#16 Interrupts Part-1: What are interrupts, and how they work
Summary
TLDRIn this lesson, Miro Samek introduces the concept of interrupts in embedded systems programming. Using the TivaC MCU, he demonstrates how interrupts work, particularly through the SysTick timer. The tutorial covers setting up the SysTick timer to generate interrupts at specified intervals, replacing inefficient polling with an interrupt-driven approach. Through this hands-on approach, users learn how interrupts preempt ongoing tasks and how to use them effectively in embedded programming. The lesson concludes with practical code modifications, setting up the interrupt handler, and testing the system with a blinking LED.
Takeaways
- 😀 Interrupts are mechanisms in microprocessors that allow them to respond to specific events or conditions without constant polling, such as user actions or timeouts.
- 😀 Polling involves checking for a condition constantly, which ties up the CPU and prevents it from doing other tasks.
- 😀 Interrupts allow the CPU to perform other work while waiting for an event to occur, similar to setting an alarm clock instead of staying awake to check the time.
- 😀 The TivaC MCU has a SysTick timer, a hardware peripheral that generates interrupts at specific time intervals.
- 😀 The SysTick timer uses a 24-bit down-counter and can trigger an interrupt when it reaches zero, after which it reloads from a predefined value.
- 😀 Interrupts are asynchronous to the executing program and occur independently of the CPU's instruction cycle.
- 😀 The ARM Cortex-M processor features preemption, where an interrupt can cause the CPU to stop its current instruction and handle the interrupt instead.
- 😀 To configure the SysTick timer to trigger interrupts, you must write the appropriate values into the SysTick control and load registers, with the interval based on the CPU clock speed.
- 😀 The program uses an interrupt handler to toggle the red LED in response to SysTick interrupts, replacing the old polling method.
- 😀 To enable interrupts in the CPU, the PRIMASK bit must be cleared, which is done through the function `__enable_interrupt()` in the code.
- 😀 The next lesson will explore preemption in more detail and compare how interrupts work on ARM Cortex-M versus MSP430 processors.
Q & A
What is the main focus of the lesson in the video?
-The main focus of the lesson is to introduce and explain interrupts in embedded systems, particularly in the context of the TivaC MCU. The lesson explains how interrupts work, how they are set up, and how to replace polling with interrupts for more efficient program execution.
What is the problem with using busy-wait polling for delays in microcontroller programs?
-Busy-wait polling, like the one used in the delay() function, ties up the CPU completely, making it unavailable for any other work. This inefficiency is akin to spending the whole night counting clock ticks instead of setting an alarm, which would allow for other tasks to be performed.
What is an interrupt and why is it called that?
-An interrupt is a mechanism in microprocessors that allows the processor to stop its current execution and respond to a specific event, like a button press or the completion of an operation. It's called an interrupt because it disrupts the ongoing task, forcing the processor to handle the interrupt before returning to its previous task.
What are the key components of an interrupt system in a microcontroller?
-The key components of an interrupt system in a microcontroller include the interrupt hardware (like the SysTick timer in the TivaC MCU), the interrupt line that signals the CPU, and the CPU itself, which has a special hardware mechanism for detecting and responding to interrupts, often by preempting the current instruction.
How does the SysTick timer work in generating interrupts?
-The SysTick timer is a 24-bit down-counter in the TivaC MCU. It decrements every CPU clock cycle and can generate an interrupt when it reaches zero. The counter then reloads from the STRELOAD register and starts counting again. This allows for periodic interrupts at regular intervals.
What is preemption in the context of interrupts?
-Preemption refers to the process where the CPU is forced to stop executing the current instruction and immediately jump to handle the interrupt. This ensures that the interrupt is serviced without delay, even if it occurs in the middle of executing another instruction.
What is the role of the SysTick->LOAD register?
-The SysTick->LOAD register determines the interval between interrupts. By writing a value to this register, the programmer can control how often the SysTick timer generates an interrupt, thus creating periodic events based on the desired time interval.
