JavaScript Strings
Summary
TLDRThis video script explores the intricacies of the String object in JavaScript, highlighting the difference between string primitives and string objects. It demonstrates properties like 'length' and methods such as 'includes', 'startsWith', 'endsWith', 'indexOf', 'replace', 'toUpperCase', 'toLowerCase', 'trim', and 'split'. The tutorial also covers escape notation and introduces template literals, offering a comprehensive guide for both beginners and experienced developers to master string manipulation in JavaScript.
Takeaways
- 📝 JavaScript has two types of strings: primitive strings and string objects. Primitive strings don't have properties or methods, but string objects do.
- 🔍 The JavaScript engine automatically wraps primitive strings with a string object when using dot notation, allowing access to string methods.
- 🔎 To learn about string methods, it's recommended to refer to the official documentation on developer.mozilla.org.
- 📊 The 'length' property of a string returns the number of characters, which is useful for input validation and character limits.
- 👉 Accessing a character at a specific index in a string can be done using square brackets, like 'message[0]'.
- 🔍 The 'includes' method checks if a string contains a specific word or character, and is case-sensitive.
- 📚 The 'startsWith' and 'endsWith' methods determine if a string begins or ends with a specified sequence, also case-sensitive.
- 🔑 The 'indexOf' method finds the index of a given character or string within a string.
- ✂️ The 'replace' method is used to replace parts of a string, returning a new string without modifying the original.
- 🔠 The 'toUpperCase' and 'toLowerCase' methods convert all characters in a string to uppercase or lowercase, respectively.
- 📝 The 'trim' method removes whitespace from both ends of a string, with variations like 'trimLeft' and 'trimRight'.
- 🚫 Escape notation is necessary for special characters in strings, such as using a backslash to escape a single quote.
- 📑 The 'split' method breaks a string into an array of words or substrings based on a specified delimiter.
- 📚 Template literals are a feature in JavaScript that allow for multiline strings and embedded expressions.
Q & A
What is the difference between a string primitive and a string object in JavaScript?
-In JavaScript, a string primitive is a basic data type that doesn't have properties or methods, while a string object is a more complex data structure that includes properties and methods. When a string primitive is accessed using dot notation, the JavaScript engine internally wraps it with a string object, allowing the use of string methods.
How can you create a string object in JavaScript?
-You can create a string object in JavaScript by using the String constructor function with the 'new' operator. For example, `let anotherString = new String('my string');`.
What is the purpose of the 'length' property in strings?
-The 'length' property returns the number of characters in a string, which is useful for validating user input length, such as ensuring a user types a minimum number of characters or not exceeding a certain limit.
How do you access a character at a specific index in a string?
-You can access a character at a specific index in a string using square brackets and the index number. For example, `message[0]` would return the first character of the string 'message'.
What does the 'includes' method do in strings?
-The 'includes' method checks if a string contains a specified sequence of characters, returning true or false. It is case-sensitive.
How can you determine if a string starts with a specific character or string?
-You can use the 'startsWith' method to determine if a string begins with a specified character or string. It is also case-sensitive.
What is the 'endsWith' method used for in strings?
-The 'endsWith' method checks if a string ends with a specified character or string and returns true or false.
How can you find the index of a character or substring within a string?
-You can use the 'indexOf' method to find the index of a character or substring within a string.
What happens when you use the 'replace' method in strings?
-The 'replace' method is used to replace a part of a string with another string. It returns a new string and does not modify the original string.
What does the 'toUpperCase' method do to a string?
-The 'toUpperCase' method converts all the characters in a string to uppercase letters and returns a new string.
What is the purpose of the 'trim' method in strings?
-The 'trim' method removes whitespace from both ends of a string. It is useful for cleaning up user input or when working with strings that have unwanted whitespace.
Can you explain the concept of escape notation in strings?
-Escape notation is used to encode special characters in strings. For example, to include a single quote in a string, you would use '\' followed by the single quote to escape it, preventing the JavaScript engine from interpreting it as the end of the string.
What is the 'split' method used for in strings?
-The 'split' method is used to split a string into an array of substrings based on a specified separator character. Each element in the resulting array is a word from the original string.
