Python для ЕГЭ. Массивы с нуля. Снова цикл for и работа с массивами.
Summary
TLDRThis video provides a comprehensive guide to Python lists, covering their fundamental properties and key operations. It explains how lists can store various data types, the concept of indexing starting from 0, and how to manipulate lists by adding or removing elements. The tutorial also introduces Python’s `for` loop for iterating over list items and highlights essential list functions like `append()` and `del`. Additionally, the video covers handling errors, understanding list lengths, and modifying list elements, making it an essential resource for beginners wanting to work effectively with lists in Python.
Takeaways
- 😀 Lists in Python are versatile data structures that can hold multiple items, including numbers, strings, and other lists.
- 😀 Python lists start indexing from 0, meaning the first element is accessed with index 0, not 1.
- 😀 You can use negative indexing to access elements from the end of the list, with -1 referring to the last element.
- 😀 Lists can hold different types of data (e.g., integers, strings, and even other lists) in a single structure.
- 😀 To add new elements to a list, you can use the `append()` method, which adds items at the end of the list.
- 😀 The `len()` function can be used to determine the number of elements in a list.
- 😀 You can delete elements from a list using methods like `del()` or by specifying an index to remove.
- 😀 Using a `for` loop in Python, you can iterate through a list, processing or printing its elements one by one.
- 😀 The `for` loop can also use a step argument to skip elements, allowing you to iterate at regular intervals.
- 😀 Python's list operations are efficient, making it easy to manage large datasets with a variety of data types.
- 😀 The script touches on list comprehensions, which offer an advanced way to create and manipulate lists more concisely, although this will be covered in future lessons.
Q & A
What is a list in Python?
-A list in Python is a collection of items that can hold elements of different data types, such as numbers, strings, and even other lists.
How do you create a list in Python?
-A list can be created by placing items inside square brackets, separated by commas, like this: `my_list = [1, 2, 3, 4, 5]`.
Can a Python list contain elements of different data types?
-Yes, a list in Python can store different types of data, such as integers, strings, and even other lists or objects.
What is list indexing in Python?
-In Python, list indexing starts from 0. The first element of the list is accessed using index 0, the second with index 1, and so on.
What is negative indexing in Python lists?
-Negative indexing allows you to access list elements starting from the end. For example, `my_list[-1]` gives the last element, `my_list[-2]` gives the second-to-last element, and so on.
How do you append a new element to a list in Python?
-You can add a new element to a list using the `append()` method. For example, `my_list.append(10)` will add `10` to the end of the list.
What happens if you try to access an index that is out of range in a Python list?
-If you try to access an index that doesn't exist in the list, Python will raise an `IndexError`, indicating that you're trying to access an invalid index.
How do you remove an element from a list by index?
-You can remove an element from a list by its index using the `del` statement. For example, `del my_list[2]` removes the element at index 2.
What is the purpose of a `for` loop when working with lists in Python?
-A `for` loop allows you to iterate over each element in a list, performing operations on each item. You can use `for` to print all items, modify elements, or execute any other action on each list element.
How can you find the number of elements in a list?
-You can find the number of elements in a list using the `len()` function. For example, `len(my_list)` returns the number of items in `my_list`.
