C_76 Pointers in C- part 6 | Pointer Arithmetic (Subtraction) with program
Summary
TLDRThe video script discusses learning programming in C, focusing on pointer variables, pointer arithmetic, and how to manipulate pointers to access array elements. It explains the concepts of pointer subtraction, pointer addition, and how to use pointers to perform operations on arrays, emphasizing the importance of understanding pointer arithmetic for effective programming.
Takeaways
- 😀 The video discusses learning programming in C, specifically focusing on pointers and how to handle them in various scenarios.
- 🔍 It explains how to declare and initialize pointers, including the difference between pointer variables and actual pointers.
- 📚 The script covers how to perform operations on pointers, such as pointer arithmetic and how to access values through pointers.
- 👨🏫 It demonstrates how to use pointers to access array elements, including how to calculate the address of elements and how pointers can be used to iterate through arrays.
- 🔢 The video clarifies the concept of pointer arithmetic, showing how to add or subtract integers from pointers to move them through memory.
- 💡 It discusses the importance of understanding the size of data types when performing pointer arithmetic to avoid undefined behavior.
- 📌 The script highlights the use of pointers in functions, explaining how to pass pointers to functions and how to return pointers from functions.
- 🚀 The video also touches on the concept of pointer subtraction, explaining how to find the difference between two pointers and what this difference represents in terms of memory.
- 🛠️ It provides examples of pointer operations, including how to increment and decrement pointers and how these operations can be used in practical programming.
- 🔎 The script emphasizes the need for careful handling of pointers to avoid issues like segmentation faults and undefined behavior, especially when dealing with pointer arithmetic and memory allocation.
Q & A
What is the main topic being discussed in the script?
-The main topic discussed in the script is the concept of pointers in programming, specifically how to handle and manipulate pointer variables in C.
What is a pointer variable?
-A pointer variable is a variable that stores the memory address of another variable. It is used to indirectly access the value stored at the memory location it points to.
What is the difference between a pointer variable and an integer variable?
-A pointer variable stores the memory address of another variable, while an integer variable stores a numerical value. Pointers are used to access and manipulate memory addresses, whereas integers are used to store and perform arithmetic operations on numbers.
How can you assign the address of an integer variable to a pointer variable?
-You can assign the address of an integer variable to a pointer variable using the address-of operator (&). For example, `int *p = &a;` assigns the address of `a` to the pointer `p`.
What is the purpose of pointer arithmetic?
-Pointer arithmetic is used to move a pointer to different memory locations. It allows programmers to access elements in arrays or other data structures by incrementing or decrementing the pointer.
How can you find the difference between two pointers?
-The difference between two pointers can be found by subtracting one pointer from another. This gives the number of elements between the two pointers, considering the size of the data type they point to.
What is the significance of pointer subtraction?
-Pointer subtraction is significant because it helps in determining the number of elements between two pointers. This is useful when working with arrays or other data structures where you need to calculate offsets.
Can you use pointer arithmetic with different data types?
-Pointer arithmetic is generally performed with pointers of the same data type. Mixing pointers of different data types can lead to undefined behavior or incorrect results.
What happens when you subtract one pointer from another?
-When you subtract one pointer from another, the result is the number of elements between the two pointers, not the difference in their memory addresses. The result is an integer value.
How can you access the value pointed to by a pointer?
-You can access the value pointed to by a pointer using the dereference operator (*). For example, `int value = *p;` retrieves the value stored at the memory address pointed to by `p`.
What is the relationship between pointer variables and memory addresses?
-Pointer variables hold the memory addresses of other variables. They act as a reference or link to the memory location where the data is stored, allowing indirect access to the data.