Outlines
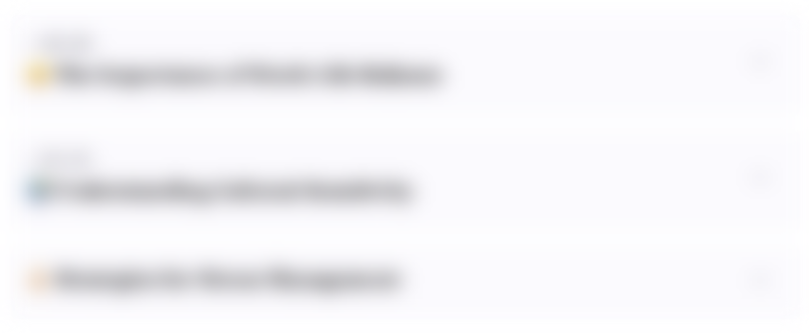
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
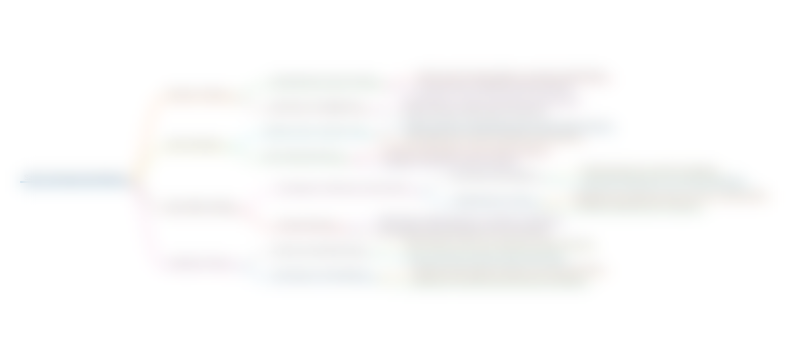
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
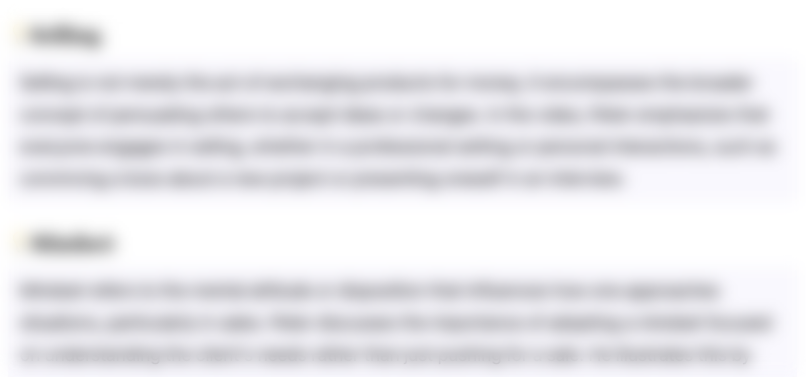
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
