How variables works in Python | Explained with Animations
Summary
TLDRThis video explains how variables work in Python, contrasting it with other languages like C or Golang. It highlights Python's dynamic typing and memory management system, emphasizing the concept of variables as references to objects in memory. The script covers topics like reference counts, garbage collection, container data types, and interning of objects like integers and strings. It also discusses function argument passing, augmented assignment operators, and the mutability of objects. The video provides a deep dive into Python's memory model and explains key behaviors related to variable references, memory management, and object mutability.
Takeaways
- 😀 Variables in Python are references to objects in memory, not containers holding values like in other languages.
- 😀 Python objects are dynamically typed, meaning variables can reference objects of different types during runtime.
- 😀 In Python, memory management includes reference counting and garbage collection to manage unused objects.
- 😀 The assignment operator in Python creates a new reference to an object rather than copying the object itself.
- 😀 Lists in Python store references to elements in an internal array, allowing different types of objects to be stored in the same list.
- 😀 Python caches or interns commonly used objects like integers (between -5 and 256) and certain strings to optimize memory usage.
- 😀 The 'is' keyword in Python compares memory addresses, while '==' compares the values of objects.
- 😀 When passing mutable objects (e.g., lists) to functions, changes within the function affect the original object unless reassigned.
- 😀 Using mutable objects as default parameters in functions can lead to unintended side effects due to shared references.
- 😀 The augmented assignment operator (e.g., '+=') mutates objects in-place and does not change the reference of the variable pointing to the object.
Q & A
What is the difference between how variables are handled in Python and other languages like C or Golang?
-In languages like C or Golang, variables are associated with specific memory locations that hold the actual values, and the type of the variable dictates the memory size. In Python, however, everything is treated as an object, and variables are simply references to these objects in memory.
Why is Python considered a dynamically typed language?
-Python is dynamically typed because the type of the value is associated with the object itself, not the variable. This allows variables to reference different types of objects, such as integers, strings, or lists, without needing explicit type declarations.
What does the 'reference count' of an object in Python signify?
-The reference count tracks how many references (variables) are pointing to a specific object. When the reference count reaches zero, meaning no variable refers to the object, it is eligible for garbage collection.
How does Python handle memory allocation for immutable objects like integers and strings?
-When you create an immutable object like an integer or a string, Python creates the object in memory and associates it with a variable. The memory address of the object is fixed, and multiple variables can reference the same object, especially for small integers or commonly used strings, which are often interned.
What happens when you assign a new value to an existing variable in Python?
-When a new value is assigned to an existing variable, a new object is created in memory at a different address. The variable is then updated to reference the new object, and the old object’s reference count is decremented. If the reference count reaches zero, the object is garbage collected.
What is the purpose of Python's garbage collection process?
-Garbage collection in Python automatically frees up memory by removing objects that are no longer referenced, ensuring efficient memory management and preventing memory leaks.
How does the memory model work for Python lists?
-In Python, lists are container objects. The list object holds pointers to the actual elements, which are stored in heap memory. When elements are added to a list, new objects are created for the new elements, and the list's size field is updated. The list object itself never changes its memory address, but the backing array of elements may be moved if the array becomes full.
What does the 'is' operator do in Python?
-'is' compares the memory addresses of two objects to check if they are the same object in memory, whereas '==' compares the values of the objects. For instance, two different list objects with the same elements will return 'True' for '==' but 'False' for 'is'.
How does Python handle default arguments in functions, particularly with mutable types?
-In Python, default argument values are evaluated when the function is defined. If a mutable object like a list is used as a default argument, it may lead to unexpected behavior because the default object is shared across all calls. A better approach is to use 'None' as a default and create a new object inside the function if needed.
Why does the '+=' operator behave differently with mutable objects like lists compared to immutable objects like integers?
-The '+=' operator calls the object's __iadd__ method, which modifies the internal state of mutable objects like lists in place. For immutable objects like integers, however, the operator creates a new object and rebinds the variable to this new object.