Outlines
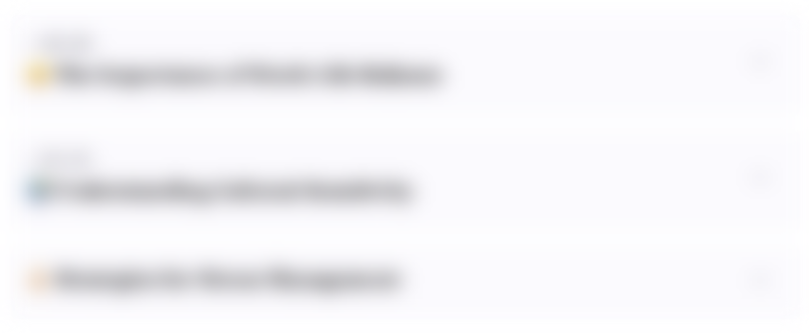
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
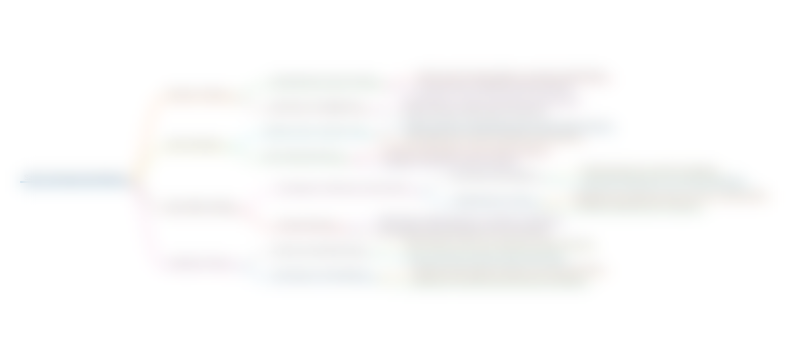
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
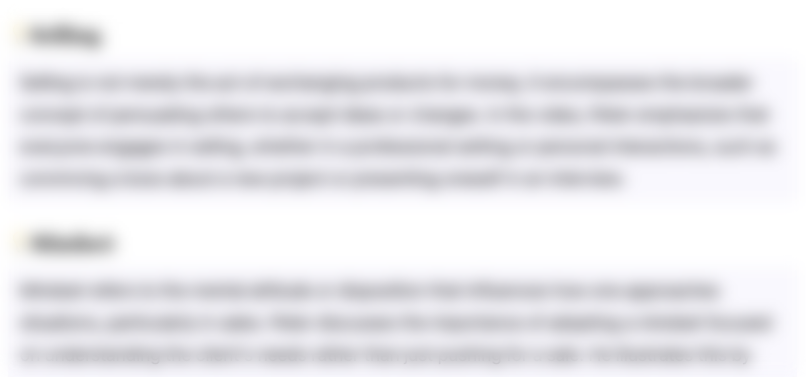
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
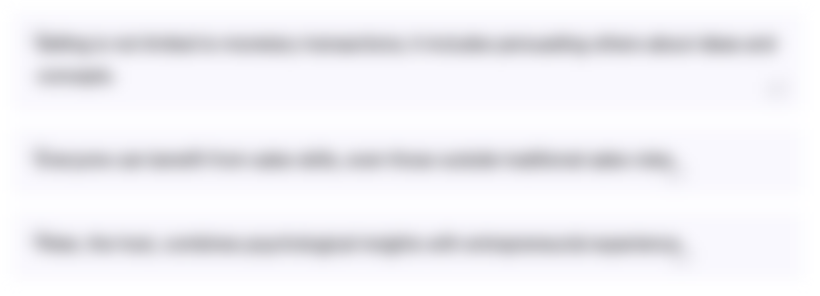
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
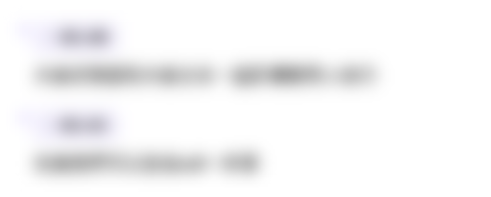
此内容仅限付费用户访问。 请升级后访问。
立即升级5.0 / 5 (0 votes)