Creating a Single Linked List (Part 1)
Summary
TLDRIn this presentation, the speaker explains how to create a single linked list in C programming. They revisit how to create a node and focus on building a linked list with three nodes containing values 45, 98, and 3. The first node's link points to the second node, the second to the third, and the third's link is set to NULL. The speaker highlights the importance of using an additional pointer, 'current,' to avoid losing the reference to the first node. They also demonstrate how to update the link of the first node to connect it to the second node.
Takeaways
- 📋 The presentation focuses on creating a single linked list in C programming.
- 📚 The speaker reminds the audience that a previous presentation covered how to create a single node of the list.
- 🔗 The goal of the presentation is to create a linked list consisting of three nodes, with data values of 45, 98, and 3.
- 📌 The first node’s link part should point to the second node, and the second node’s link part should point to the third node, while the third node’s link part should be NULL.
- 🔍 A head pointer is necessary to point to the first node of the list.
- ⚙️ The process starts by creating a node structure using `struct node`, containing both data and a link part.
- 💡 The malloc function is used to allocate memory for the nodes, and the head pointer holds the address of the first node.
- 🚨 A problem arises when the head pointer is updated to point to the second node, causing the first node's reference to be lost.
- 🔄 The solution is to use an additional pointer (current) to keep track of the second node, while the head pointer continues to point to the first node.
- ✅ After this adjustment, the first node is linked to the second node by updating the link part of the first node.
Q & A
What is the primary focus of this presentation?
-The presentation focuses on how to create a single linked list in C programming, specifically covering the creation of multiple nodes and linking them together.
What is a single linked list?
-A single linked list is a data structure consisting of nodes where each node contains data and a link to the next node. The last node's link points to NULL, indicating the end of the list.
What data does the example linked list contain?
-The example linked list contains three nodes with the data values 45, 98, and 3.
How is memory allocated for a node in the linked list?
-Memory for a node is allocated using the `malloc` function in C. The size of the memory allocated is equal to the size of the `struct node`, which contains both data and the link part.
Why is it necessary to use a head pointer in the linked list?
-The head pointer is necessary because it points to the first node of the linked list, providing access to the entire list. Without it, the list cannot be traversed or modified.
What issue arises when creating the second node in the list, and how is it solved?
-When creating the second node, the head pointer is reassigned to point to the second node, causing the reference to the first node to be lost. This is solved by using a new pointer, 'current', to point to the second node, while the head pointer continues pointing to the first node.
How is the first node connected to the second node?
-The first node is connected to the second node by updating its link part to store the address of the second node, which is held in the 'current' pointer.
What is the role of the 'current' pointer in the linked list?
-The 'current' pointer helps in linking the nodes without losing the reference to the head node. It points to the second node (and subsequent nodes), allowing traversal while the head remains fixed on the first node.
What happens to the link part of the third node in the linked list?
-The link part of the third node will contain NULL, indicating the end of the linked list.
What will be covered in the next presentation, according to the script?
-In the next presentation, the third node of the linked list will be created, and it will be added to the list already created in this presentation.
Outlines
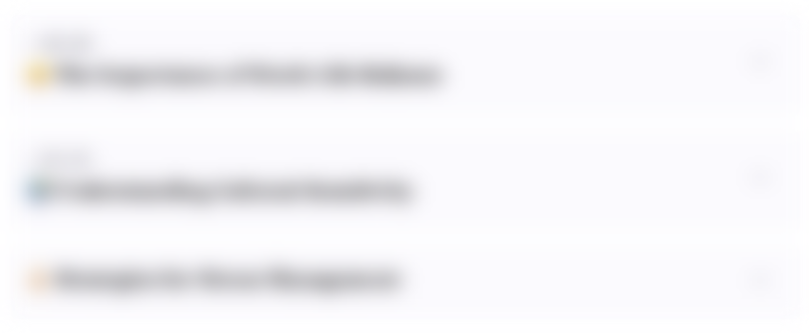
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
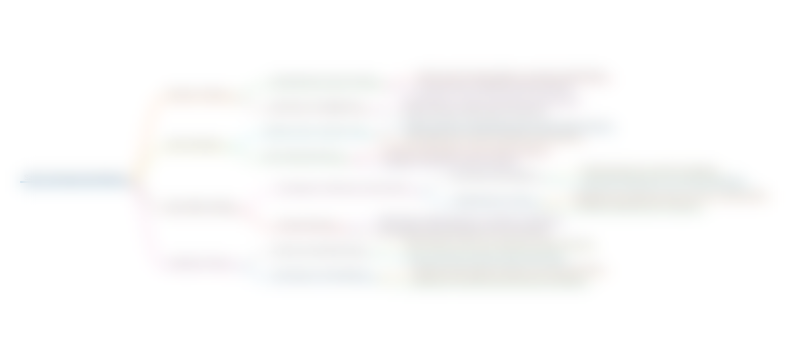
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
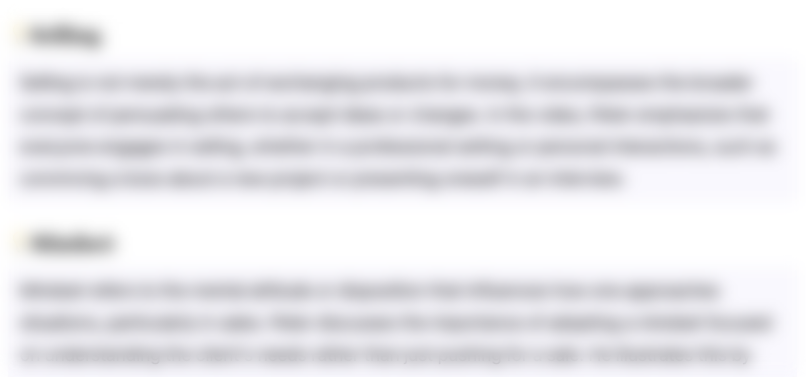
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
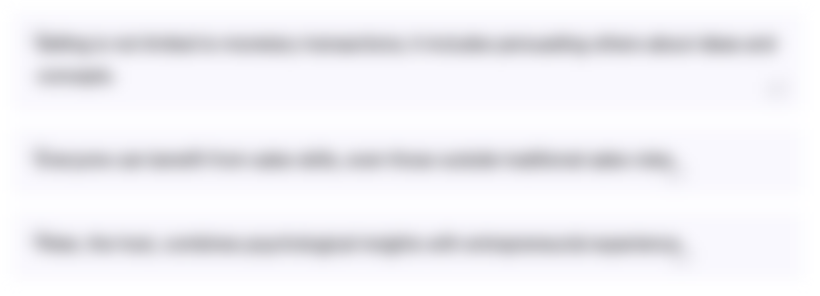
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
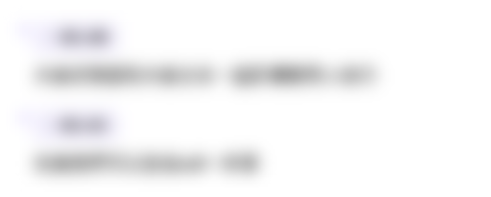
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
5.0 / 5 (0 votes)