Belajar Java [OOP] - 22 - Latihan Inheritance
Summary
TLDRThis tutorial provides a hands-on guide to understanding inheritance in Java, focusing on creating and working with hero classes. The speaker walks through building two hero objects with unique attributes such as attack power and health, and demonstrates how one class can inherit from another. Key topics include class creation, constructors, methods for displaying attributes, and implementing attack and defense mechanisms. The tutorial also briefly introduces the concept of polymorphism, offering a practical approach to understanding object-oriented programming in Java, with a gaming context that involves hero characters and combat interactions.
Takeaways
- 😀 The tutorial explains how to create a basic Java program with classes, using a game scenario involving heroes battling each other.
- 😀 It introduces the concept of inheritance in object-oriented programming, where one class (Hero) can extend another (HeroStrange).
- 😀 A key focus is on creating classes with constructors, attributes like attack power and health, and how to display these attributes using methods.
- 😀 The tutorial demonstrates how to initialize objects of classes and call methods to test the setup, showing how heroes' stats (like attack power and health) are displayed.
- 😀 HeroStrange extends Hero and introduces an additional attribute, Armor, which modifies how damage is calculated during a battle.
- 😀 It covers how polymorphism works, allowing HeroStrange to access and modify methods and attributes from its parent class, Hero.
- 😀 The tutorial emphasizes method overriding, explaining how HeroStrange can modify methods from Hero to include unique features (e.g., defense capabilities).
- 😀 A damage calculation system is introduced, where heroes attack one another, and the health of the attacked hero decreases based on the damage value.
- 😀 It shows how to implement methods to handle the attack and defense mechanics between heroes, including calculating the damage taken by each hero.
- 😀 The tutorial concludes by highlighting how polymorphism is central to allowing different types of heroes to interact within the same framework, with potential for future enhancements like adding new hero types.
Q & A
What is the primary concept demonstrated in this tutorial?
-The primary concept demonstrated is inheritance in Java, where one class (Hero) is extended by another class (HeroStrength), showing how to create subclasses and reuse methods and attributes from a superclass.
How does inheritance work between Hero and HeroStrength classes?
-The HeroStrength class extends the Hero class, inheriting its attributes like attack power, health, and methods. This allows HeroStrength to use the properties of Hero while adding or modifying functionality, such as additional attributes like armor.
What role does the constructor play in the Hero class?
-The constructor in the Hero class initializes the hero's attributes like name, attack power, and health, ensuring that every Hero object has these properties set up when it's created.
What is the purpose of the 'display' method in the Hero class?
-The 'display' method is used to print out the hero's information, including the hero's name, attack power, and health, helping to show the state of the Hero object.
How does method overriding come into play in this tutorial?
-Method overriding occurs when a subclass (HeroStrength) provides its own implementation of a method that was originally defined in the superclass (Hero), like the 'display' method, to add more specific behavior (e.g., showing the hero type).
What is the significance of polymorphism in the context of this tutorial?
-Polymorphism is introduced when discussing how a superclass reference (Hero) can point to a subclass object (HeroStrength). It allows the program to use methods of the subclass while treating the object as an instance of the superclass.
How does the attack system between heroes work?
-The attack system involves one hero (Hero1) calling a method to attack another hero (Hero2). The attacked hero's health is then reduced based on the attack power of the attacking hero.
What is the 'takeDamage' method used for?
-The 'takeDamage' method is used to decrease the health of a hero by a certain amount of damage, typically the attack power of the attacking hero.
How does the armor attribute in HeroStrength modify damage received?
-The armor attribute in HeroStrength reduces the damage received. For example, if the hero has armor, only half of the damage (50%) is applied to the health, thus reducing the amount of health lost.
What would happen if the HeroStrength class did not override the 'takeDamage' method?
-If the HeroStrength class did not override the 'takeDamage' method, the method from the Hero class would be used, meaning no armor reduction would occur, and the full damage would be applied to the health.
