Go 58: io.Reader & io.Writer with Code Examples
Summary
TLDRThe video script provides a comprehensive introduction to the io.Reader and io.Writer interfaces in Golang. It explains the purpose and usage of these interfaces, highlighting their crucial roles in reading from and writing to various data streams. The script dives into common implementations, such as files, strings, and network connections. Through insightful examples, it demonstrates how these interfaces enable code reusability and abstraction, allowing developers to work with different data sources seamlessly. The video promises to equip viewers with a solid understanding of these fundamental interfaces, empowering them to build robust and efficient Go projects.
Takeaways
- 🔑 The io.Reader interface is used for reading data from sources like files, network connections, etc., and has a single Read method that takes a byte slice and returns the number of bytes read and an error.
- ☝️ When using io.Reader, the caller should always process the bytes read before considering the error, handling io.EOF (end of file) appropriately.
- 📚 Common implementations of io.Reader include *os.File for reading files, strings.NewReader for reading strings, bufio.Reader for buffered reading, and http.Request.Body for reading HTTP request bodies.
- 🖥️ The io.Writer interface is used for writing data to destinations like files, network connections, etc., and has a single Write method that takes a byte slice and returns the number of bytes written and an error.
- ✍️ Common implementations of io.Writer include *os.File for writing to files, os.Stdout for writing to standard output, http.ResponseWriter for writing HTTP responses, and bytes.Buffer for writing to memory buffers.
- 🔄 Using these interfaces makes code more generic and reusable, as it can work with any source or destination that implements the respective interface.
- 🧱 The script provides examples of using io.Reader and io.Writer, such as a function to count alphabets in a Reader and a function to write a string to a Writer.
- 🔒 When implementing io.Writer, if the number of bytes written is less than the provided byte slice length, an error should be returned.
- ♻️ It's important to close files and free up resources when done using them, as demonstrated in the script with the defer statement.
- 📖 The script aims to teach Go's standard library interfaces io.Reader and io.Writer, their common implementations, and how to use them effectively in Go programs.
Q & A
What is the io.Reader interface in Go, and what is its purpose?
-The io.Reader interface in Go is a simple interface with one method called Read. Its purpose is to provide a way to read data from different sources, such as files, strings, network connections, and more, using a common interface. This allows for code reusability and abstraction.
What are some common implementations of the io.Reader interface?
-Some common implementations of the io.Reader interface include *os.File (for reading from files), strings.NewReader (for creating a reader from a string), bufio.Reader (for buffered reading), and http.Request.Body (for reading the request body in HTTP requests).
What does the Read method of the io.Reader interface do?
-The Read method of the io.Reader interface reads data from the source and stores it in a provided byte slice. It returns the number of bytes read and an error value (if any). The Go documentation recommends processing the read bytes before considering the error.
How does the io.Reader interface handle the end-of-file (EOF) condition?
-When the reader reaches the end of the data stream, the Read method returns an io.EOF error. However, this is not considered an actual error but rather an indication that the end of the data has been reached. The caller should handle io.EOF as a normal condition rather than an error.
What is the purpose of the io.Writer interface in Go?
-The io.Writer interface serves the opposite purpose of the io.Reader interface. It is used to write data to a destination, such as files, network connections, or standard output. It provides a common interface for writing data from various sources.
What is the Write method of the io.Writer interface, and what are its requirements?
-The Write method of the io.Writer interface takes a slice of bytes as input and writes that data to the destination. It returns the number of bytes written and an error (if any). If the number of bytes written is less than the length of the provided byte slice, it should return an error indicating an error state. The provided byte slice should not be modified inside the Write method.
Give some examples of common implementations of the io.Writer interface.
-Common implementations of the io.Writer interface include *os.File (for writing to files), os.Stdout (for writing to standard output), http.ResponseWriter (for writing the response body in HTTP requests), and bytes.Buffer (for writing to an in-memory byte buffer).
How does the use of interfaces like io.Reader and io.Writer promote code reusability in Go?
