03 - Conditional A - Python for Everybody Course
Summary
TLDRThis video script introduces the concept of conditional execution in Python, focusing on the 'if' statement as a decision-making tool. It explains the syntax and usage of the 'if' statement, including comparison operators and the importance of proper indentation. The script also touches on nested 'if' statements and the 'if-else' structure, illustrating how to control the flow of a program based on conditions. The emphasis is on clear code structure and the significance of indentation in Python.
Takeaways
- 👋 Hello and welcome to chapter three, Conditional Execution.
- 📜 In conditional execution, we meet the if statement, which allows Python to make decisions based on conditions.
- 🔄 Sequential code executes tasks in order, but with if statements, code can branch and make decisions.
- ❓ The if statement checks a condition and executes the indented block of code if the condition is true.
- 📐 Python uses indentation to define the scope of if statements and other blocks of code.
- 🔢 Comparison operators like <, <=, ==, >=, >, and != are used to form conditions in if statements.
- 📋 Multiple lines can be included in the indented block of an if statement.
- ⚠️ Proper indentation is crucial in Python to avoid syntax errors.
- 🔁 Nested blocks and multiple levels of indentation are supported in Python.
- ↔️ Two-branch if statements use if and else to provide alternative paths of execution based on conditions.
Q & A
What is the main purpose of the 'if' statement in Python?
-The 'if' statement in Python is used for conditional execution, allowing the program to make decisions based on a given condition, and to execute different blocks of code depending on whether the condition is true or false.
How does Python determine whether to execute the code within an 'if' statement?
-Python checks the condition specified in the 'if' statement. If the condition evaluates to true, the code within the indented block following the 'if' statement is executed. If the condition is false, the code is skipped.
What is the significance of the colon (:) in an 'if' statement?
-The colon (:) in an 'if' statement signifies the end of the 'if' statement declaration and indicates the start of the indented block of code that will be executed if the condition is true.
Can the value of a variable be changed by evaluating it in an 'if' statement condition?
-No, evaluating a variable in an 'if' statement condition does not change the value of the variable. The condition is merely a check and does not have side effects on the variable's value.
What are the different comparison operators used in 'if' statement conditions?
-The comparison operators used in 'if' statement conditions include less than (<), less than or equal to (<=), equal to (==), greater than or equal to (>=), greater than (>), and not equal to (!=).
What is the difference between the assignment operator and the equality check in Python?
-The assignment operator in Python is a single equals sign (=), used to assign a value to a variable. The equality check, on the other hand, uses a double equals sign (==) to check if two values are equal.
What is the shorthand syntax for an 'if' statement when there is only one line of code to execute?
-In Python, if there is only one line of code to execute within an 'if' statement, the line can be written on the same line as the condition, following the colon (:) and indented appropriately.
Why is indentation important in Python?
-Indentation is important in Python because it is used to define the scope and structure of code blocks. Incorrect indentation can lead to syntax errors and affect the execution flow of the program.
How does Python handle tabs and spaces for indentation?
-Python allows the use of spaces for indentation but can get confused if tabs and spaces are mixed. It is recommended to use spaces, typically four spaces per indent level, to avoid inconsistencies and errors.
What is a nested block in Python?
-A nested block in Python is an inner block of code that is indented within an outer block. This allows for complex conditional or looping structures, where the inner block is executed only when the conditions of the outer block are met.
What is the difference between a one-branch 'if' statement and an 'if-else' statement?
-A one-branch 'if' statement executes a block of code only if the condition is true. An 'if-else' statement, on the other hand, provides two branches: one that executes if the condition is true (the 'if' branch), and another that executes if the condition is false (the 'else' branch).