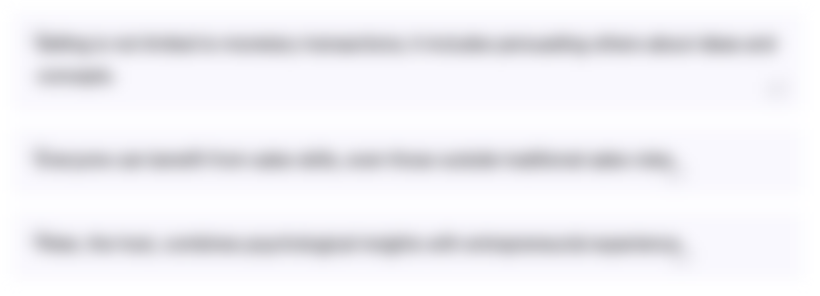
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
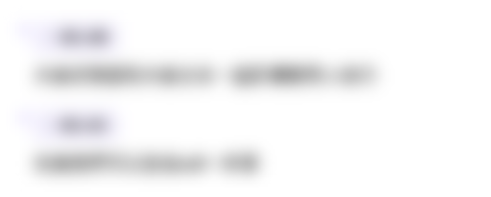
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
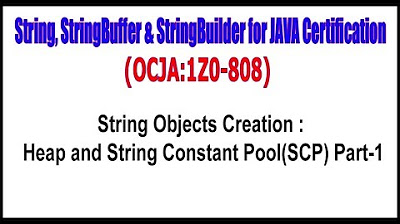