Outlines
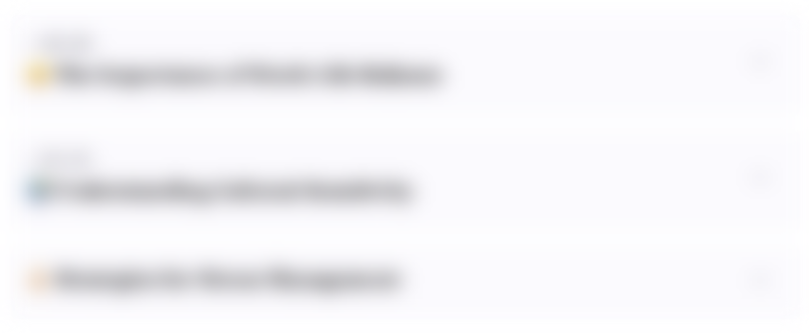
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
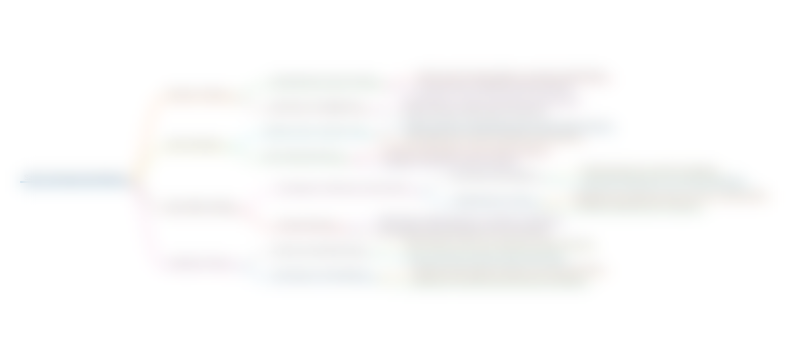
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
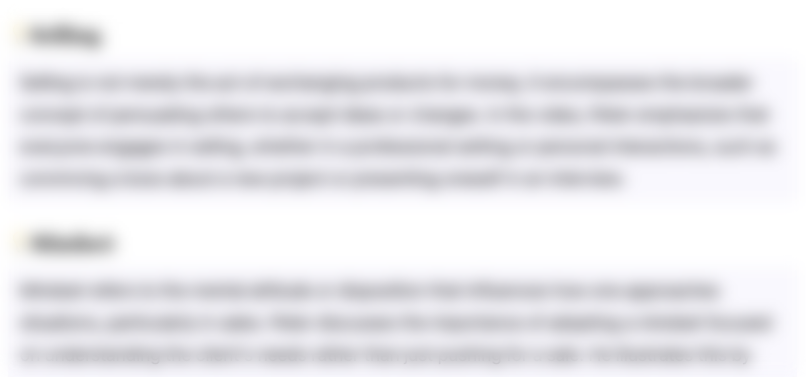