Outlines
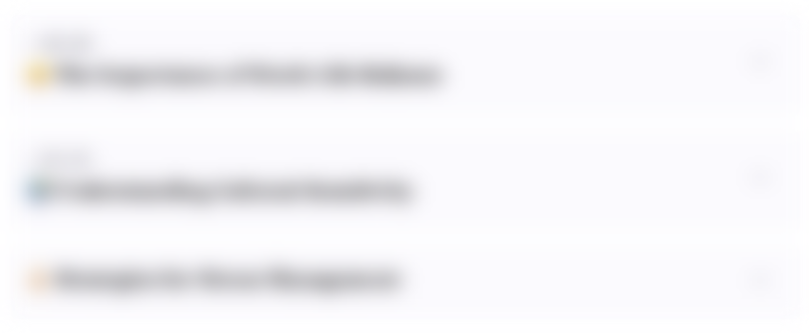
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
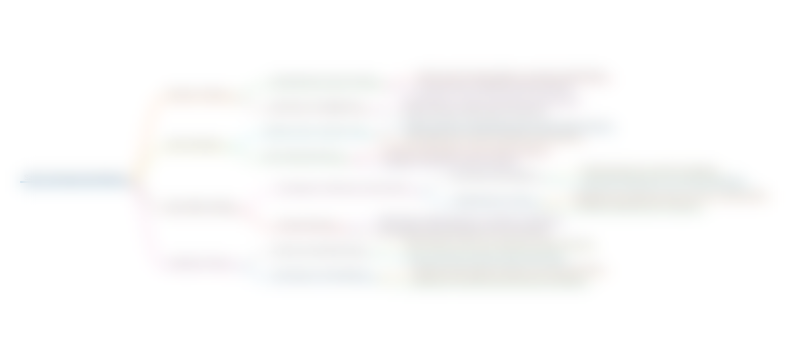
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