Why is it important to subtract 1 when setting the SysTick->LOAD register?
-It is important to subtract 1 when setting the SysTick->LOAD register because the SysTick counter counts down to zero. Without subtracting 1, the counter would generate one more interrupt than expected, as the counter includes zero in its count.
What is the function of the __enable_interrupt() call in the program?
-The __enable_interrupt() call is used to clear the PRIMASK bit in the CPU, which blocks interrupts. By clearing this bit, interrupts are enabled, allowing the CPU to respond to interrupt signals such as those generated by the SysTick timer.
What happens when the code is executed after the interrupt system is set up?
-When the interrupt system is set up and the code is executed, the red LED toggles every second, as the SysTick timer generates an interrupt every half-second. The program enters the infinite loop, and the interrupt handler toggles the LED each time the interrupt is triggered.
Outlines
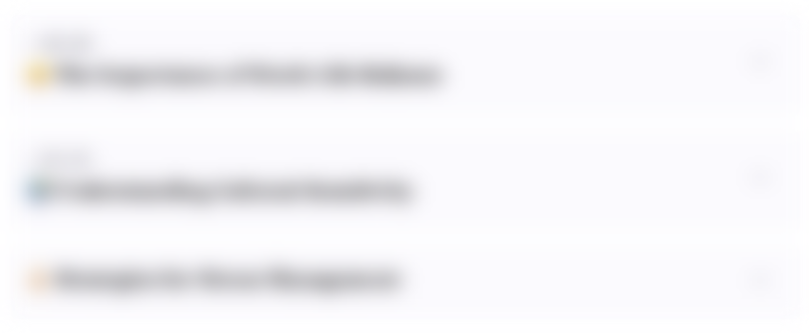
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