Outlines
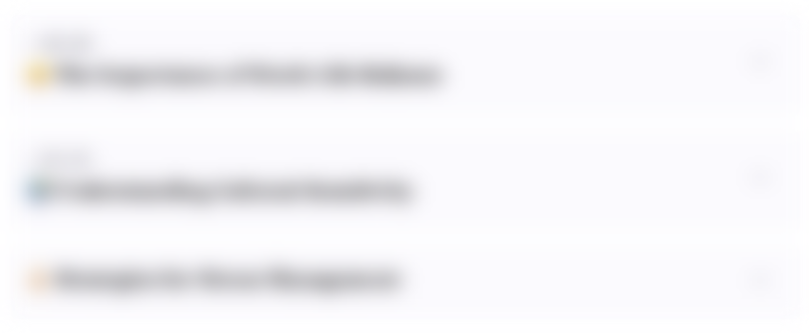
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
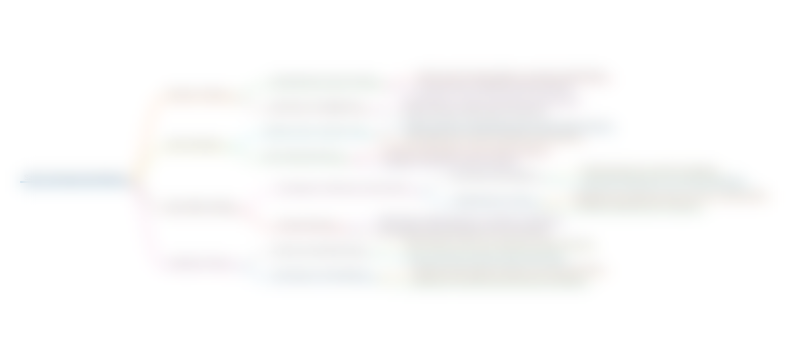
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