-By using interfaces like io.Reader and io.Writer, Go code can be written in a way that abstracts the underlying data source or destination. This allows the same code to work with different types of data sources or destinations, as long as they implement the respective interface. This promotes code reusability and reduces duplication.
In the provided example, how does the countAlphabets function utilize the io.Reader interface?
-The countAlphabets function takes an io.Reader as input and counts the number of alphabetic characters (letters) in the data read from that reader. It uses the Read method of the io.Reader to read data into a buffer and then iterates over the buffer to count the alphabetic characters. This function can work with any data source that implements the io.Reader interface, such as files or strings.
What is the purpose of the writeString function demonstrated in the script, and how does it utilize the io.Writer interface?
-The writeString function takes a string and an io.Writer as input and writes the string to the provided writer destination. It utilizes the Write method of the io.Writer interface to write the byte representation of the string to the destination. This function can be used to write data to any destination that implements the io.Writer interface, such as files or network connections.
Outlines
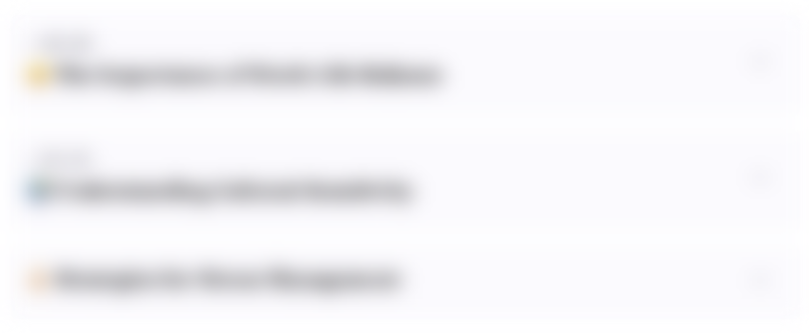
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
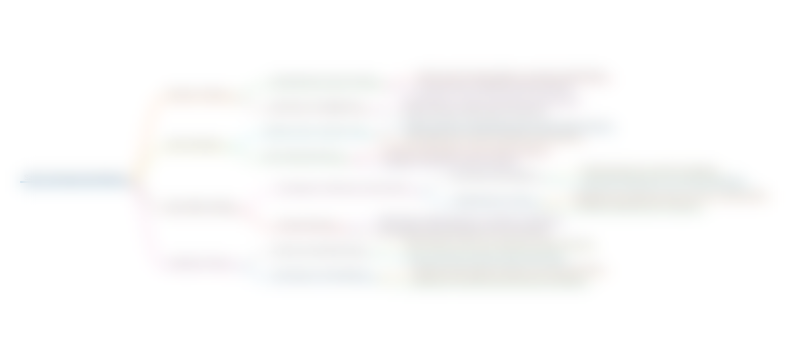
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
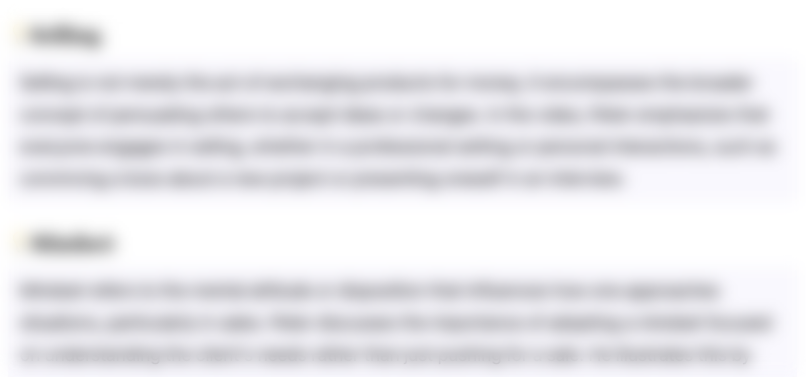
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
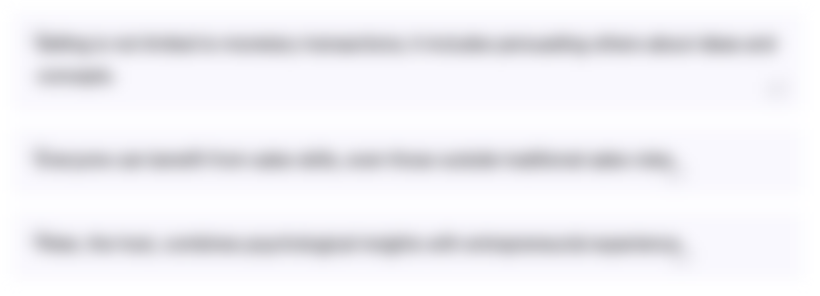