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
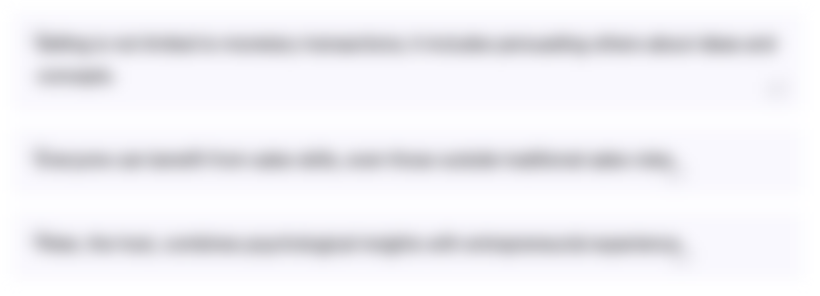
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
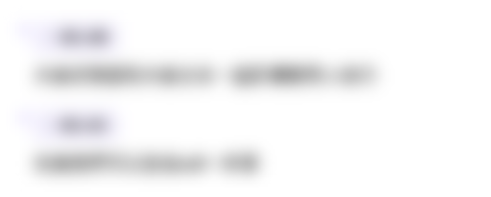
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
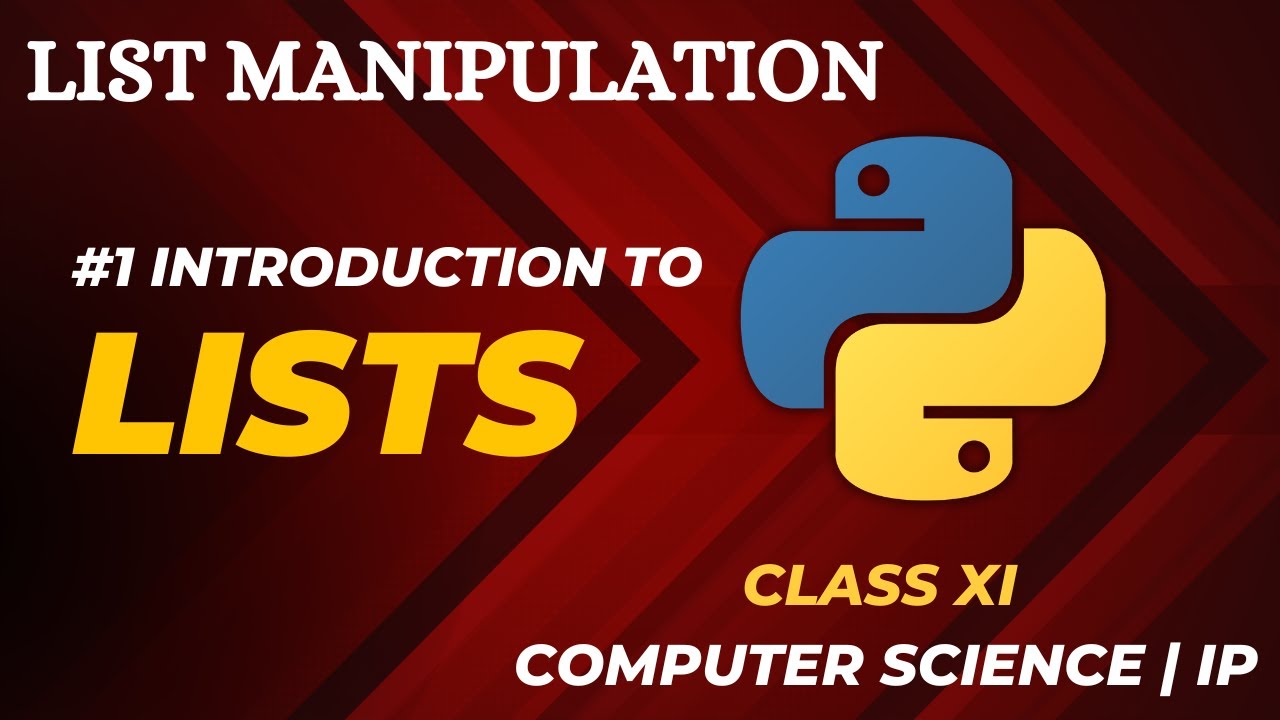
#1 Introduction to Lists | List Manipulation | Class 11 CBSE Computer Science and IP

Introduction To Lists In Python (Python Tutorial #4)
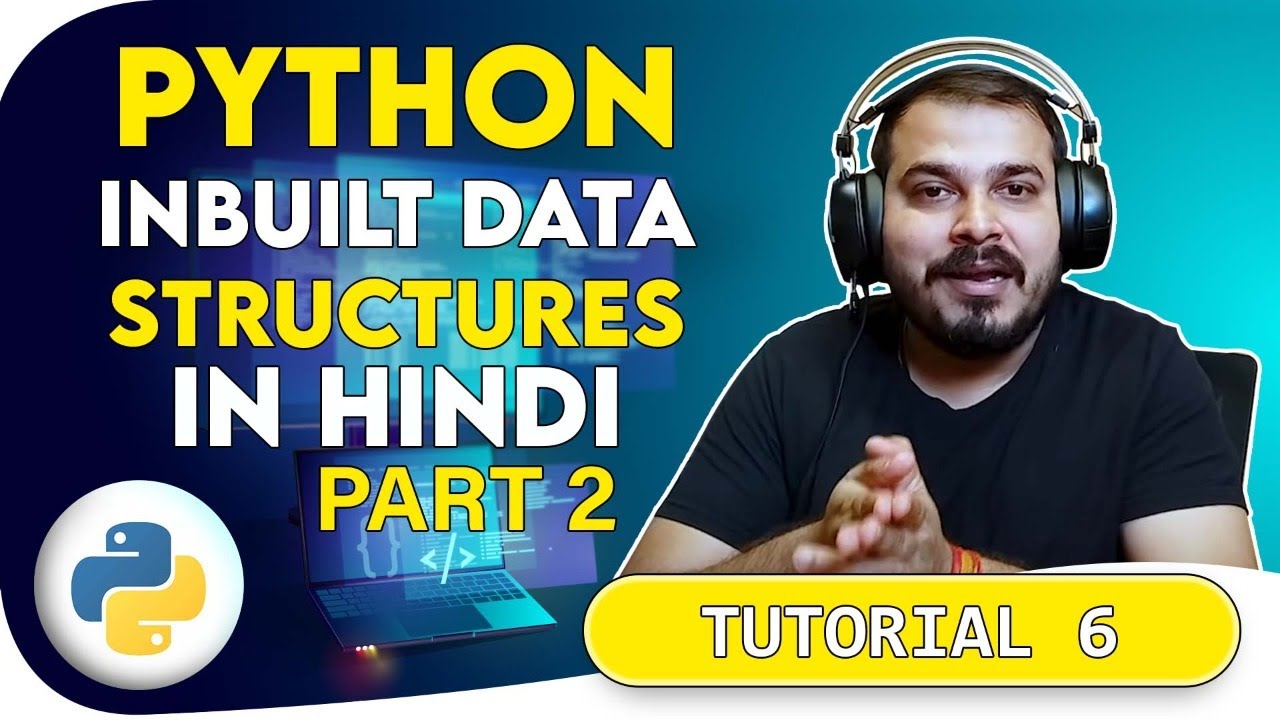
Tutorial 6- Python List And Its Inbuilt Function In Hindi