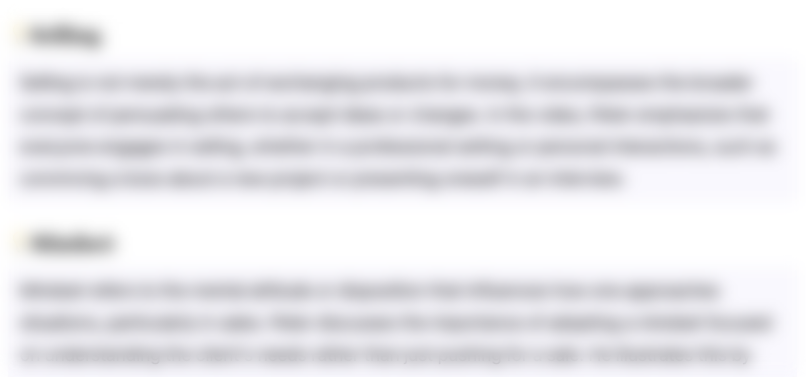
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
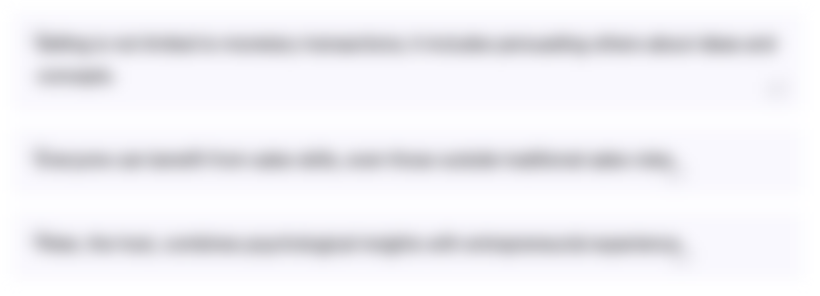
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
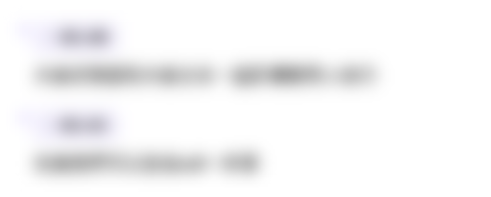