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
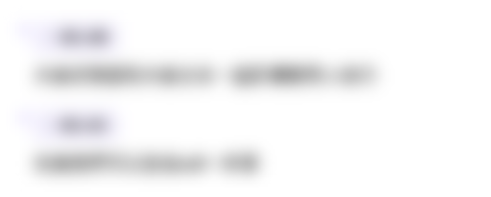
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
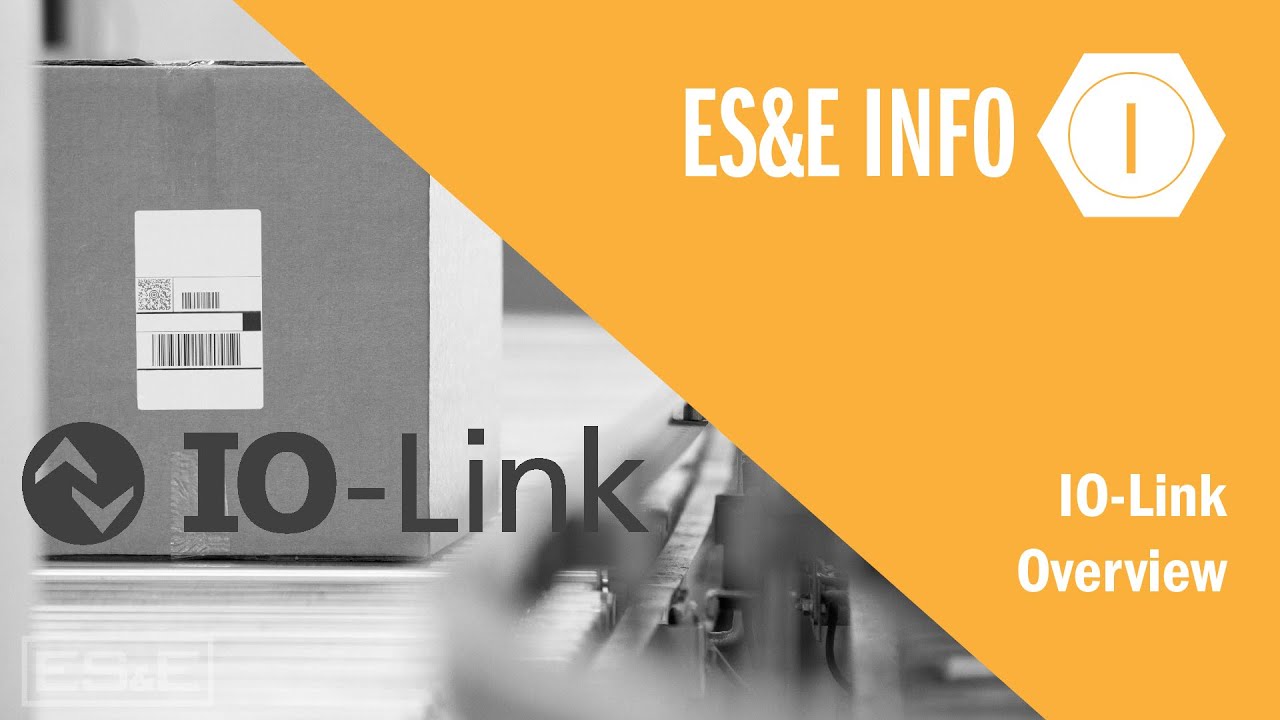
IO-Link Overview

Set up a Mettler Toledo SLP33 to a 5032 IO-Link Master
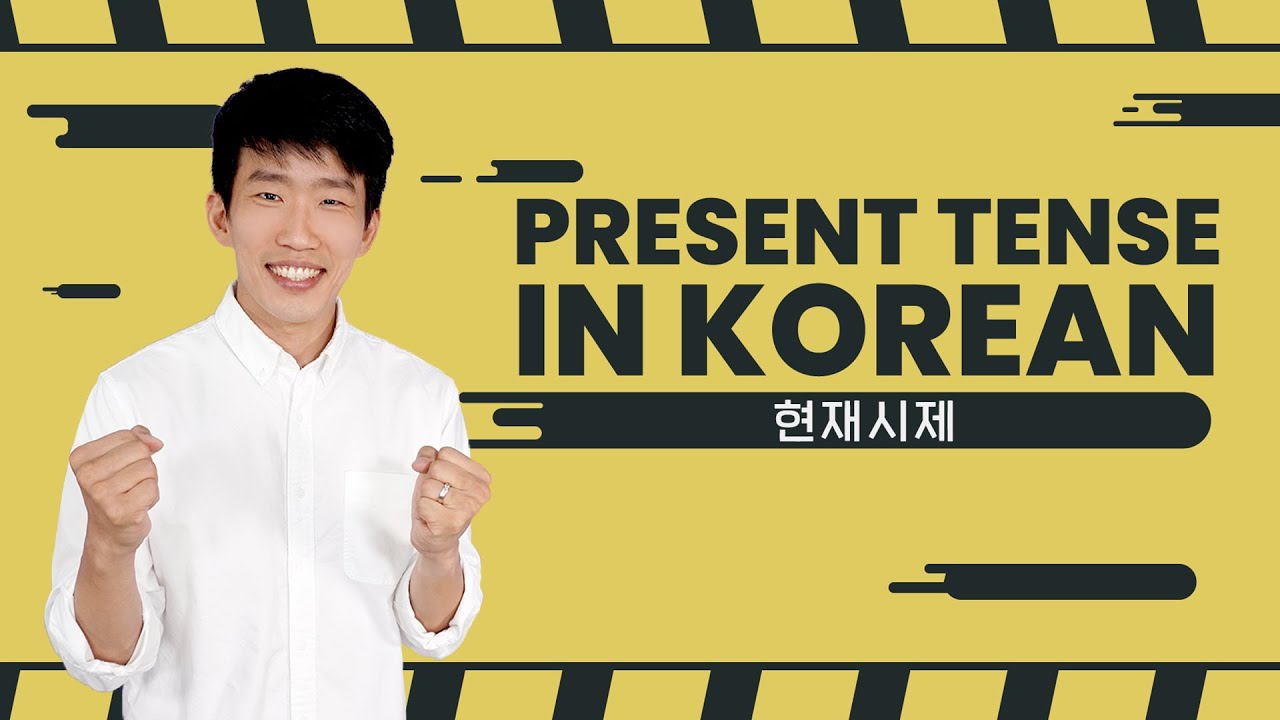
How to make Korean sentences in the present tense (for beginners)
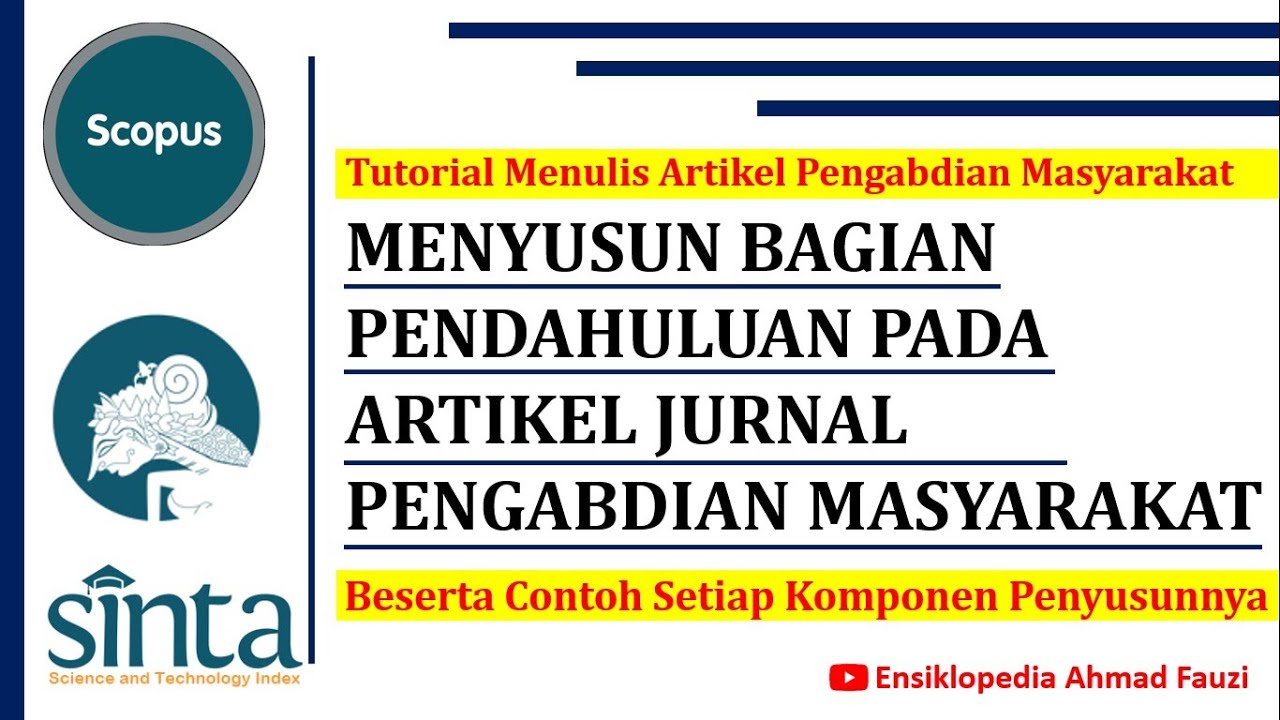