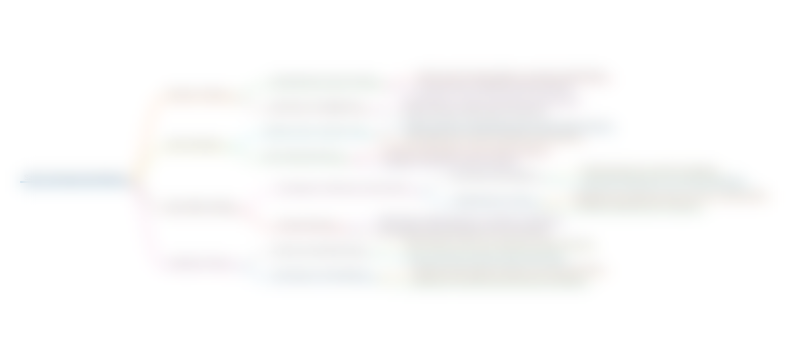
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
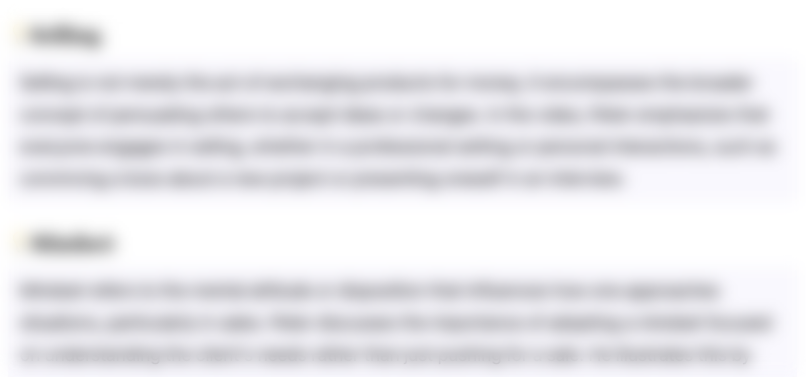
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
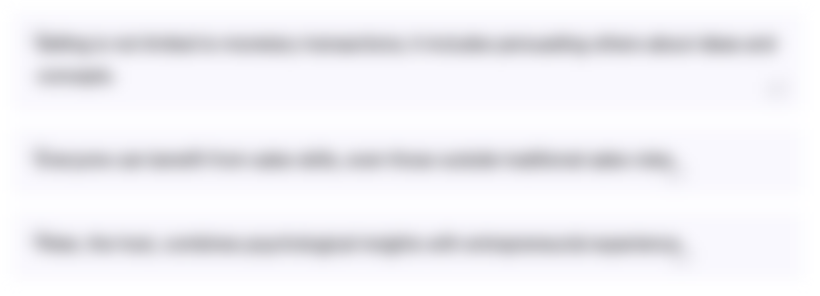
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
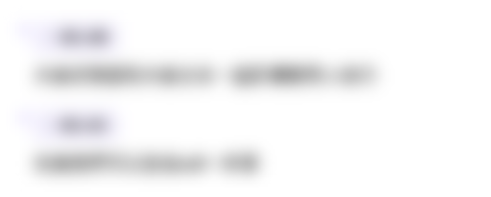
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
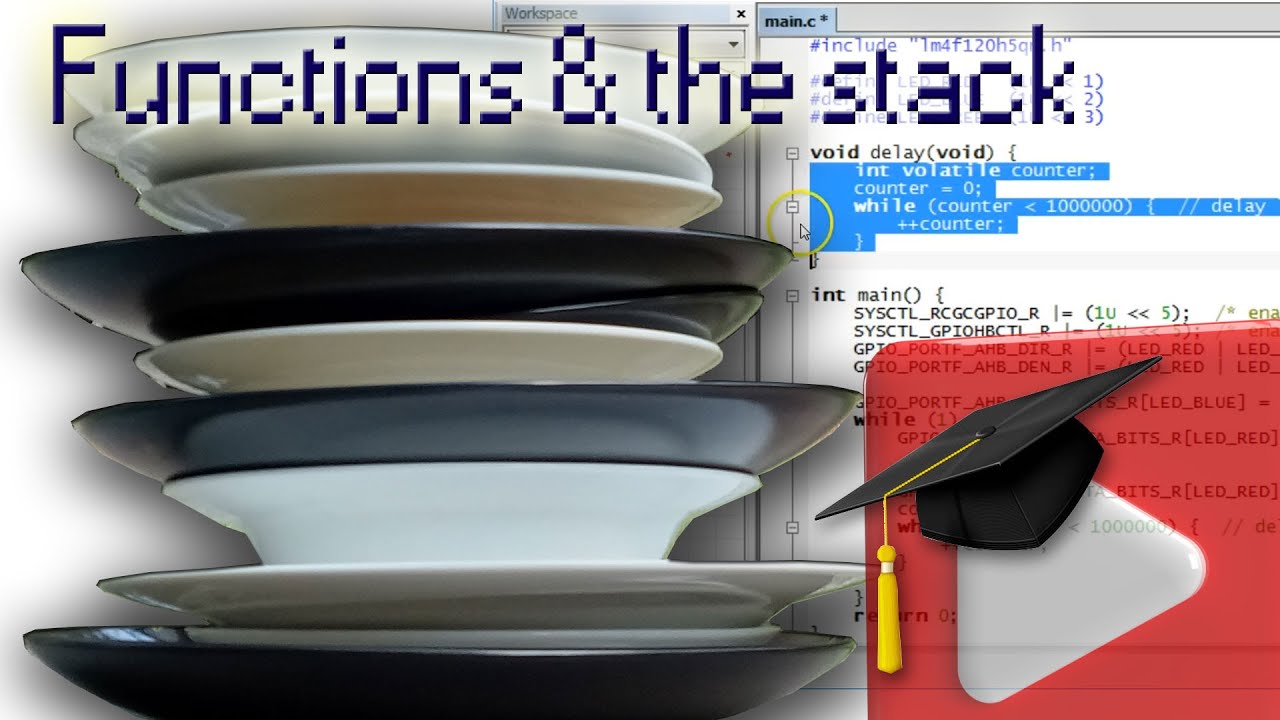
#8 Functions in C and the call stack
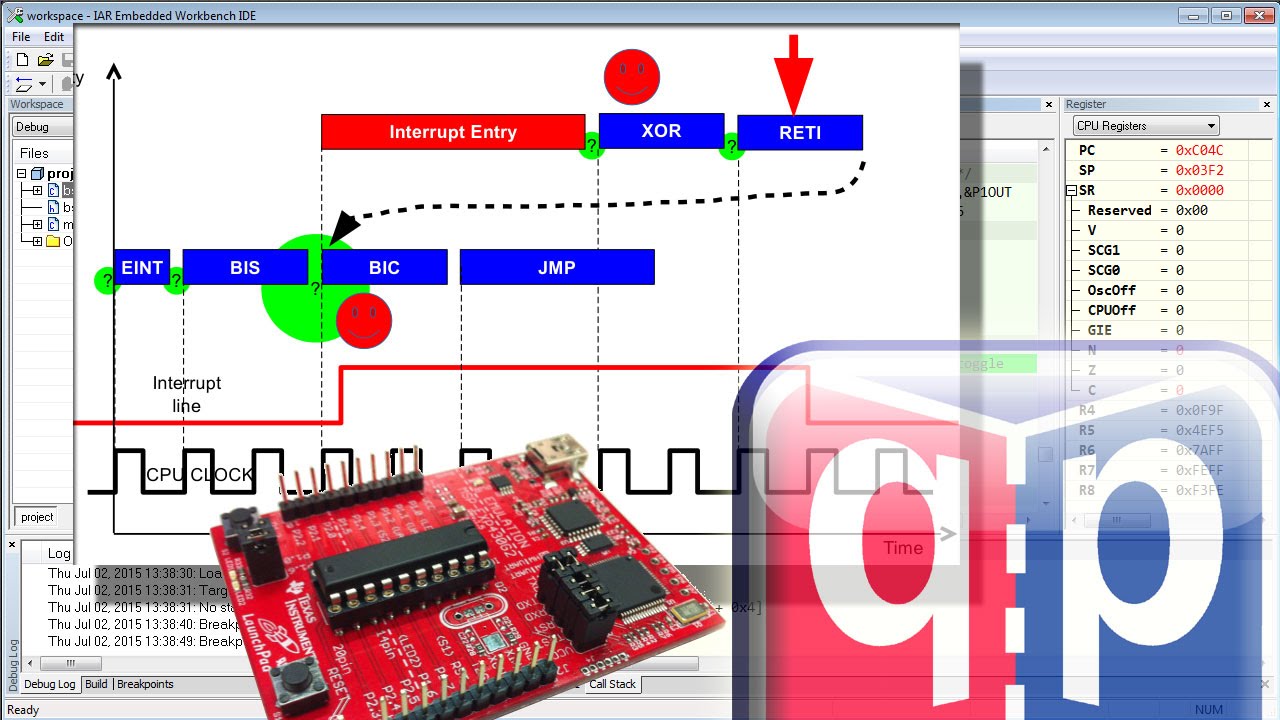
#17 interrupts Part-2: How most CPUs (e.g. MSP430) handle interrupts?