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
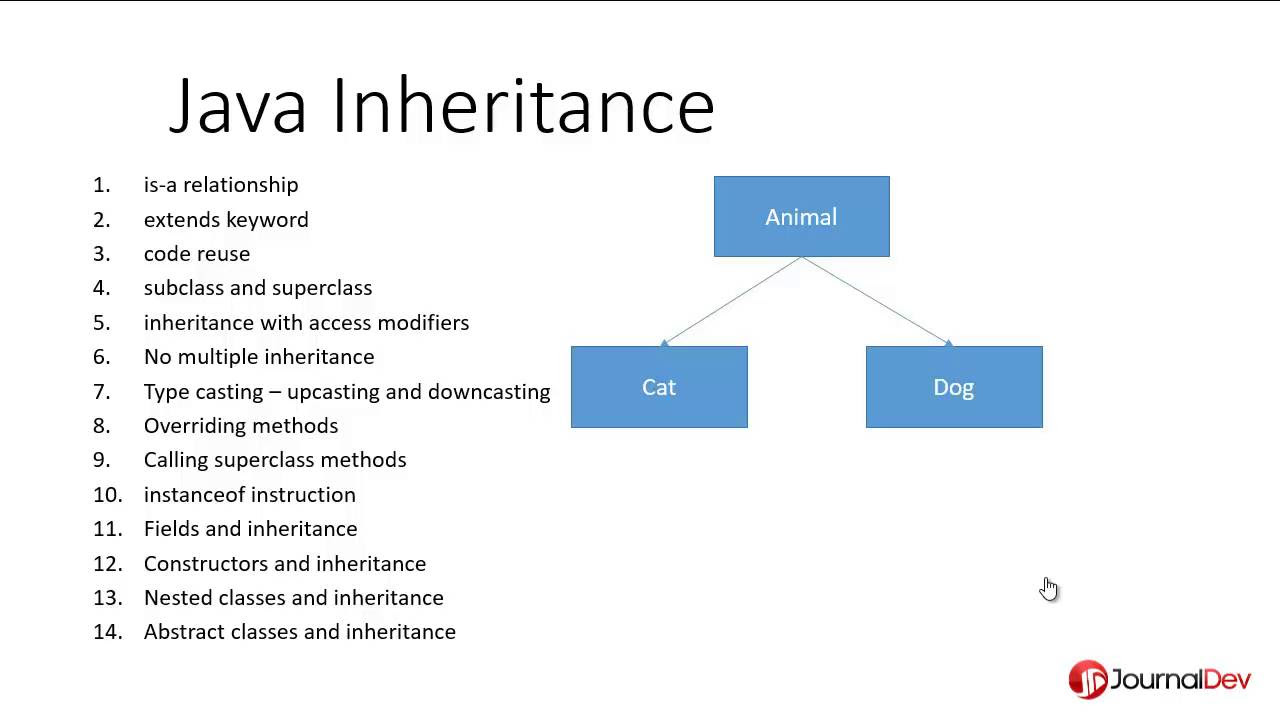
Inheritance in Java - Java Inheritance Tutorial - Part 2
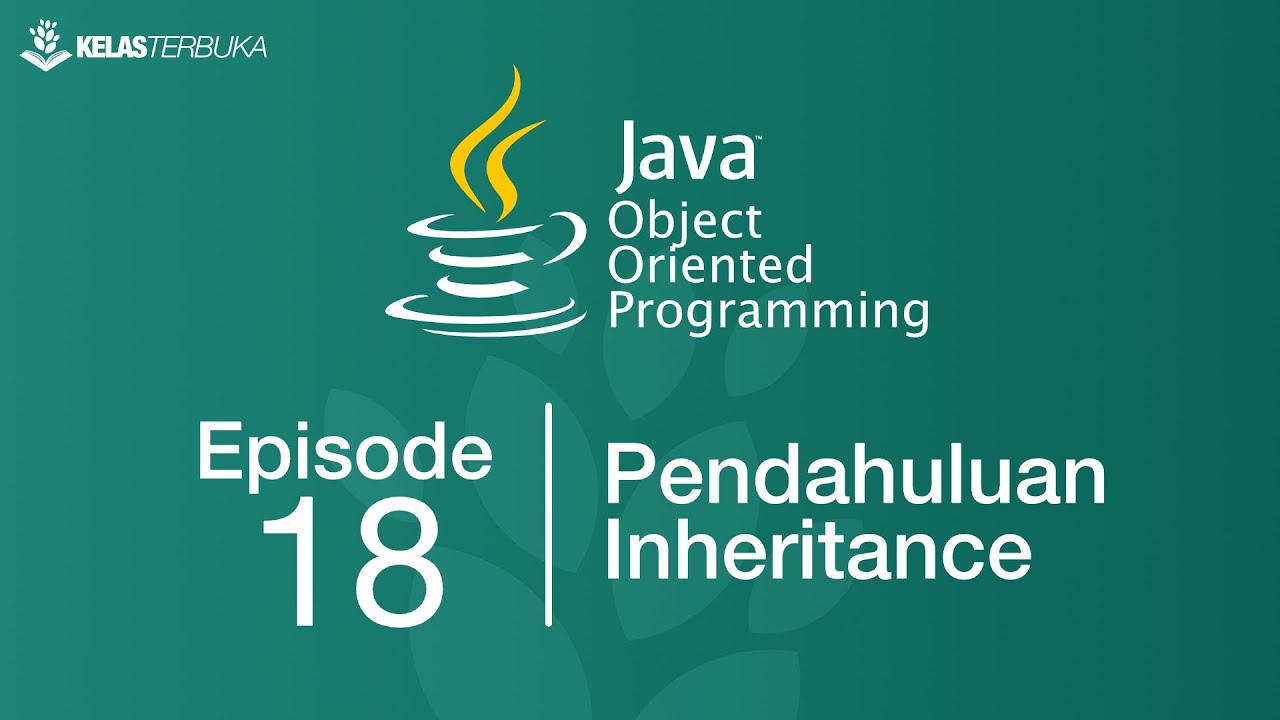