Outlines
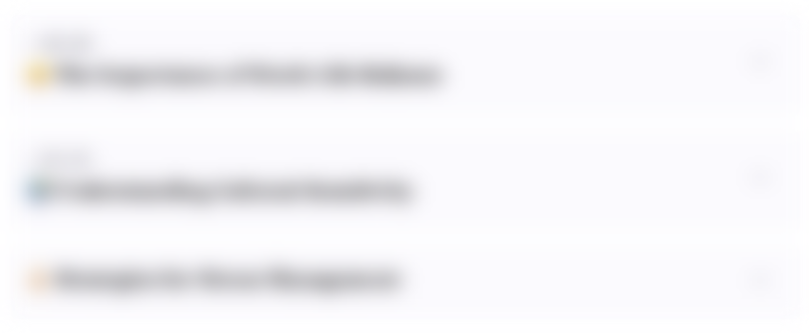
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
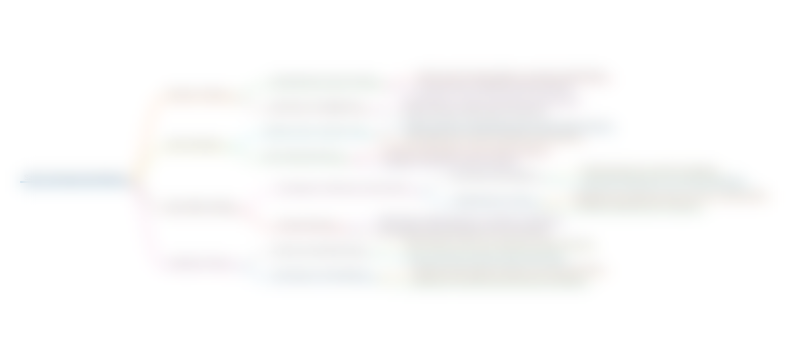
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
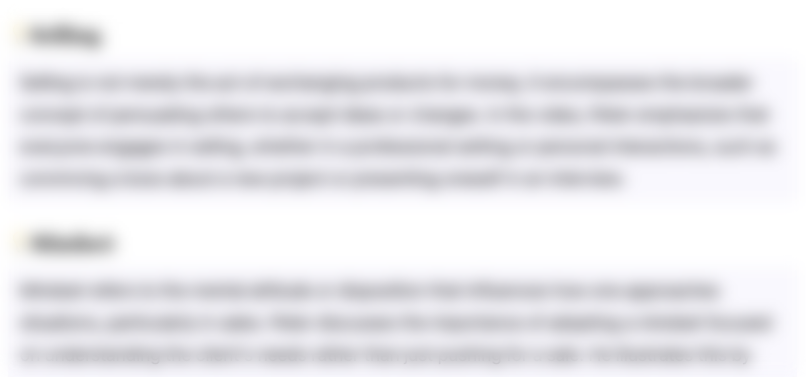
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
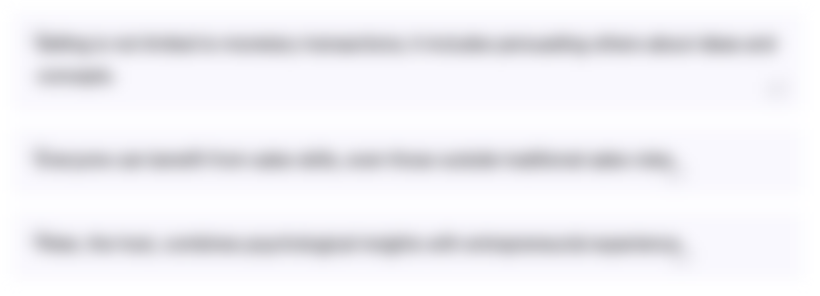
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
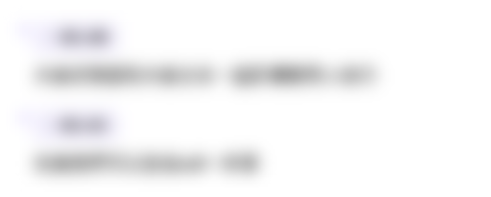
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频

Python series launch | chai aur python for beginners

Start using this Go design pattern.. Consumer Interfaces!

💻 ¿QUÉ ES UN LENGUAJE DE PROGRAMACIÓN Y PARA QUÉ SIRVE? 💻 | lenguaje de programación que es.