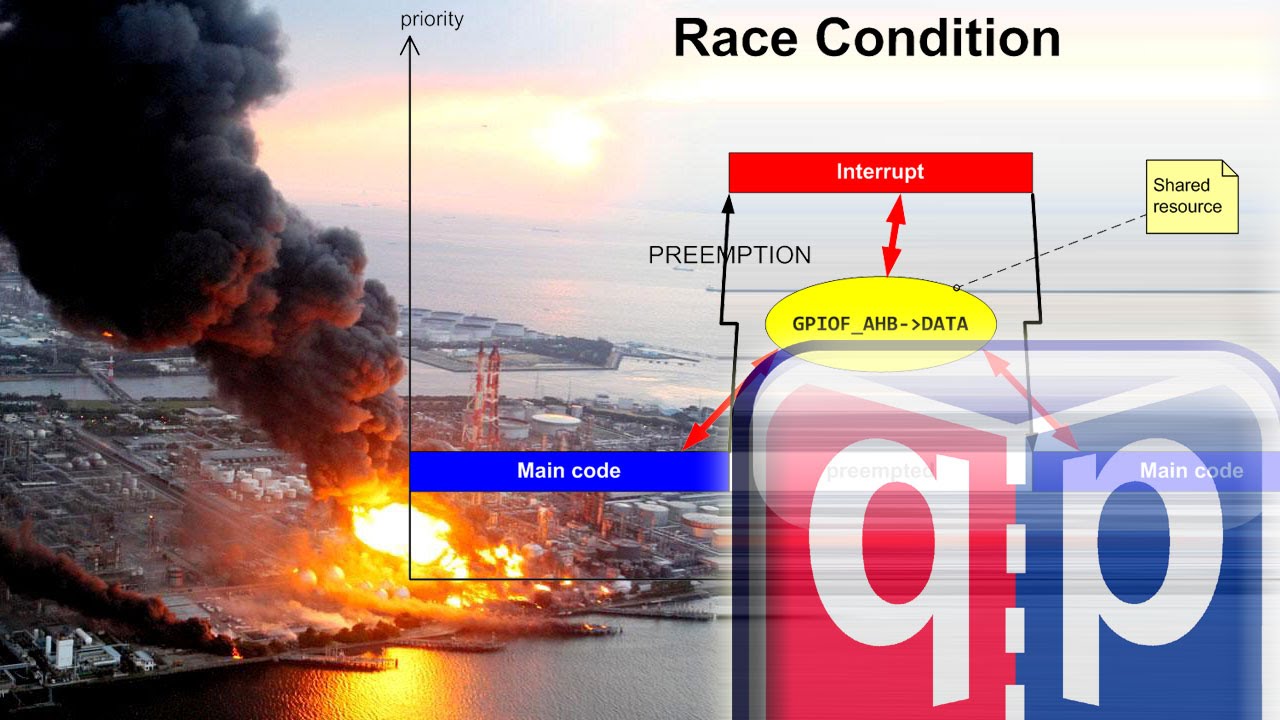
#20 Race Conditions: What are they and how to avoid them?
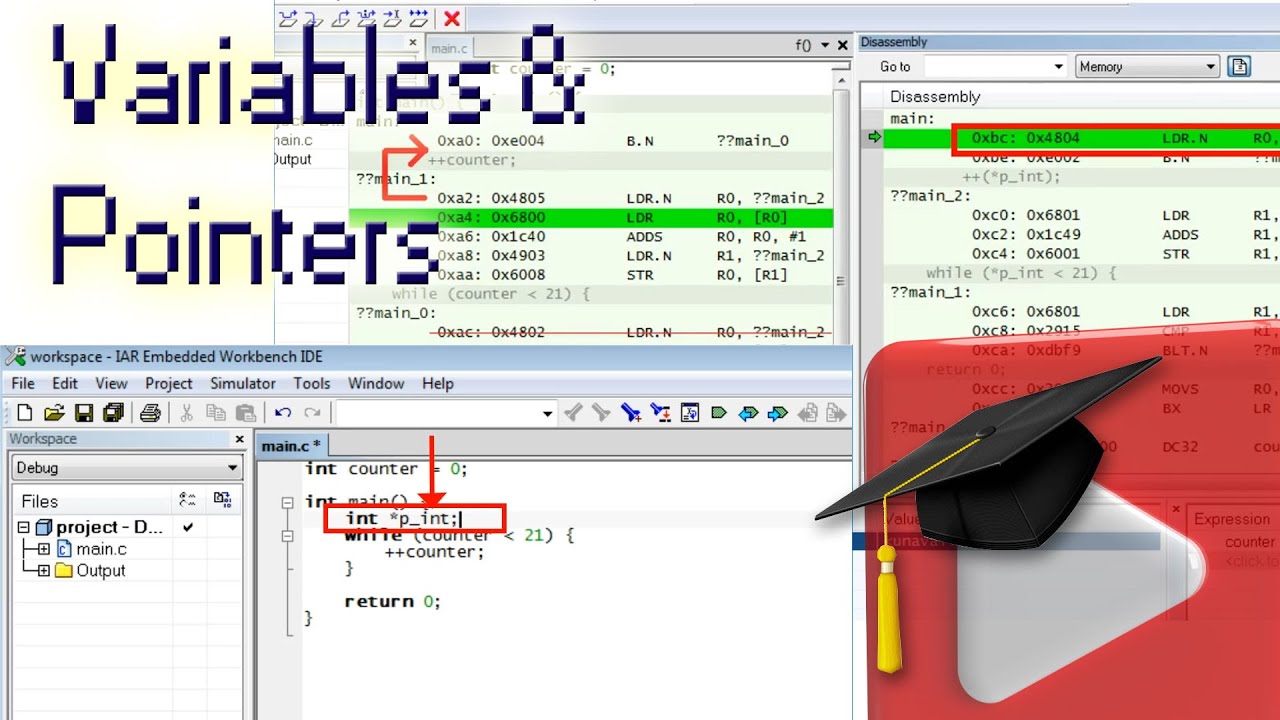