Belajar Java [OOP] - 18 - Pengenalan Inheritance
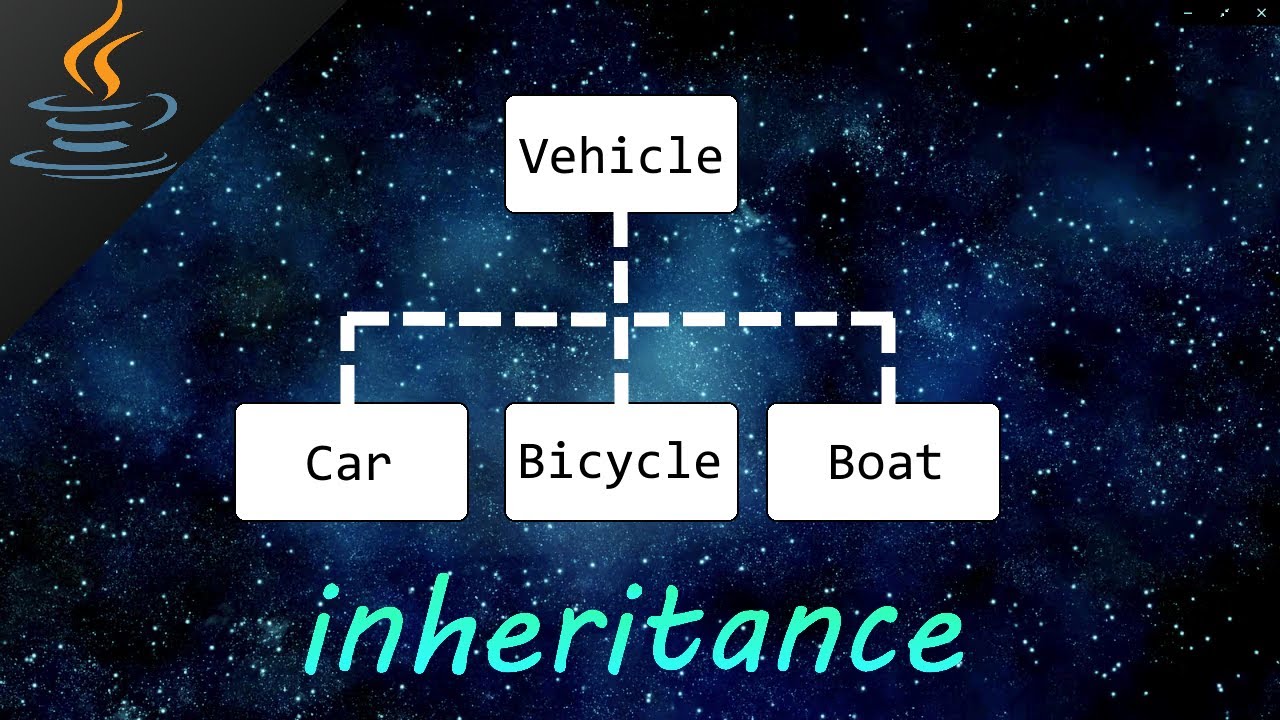
Java inheritance 👪
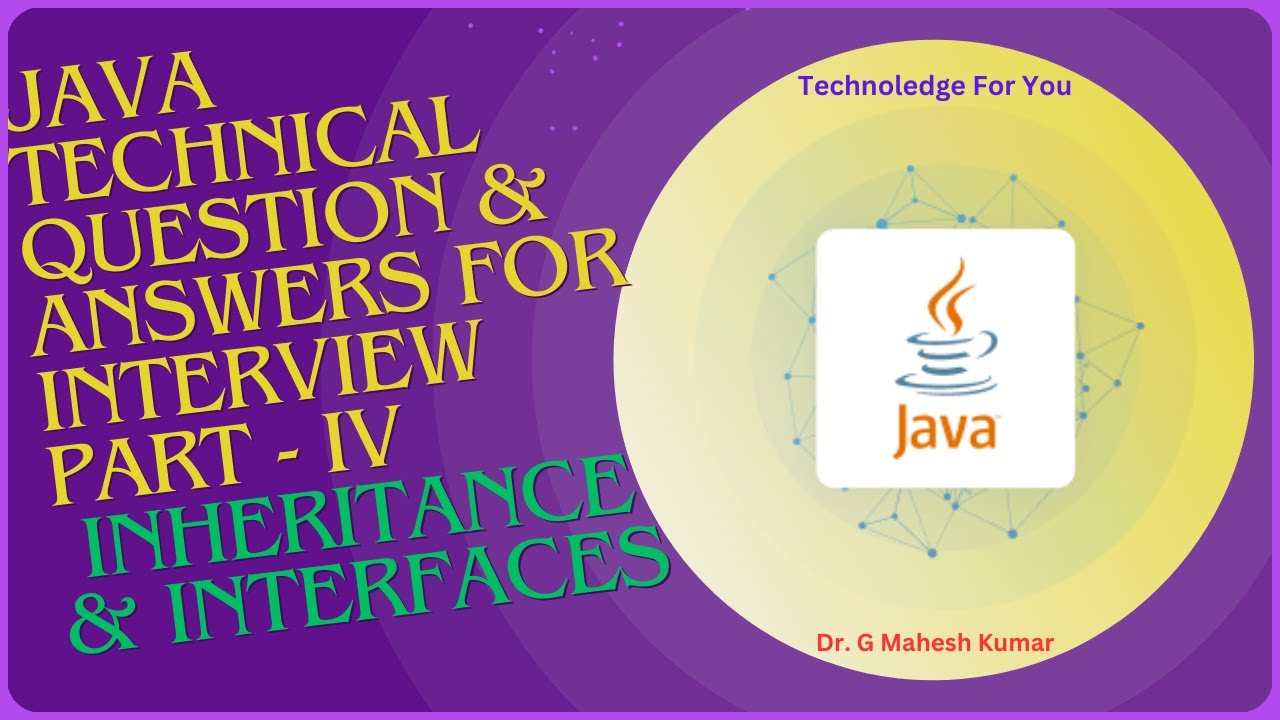
JAVA TECHNICAL QUESTION AND ANSWERS FOR INTERVIEW PART IV INHERITANCE & INTERFACES