OCJA(1Z0 - 808) || String Objects Creation Heap and String Constant Pool (SCP) Part - 1
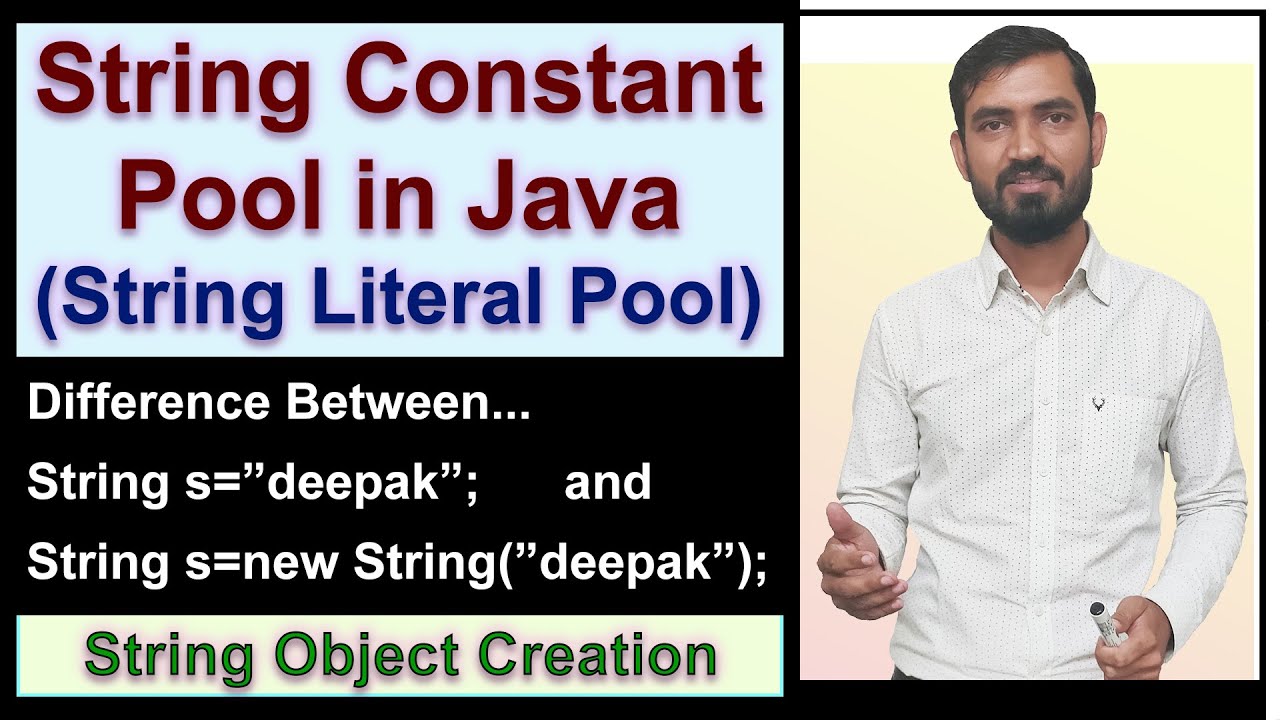
String Constant Pool in Java || String Object Creation in Java
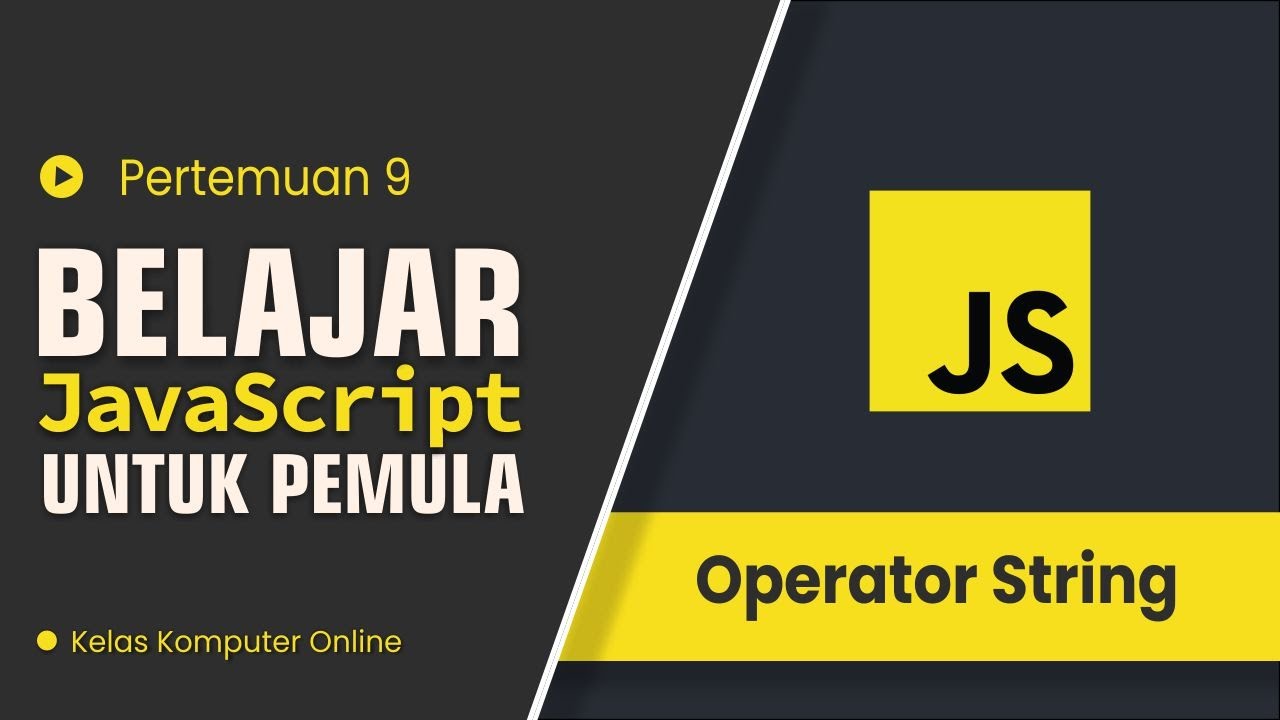
Learn JavaScript Programming Basics: String Operators in JavaScript