#3 Variables and Pointers
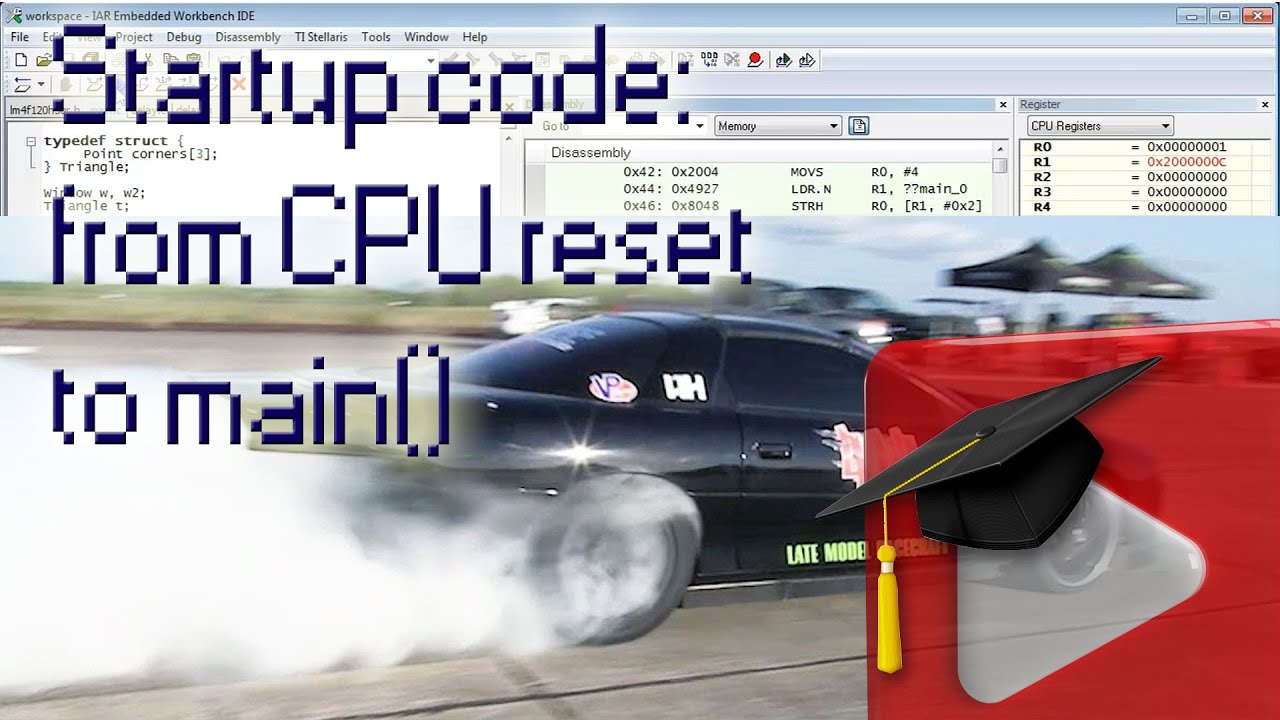
#13 Startup Code Part-1: What is startup code and how the CPU gets from reset to main?
