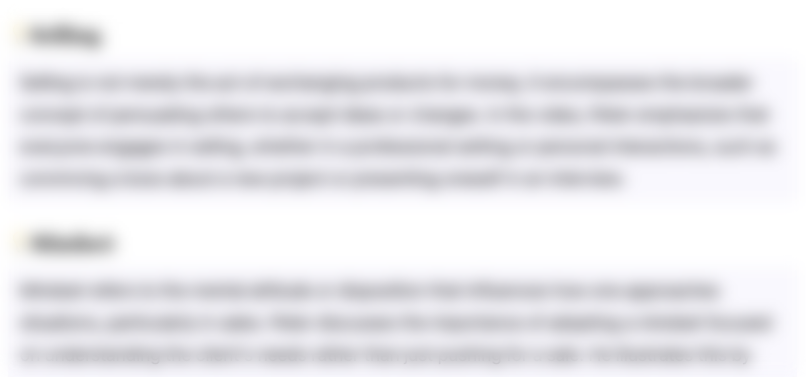
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
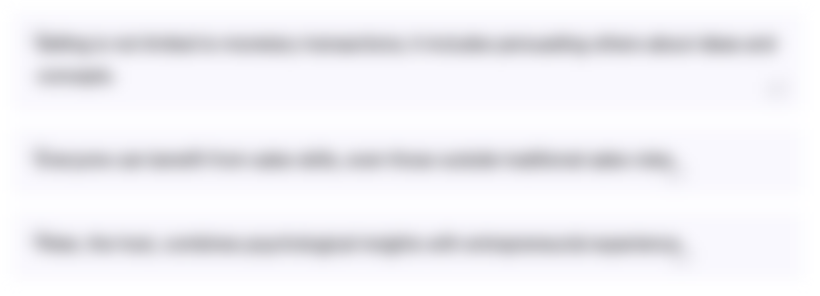
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
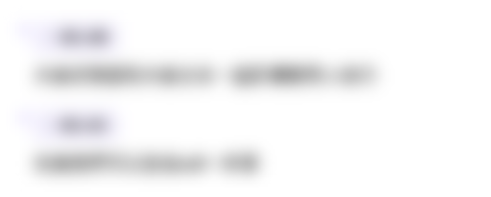
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)