Membuat Pendahuluan Artikel Pengabdian yang Menawan: Komponen Utama dan Teknik Penulisan
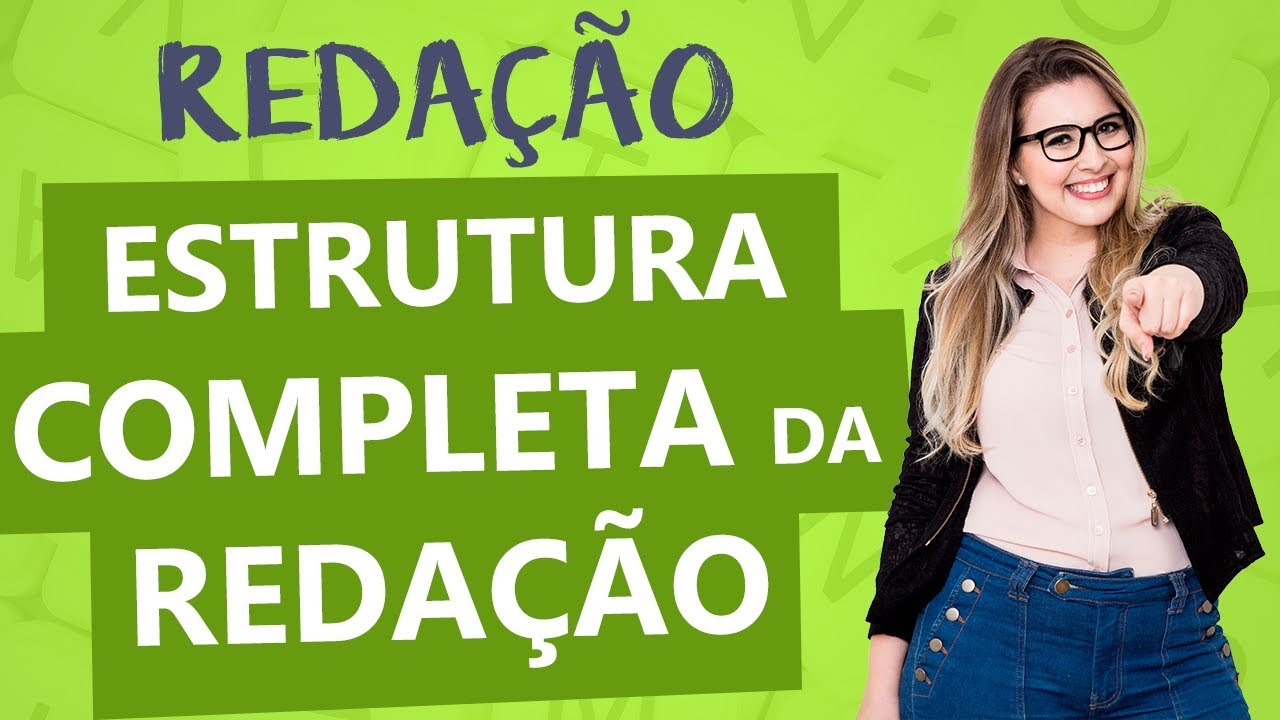
ESTRUTURA DA REDAÇÃO COMPLETA: TEXTO DISSERTATIVO - Aula 2 - Profa. Pamba
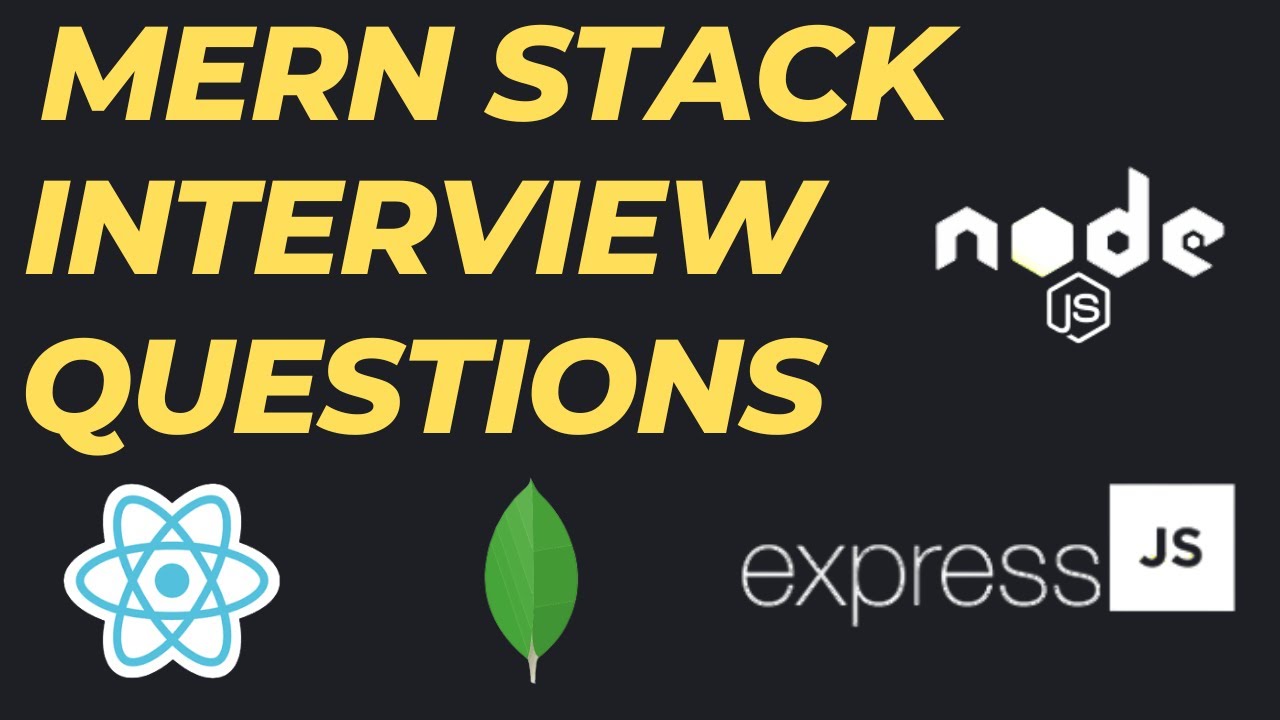
MERN Stack Interview Questions | Node Js Interview Questions | | Node | MongoDB | React | Express JS
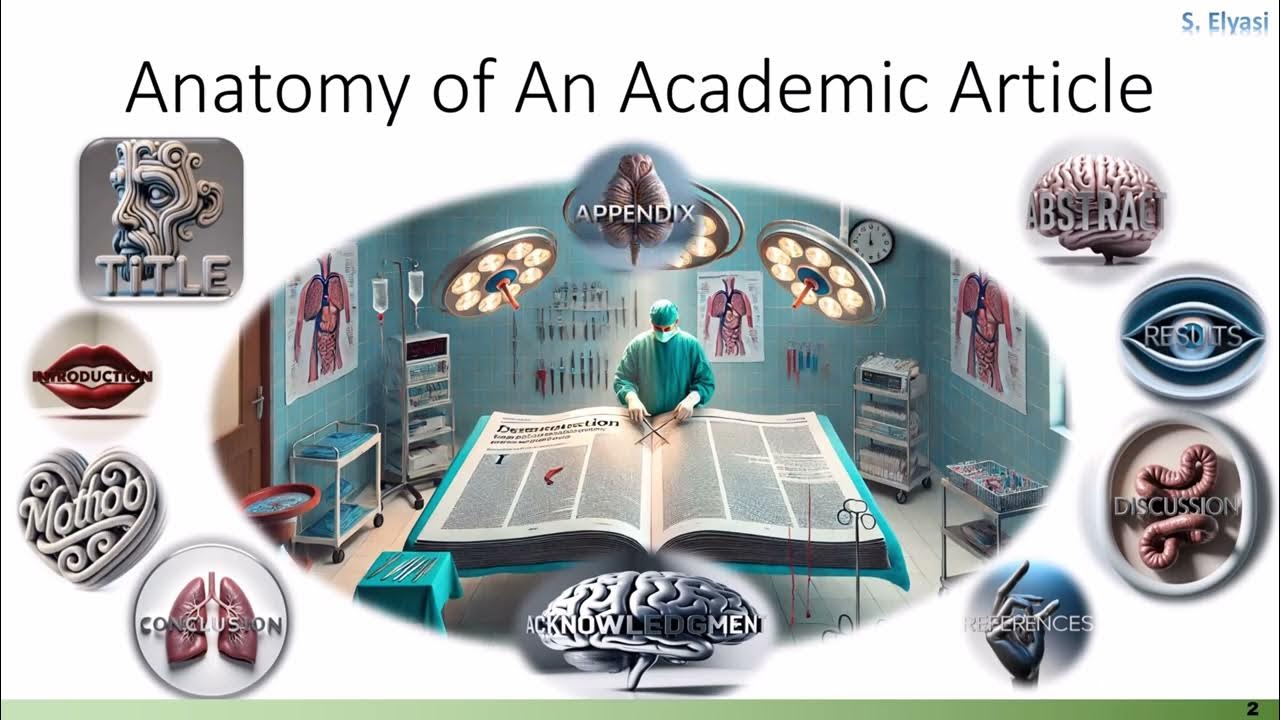
Anatomy Of An Academic Article Part 1 Overview and Title
5.0 / 5 (0 votes)