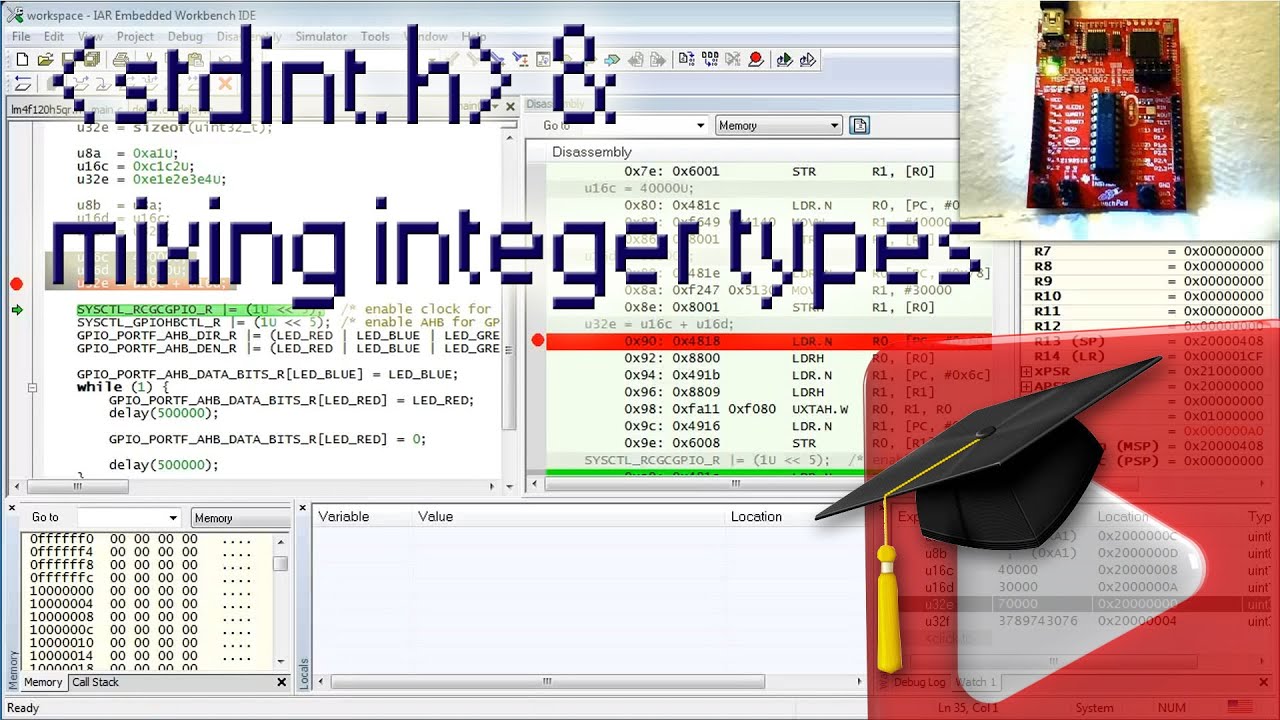
#11 Standard integers (stdint.h) and mixing integer types
5.0 / 5 (0 votes)