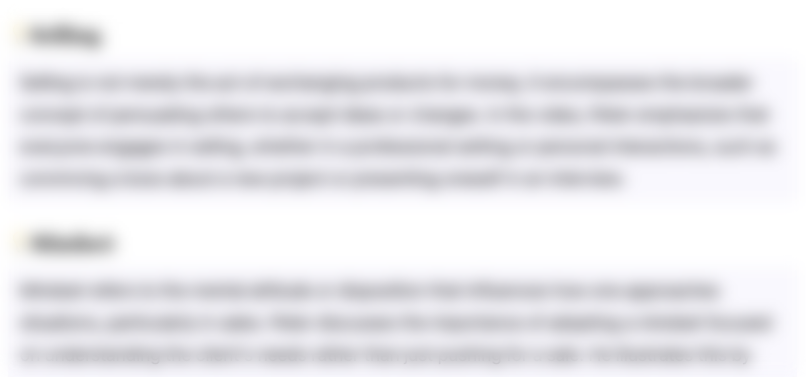
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
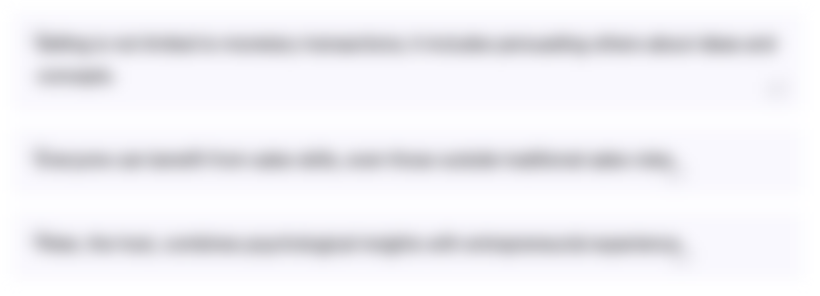
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
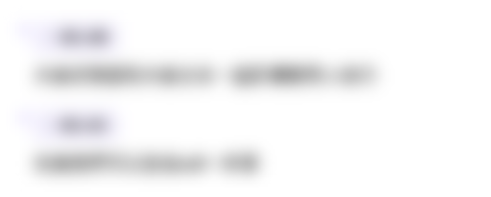