C 語言入門 | 01 - 01 | C 語言入門課程簡介
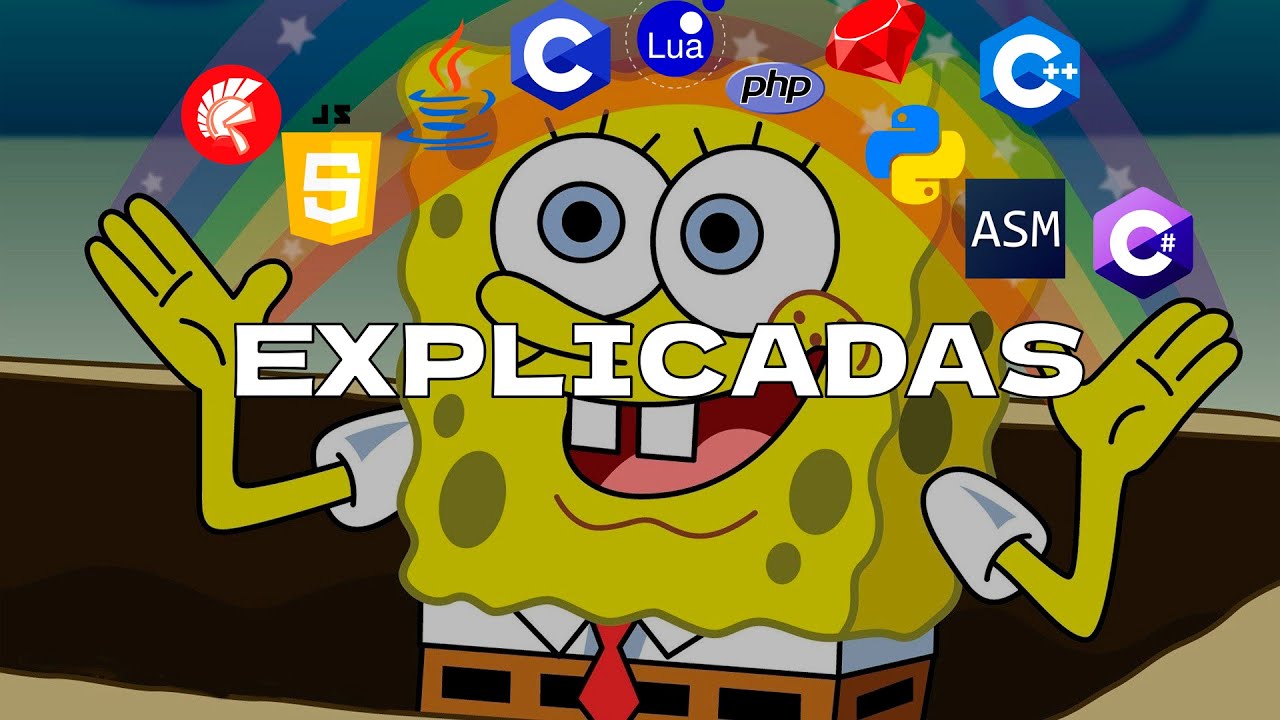
Conheça 13 linguagens de programação em 6 minutos
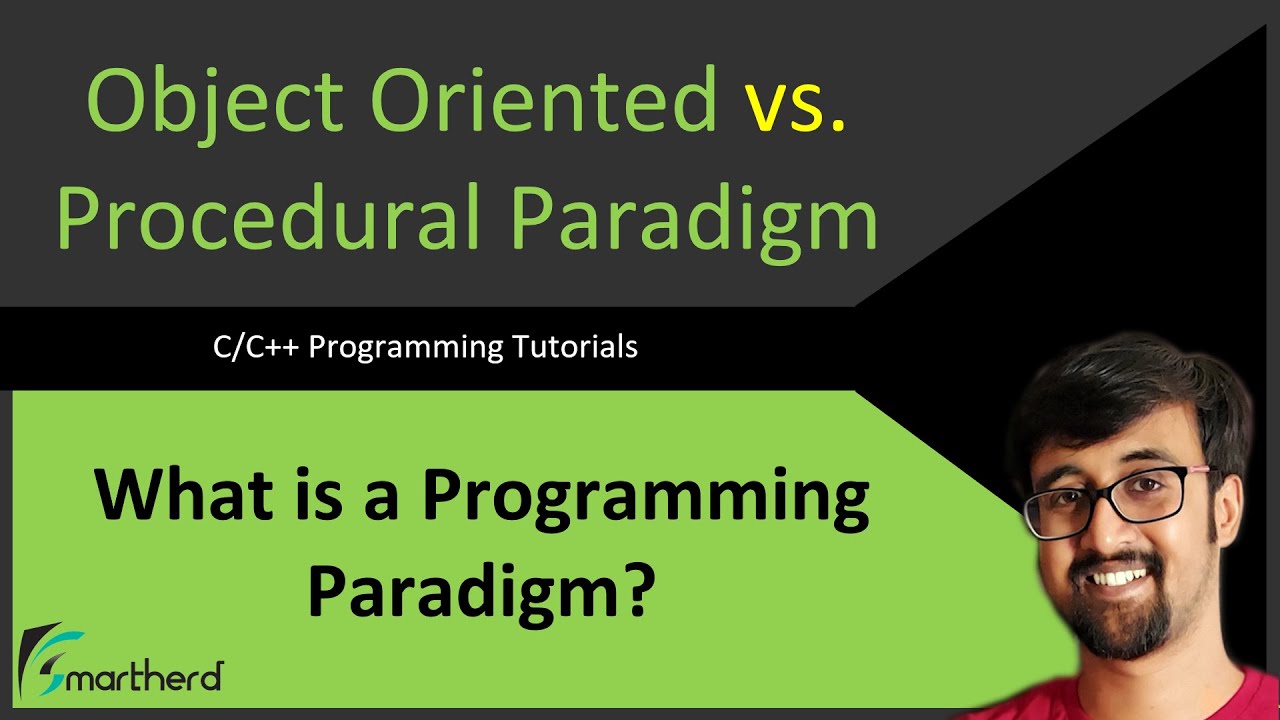
Object Oriented vs. Procedural Programming Paradigm
5.0 / 5 (0 votes)