Accessing Elements of a List in Python
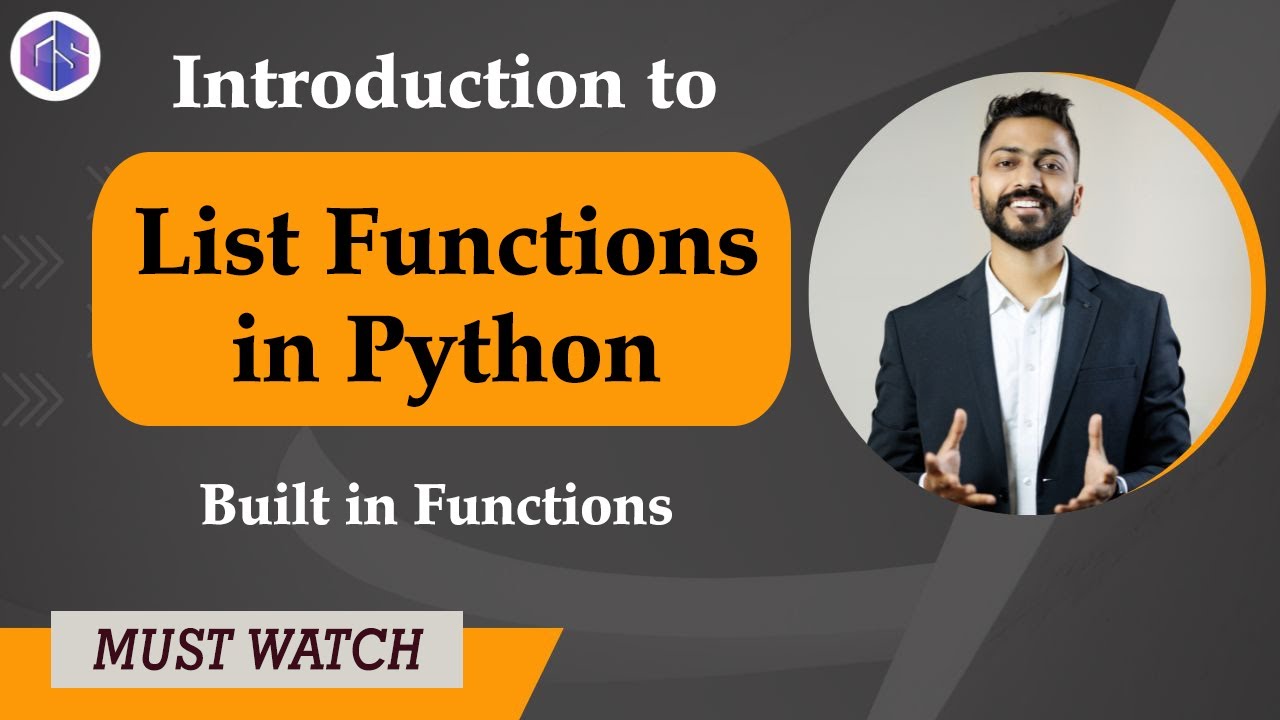
Lec-22: List Functions In Python 🐍 | Built in Functions Python 🐍
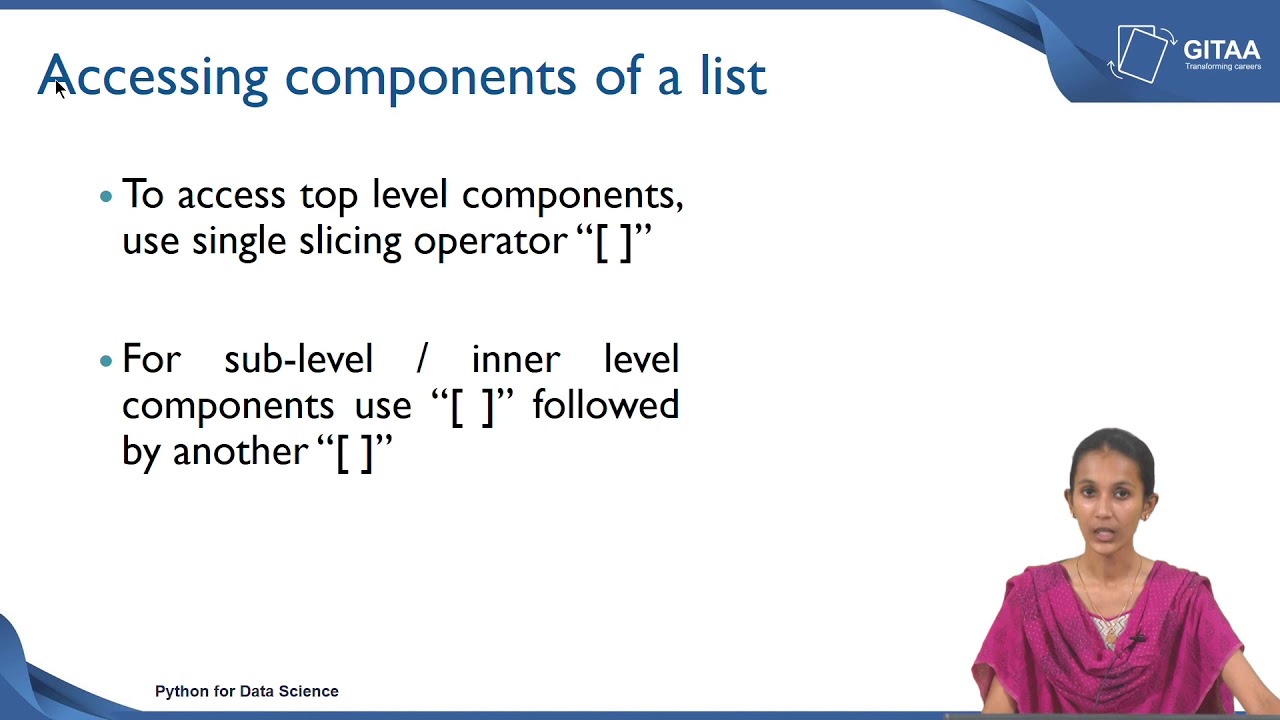
Lists Part- 1
5.0 / 5 (0 votes)