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
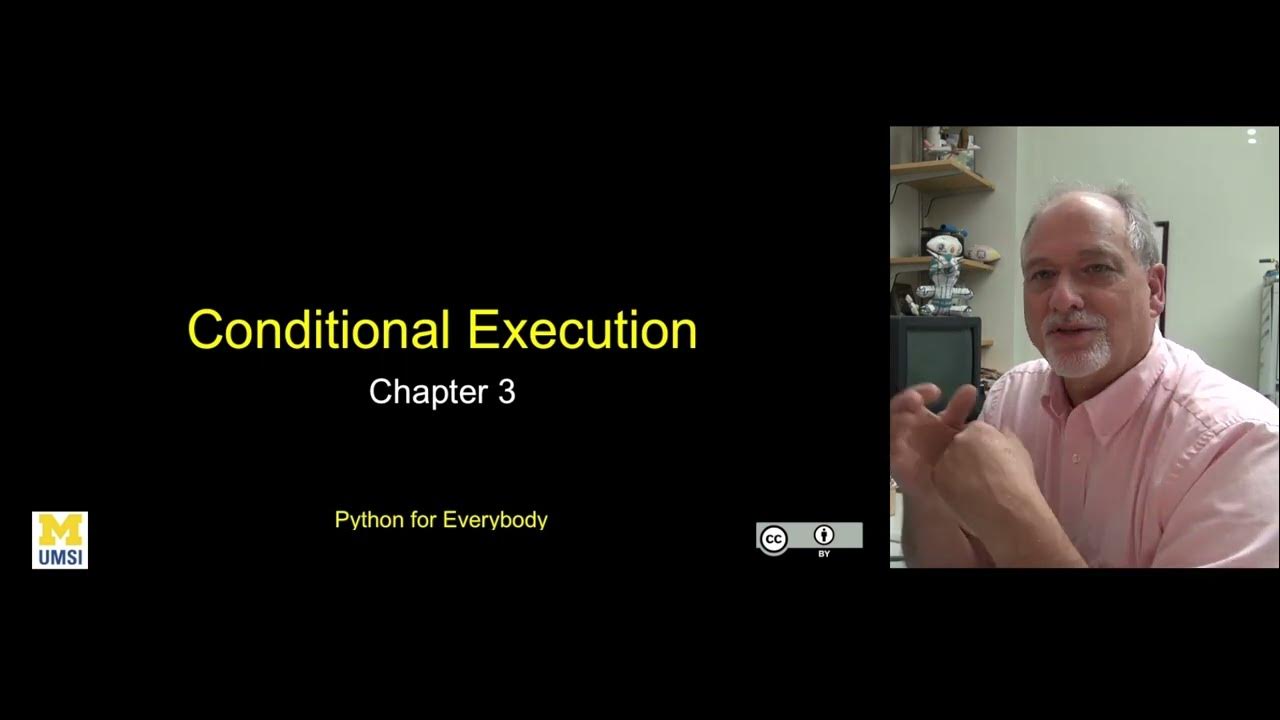
PY4E - Conditionals (Chapter 3 Part 1)
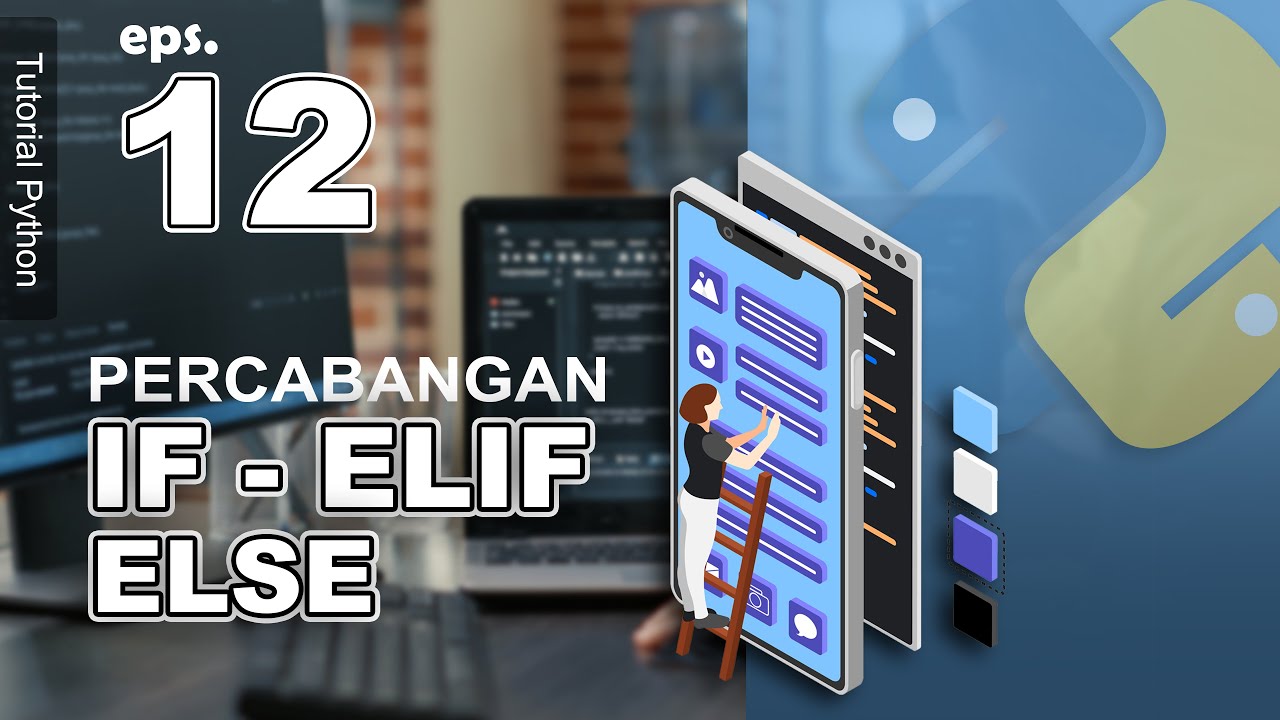