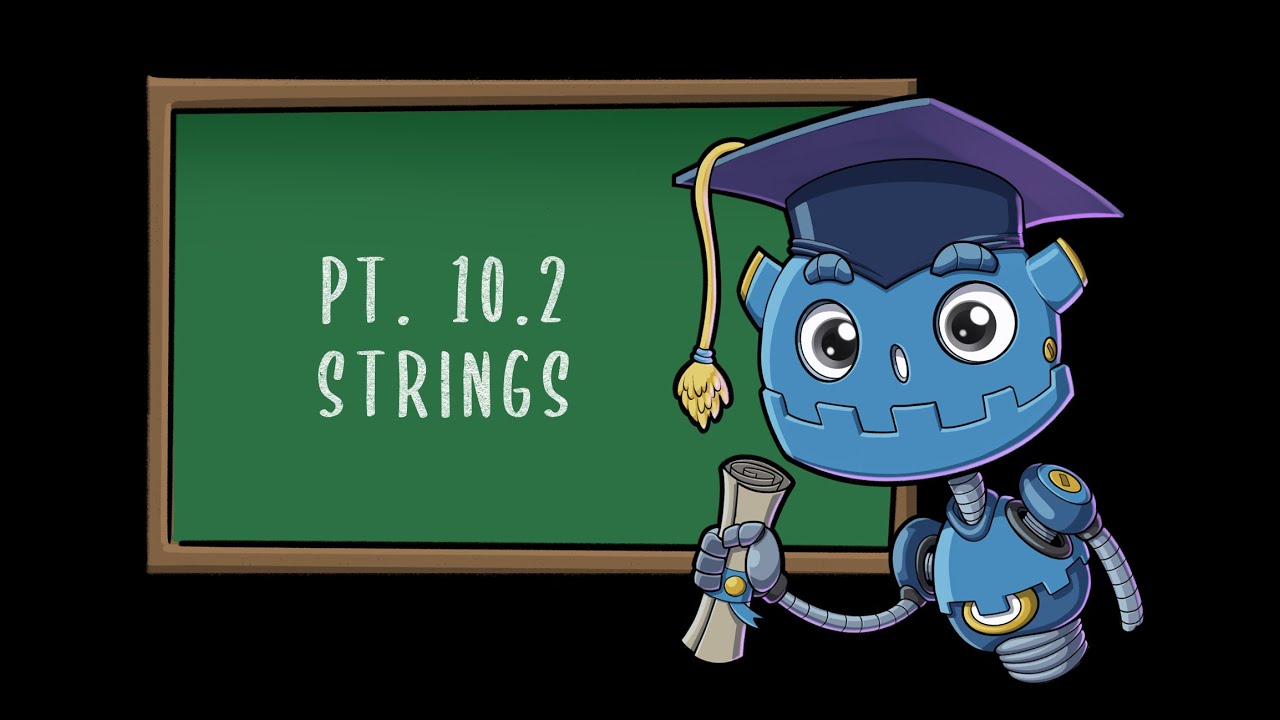
Strings (Basics of Memory) | Godot GDScript Tutorial | Ep 10.2
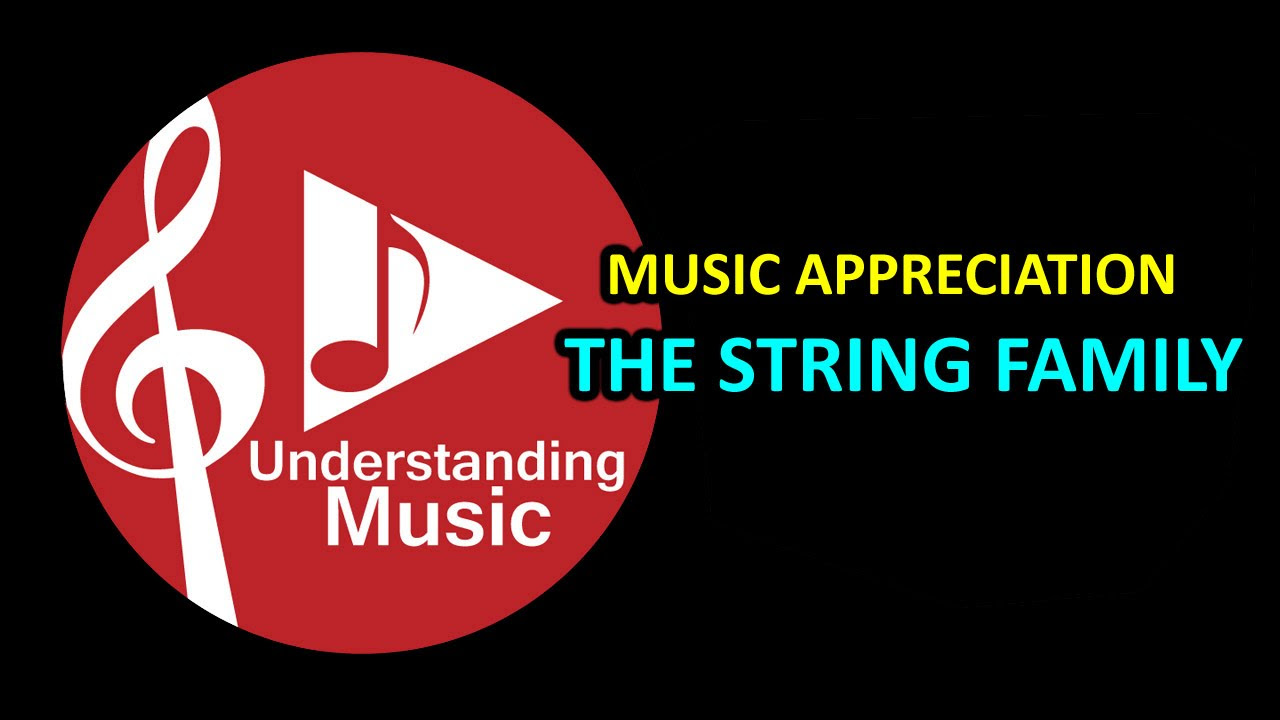
Instruments of the Orchestra-STRINGS

C++ Strings | What is String? full Explanation
5.0 / 5 (0 votes)