Java Inheritance | Java Inheritance Program Example | Java Inheritance Tutorial | Simplilearn
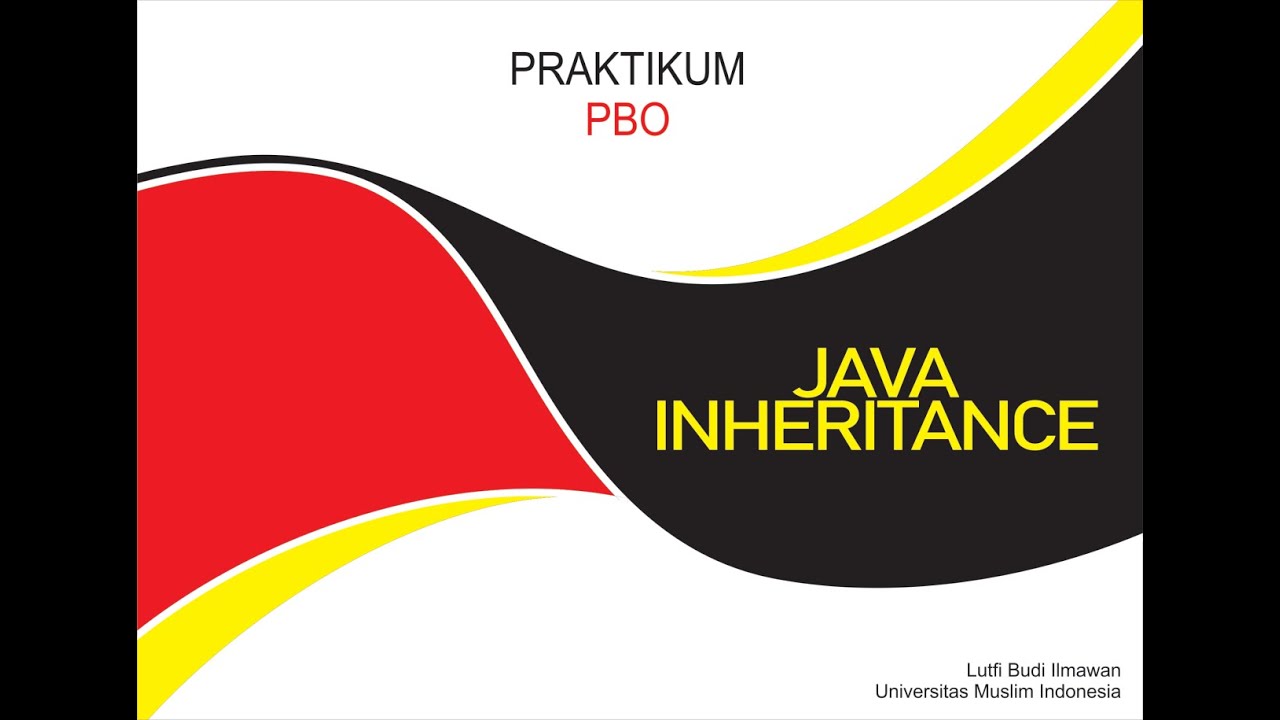
Praktikum 2 PBO - Java Inheritance
5.0 / 5 (0 votes)