12 - IF - ELIF- ELSE (Conditional Statement) Branching - Indonesian Python Tutorial
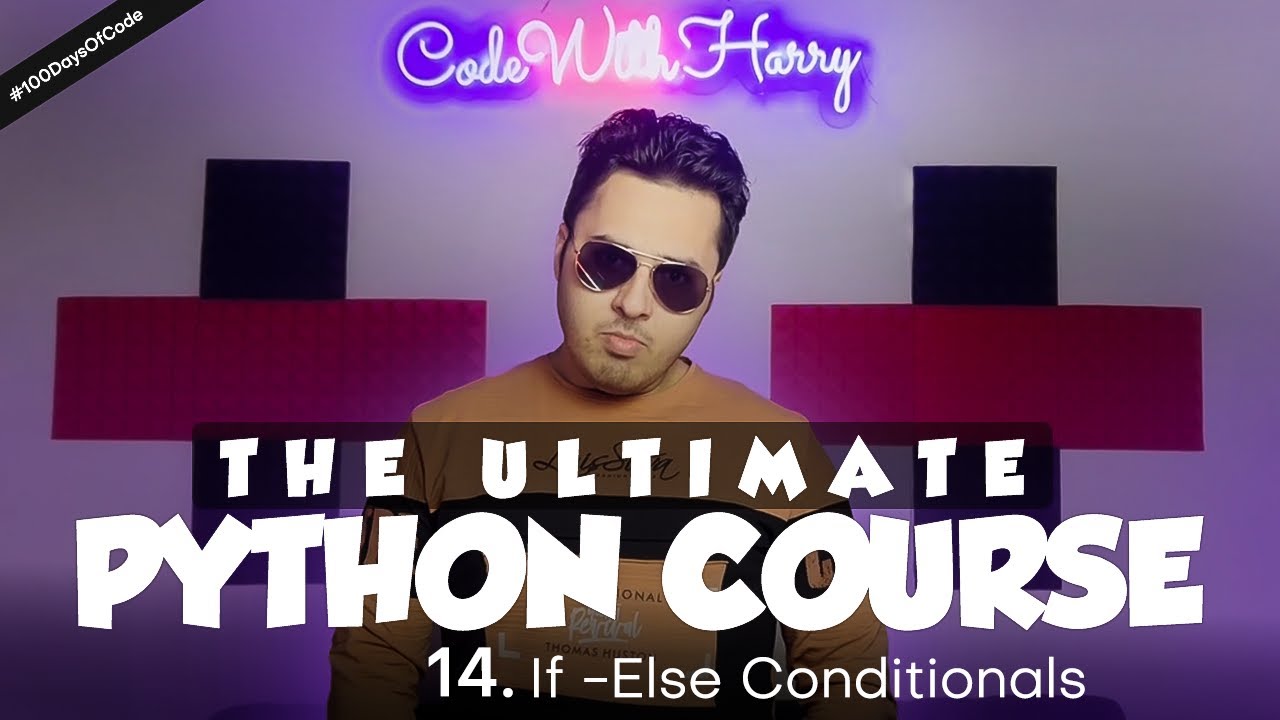
If Else Conditional Statements in Python | Python Tutorial - Day #14
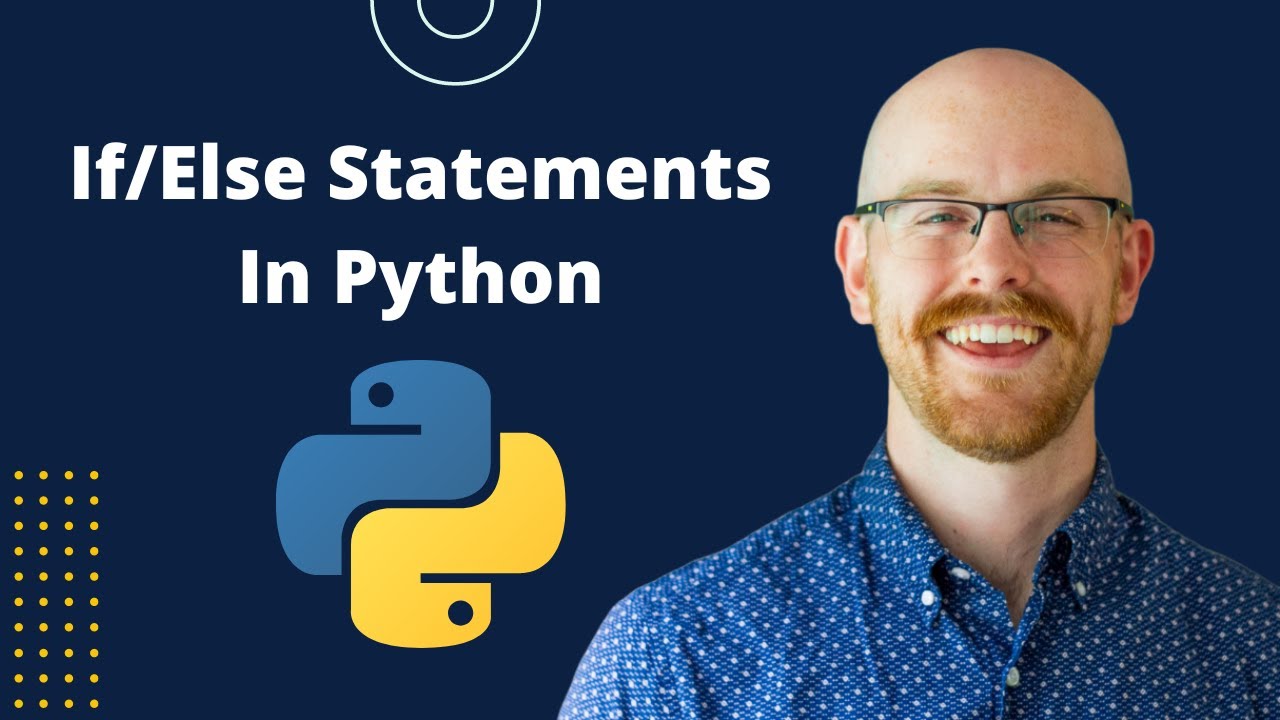
If Else Statements in Python | Python for Beginners

Minggu 3 - Eksekusi Kondisional
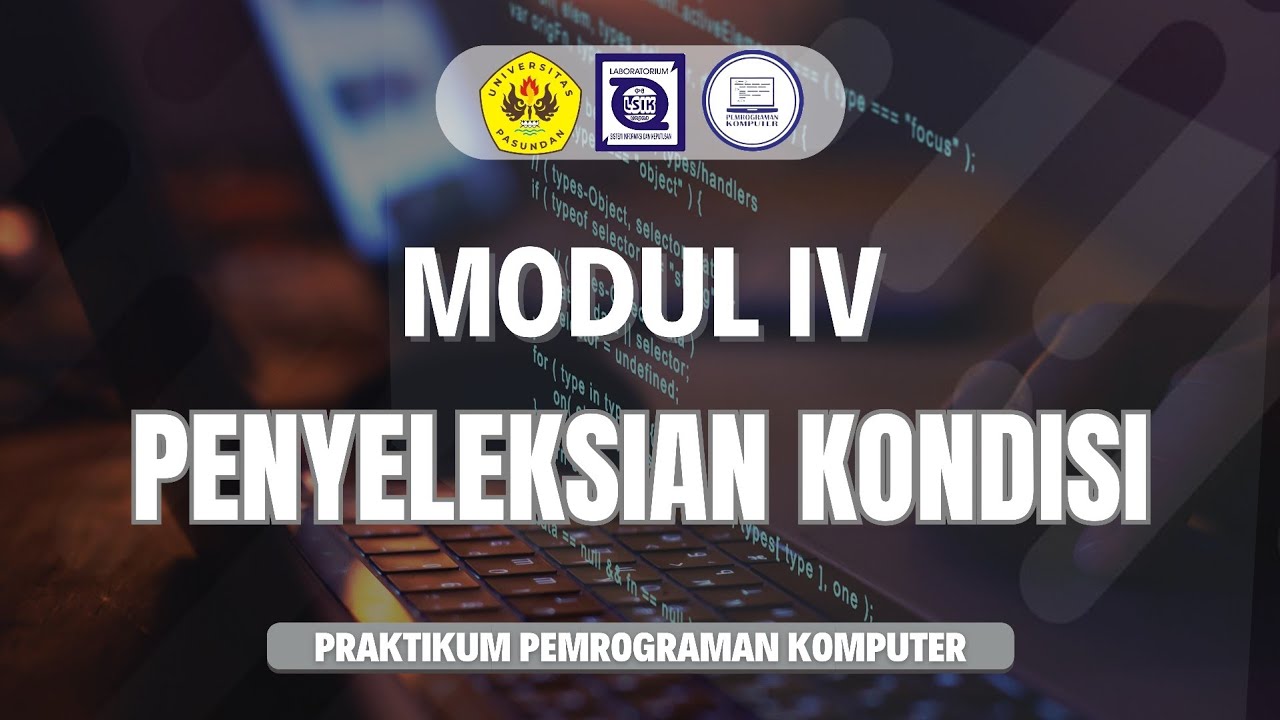
MODUL IV PENYELEKSIAN KONDISI - PRAKTIKUM PENYELEKSIAN KONDISI
5.0 / 5 (0 votes)